This content originally appeared on Stefan Judis Web Development and was authored by Stefan Judis
That's a quick one. ?
When writing Node.js scripts that use the fs
module, I usually used the util.promisify
method to promisify the file-system methods. Promises-based metheds allow using async/await
that makes code easier to grasp.
Today I learned that since Node.js 11 the fs
module provides "promisified" methods in a promises
property. ?
// old way have using promise-based fs methods
const { readFile } = require("fs");
const { promisify } = require('util');
const promisifiedReadFile = promisify(readFile);
promisifiedReadFile(__filename, { encoding: "utf8" })
.then(data => console.log(data));
// --------------------
// new way of using promise-based fs methods
// no util.promisify!!!
const { readFile } = require("fs").promises;
readFile(__filename, { encoding: "utf8" })
.then(data => console.log(data));
Using the promises
property you can now skip the step to transform callbacks into promises and there is no need to use promisify
. That's excellent news to flatten some source code and go all in with async/await
!
fs/promises
is available since Node.js 14
Section titled `fs/promises` is available since Node.js 14
Update: Since Node.js 14 the fs
module provides two ways to use the promises-based file-system methods. The promisified methods are available via require('fs').promises
or require('fs/promises')
.
// Since Node.js v14: use promise-based fs methods
// no util.promisify!!!
const { readFile } = require("fs/promises");
readFile(__filename, { encoding: "utf8" })
.then(data => console.log(data));
I'm very excited about the /promises
path addition. The Node.js maintainers seemed to agree on this way to expose more promise-based methods of existing modules in the future.
In Node.js v15 the Timers module also provides an experimental timers/promises
package. That means you can do await setTimeout
soon – Node.js is evolving and that means less util.promisify
and more coding! ?
If you want to read more Node.js tips and tricks head over to the Node.js section on my blog or subscribe to my newsletter below.
Reply to Stefan
This content originally appeared on Stefan Judis Web Development and was authored by Stefan Judis
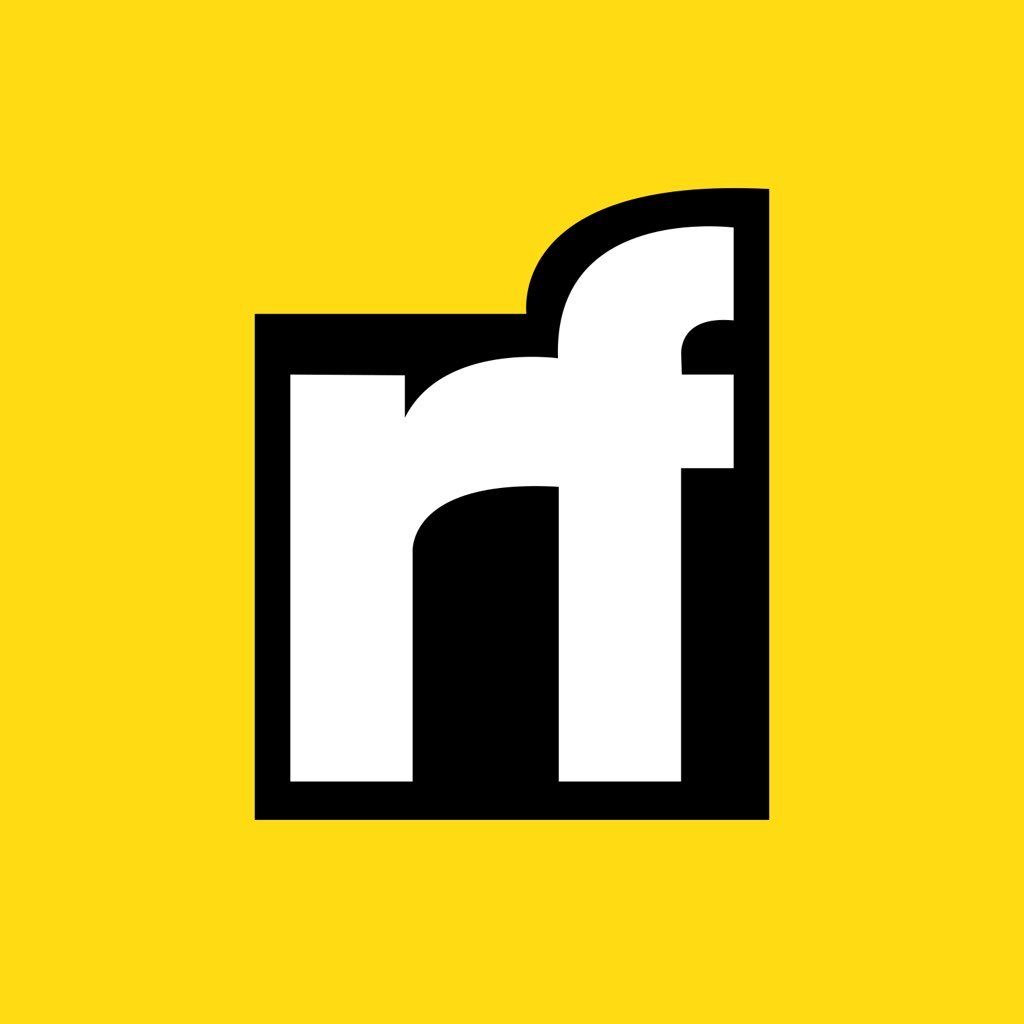
Stefan Judis | Sciencx (2019-10-09T22:00:00+00:00) The fs module includes promisified methods since Node 11 (#tilPost). Retrieved from https://www.scien.cx/2019/10/09/the-fs-module-includes-promisified-methods-since-node-11-tilpost/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.