This content originally appeared on Level Up Coding - Medium and was authored by Christian Zink
How to use K8S on Docker Desktop with Ingress to Develop Locally for the Cloud Using an ASP.NET Core REST API and Angular.
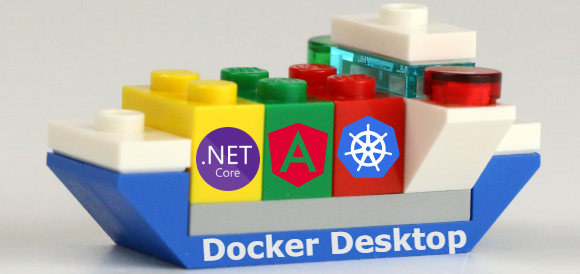
Kubernetes runs in a local environment with docker desktop. It is similar to a cloud environment. You can use it for developing and testing. There are no extra costs. You can always easily deploy it later to the cloud.
In this guide, you will create a raw microservice-based cloud architecture. It uses .NET Core with REST-APIs in the backend services and Angular as the frontend. All components are dockerized and Kubernetes orchestrates the containers. The application is exposed via an Ingress controller.
Overview diagram of the components:
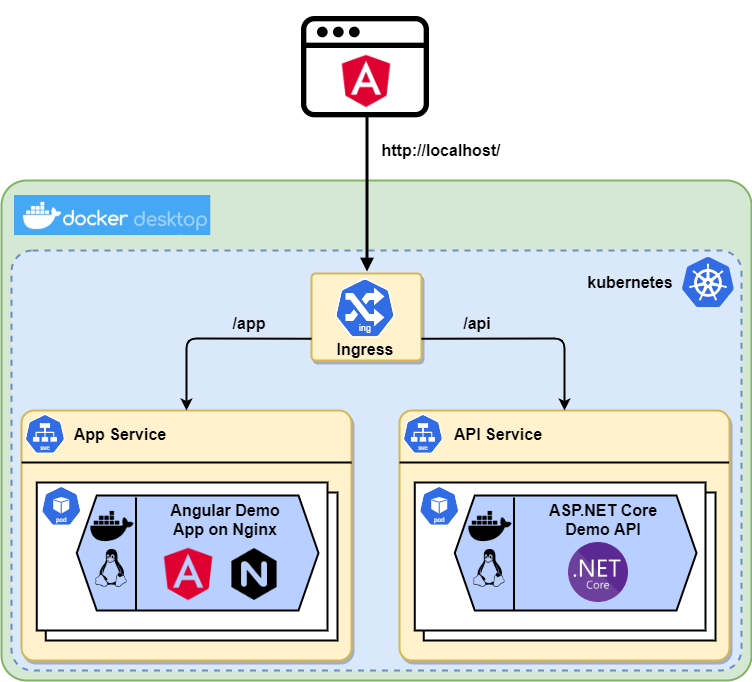
Contents
- Build the ASP.NET Core REST-API Backend
- Create the Angular Frontend App
- Dockerize the .NET Core API and the Angular App
- Deploy the Containers to Kubernetes on Docker Desktop
- Final Thoughts and Outlook
1. Build the ASP.NET Core REST-API Backend
Install Visual Studio Community (it’s free) with the ASP.NET and web development workload.
Create an ASP.NET Core 5.0 Web API project and call it “DemoApi”. Activate Docker and use the “Linux” setting. Disable HTTPS:
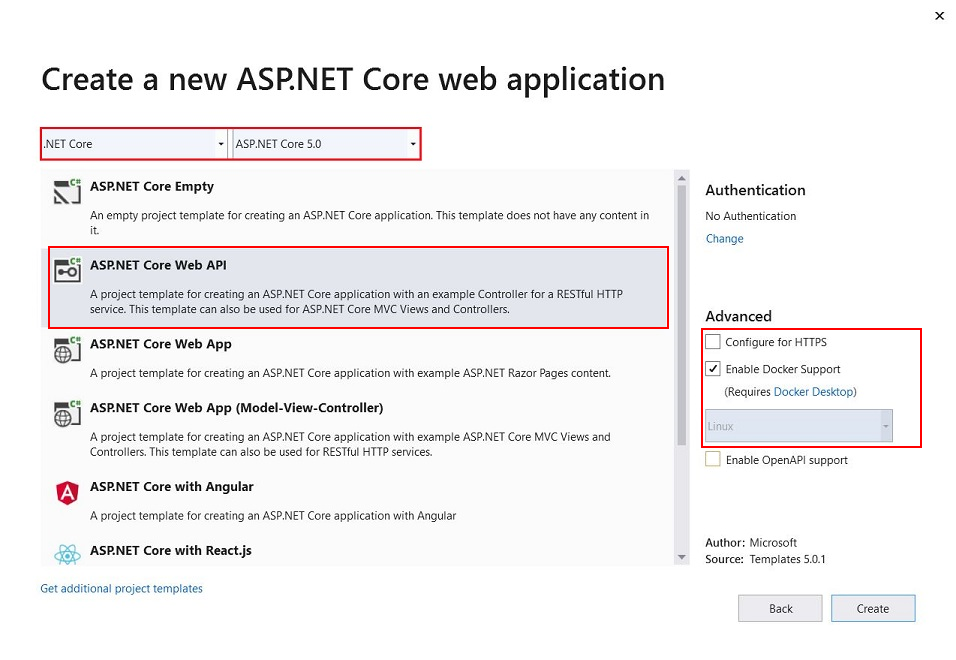
Add a new controller “DemoController”. It just returns “Hello World”:
Enable CORS for the Angular app on port 4200 (develop) and 80:
Edit launchsettings.json and change the URL of the “DemoApi” configuration to Port 80. Then choose the DemoApi configuration and start it:
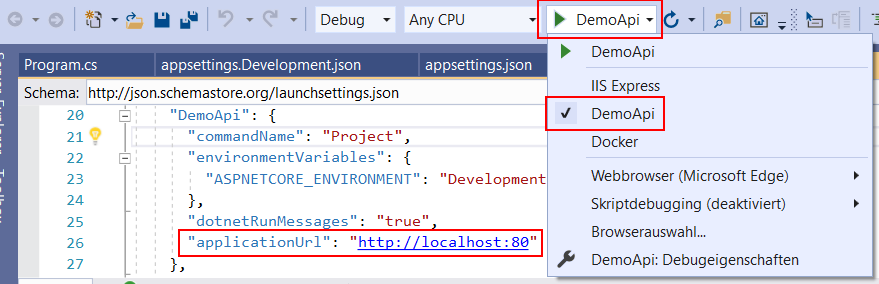
Test it in the browser:
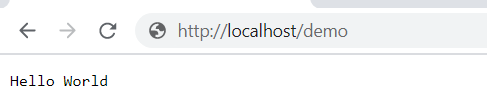
2. Create the Angular Frontend App
Download and install Node.js/NPM.
Create the folder C:\dev\demo and open a command prompt.
Install Angular (I used Angular 9 when writing this guide):
C:\dev\demo>npm install -g @angular/cli
Create the app:
C:\dev\demo>ng new DemoApp
Further reading: Understanding Angular and Creating Your First Application by
Call the REST-API
Adjust the code in C:\dev\demo\DemoApp\src\app
Add the HttpClientModule to app.module.ts:
Call the REST-API in app.component.ts:
Display the loaded data in app.component.html:
Test the app
Make sure the .NET Core API is running. Start the Angular app and open it in your browser to see if everything is working:
C:\dev\demo\DemoApp>ng serve
It should load and display the data “Hello World”:
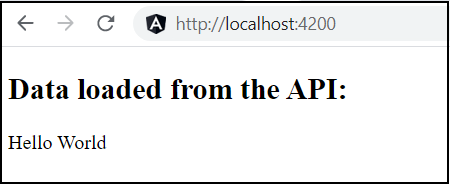
3. Dockerize the .NET Core API and the Angular App
I will show you two different ways to create and run a docker image. For the .NET Core API, you will use the UI. For the Angular App the command line.
Install Docker Desktop for Windows
Dockerize the .NET Core API
In the Visual Studio DemoApi project rename the file “Dockerfile” in the Project-Explorer to “dockerfile” (first character lowercase). Then right-click the dockerfile and select “Create Docker Image”.
Check the build output if everything worked:
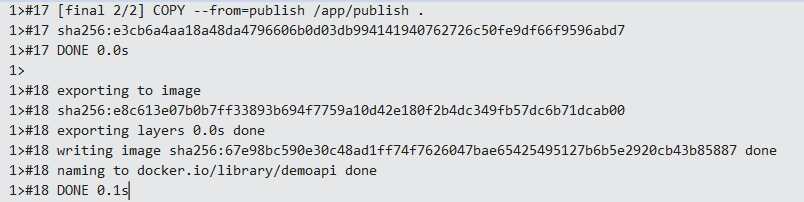
Stop your API project in Visual Studio if it is still running, because docker and Visual Studio can’t bind to port 80 at the same time.
Run the “latest” “demoapi” image in the Docker Desktop UI and map port 80:
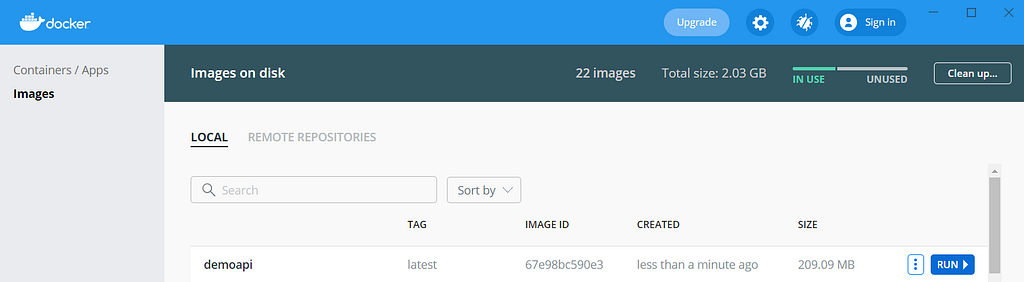
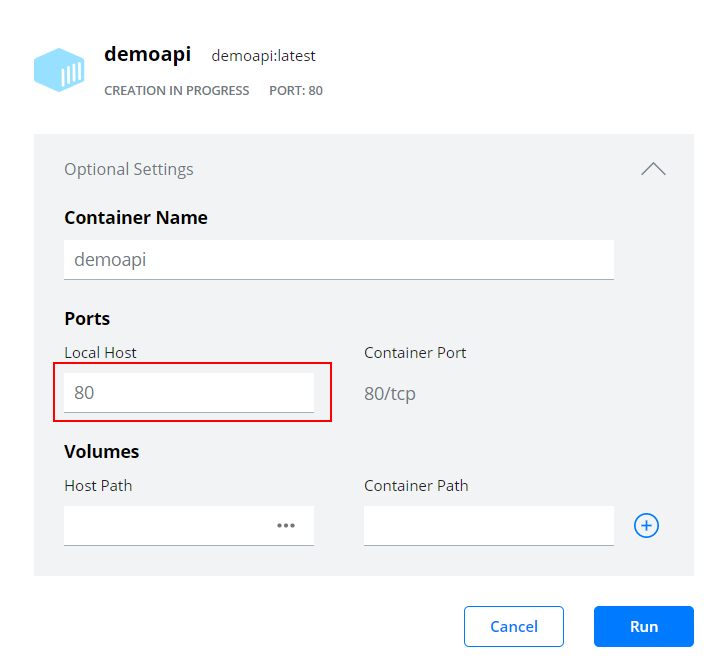
Further reading: How to deploy a .NET 5 API in a Kubernetes cluster by Ivan Porta
Dockerize the Angular App
Create the fileC:\dev\demo\DemoApp\dockerfile
Make sure the file “dockerfile” has no file extension
This will use NGINX to serve the app:
Create the file “.dockerignore” (with a dot at the beginning):
Open a command prompt and build the docker image (the dot at the end is important):
C:\dev\demo\DemoApp>docker build -t demoapp .
Stop your Angular app if it still running. Use the command line (the whole command in one line) to start the app in the container and publish it on port 4200:
C:\dev\demo\DemoApp>docker run --publish 4200:80 --name demoappcontainer demoapp
Further reading: Build and run Angular application in a Docker container by Wojciech Krzywiec
Test Both Containers
You can list your containers (your container IDs and timestamps will be different):

Start the app in the browser:
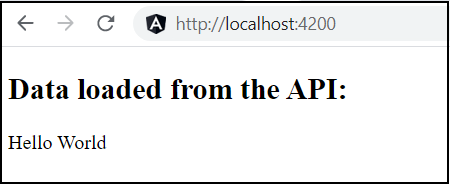
Stop both containers so the ports are not blocked for the next step of this guide:
C:\dev\demo\DemoApp>docker stop demoappcontainer
C:\dev\demo\DemoApp>docker stop demoapi
4. Deploy to Kubernetes on Docker Desktop
Enable Kubernetes in Docker Desktop:
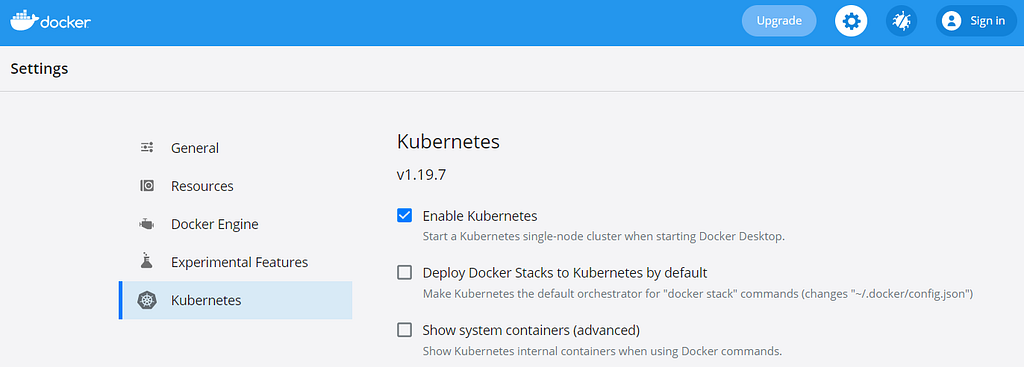
Install the NGINX Ingress controller (in one line on the command line):
C:\dev\demo>kubectl apply -f https://raw.githubusercontent.com/kubernetes/ingress-nginx/controller-v0.41.2/deploy/static/provider/cloud/deploy.yaml
See the NGINX Ingress Installation Guide for details
Create C:\dev\demo\kubernetes.yaml:
Apply the yaml:
C:\dev\demo>kubectl apply -f kubernetes.yaml
This will create two Kubernetes “Deployments” and for each deployment a “Service”. Both services are then exposed via an Ingress on port 80.
See the Kubernetes documentation for details
The first deployment uses the DemoAPI image and two replicas to show how it is possible to scale. The second deployment uses a single replica of the DemoApp. The Ingress routes to the service based on the path prefix:
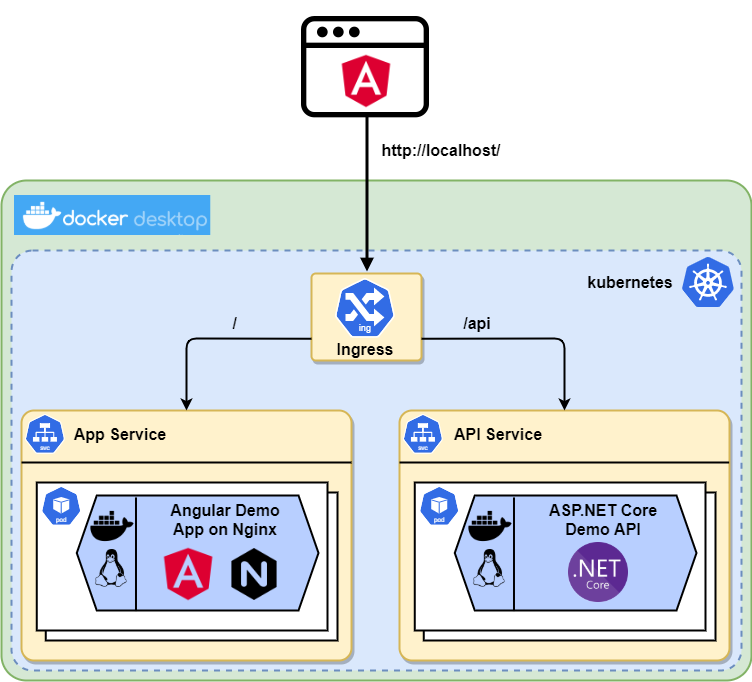
Test the Deployments
“get deployments” shows the deployments and number of pods:
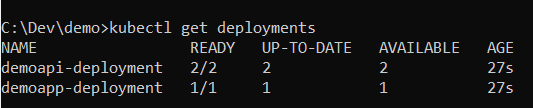
Open your browser on port 80(!) and test your Kubernetes deployments:
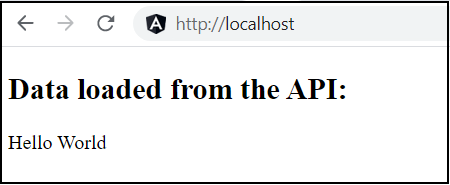
Further reading: How to deploy a .NET 5 API in a Kubernetes cluster by Ivan Porta
5. Final Thoughts and Outlook
You created the working basis for a microservice-based cloud architecture. But there is still a lot missing.
I will show you some of it in further posts: Kubernetes secrets, database/persistence, security aspects like SSL, inter-microservice communication, logging, debugging, CI/CD, Helm charts, (code) quality, (auto) scaling and self-healing, etc.
See my other stories how to:
- Sign-In with Google in Angular and use JWT based .NET Core API Authentication with Asymmetrically (RSA) Signed Tokens
- Use NSwag to Autogenerate a TypeScript Client to Access a .NET Core service API and use OpenAPI/Swagger to describe the API
Please contact me if you have any questions, ideas, or suggestions.
Kubernetes Angular ASP.NET Core Microservice Architecture was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Christian Zink
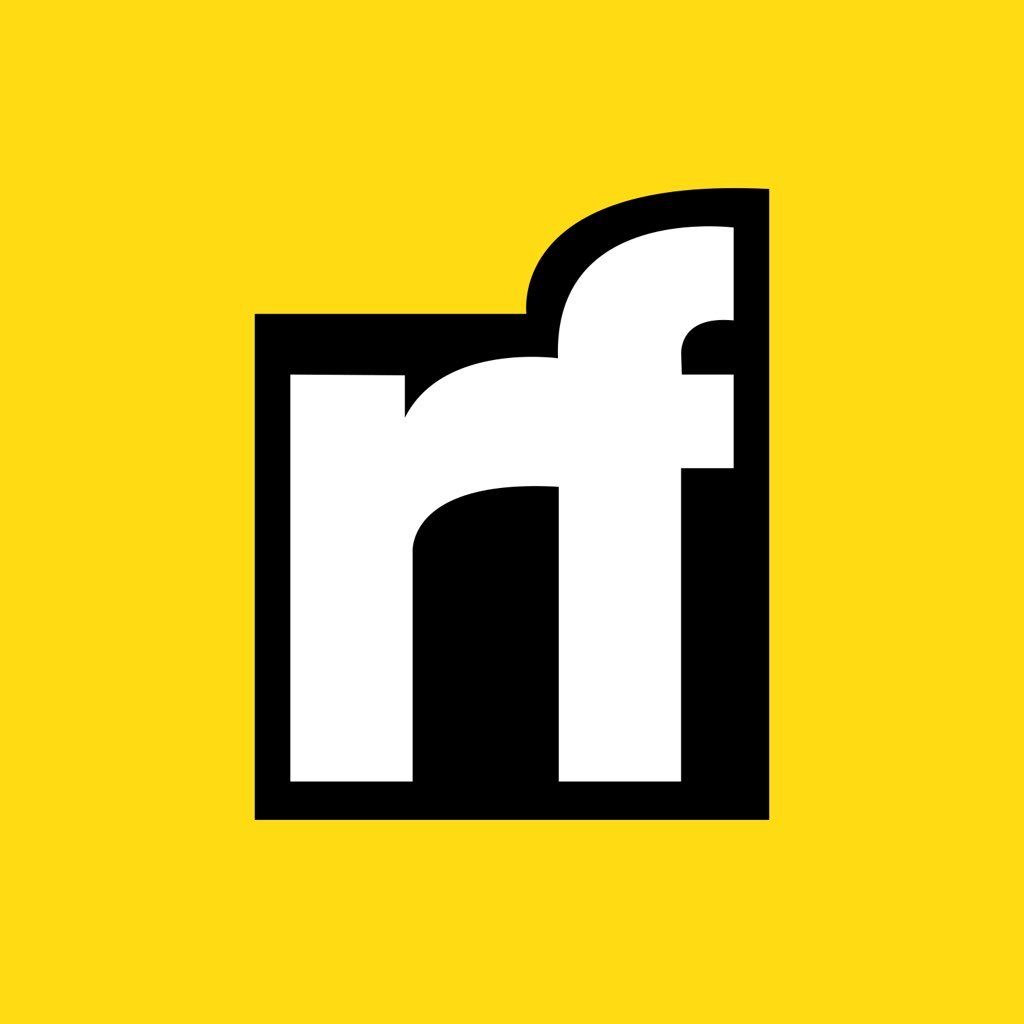
Christian Zink | Sciencx (2021-04-11T13:46:34+00:00) Kubernetes Angular ASP.NET Core Microservice Architecture. Retrieved from https://www.scien.cx/2021/04/11/kubernetes-angular-asp-net-core-microservice-architecture/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.