This content originally appeared on Level Up Coding - Medium and was authored by Mohamed Hijazi
In many game genres, like strategy games or action games such as Diablo or Desperados, that use an isometric perspective depend on a point-and-click system in order to move the player around.
Create a point and click system in a few simple steps
Firstly in order to create this system, you need to understand that this navigation system depends on a mesh that is called the Navmesh. Basically, the Navmesh allows you the player or the AI to intelligentially navigate through the scene. So the character will automatically calculate the path it needs to take to its destination.
After setting up your scene, open Windows > AI > Navigation. In the navigation window, open the Object tab and then select in your hierarchy the plane or terrain that your characters will move on. Make sure to choose “Navigation Static”, then open the Bake tab and bake the mesh.
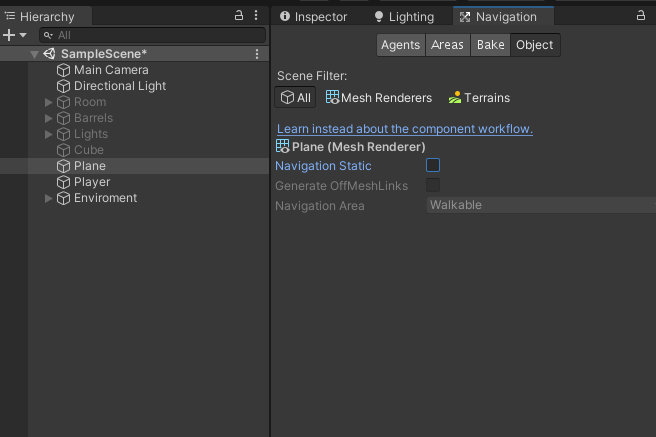
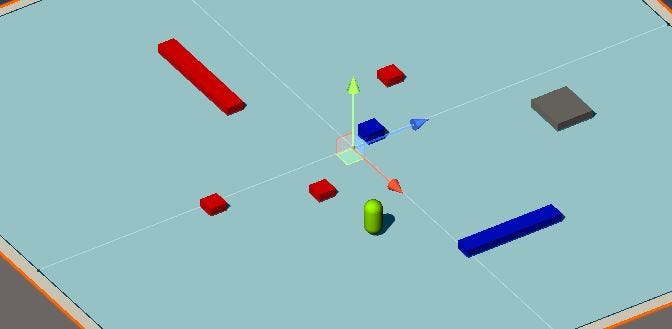
Your scene should now have a blue highlight as to where your character can move using the Navmesh. For more precision, choose the obstacles/objects in your scene that will not move and the character cannot go to, make them as static, and then bake the mesh again.
Player Setup
A. On your player gameObject, add a Nav Mesh Agent Component.
B. Create a C# script and add it to the player.
C. In the script get hold of your NavMeshAgent (you should be using the UnityEngine.AI namespace)
NavMeshAgent _navAgent;
_navAgent = GetComponent<NavMeshAgent>();
// In order for this to work, we need to know where is the mouse clicked on the screen and return the coordinates that will be used for character destination. To do so, we need to use Raycasting.
D. In Update(), check for a mouse click, and declare two variables, one for a RaycastHit and another for a Ray.
if(Input.GetMouseButtonDown(0))
{
RaycastHit hit;
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
}
E. Now we need to check if ray actually hit a collider on the walkable area mesh using the Physics.Raycast method.
if (Physics.Raycast(ray, out hit, 1000, _navAgent.areaMask))
{
_navAgent.SetDestination(hit.point);
}
//out hit returns the info of the collision of the ray
//_navAgent.areMask represents the layermask that are walkable
The final navigation code should be like this:
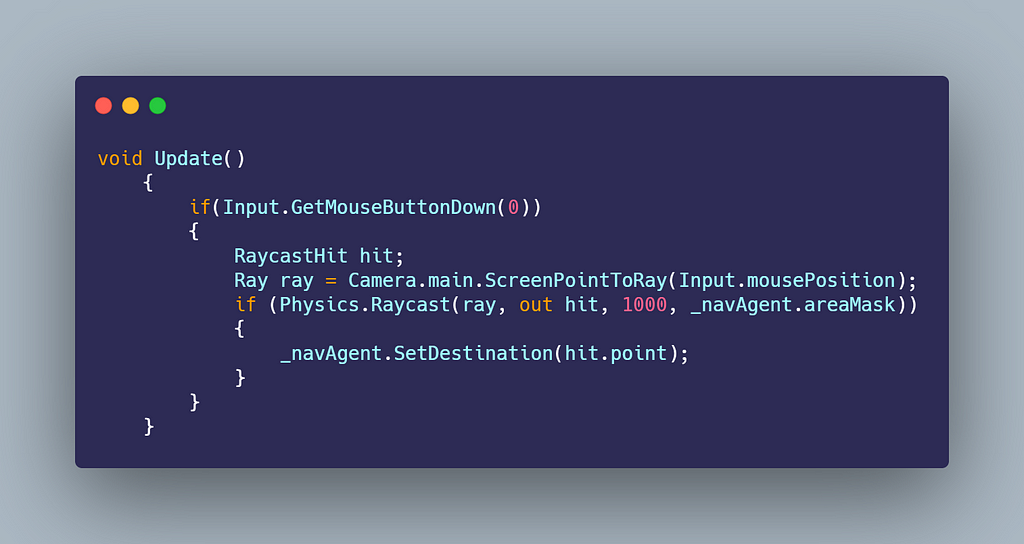
If you want this can be extended to instantiate a mouse click cursor directly on the destination to show the player where he is going for example. And this method can also be used to AI characters to navigate the NavMesh without any input.
Now, the character should be able to calculate the fastest path to its destination.
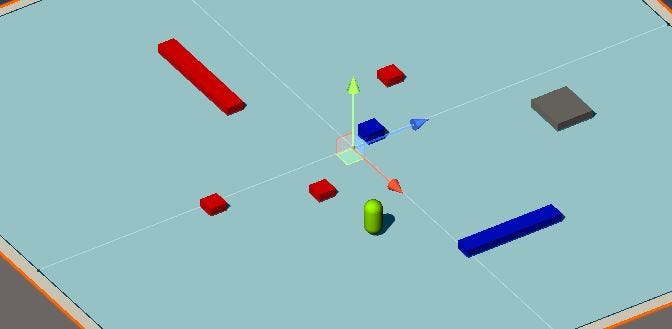
Tip of the Day: Create a Point and Click System in Unity 3D was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Mohamed Hijazi
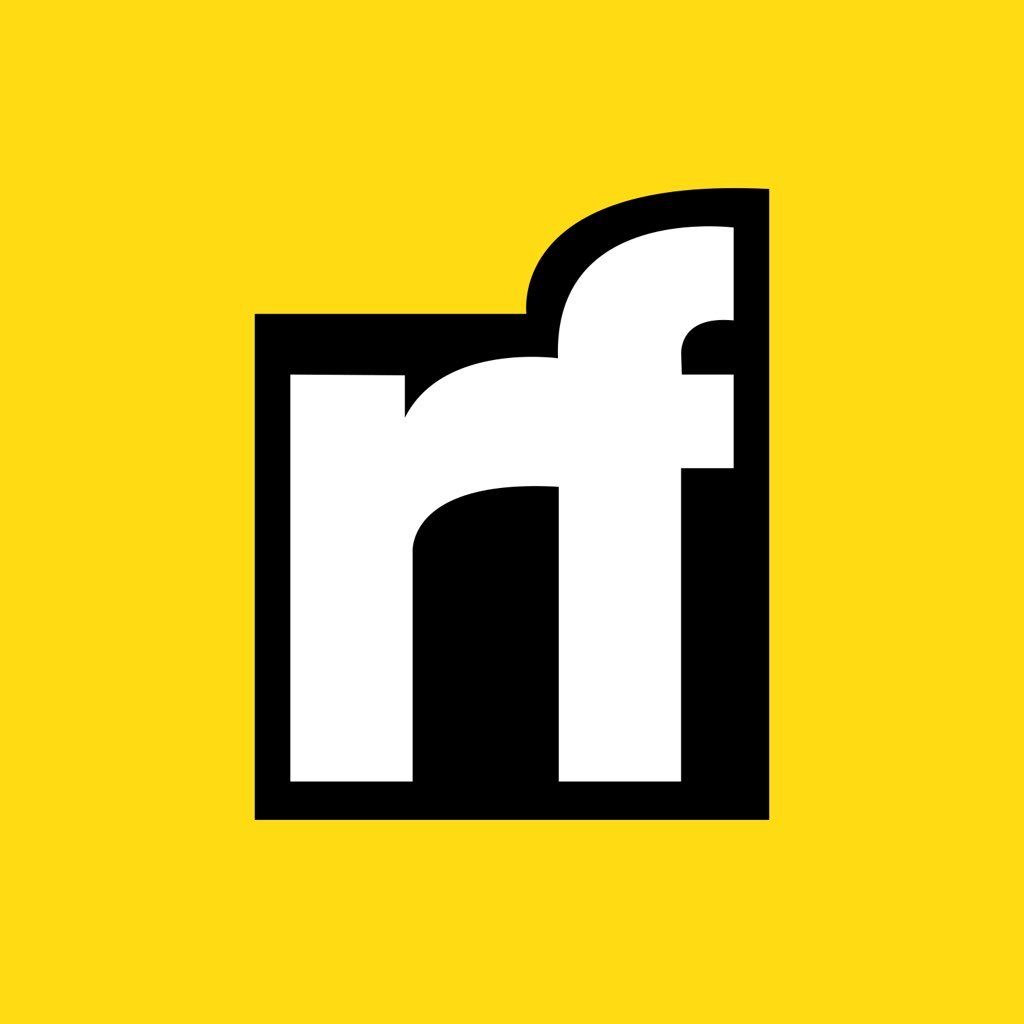
Mohamed Hijazi | Sciencx (2021-04-18T14:43:22+00:00) Tip of the Day: Create a Point and Click System in Unity 3D. Retrieved from https://www.scien.cx/2021/04/18/tip-of-the-day-create-a-point-and-click-system-in-unity-3d/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.