This content originally appeared on Level Up Coding - Medium and was authored by Joshua Mabina
Another Write-up About ES6 Things

Contents
- Let + Const
- Arrows
- Destructuring
- Rest + Spread
- Template Literals
- Promises
- for…of
Let + Const
var in JavaScript is function scoped. let and const bring block-scoped variables to JavaScript i.e. variables, once declared within a block of code, can not be accessed outside it.
let foo = 10;
if (true) {
let foo = 1;
console.log(foo); // 1
}
console.log(foo); // 10
const also ensures that the variable is only assigned once.
const pi = 3.14;
pi = 3.14159 // TypeError: Assignment to constant variable.
Arrows
Arrows are a shorthand for function syntax using an arrow =>, hence the name.
const numbers = [1, 2, 3, 4];
numbers.map((value) => Math.pow(value, 2)); // 1 4 9 16
Unlike whom they represent, this in an Arrow function, remains the same i.e. it remains bound to the parent this.
const foo = { name: 'Batman' };
foo.getUndefinedName = () => this.name; // undefined
foo.getRealName = function () { return this.name; } // Batman
An arrow function does not have its own binding to arguments, and like normal function calls, return undefined for new.target.
ProTip Arrow function expressions are best suited for non-method functions, and they cannot be used as constructors.
Destructuring
Destructuring provides a convenient way to unpack values from arrays or properties from objects. It uses pattern matching, much like the way array/object lookup foo["bar"] works; producing undefined when a property or value is not found.
const [a, b, c, d] = [1, 2, 3];
const {x, y, z} = {x: 1, y: 2};
console.log(a, b, c, d); // 1 2 3 undefined
console.log(x, y, z); // 1 2 undefined
It is also possible to provide default values.
const [a, b = 0, c = 3] = [1, 2];
const { foo, bar = 10} = {foo: 1}
console.log(a, b); // 1 2 3
console.log(foo, bar); // 1 10
Rest + Spread
Rest syntax ... introduces a way to specify that a function can accept zero or more arguments. It allows us to represent an indefinite number of values as function arguments into an array.
function sum (...values) {
return values.reduce((previous, current) => {
return previous + current;
});
}
console.log(sum(1,2,3)); // 6
The spread syntax ... (same as the rest of syntax) allows us to unpack an iterable such as an array or an object.
function sum (x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // 6
Suppose you want to merge the contents of two objects, spread syntax can help us do that as well.
const foo = {a: 1, b: 2};
const bar = {c: 3, d: 4};
const foobar = { ...foo, ..bar};
console.log(foobar); // {a: 1, b: 2, c: 3, d: 4}
ProTip: Where rest structures, spread destructures.
Template Literals
Template literals provide an easy way to embed expressions into strings without the clutter that comes with +concatenation. This is similar to string interpolation as used in other languages like Java, PHP, Python e.t.c.
const foo = 1;
const bar = 2;
console.log(`foo is ${foo}`); // foo is 1
console.log(`foo + bar is ${foo + bar}`); // bar is 3
console.log(`template literals
can also do multilines`);
Promises
Promises allow us to have access to the results of an asynchronous action at a later time. Promises come in place of callbacks i.e. where you would attach a callback you handle a promise. This makes the code less verbose.
Asynchronous code using callbacks:
function onError(error) {
console.error("Error:", error);
}
function onSuccess(result) {
console.log("Result:", result);
}
isEvenAsync(data, onError, onSuccess);
If the above code is re-written using promises, it becomes as simple as:
isEvenAsync(data).then(onSuccess).catch(onError);
Let’s look at how one would go about creating a promise:
let isEvenAsync = (data) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
if ((data % 2) === 0) {
resolve(`${data} is even!`);
} else {
reject(`${data} is not even close!`);
}
}, 1000);
});
}
for…of
ES6 introduces the for...of a statement which likes the for...in the statement makes running through iterables like strings and arrays way easier.
let foo = [1, 2, 3];
for (const value of foo) {
console.log(value);
}
Feels very nice to have run through the foo array without using the classical foo[i] syntax. One other nice thing about the code above is that you can break out of the loop whenever you wish. This has not been the case for the forEach statement introduced with ES5.
The for...of and for...in statements though alike, differ in that, (as seen above) the for...ofiterates over the values of a collection while for...in iterates over the properties of a collection.
let foo = [1, 2, 3];
for (let value in foo) {
value += 1;
console.log(value);
}
// expected output: 01 11 21
let foo = [1, 2, 3];
for (let value of foo) {
value += 1;
console.log(value);
}
// expected output: 0 1 2
ProTip: The for–in statement was designed to work on plain old Objects with string keys. For Arrays, it’s not so great.
Closing Remarks
I really appreciate you for reading this far. I can not ask for much more — if anything, I hope that you have learned something and enjoyed it.
- Frankly, I am only a dwarf standing on the shoulders of giants. Luke Hoban, did a fantastic job with this big list and we can’t b any more proud of Jason Orendorff for diving deeper into the ES6 and its great power, here.
- If you can relate, why not leave your thoughts in the comment section below? I am happy to share with you my train of thought.
- If you know someone who can benefit, why not tweet and tag @joshuamabina? I will appreciate it.
- If you’re feeling a little-extra charitable, why not follow me on Twitter and GitHub? I will appreciate it.
ES6 & Beyond: Gotchas! was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Joshua Mabina
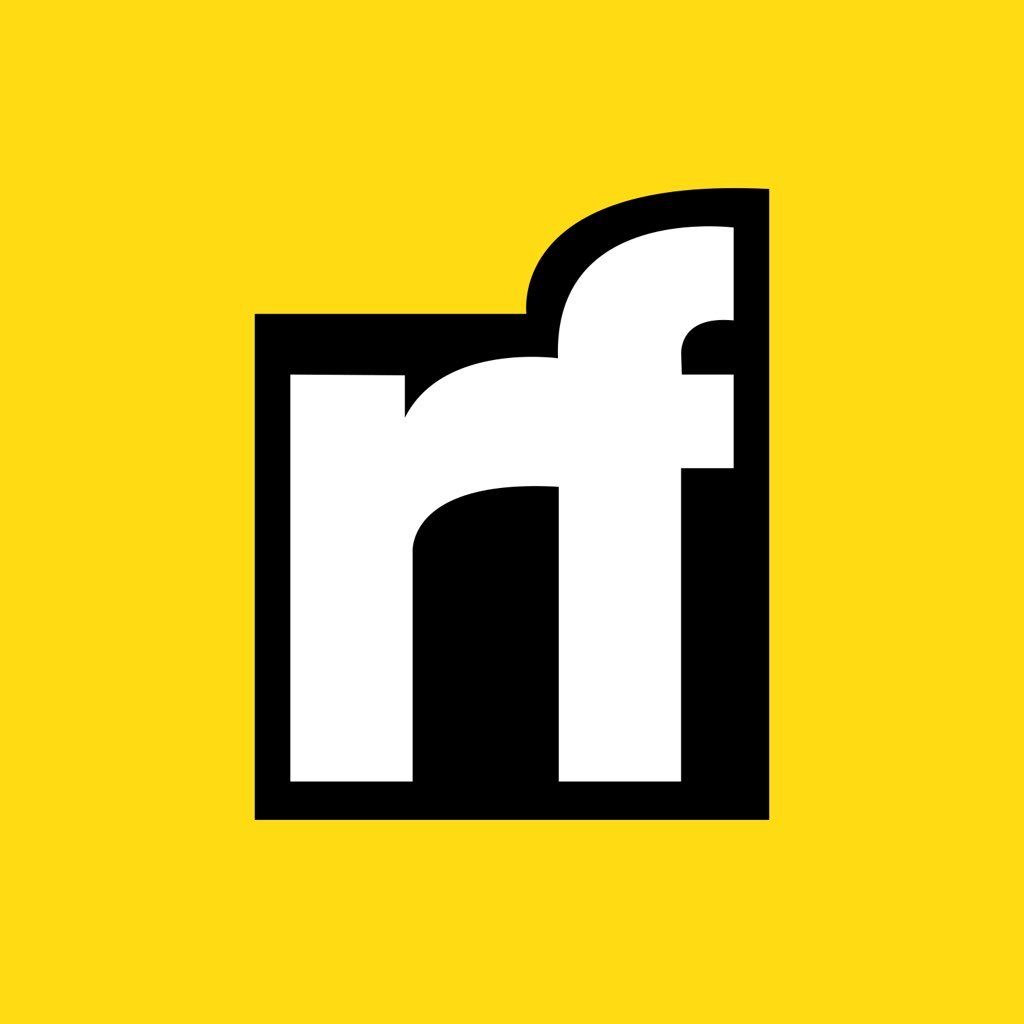
Joshua Mabina | Sciencx (2021-07-21T17:16:01+00:00) ES6 & Beyond: Gotchas!. Retrieved from https://www.scien.cx/2021/07/21/es6-beyond-gotchas/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.