This content originally appeared on DEV Community and was authored by Kush Parsaniya
Java Deadlocks Debugging: A Comprehensive Guide
=====================================================
Java deadlocks are a common issue in multithreaded programs, where two or more threads are blocked indefinitely, waiting for each other to release resources. This can lead to system crashes, performance issues, and even data corruption. In this article, we will delve into the world of Java deadlocks, exploring their causes, detection methods, and strategies for debugging and resolving these issues.
Understanding Deadlocks
A deadlock is a situation where two or more threads are blocked, waiting for each other to release resources. This can occur when threads are competing for shared resources, such as locks, semaphores, or monitors. Deadlocks can be caused by a variety of factors, including:
- Poorly designed synchronization mechanisms: Inadequate synchronization can lead to deadlocks, especially when dealing with multiple threads and shared resources.
- Inadequate resource management: Failing to manage resources effectively can lead to deadlocks, as threads may wait indefinitely for resources to become available.
- Insufficient error handling: Inadequate error handling can mask deadlock issues, making them more challenging to detect and resolve.
Detecting Deadlocks
Detecting deadlocks can be challenging, but there are several tools and techniques that can help identify these issues. Some common methods include:
- Thread dumps: Thread dumps can provide information about the current state of threads, including the resources they are waiting for and the locks they hold.
- Stack traces: Stack traces can help identify the point in the code where the deadlock occurred.
- Profiling tools: Profiling tools can help identify performance issues caused by deadlocks.
Using Thread Dumps to Detect Deadlocks
Thread dumps can be obtained using the jstack
command-line tool or by using a Java IDE's built-in debugging features. Here's an example of a thread dump that indicates a deadlock:
"Thread-1" prio=5 tid=0x00007f93400a0000 nid=0x12f waiting for monitor entry [0x00007f933f610000]
java.lang.Thread.State: BLOCKED (on object monitor)
"Thread-2" prio=5 tid=0x00007f93400b0000 nid=0x130 waiting for monitor entry [0x00007f933f510000]
java.lang.Thread.State: BLOCKED (on object monitor)
Debugging Deadlocks
Once a deadlock has been detected, the next step is to debug the issue. This can involve:
- Analyzing thread dumps and stack traces: To identify the resources involved in the deadlock and the point in the code where the deadlock occurred.
- Inspecting the code: To identify the synchronization mechanisms and resource management strategies used.
- Using debugging tools: Such as Java Mission Control or VisualVM, to inspect the state of threads and resources.
Resolving Deadlocks
Resolving deadlocks typically involves one or more of the following strategies:
- Avoiding nested locks: Nested locks can increase the likelihood of deadlocks. Avoiding nested locks can help reduce the risk of deadlocks.
- Using timeouts: Implementing timeouts can help prevent deadlocks by allowing threads to recover from blocked states.
- Improving resource management: Improving resource management, such as using pools or queues, can help reduce the likelihood of deadlocks.
Example Code: Avoiding Nested Locks
Here's an example of how to avoid nested locks to prevent deadlocks:
public class DeadlockExample {
private static Object lock1 = new Object();
private static Object lock2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (lock1) {
System.out.println("Thread 1: Holding lock 1");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
// Avoiding nested locks
synchronized (lock2) {
System.out.println("Thread 1: Holding lock 2");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (lock2) {
System.out.println("Thread 2: Holding lock 2");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
// Avoiding nested locks
synchronized (lock1) {
System.out.println("Thread 2: Holding lock 1");
}
}
});
thread1.start();
thread2.start();
}
}
Conclusion
Deadlocks are a common issue in multithreaded Java applications. By understanding the causes of deadlocks, detecting them using tools and techniques, and debugging and resolving them using strategies such as avoiding nested locks and improving resource management, developers can write more robust and reliable code. We hope this article has provided valuable insights into Java deadlocks debugging. If you have any questions or comments, please feel free to share them below.
Try this code snippet: Copy and paste the example code into your Java IDE to see how deadlocks can occur. Experiment with different synchronization mechanisms and resource management strategies to resolve the deadlock.
Share your thoughts: Have you encountered deadlocks in your Java applications? How did you resolve them? Share your experiences and insights in the comments below.
This content originally appeared on DEV Community and was authored by Kush Parsaniya
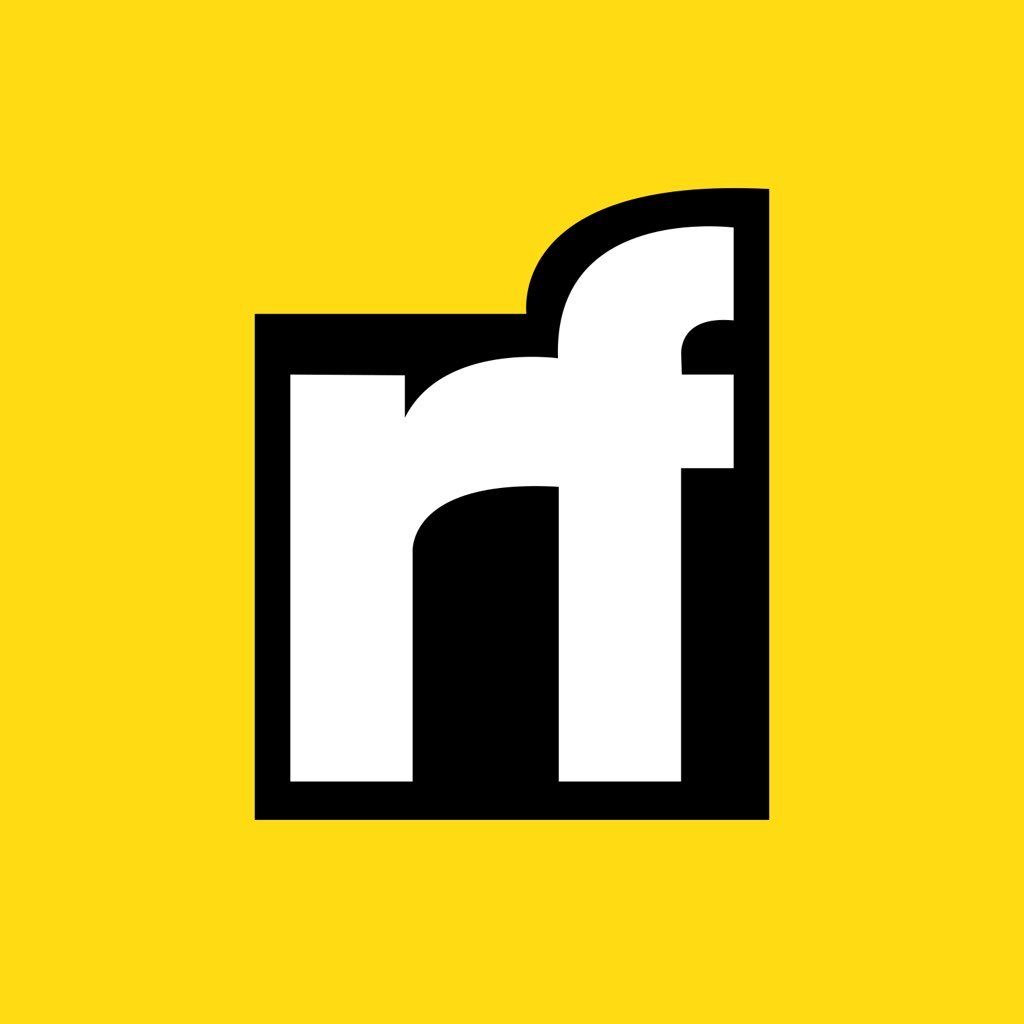
Kush Parsaniya | Sciencx (2025-02-01T10:55:36+00:00) Java Deadlocks Debugging: A Comprehensive Guide. Retrieved from https://www.scien.cx/2025/02/01/java-deadlocks-debugging-a-comprehensive-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.