This content originally appeared on Envato Tuts+ Tutorials and was authored by Shalabh Aggarwal
REST (REpresentational State Transfer) is a web development architecture design style that refers to logically separating your API resources to enable easy access, manipulation, and scaling. Reusable components are written to be easily managed via simple and intuitive HTTP requests, which can be GET, POST, PUT, PATCH, and DELETE (there can be more, but above are the most commonly used ones).
Despite what it looks like, REST does not command a protocol or a standard. It just sets a software architectural style for writing web applications and APIs and simplifies the interfaces within and outside the application. Web service APIs that are written to follow the REST principles are called RESTful APIs.
In this three-part tutorial series, I will cover how RESTful APIs can be created using Flask as a web framework. The first part will cover how to create class-based REST APIs in a DIY way (do it yourself)—implementing them all by yourself without using any third-party extensions. In the latter parts of this series, I will cover how to leverage various Flask extensions to build more effective REST APIs more easily.
Getting Started
Lets start by creating a project directory and a virtual environment
mkdir flask_app cd flask_app python3.8 -m venv env source env/bin/activate
Installing Dependencies
The following packages need to installed for the application that we'll be developing.
$ pip install flask $ pip install -U Flask-SQLAlchemy
The above commands should install all the required packages that are needed for this application to work.
The Flask Application
For this tutorial, we will create a small application to create a trivial model for Product. Then demonstrate how we can write a RESTful API for the same. Below is the structure of the application.
flask_app/ my_app/ - __init__.py product/ - __init__.py // Empty file - models.py - views.py - run.py
We won't be creating a front-end for this application as RESTful APIs endpoints can be tested directly by making HTTP calls using various other methods. Open my_app/__int__.py and add the following code.
# flask_app/my_app/__init__.py from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydb.db' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False db = SQLAlchemy(app) db.create_all()
We first initialize a Flask app instance in the code above, configure it with an SQlite database, and finally create the database. db.create_all()
will create a new database at the location provided against SQLALCHEMY_DATABASE_URI
if a database does not already exist at that location; otherwise, it loads the application with the same database. We won't be creating a front-end for this application as RESTful APIs endpoints can be tested directly by making HTTP calls using various other methods.
Open product/models.py and add the models for our product. The model will have 3 fields, namely.
id
: a unique primary keyname
price
from my_app import db class Product(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(255)) price = db.Column(db.Float(asdecimal=True)) def __init__(self, name, price): self.name = name self.price = price def __repr__(self): return '<Product %d>' % self.id
In the file above, we have created a very trivial model for storing the name and price of a Product
. This will create a table in SQLite
corresponding to the details provided in the model.
Flask Blueprint
Flask blueprint help to create structure in Flask application by grouping views templates e.t.c into reusable components. Open product/views.py and create a Flask Blueprint that contains the home view and then use it in the application.
from flask import Blueprint, abort from my_app import db, app from my_app.product.models import Product catalog = Blueprint('catalog', __name__) @catalog.route('/') @catalog.route('/home') def home(): return "Welcome to the Catalog Home.
To use the blueprint, you have to register it in the application using the register_blueprint()
command. Open my_app/__init__.py
from my_app.product.views import catalog app.register_blueprint(catalog)
Views
Open product.views.py and create our views. We will use pluggable class-based views which provide flexibility.
import json from flask import request, jsonify, Blueprint, abort from flask.views import MethodView from my_app import db, app from my_app.catalog.models import Product catalog = Blueprint('catalog', __name__) @catalog.route('/') @catalog.route('/home') def home(): return "Welcome to the Catalog Home." class ProductView(MethodView): def get(self, id=None, page=1): if not id: products = Product.query.paginate(page, 10).items res = {} for product in products: res[product.id] = { 'name': product.name, 'price': str(product.price), } else: product = Product.query.filter_by(id=id).first() if not product: abort(404) res = { 'name': product.name, 'price': str(product.price), } return jsonify(res) def post(self): name = request.form.get('name') price = request.form.get('price') product = Product(name, price) db.session.add(product) db.session.commit() return jsonify({product.id: { 'name': product.name, 'price': str(product.price), }}) def put(self, id): # Update the record for the provided id # with the details provided. return def delete(self, id): # Delete the record for the provided id. return
The major crux of this tutorial is dealt with in the file above. Flask provides a utility called pluggable views, which allows you to create views in the form of classes instead of normally as functions. Method-based dispatching (MethodView
) is an implementation of pluggable views which allows you to write methods corresponding to the HTTP methods in lower case. In the example above, I have written methods get()
and post()
corresponding to HTTP's GET
and POST
respectively.
Here we create a ProductView
class that defines a get and post function. The get function retrieves the products from the database and paginates the results.
The post method obtains request data in json format and adds the data to the database.
Routing
Routing is also implemented in a different manner. After adding the views, add the routes.
product_view = ProductView.as_view('product_view') app.add_url_rule( '/product/', view_func=product_view, methods=['GET', 'POST'] ) app.add_url_rule( '/product/<int:id>', view_func=product_view, methods=['GET'] )
In the code above, we can specify the methods that will be supported by any particular rule. Any other HTTP call would be met by Error 405 Method not allowed
.
Running the Application
To run the application, execute the script run.py
. The contents of this script are:
from my_app import app app.run(debug=True)
Now just execute from the command line:
$ python run.py
To check if the application works, fire up http://127.0.0.1:5000/ in your browser, and a simple screen with a welcome message should greet you.
Testing the RESTful API
To test this API, we can simply make HTTP calls using any of the many available methods. GET calls can be made directly via the browser. POST calls can be made using a Chrome extension like Postman or from the command line using curl
, or we can use Python's requests
library to do the job for us. I'll use the requests library here for demonstration purposes. Start by installing the request library using pip
pip install requests
Let's make a GET
call first to assure that we don't have any products created yet. As per RESTful API's design, a get call which looks something like /product/
should list all products. Then I will create a couple of products by making POST
calls to /product/
with some data. Then a GET
call to /product/
should list all the products created. To fetch a specific product, a GET
call to /product/<product id>
should do the job.
Enter the python interactive shell
python3.8
Below is a sample of all the calls that can be made using this example.
>>> import requests >>> r = requests.get('http://localhost:5000/product/') >>> r.json() {} >>> r = requests.post('http://localhost:5000/product/', data={'name': 'iPhone 6s', 'price': 699}) >>> r.json() {u'1': {u'price': u'699.0000000000', u'name': u'iPhone 6s'}} >>> r = requests.post('http://localhost:5000/product/', data={'name': 'iPad Pro', 'price': 999}) >>> r.json() {u'2': {u'price': u'999.0000000000', u'name': u'iPad Pro'}} >>> r = requests.get('http://localhost:5000/product/') >>> r.json() {u'1': {u'price': u'699.0000000000', u'name': u'iPhone 6s'}, u'2': {u'price': u'999.0000000000', u'name': u'iPad Pro'}} >>> r = requests.get('http://localhost:5000/product/1') >>> r.json() {u'price': u'699.0000000000', u'name': u'iPhone 6s'}
Conclusion
In this tutorial, you saw how to create RESTful interfaces all by yourself using Flask's pluggable views utility. This is the most flexible approach while writing REST APIs but involves much more code to be written.
There are extensions that simplify life and automate the implementation of RESTful APIs to a huge extent. We will be covering these in the next couple of parts of this tutorial series.
This post has been updated with contributions from Esther Vaati. Esther is a software developer and writer for Envato Tuts+.
This content originally appeared on Envato Tuts+ Tutorials and was authored by Shalabh Aggarwal
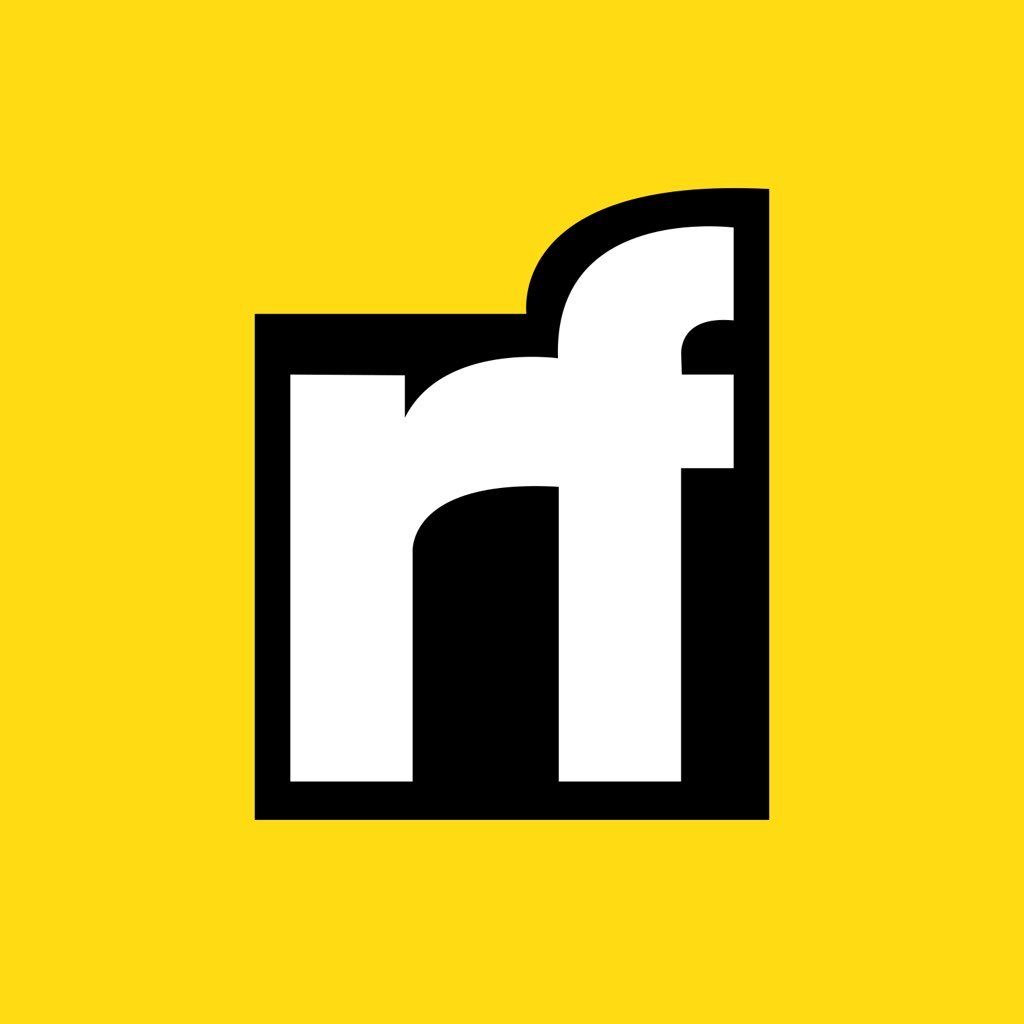
Shalabh Aggarwal | Sciencx (2016-05-30T18:06:58+00:00) Building RESTful APIs With Flask: The DIY Approach. Retrieved from https://www.scien.cx/2016/05/30/building-restful-apis-with-flask-the-diy-approach/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.