This content originally appeared on DEV Community and was authored by matt swanson
In almost all email programs, you can add a display name before your email address like so:
To: Matt Swanson <matt@example.com>
It’s a small touch, but it is a more human-readable way of addressing an email. Rails provides a helper utility to format email addresses in this style without resorting to manual string manipulation.
Usage
Use email_address_with_name
to add a name in-front on an email address in a standard way
ActionMailer::Base.email_address_with_name("swan3788@gmail.com", "Matt Swanson")
=> "Matt Swanson <swan3788@gmail.com>"
This helper is available in all Rails mailers.
class UserMailer < ApplicationMailer
default from: 'notifications@example.com'
def welcome_email
@user = params[:user]
mail(
to: email_address_with_name(@user.email, @user.display_name),
subject: 'You have a new message'
)
end
end
Options
This helper handles nil
gracefully as well.
ActionMailer::Base.email_address_with_name("swan3788@gmail.com", nil)
=> "swan3788@gmail.com"
And it handles escaping characters automatically:
ActionMailer::Base.email_address_with_name("mike@example.com", "Michael J. Scott")
=> "\"Michael J. Scott\" <mike@example.com>"
ActionMailer::Base.email_address_with_name("chip@example.com", 'John "Chip" Smith')
=> "\"John \\\"Chip\\\" Smith\" <chip@example.com>"
Additional Resources
Rails API: ActionMailer::Base#email_address_with_name
This content originally appeared on DEV Community and was authored by matt swanson
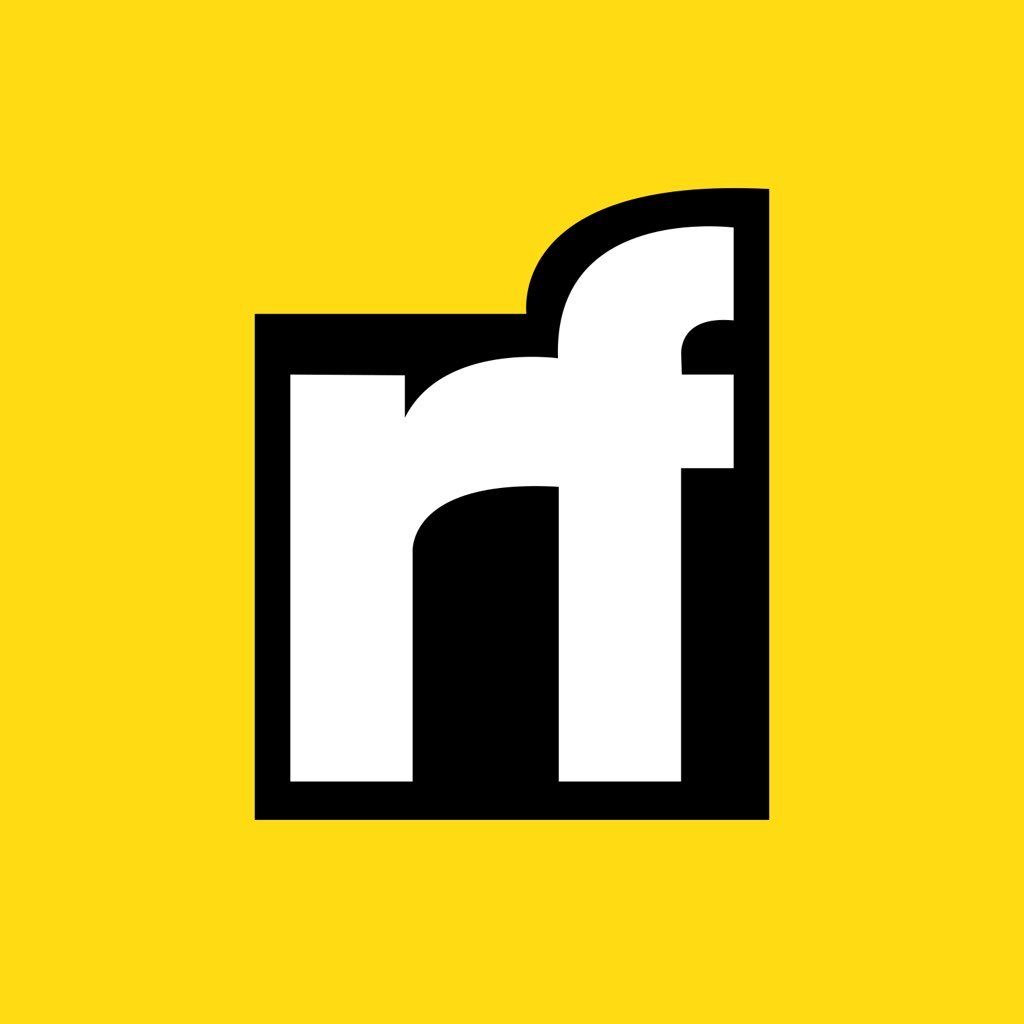
matt swanson | Sciencx (2021-05-03T13:00:00+00:00) Improving your Rails mailers with `email_address_with_name`. Retrieved from https://www.scien.cx/2021/05/03/improving-your-rails-mailers-with-email_address_with_name/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.