This content originally appeared on DEV Community and was authored by Terry Threatt
When we develop programs for people to use in Javascript, We need to take advantage of data structures. These structures hold values of many types including strings, numbers, and objects.
One of the most common data structures that you will come across is the Array data structure. Mastering Arrays will put you in great shape to build useful programs as they are also used to build complex and abstract data structures as well.
The Array data type
Arrays hold many values of any data type. The structure is identified by a sequential list of values that can be accessed through a numbered index.
This helps to make it easy to traverse through this index very easily. Arrays are considered objects in Javascript which means they are called by reference.
// our first array
const arr = ["Nas", 13, {car: "Tesla"}]
// is this an array?
// isArray() is a helpful method to prove an Array
Array.isArray(arr) // return: true
// How many elements are in the array?
// .length will return the element count
console.log(arr.length) // return: 3
Working with Arrays
// Creating Arrays
// There are two ways to create arrays
const arr = [] // Array literal: This is the recommended way
const arr = new Array() // Array constructor
// Creating Array Elements
const primeNumbers = [2, 3, 5, 7, 11]
// .push() - Adds new elements to the end of the array
primeNumbers.push(13)
console.log(primeNumbers) // return: [2, 3, 5, 7, 11, 13]
// Accessing Arrays
// You can find array values through bracket notation:
// Arrays a zero-based indexes
console.log(primeNumbers[0]) // return: 2
console.log(primeNumbers[4]) // return: 11
// Changing Array Elements
// We can update an element with bracket notation
console.log(primeNumbers) // return: [2, 3, 5, 7, 11, 13]
console.log(primeNumbers[0]) // return 2
primeNumbers[0] = 1
console.log(primeNumbers[0]) // return 1
console.log(primeNumbers) // return: [1, 3, 5, 7, 11, 13]
// Deleting Array Elements
// .pop() - removes the last element in array
primeNumbers.pop() // return: 13
console.log(primeNumbers) // return: [1, 3, 5, 7, 11]
Array methods
Here is a list of common array methods
Array.prototype.shift()
Removes the first element from the array
const colors = ["blue", "red"]
colors.shift() // return: red
console.log(colors) // return: ["red"]
Array.prototype.unshift()
Adds an element to the end of the array
const fruits = ["apples", "oranges", "grapes"]
fruits.unshift("bananas") // return: 4
console.log(fruits) // return: ["bananas", "apples", "oranges", "grapes"]
Array.prototype.sort()
Sorts the elements for the array
const nums = [44, 33, 22]
nums.sort()
console.log(nums) // return: [22, 33, 44]
Array.prototype.filter()
Creates a new filtered array
const age = [15, 22, 43]
const legalAge = age.filter(age => age >= 21)
console.log(legalAge) // return: [22, 43]
Array.prototype.forEach()
Allows you to perform an operation to each element in the array
const sports = ["baseball", "basketball", "football"]
const favSport = sports.forEach(sport => {
console.log(`My favorite sport is ${sport}.`)
})
// return: My favorite sport is baseball.
// return: My favorite sport is basketball.
// return: My favorite sport is football.
Loops with Arrays
const nums = [ 11, 24, 36, 47, 53, 66]
function findEven(nums) {
const evenNums = []
for(let i = 0; i < nums.length; i++){
if(nums[i] % 2 === 0) {
evenNums.push(nums[i])
}
}
return evenNums
}
findEven(nums) // return [24, 36, 66]
Let's Chat About Arrays
Arrays are a key data structure to know and I hope this crash course was helpful in showing how to work Arrays. If you enjoyed this post feel free to leave a comment about your thoughts and experiences with Arrays.
Happy Coding,
Terry Threatt
This content originally appeared on DEV Community and was authored by Terry Threatt
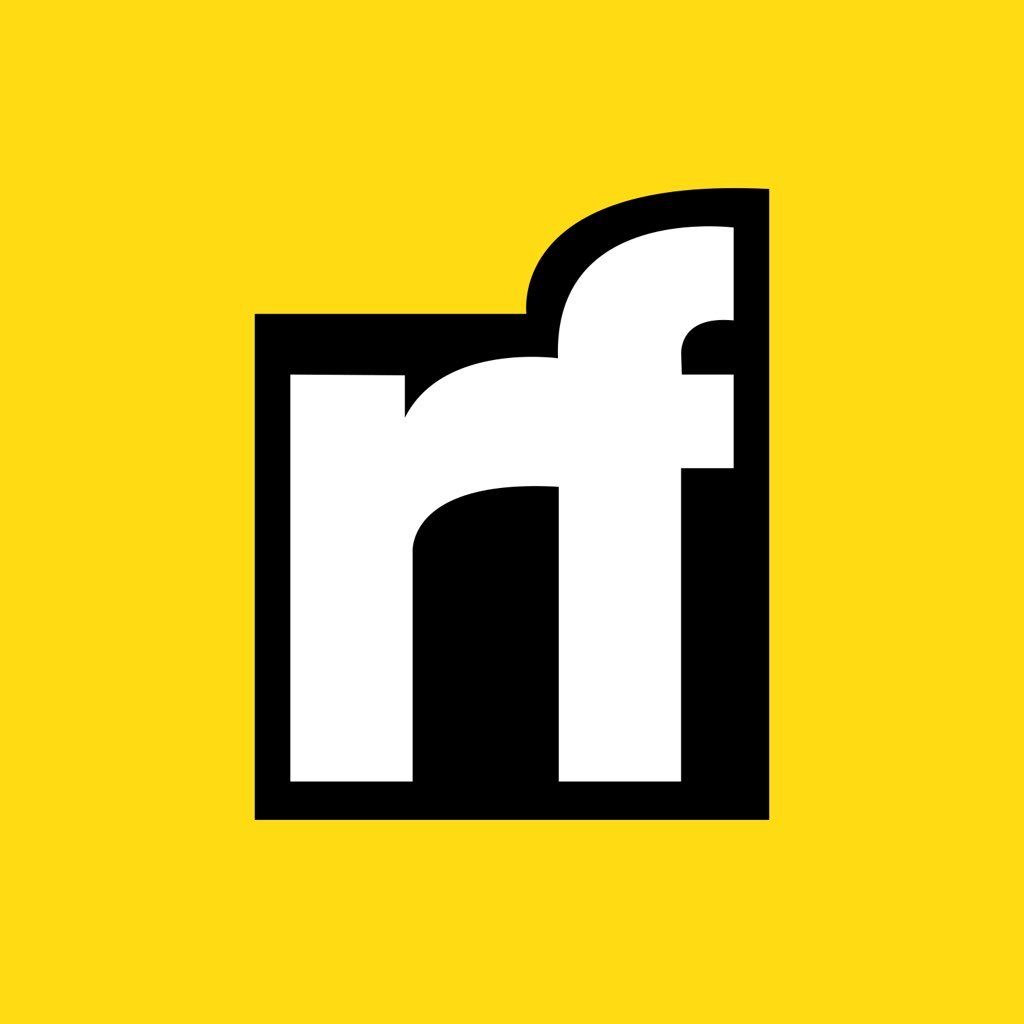
Terry Threatt | Sciencx (2021-05-01T21:40:58+00:00) Javascript Array Crash Course. Retrieved from https://www.scien.cx/2021/05/01/javascript-array-crash-course/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.