This content originally appeared on Level Up Coding - Medium and was authored by Rebecca Hickson
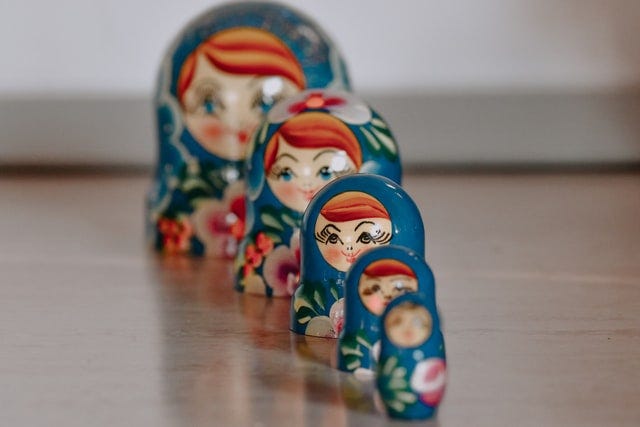
Although it can be easy to get lost while exploring deeply nested objects, sometimes they’re the best route to take. Based on my own (often frustrating) experience with them, I’ve put together a map to get you on the right track. This guide will walk you through using a React/Redux frontend to work with your Rails API backend.
The Setup
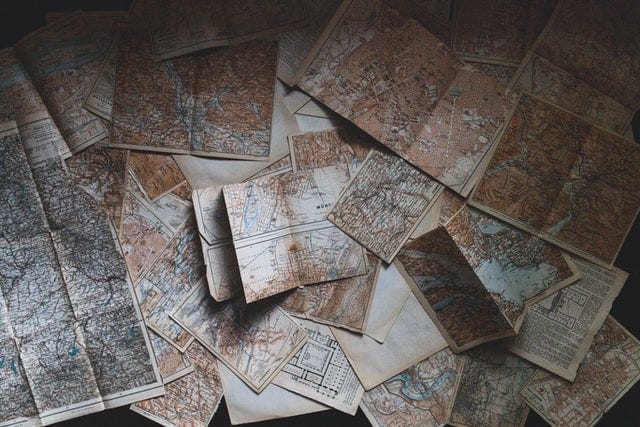
First, make sure to setup your database with the correct associations. Here’s how your schema might look. In this example, the Ingredients model has_many Recipes through RecipeIngredients.
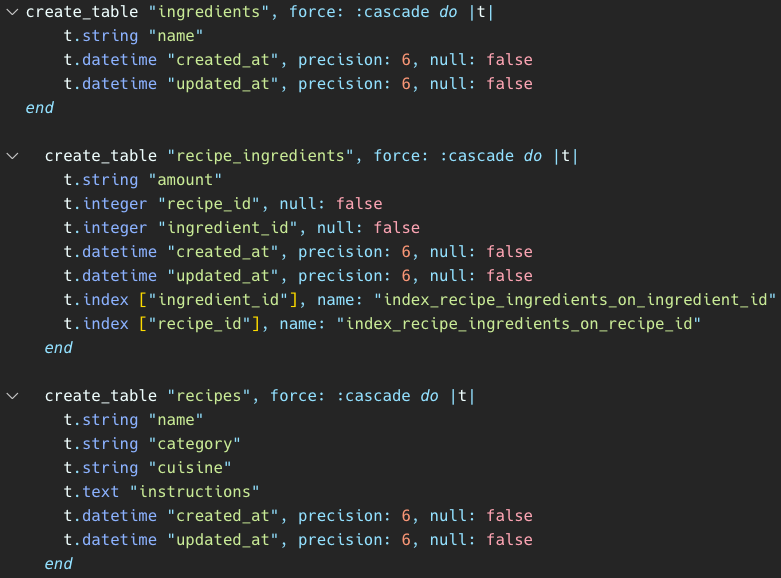
Next, we’ll set up our RecipeForm component. For our example, we’d like to create a new Recipe along with its associated RecipeIngredients and Ingredients. Your state should include a top-level property corresponding to the name of your model (in this case recipe) containing its attributes. Below is an example of the state for my models.
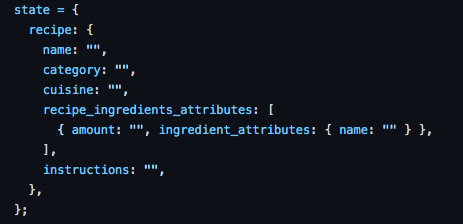
Very important — append _attributes after each associated model (e.g.recipe_ingredients_attributes and ingredient_attributes). This step will make sure your database processes the information correctly.
The Good Stuff
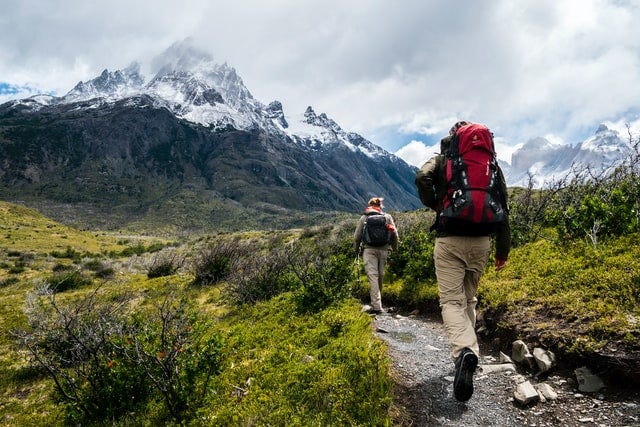
Don’t forget to set up a controlled form along with a Redux action and reducer. Since there are many great resources available to help on those fronts, we’ll stick to handling things unique to our mission.
Let’s start with the RecipesController. We’ll need to update our strong parameters to accept the nested attributes for RecipeIngredients and Ingredients.

Now we’ll customize our attribute writer methods to control how our Ingredients data gets inserted. In the Recipe model, we can add the following code to build a new associated RecipeIngredient for each recipe_ingredients_attributes that we are passing in.
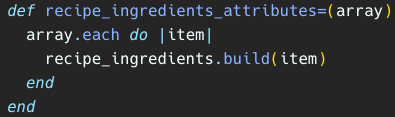
Now we need to handle our Ingredients. In the RecipeIngredient model (i.e. the through association that links Recipes and Ingredients), we should customize the attribute writer method as shown below. For this application, we want to prevent creating multiple Ingredients by the same name, so we will use the find_or_create_by method to associate the RecipeIngredient to the right Ingredient.

The Destination
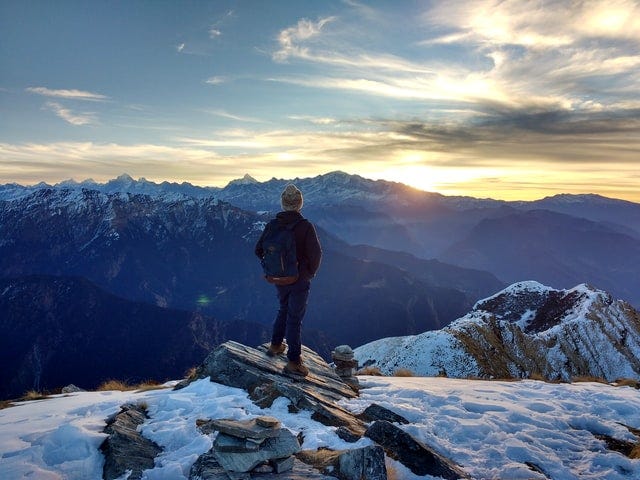
That’s it! We can now create a new Recipe, automatically create new RecipeIngredients, and through those we can connect to a previous instance of Ingredient or create a new one — all in one go! It takes a bit more setup and care at the frontend, but using this method can help you create a quick, intuitive application. If you would like to see this code in action, feel free to check out my application Frying Plan on Github. Happy Coding!
Handling Nested Attributes with a Has Many Through Association with Rails API was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Rebecca Hickson
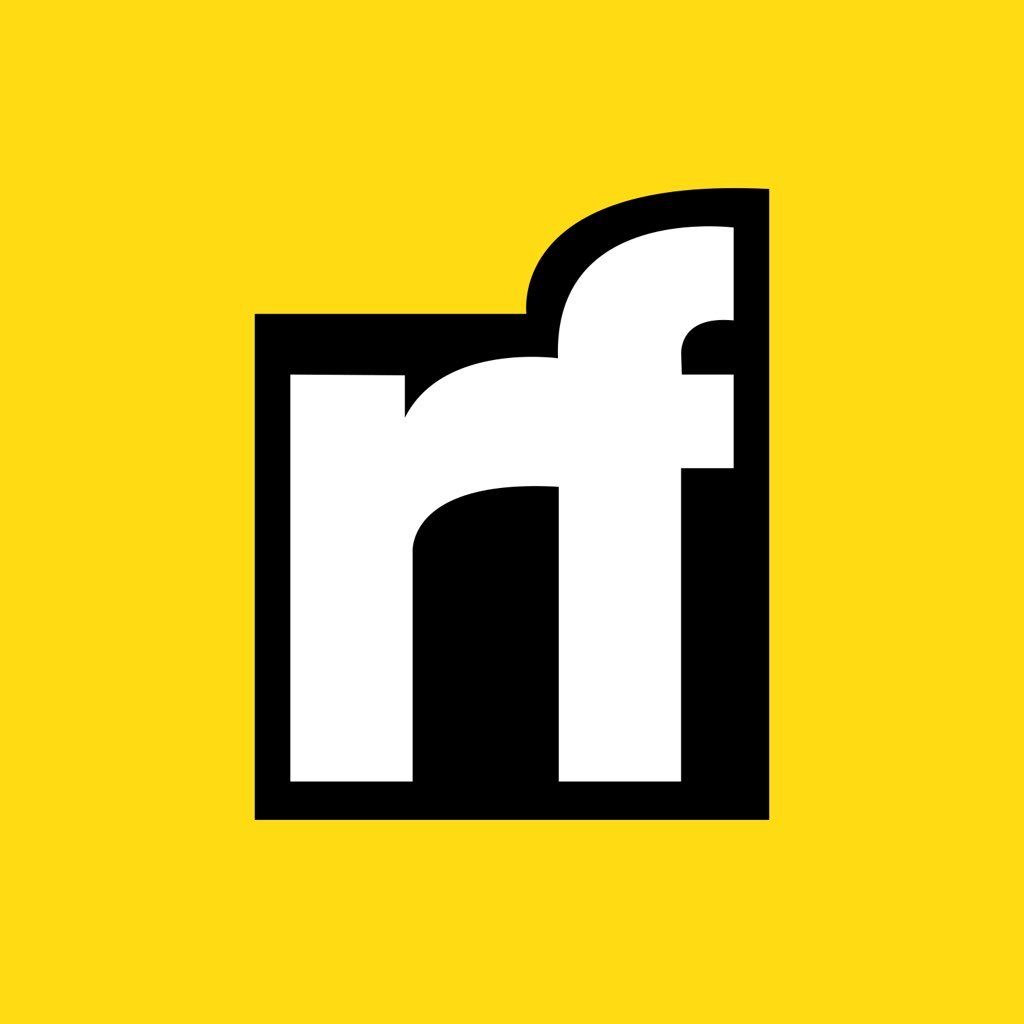
Rebecca Hickson | Sciencx (2021-03-20T23:50:41+00:00) Handling Nested Attributes with a Has Many Through Association with Rails API. Retrieved from https://www.scien.cx/2021/03/20/handling-nested-attributes-with-a-has-many-through-association-with-rails-api/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.