This content originally appeared on Level Up Coding - Medium and was authored by Radhakishan Surwase
An enum group related constants together in one type. Enums are a powerful feature with a wide range of uses. However, in Go, they’re implemented quite differently than most other programming languages.
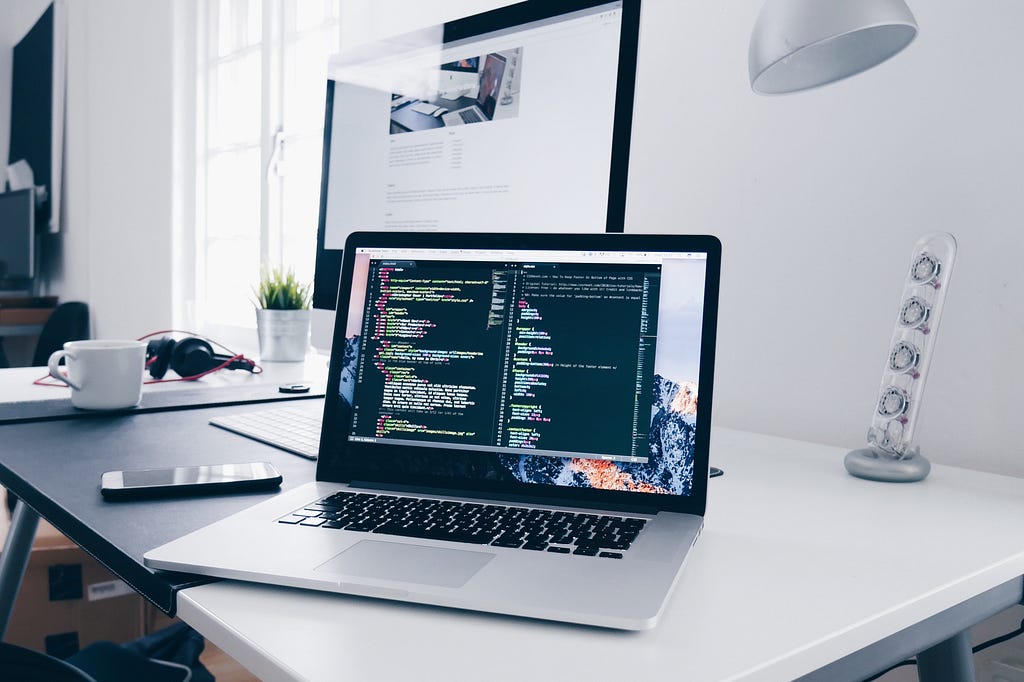
Note: Before you go any further, I expect you all have a beginner-level understanding of Go syntax and primitive types to understand the source-code.
In this article, I will show you how to implement enums in Golang using a predeclared identified iota. Before proceeding with enums, let’s first understand what is iota and how it is used.
What is iota?
iota is an identifier that is used with constant and which can simplify constant definitions that use auto-increment numbers. The iota keyword represents integer constant starting from zero.
The iota keyword represents successive integer constants 0, 1, 2,….It resets to 0 whenever the word const appears in the source code, and increments after each const specification.
You can avoid writing successive iota in front of every constant. This can be simplified as in bellow code listing:
To start a list of constants at 1 instead of 0, you can use iota in an arithmetic expression.
You can use the blank identifier to skip a value in a list of constants.
Implement Enums using iota
I order to implement custom enum type we have to perform consider the following
- Declare a new custom type — Integer Type.
- Declare related constants — Using iota.
- Creating common behavior — give the type a String function.
- Creating additional behavior — give the type a EnumIndex function.
Example 1: Create an enum for the Weekdays.

Example 2: Create an enum for the Directions.
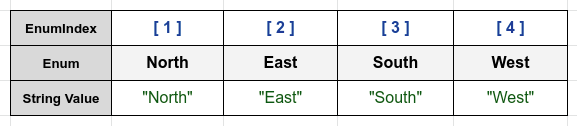
Conclusion
An enum is a data type consisting of a set of named constant values. Enums are a powerful feature with a wide range of uses. However, in Golang, they’re implemented quite differently than most other programming languages. Golang does not support enums directly. We can implement it using iota and constants.
That's all for now… Keep Learning… Happy Learning ?
Implementing Enums in Golang was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Radhakishan Surwase
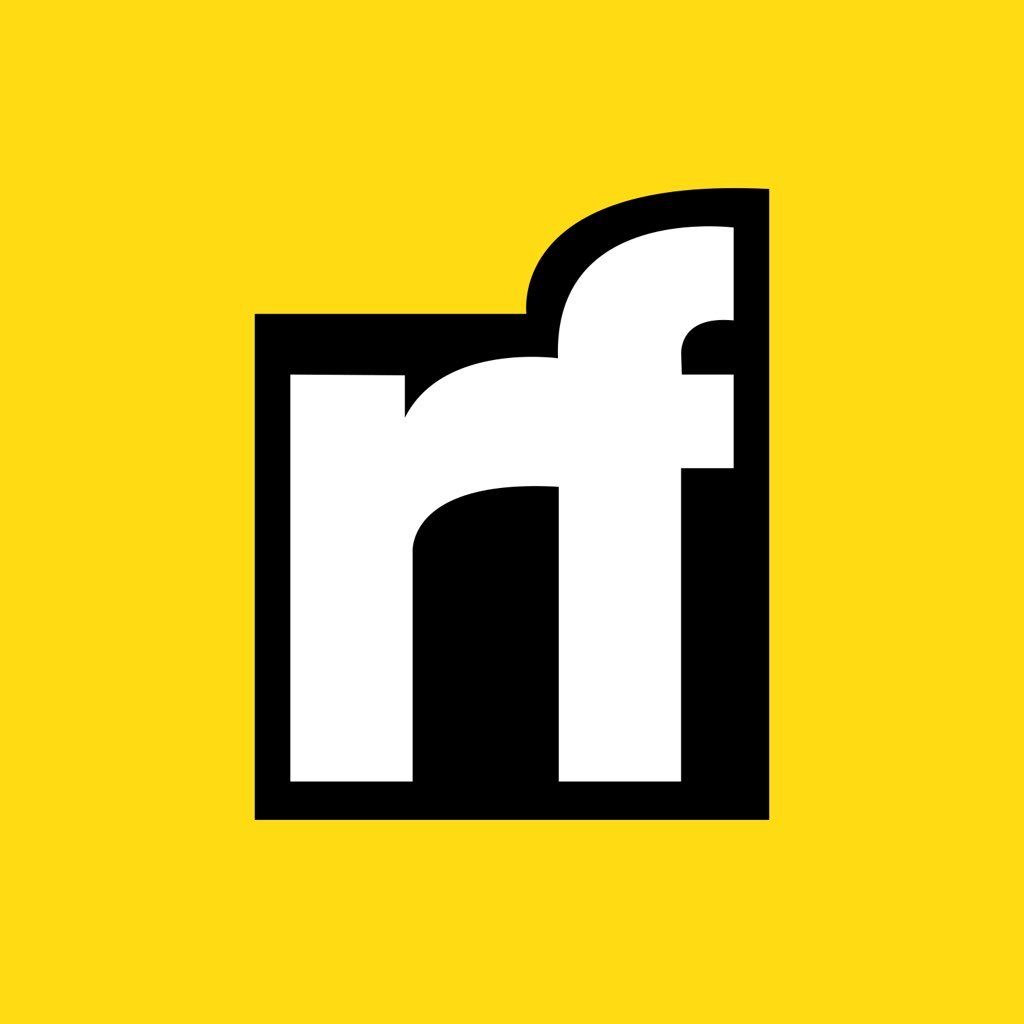
Radhakishan Surwase | Sciencx (2021-03-15T00:37:03+00:00) Implementing Enums in Golang. Retrieved from https://www.scien.cx/2021/03/15/implementing-enums-in-golang/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.