This content originally appeared on DEV Community and was authored by Eric Chapman
I develop in Javascript, Python, PHP and Ruby. By far Ruby is my favorite programming language. Together let start a journey and revisit our Ruby foundations.
You want to learn Ruby or your Ruby is a bit rusty?
In this series we will start from the beginning and will learn every aspect of Ruby one step at a time.
Each post will include some theory but also exercise and solution.
If you have any questions/comments or your are new and need help, you can comment below or send me a message.
Strings Declaration
String variable represent any text data. A key point for the syntax of strings declaration is that they have to be enclosed in single or double quotes. The program will throw an error if they are not wrapped inside quotation marks.
# Bad
name = Mike # Will throw a error
# Good
name = 'Mike'
# Good
name = "Mike"
Number can also be represent as string
text_age = "45"
number_age = 45
The variable text_age is a string variable. It cannot be process in Ruby as a number like be use in addition or multiplication etc.
The number_age variable is integer number so that variable can be part of any Ruby number manipulations methods.
String Interpolation
String interpolation is replacing placeholders with values in a string literal.
For string interpolation to work. String have to be wrapped inside double quotation mark. Here a example
name = 'Mike'
message = "Hello #{name}"
# Hello Mike
If the last code snippet, the message variable will be process by Ruby before assignment. The #{name} placeholder will be replace by the containing variable value.
Inside placeholder #{} any Ruby expression can be use...
age = 45
message = "Your age in 2 years will be #{age + 2}"
# Your age in 2 years will be 47
In Ruby everything is an object!
You maybe have ear this before. What that mean for us the developer?
First, what is an object? An object refers to a particular instance of a class with their own methods and properties.
In Ruby type are define as classes, so for example if you have a string variable, it's an instance of the String class.
For example take this variable
message = "Hello World"
This 'message' variable will be dynamically type by Ruby as a string. That string is a class. So message is an instance of the class string.
In Ruby the String class already have many methods to help do basic and advance string manipulations.
That's also mean that 'message' variable will inherits all the methods and properties of his parent class (String).
Example of method call (syntax: object.method)
name = 'Mike'
puts name.upcase
# MIKE
'upcase' is a method of the String class. This method convert all the string characters to uppercase.
For now if you don't understand all that class instance thing THAT'S NORMAL! We will cover class and object later.
The only thing we need to understand for now is variable like a string variable have methods we can call to do some stuff automatically.
Here are some string methods available in Ruby.
puts 'Mike'.upcase
# MIKE
puts 'Mike'.downcase
# mike
puts 'mike'.capitalize
# Mike
puts 'Mike'.reverse
# ekiM
name = 'Mike Taylor'
puts name.length
# 11
Look how those methods name are self descriptive. We do not need any comments and any explanation to understand what each method do... Welcome to Ruby world!
More String methods
Now that we understand the basic we will start to learn more advance string methods.
The sub and gsub method
Ruby have a handy string method to replace part of a string.
message = 'The sky is blue'
puts message.sub 'blue', 'red'
# The sky is red
Note the method call have no parentheses to enclose parameters. In Ruby those are optionals.
# Valid
puts message.sub 'blue', 'red'
# Also valid
puts message.sub('blue', 'red')
The convention is to omit the parentheses unless the code seem clearer with them.
The sub method replace the first occurrence. The gsub method replace all the occurrences.
message = 'The sky is blue and the car is also blue'
puts message.gsub 'blue', 'red'
# The sky is red and the car is also red
Strip method
Remove white space before or after string
puts ' Welcome to Ruby World '.strip
# 'Welcome to Ruby World'
Chaining methods
It is possible to chain string methods
name = ' Mike Taylor '
puts name.sub('Mike', 'Paul').strip.downcase
# paul taylor
# In that specific situation, using the parentheses make the code easier to read
Exercice
Create a little program that:
- Input the user name and store the result in a variable
- Input the user password and store the result in a variable
- Remove password before or after white space
- Convert the password to lowercase
- Display user name and password but replace the password letter 'e' with a star
Here are the result
Enter user name: _Mike
Enter user password: _secret
The user name is Mike and his password is s*cr*t
Solution
print 'Enter user name: '
name = gets.chomp
print 'Enter user password: '
password = gets.chomp.strip.downcase
puts "The user name is #{name} and his password is #{password.gsub('e', '*')}"
Conclusion
That's it for today. The journey just started, stay tune for the next post very soon. (later today or tomorrow)
If you have any comments or questions please do so here or send me a message on twitter.
I am new on twitter so if you want to make me happy
Follow me: Follow @justericchapman
This content originally appeared on DEV Community and was authored by Eric Chapman
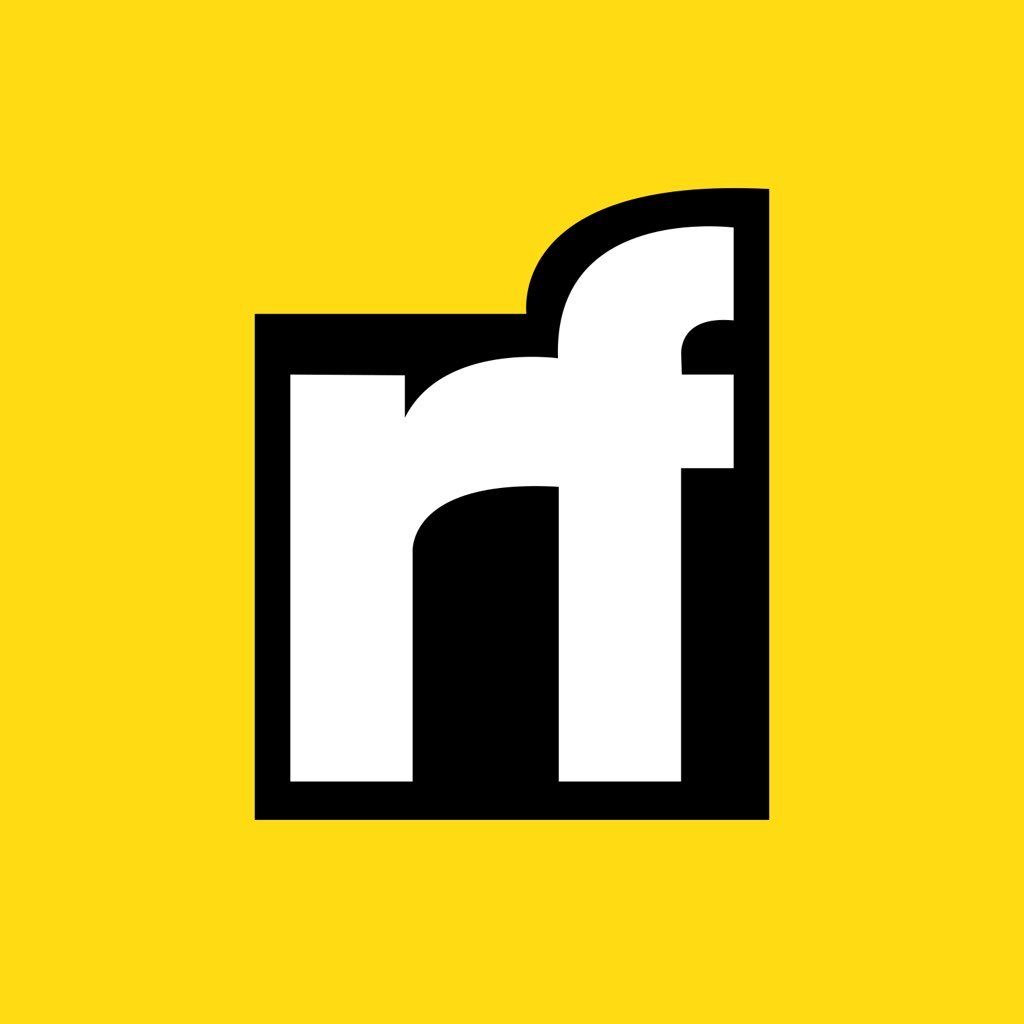
Eric Chapman | Sciencx (2021-04-25T00:42:42+00:00) Ruby 101: Strings. Retrieved from https://www.scien.cx/2021/04/25/ruby-101-strings/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.