This content originally appeared on DEV Community and was authored by dev0928
Data classes is a new feature introduced in Python 3.7. This feature aims to reduce boilerplate code sucs as __init__()
and __eq()__
methods in user-defined Python classes. By using the dataclasses
module’s decorators and functions, one can create concise Python classes. Let’s review the use of the data classes feature with an example.
Employee class could be declared concisely like shown below with the data classes decorator.
from dataclasses import dataclass, field
@dataclass
class Employee:
id: int
first_name: str
last_name: str
email: str
base_pay: float
tax_rate: float = field(default=0.01, init=False)
hobbies: list = field(default_factory=list())
def calculate_pay(self):
net_pay = self.base_pay - (self.base_pay * self.tax_rate)
return net_pay
emp1 = Employee(1, 'John', 'Doe', 'john.doe@example.com', 10000, ['Fishing', 'Soccer'])
print(emp1) #Employee(id=1, first_name='John', last_name='Doe', email='john.doe@example.com', base_pay=10000, tax_rate=0.01, hobbies=['Fishing', 'Soccer'])
print(emp1.calculate_pay()) #9900.0
-
@dataclass
decorator above generatesinit
,repr
andeq
methods by default.@dataclass
generator without any parameters like declared above is equivalent to the below declaration:
@dataclass(init=True, repr=True, eq=True, order=False, unsafe_hash=False, frozen=False)
- If
order
parameter is set to true, it will generate boilerplate methodslt
,gt
,le
, andge
. Also, true value supplied to theunsafe_hash
parameter would generatehash
method. - Dataclasses use the
typing
module to define the data type of the declared class fields. However, if one wishes to not specify the field data types, they can usetyping.Any
for all of their fields in the class. - By default, all fields of the class are included in the generated methods. But this can be customizable using field declaration. For example,
tax_rate
field in the example is excluded from theinit
method.
tax_rate: float = field(default=0.01, init=False)
- Also, we can use the
default_factory
parameter to initialize complex data types such as list. - Dataclasses module has several convenience functions. For example,
dataclasses.fields
function provides a list of fields in the form of tuple. Also,dataclasses.asdict
returns a dictionary representation of the supplied object.
Dataclasses is one of the powerful features introduced in the recent versions of Python. Here is the link for learning more about it's capabilities so you can start using this feature in your projects.
This content originally appeared on DEV Community and was authored by dev0928
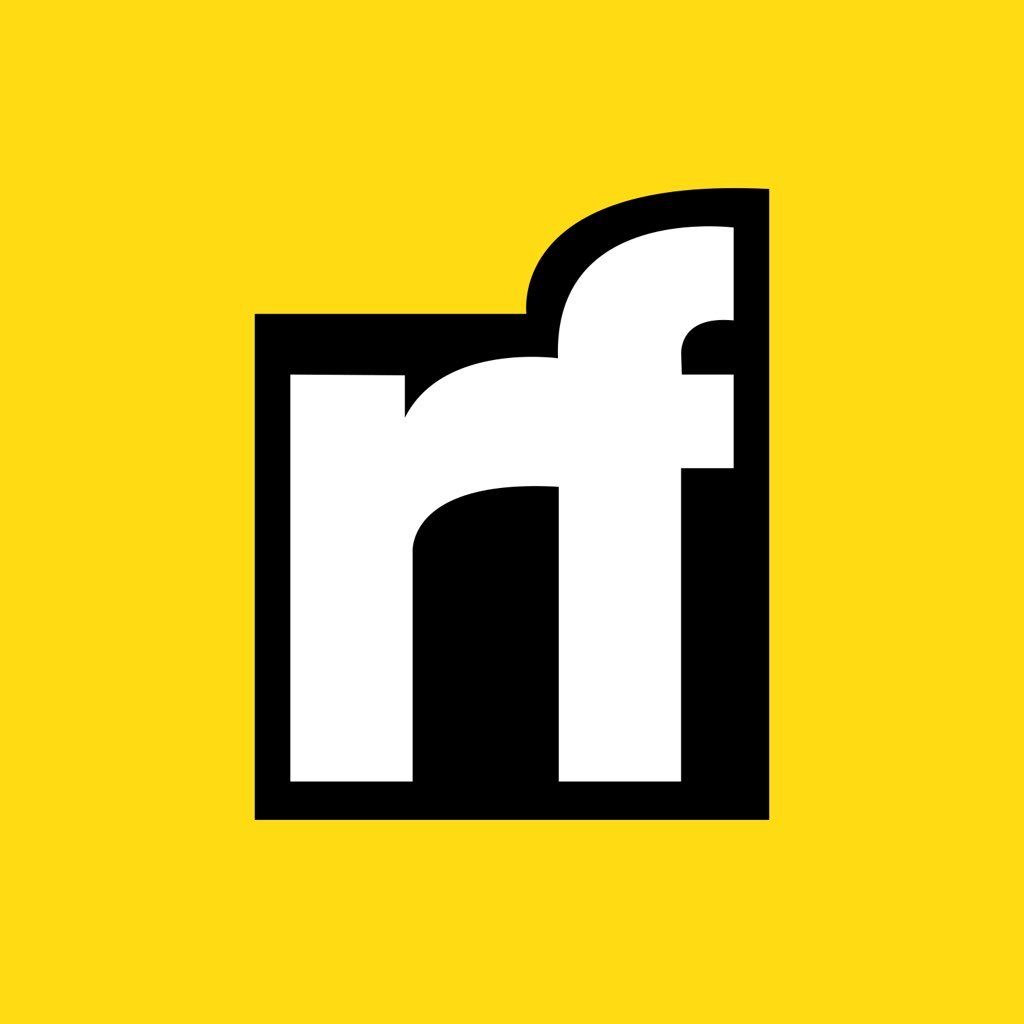
dev0928 | Sciencx (2021-04-12T00:59:33+00:00) What are Data Classes in Python?. Retrieved from https://www.scien.cx/2021/04/12/what-are-data-classes-in-python/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.