This content originally appeared on Level Up Coding - Medium and was authored by Kristian Ivanov
While building Web à la Carte, I discovered that service workers can’t do everything we need in modern extensions. Enter the Offscreen API — Chrome’s solution for running DOM operations in the background. Let’s dive into how this powerful API can transform your extensions.
The Background/Info
Offscreen API
— Released: Late 2022
— Part of Manifest V3’s solutions
— Designed to replace background page capabilities
— Currently in stable release
Why Do We Need It?
With Manifest V3’s shift to service workers, we lost the ability to:
— Use DOM APIs in the background
— Play audio (something I’ve used heavily in an older (no longer supported) extension of mine)
— — Something to keep in mind is that if you are usingthe AUDIO_PLAYBACK reason it will close the offscreen document after 30 seconds without audio playing.
— Create canvases
— Make AJAX requests with certain headers
— Use WebSockets
The Offscreen API solves these problems by providing a real DOM environment that runs in the background.
What Makes It Special?
The Offscreen API is unique in several ways:
— Creates a hidden document with full DOM access
— Runs in the background without opening new windows
— Inherits extension permissions (with some limits)
— Only uses chrome.runtime API for communication
Important Limitations
Before diving in, there are some key things to understand:
1. You can only use static HTML files from your extension
2. Only one offscreen document can be open at a time*
3. Documents can’t be focused
4. The window.opener property is always null
5. Only chrome.runtime API is available
*Note: In split mode with an active incognito profile, you can have one document per profile.
The Basics
First, declare it in your manifest:
{
"manifest_version": 3,
"permissions": ["offscreen"],
"background": {
"service_worker": "background.js"
}
}
Then create an offscreen document:
// background.js
async function createOffscreen() {
// Check if we already have an offscreen document
const existing = await chrome.offscreen.getOffscreenDocument();
if (existing) return;
// Create an offscreen document
await chrome.offscreen.createDocument({
url: 'offscreen.html',
reasons: ['AUDIO_PLAYBACK'], // Or other reasons
justification: 'Playing notification sounds' // Explain why you need it
});
}
Real World Examples
1. Audio Notifications
// background.js
async function playNotificationSound() {
await createOffscreen();
// Send message to offscreen document
chrome.runtime.sendMessage({
target: 'offscreen',
type: 'PLAY_SOUND',
sound: 'notification.mp3'
});
}
// offscreen.html
const audio = new Audio();
chrome.runtime.onMessage.addListener((message) => {
if (message.target !== 'offscreen') return;
if (message.type === 'PLAY_SOUND') {
audio.src = message.sound;
audio.play();
}
});
2. Image Processing
// background.js
async function processImage(imageUrl) {
await createOffscreen();
return new Promise((resolve) => {
chrome.runtime.onMessage.addListener(function listener(message) {
if (message.type === 'IMAGE_PROCESSED') {
chrome.runtime.onMessage.removeListener(listener);
resolve(message.data);
}
});
chrome.runtime.sendMessage({
target: 'offscreen',
type: 'PROCESS_IMAGE',
imageUrl
});
});
}
// offscreen.html
async function processImage(url) {
const img = new Image();
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
await new Promise((resolve) => {
img.onload = resolve;
img.src = url;
});
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
// Apply image processing
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
// ... process image data ...
return canvas.toDataURL();
}
chrome.runtime.onMessage.addListener(async (message) => {
if (message.type === 'PROCESS_IMAGE') {
const processed = await processImage(message.imageUrl);
chrome.runtime.sendMessage({
type: 'IMAGE_PROCESSED',
data: processed
});
}
});
Mastering the Offscreen API
The Art of Resource Management
Think of the Offscreen API like a smart assistant — don’t keep them around when you don’t need them. Here’s a pattern I’ve found incredibly useful:
class OffscreenManager {
constructor() {
this.activeOperations = new Map();
this.retryAttempts = 0;
this.maxRetries = 3;
}
async execute(task, timeout = 5000) {
const operationId = crypto.randomUUID();
try {
// Create offscreen document if needed
await this.ensureOffscreen();
// Set up task tracking
const operation = {
startTime: Date.now(),
status: 'running',
timeout: setTimeout(() => this.handleTimeout(operationId), timeout)
};
this.activeOperations.set(operationId, operation);
// Execute and track
const result = await task();
this.completeOperation(operationId);
return result;
} catch (error) {
if (this.retryAttempts < this.maxRetries) {
this.retryAttempts++;
return this.execute(task, timeout);
}
throw error;
}
}
async cleanup() {
if (this.activeOperations.size === 0) {
await chrome.offscreen.closeDocument();
this.retryAttempts = 0;
}
}
}
// Usage
const manager = new OffscreenManager();
// Process multiple images in batch
const results = await manager.execute(async () => {
const images = ['image1.jpg', 'image2.jpg', 'image3.jpg'];
return Promise.all(images.map(img => processImage(img)));
});
Smart Error Recovery
Instead of just handling errors, let’s make our offscreen operations self-healing:
const smartOffscreen = {
async createWithFallback(options) {
try {
await chrome.offscreen.createDocument(options);
} catch (error) {
// If creation fails, check if we have zombie documents
const existing = await chrome.offscreen.getOffscreenDocuments();
if (existing.length > 0) {
// Clean up and retry
await Promise.all(existing.map(doc =>
chrome.offscreen.closeDocument(doc.url)));
await chrome.offscreen.createDocument(options);
}
}
},
// Or automatically retry failed operations
async withRetry(operation, maxAttempts = 3) {
for (let attempt = 1; attempt <= maxAttempts; attempt++) {
try {
return await operation();
} catch (error) {
if (attempt === maxAttempts) throw error;
// Exponential backoff
await new Promise(r => setTimeout(r, Math.pow(2, attempt) * 100));
}
}
}
};
These patterns make your offscreen operations more robust and self-managing. The key is to treat your offscreen document like a valuable resource — use it wisely, monitor it carefully, and clean up when you’re done.
When to Use the Offscreen API
Use It When:
— You need DOM APIs in the background
— You’re playing audio or processing media
— You need WebSocket connections
— You’re doing complex canvas operations
— You need to use certain web APIs unavailable in service workers
Don’t Use It When:
— Simple data processing is enough
— Content scripts or a background script can handle the task
— You don’t need DOM capabilities
Final Thoughts
The Offscreen API bridges the gap between service workers and traditional background pages. While it shouldn’t be your go-to solution for everything, it’s invaluable when you need DOM capabilities in the background.
Remember:
— Only create offscreen documents when needed
— Clean up resources when done
— Use appropriate reasons and justifications
— Consider performance implications
If you found this article helpful, feel free to clap and follow for more JavaScript and Chrome.API tips and tricks.
You can also give a recent chrome extension I released that uses a lot of the functionalities from the articles — Web à la Carte
If you have gotten this far, I thank you and I hope it was useful to you! Here is a cool image of cats next to a screen that is off as a thank you!
Chrome’s Offscreen API: The Hidden Powerhouse for Modern Extensions was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Kristian Ivanov
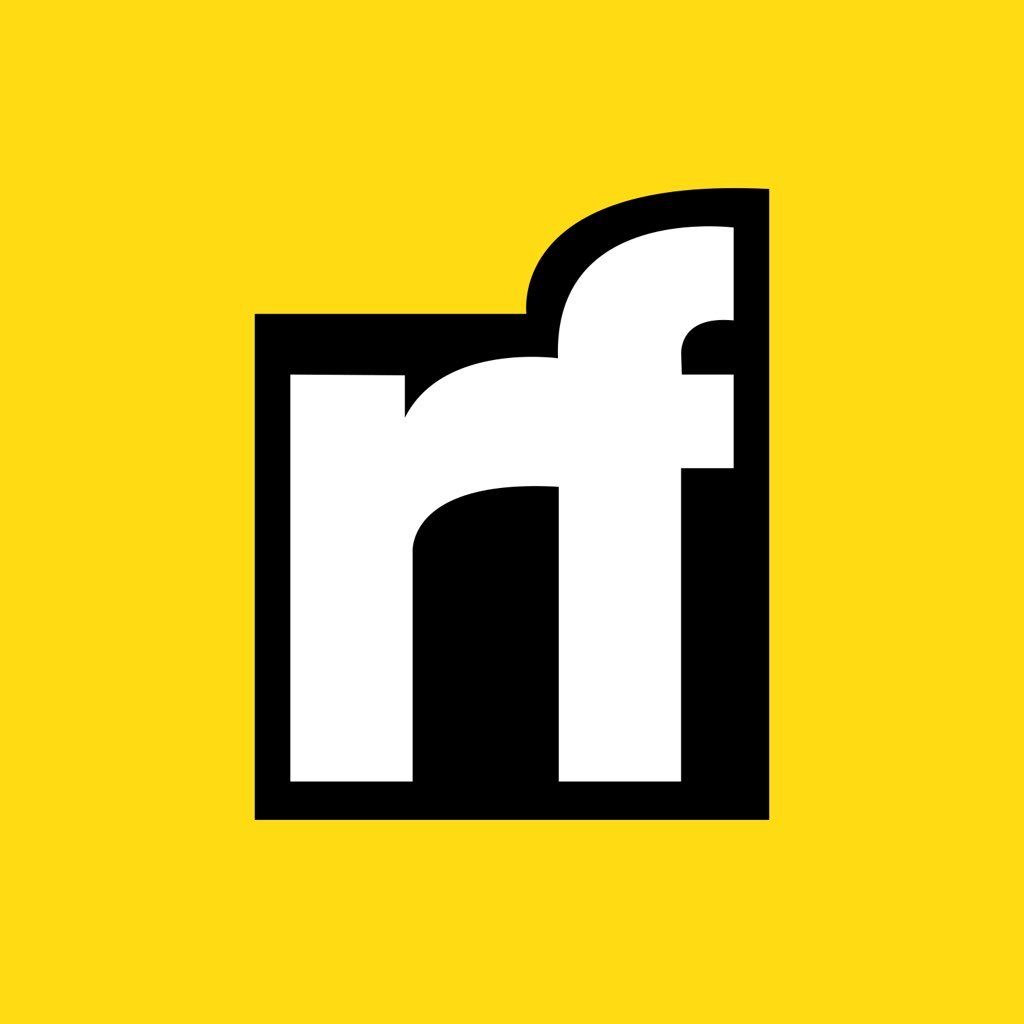
Kristian Ivanov | Sciencx (2025-02-24T14:13:47+00:00) Chrome’s Offscreen API: The Hidden Powerhouse for Modern Extensions. Retrieved from https://www.scien.cx/2025/02/24/chromes-offscreen-api-the-hidden-powerhouse-for-modern-extensions/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.