This content originally appeared on DEV Community and was authored by Mikey
In this article, we're going to create a Discord bot that can guide users through an automatic partnership process!
What's a Discord server partnership?
A discord server partnership is when two servers will send the other's invite link in a designated channel, to share members.
Our end product will look something like this:
The staff of your server will see this:
And will be able to click the checkmark or cross to accept or deny the request!
When it is accepted, it will automatically post the ad in a designated channel!
Installing discord.ext.forms ?
Discord.ext.forms is a module designed specifically to assist bot developers with making forms and surveys! To install it, you need to open a shell and type:
pip install discord-ext-forms
Once that's done, you're ready to start coding!
Coding the bot ⚙
To start this off, we will need to start our commands.Bot
instance.
from discord.ext import commands
import discord
bot = commands.Bot(command_prefix="!")
bot.run(TOKEN_HERE)
Now that we have our bot, let's make our partner
command!
from discord.ext import commands
import discord
bot = commands.Bot(command_prefix="!")
@bot.command()
async def partner(ctx):
...
bot.run(TOKEN)
We need to import the Form
class from discord.ext.forms to make our form for the partnership command!
from discord.ext.forms import Form
Now, let's start on our form! We likely want to ask for an invite link, a description, and an advertising server, but you can add or remove any of these questions!
In our command, let's make our form:
form = Form(ctx, "Partnership Request")
To add a question, we use the .add_question
method of the form.
form.add_question(
"What's the invite link to your server?", # The question which it will ask
"invitelink", # What the form will call the result of this question
"invite" # The type the response should be
)
Because we have invite
as the type, discord.ext.forms will check that the response is an invite and return an invite object.
Now, let's add our other two questions!
@bot.command()
async def partner(ctx):
form = Form(ctx, "Partnership Request")
form.add_question(
"What's the invite link to your server?", # The question which it will ask
"invitelink", # What the form will call the result of this question
"invite" # The type the response should be
)
form.add_question(
"What's a description of your server?",
"description"
)
form.add_question(
"What's your server's advertisement?",
"advertisement"
)
The final two don't have a type, because we just want the message's content.
Now, we'll want to begin the form!
results = await form.start()
results
will have 3 attributes:
invitelink
description
advertisement
These are the keys that we set these values to earlier!
Now, let's make our embed that shows the staff the submission:
embed = discord.Embed(
title=f"Partnership Request from {results.invitelink.guild.name}",
description=f"**Description:** {results.description}",
)
embed.set_thumbnail(url=results.invitelink.guild.icon_url)
embed.set_author(name=ctx.author, icon_url=ctx.author.avatar_url)
Now, let's get our partnerships channel so that we can send our embed there!
partnershipreq = bot.get_channel(partnership_request_channel_id_here)
Lastly, let's send it!
prompt = await partnershipreq.send(embed=embed)
For staff members to be able to accept and deny, we're going to need another form from discord.ext.forms: ReactConfirm
.
from discord.ext.forms import Form, ReactConfirm
The reason we saved prompt
earlier is because we're going to need it for the accept/deny process. Use ReactConfirm to create a new form called confirm
, like this:
confirm = ReactConfirm(message=prompt, bot=bot)
accepted = await confirm.start()
Now, the bot, after sending the message, will react with the ✔ and ❌ emoji, and wait for a staff member to choose one.
We're nearly there! Now we just have to send the ad, in an embed of course, into the partnership channel if it gets accepted.
if accepted:
partners = bot.get_channel(partners_channel_id_here)
em = discord.Embed(title=results.invitelink.guild.name,
description=results.advertisement, color=0x2F3136)
em.set_author(name=ctx.author, icon_url=ctx.author.avatar_url)
em.set_thumbnail(url=results.invitelink.guild.icon_url)
await partners.send(embed=em)
Now we're done! Our final code looks something like this:
from discord.ext import commands
import discord
from discord.ext.forms import Form, ReactConfirm
bot = commands.Bot(command_prefix="!")
@bot.command()
async def partner(ctx):
form = Form(ctx, "Partnership Request")
form.add_question(
"What's the invite link to your server?", # The question which it will ask
"invitelink", # What the form will call the result of this question
"invite" # The type the response should be
)
form.add_question(
"What's a description of your server?",
"description"
)
form.add_question(
"What's your server's advertisement?",
"advertisement"
)
results = await form.start()
embed = discord.Embed(
title=f"Partnership Request from {results.invitelink.guild.name}",
description=f"**Description:** {results.description}",
)
embed.set_thumbnail(url=results.invitelink.guild.icon_url)
embed.set_author(name=ctx.author, icon_url=ctx.author.avatar_url)
partnershipreq = bot.get_channel(partnership_request_channel_id_here)
prompt = await partnershipreq.send(embed=embed)
confirm = ReactConfirm(message=prompt, bot=bot)
accepted = await confirm.start()
partners = bot.get_channel(partners_channel_id_here)
em = discord.Embed(title=results.invitelink.guild.name, description=results.advertisement, color=0x2F3136)
em.set_author(name=ctx.author, icon_url=ctx.author.avatar_url)
em.set_thumbnail(url=results.invitelink.guild.icon_url)
await partners.send(embed=em)
bot.run(TOKEN)
If you want to see other use cases for this module, check it out here:
isigebengu-mikey
/
discord-ext-forms
A simpler way to make forms, surveys, and reaction input using discord.py.
This content originally appeared on DEV Community and was authored by Mikey
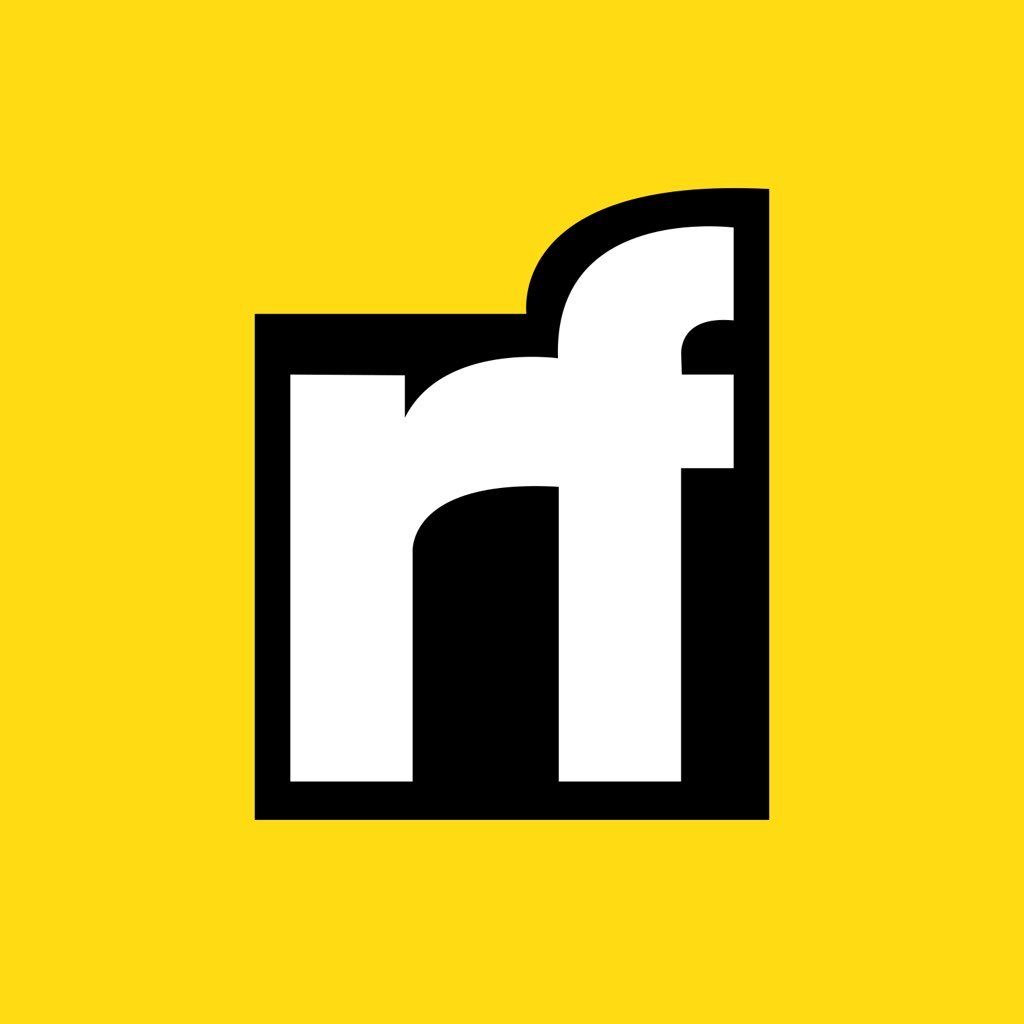
Mikey | Sciencx (2021-05-05T15:21:20+00:00) discord.py Project 4: ✍?Partnership Bot!. Retrieved from https://www.scien.cx/2021/05/05/discord-py-project-4-%e2%9c%8d%f0%9f%8f%bdpartnership-bot/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.