This content originally appeared on Stefan Judis Web Development and was authored by Stefan Judis
HTML form
elements are the foundation for the interactions in web pages. And they improved quite a little bit over the last years. Today, developers can use different types (number
, tel
, color
, ...) and set different input modes (text
, decimal
, email
, ...) to name two examples.
What remained tricky was to submit forms from within the scope of JavaScript. The HTMLFormElement
defines a submit
method, but it does not quite behave as one would expect.
HTML's default behavior and the not matching submit
method
Section titled HTML's default behavior and the not matching `submit` method
Let's assume we have the following HTML form:
<form action="">
<label>
Your name
<input type="text" required>
</label>
<button>Submit</button>
</form>
And some JavaScript:
document.querySelector('form')
.addEventListener('submit', (event) => {
// don't submit the form and
// only log to the console
event.preventDefault();
console.log('submitted form');
});
When one clicks the submit
button the following happens:
- the form is validated and possible errors are shown
- if the form is valid, it fires a
submit
event - the
submit
handler is called and it prevents the form submission due toevent.preventDefault()
This triggered submit
event gives developers a way to react to form submissions in JavaScript. And it's used a lot! Common scenarios in modern applications are to call preventDefault
, make AJAX requests using JavaScript and replace the page contents dynamically.
But what happens when you get the form from the DOM and submit it in JavaScript via submit
?
document.querySelector('form').submit();
The answer is – the form is submitted! (?♂️ duh!) What's surprising is that there won't be input validation, and there won't be a submit
event. All the values are submitted no matter if the inputs are valid or not. This is unexpected behavior and it should behave like pressing the submit
button. There are surely reason for skipping the validation, but I'd expect that submit
also validates the form and only proceeds if everything is valid.
You can work around this problem by triggering the click
on the submit button. click
triggers the standard behavior that users see when they interact with a form, including validation and a fired submit
event.
Mimicking, user behavior works fine and that's great – case closed! I never thought of it as elegant or pretty, though.
A new JavaScript method that validates forms before submitting
Section titled A new JavaScript method that validates forms before submittingPeople started to work on a solution to this behavior in June 2019 (the proposal is an interesting read). The HTMLFormElement
now includes an extra method called requestSubmit
. And this method does the same as clicking a submit
button. ?
There is no magic to it – it does what you expect and offers the great goodies HTML forms ship by default. I have to say – I'm excited about it!
submit |
requestSubmit |
---|---|
doesn't trigger submit event |
triggers submit event |
doesn't trigger validation | triggers validation |
can't be canceled | can be canceled via event.preventDefault in a submit event handler |
The browser support as of November 2020 is as follows:
- ✅ Chromium browsers (the new Microsoft Edge, Chrome, Opera, ...)
- ✅ Firefox
- ❌ Safari
Unfortunately, it's not cross browser supported yet.
You can read more about the requestSubmit
method on MDN, read about it in the spec or see it in action on CodePen.
You can see a #devsheet visualizing the difference in the video below.
If you're interested in more reading more quick TIL ("today i learned") posts, subscribe to my monthly newsletter. ?
Reply to Stefan
This content originally appeared on Stefan Judis Web Development and was authored by Stefan Judis
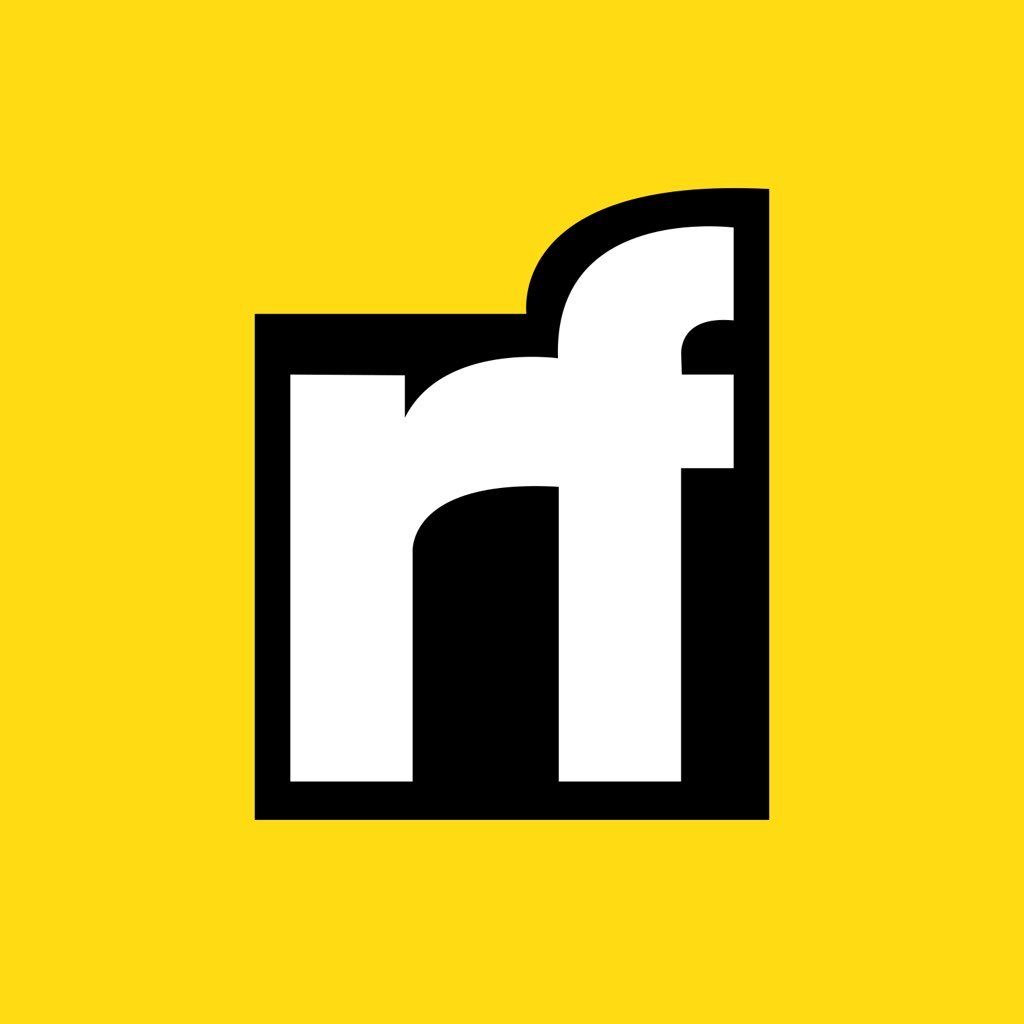
Stefan Judis | Sciencx (2020-02-13T23:00:00+00:00) requestSubmit offers a way to validate a form before submit (#tilPost). Retrieved from https://www.scien.cx/2020/02/13/requestsubmit-offers-a-way-to-validate-a-form-before-submit-tilpost/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.