This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
Functions, variables and objects can be analyzed using introspection.
First, using the help()
global function we can get the documentation if provided in form of docstrings.
Then, you can use print() to get information about a function:
def increment(n):
return n + 1
print(increment)
# <function increment at 0x7f420e2973a0>
or an object:
class Dog():
def bark(self):
print('WOF!')
roger = Dog()
print(roger)
# <__main__.Dog object at 0x7f42099d3340>
The type()
function gives us the type of an object:
print(type(increment))
# <class 'function'>
print(type(roger))
# <class '__main__.Dog'>
print(type(1))
# <class 'int'>
print(type('test'))
# <class 'str'>
The dir()
global function lets us find out all the methods and attributes of an object:
print(dir(roger))
# ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'bark']
The id()
global function shows us the location in memory of any object:
print(id(roger)) # 140227518093024
print(id(1)) # 140227521172384
It can be useful to check if two variables point to the same object.
The inspect
standard library module gives us more tools to get information about objects, and you can check it out here: https://docs.python.org/3/library/inspect.html
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
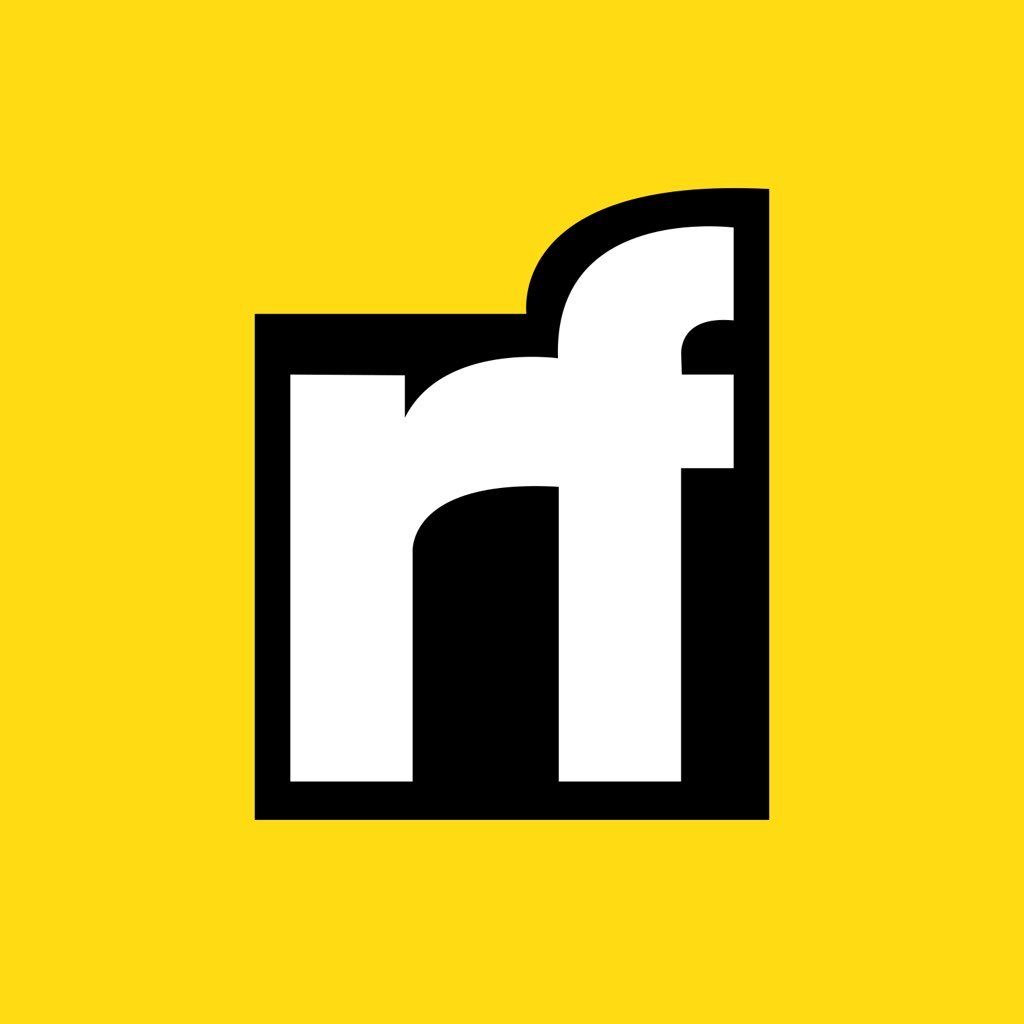
flaviocopes.com | Sciencx (2021-01-20T05:00:00+00:00) Python Introspection. Retrieved from https://www.scien.cx/2021/01/20/python-introspection/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.