This content originally appeared on DEV Community and was authored by Randy Rivera
If you have a multi-dimensional array, you can use the same logic as the prior waypoint to loop through both the array and any sub-arrays.
- Here is an example:
function multiplyAll(arr) {
var product = 1;
for (var i = 0; i < arr.length; i++) {
for (var j = 0; j < arr[i].length; j++) {
product *= arr[i][j];
}
}
return product;
}
console.log(multiplyAll([[3,4],[1,2],[5,6,7]])); will display 5040
Here we modified function multiplyAll so that it returns the product of all the numbers in the sub-arrays of arr.
for (var i = 0; i < arr.length; i++) {
}
console.log(arr[i]); displays
[ 3, 4 ]
[ 1, 2 ]
[ 5, 6, 7 ]
for (var j = 0: j < arr[i].length; i++) {
}
console.log(arr[i][j]); displays
3
4
1
2
5
6
7
product *= arr[i][j];
console.log(product); // displays
3 (1 (beginning product = 1;) * 3 <-- arr[0][0]); 1 * 3
12 (now product is equal to 3 (product = 3) * 4 <-- arr[0][1]); 3 * 4
12 (product = 12) * 1 <-- arr[1][0]; 1 * 12
24 (product = 12) * 2 <-- arr[1][1]; 2 * 12
120 (product = 24) * 5 <-- arr[2][0]; 5 * 24
720 (product = 120) * 6 <-- arr[2][1]; 6 * 120
5040 (product = 720) * 7 <-- arr[2][2]; 7 * 720
return product; will display 5040
This content originally appeared on DEV Community and was authored by Randy Rivera
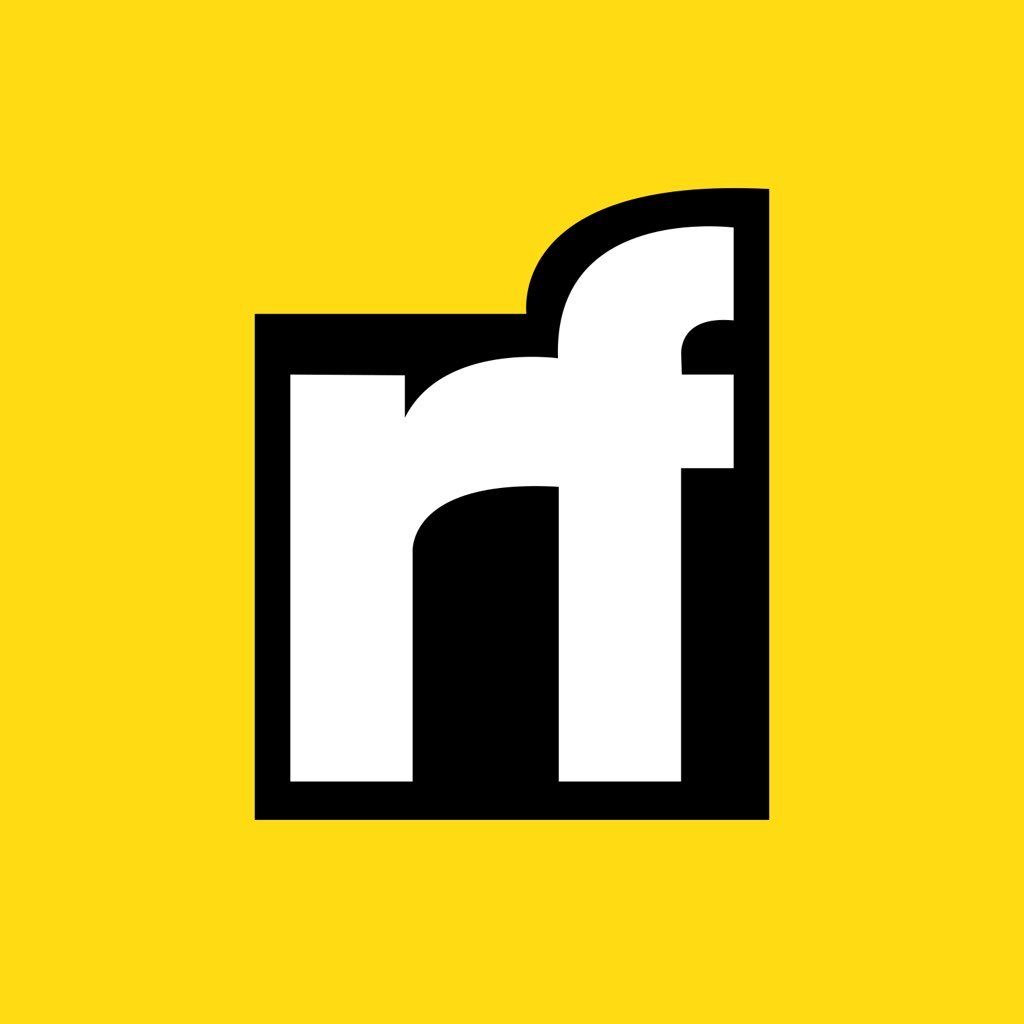
Randy Rivera | Sciencx (2021-04-22T21:36:32+00:00) Nesting For Loops. Retrieved from https://www.scien.cx/2021/04/22/nesting-for-loops/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.