This content originally appeared on DEV Community and was authored by Youssef Allali
Install NodeJS and NPM
First thing we need to do is prepare an environnement for Express to run. We are going to install NodeJS and follow the installation instructions.
Prepare a workspace and start a project
Once we have installed Node.js and Node Package Manager (NPM) on our machine, let's open up a terminal window (or CMD, on Windows) in the desired folder or use the following command to create a folder and use it as a workspace:
$ mkdir ./hello_world
$ cd ./hello_world
Now, we are ready to start our first application, to do that, type in the following command:
$ npm init -y
What it basically does is create a file named package.json
that contains all the information about our project and its dependencies.
Install Express
Next, we need to install express module using NPM via the command:
$ npm install express --save
Now all we need is to create our main script, we'll be naming it index.js
since that's the default name (other conventional names can be app.js
or server.js
).
We can create a new empty file from the terminal using the following command:
touch index.js
Let's open our newly created file in any IDE or text editor (Notepad
, Notepad++
, Atom
...) but I would recommend using a sophisticated IDE like VS Code
and let's type in the following lines in order:
const express = require('express');
The first line would tell our app to import the module we are using (express).
const app = express();
This second line let us define express as a function
After that we need to define something called "a route" to the root of our website that will let us send an HTTP request to our server and GET a response that says Hello World!
:
app.get('/', (req, res) => {
res.send('Hello World!');
});
Last thing we need to do is instruct our app to be listening on a port, for example port 3000:
app.listen(3000)
Run the server app
Now let's head back to our terminal window and type in the following command which will compile our code and start our server.
$ node ./index.js
Check if it works
Finally, we can load http://localhost:3000/ in a browser to see the result.
This content originally appeared on DEV Community and was authored by Youssef Allali
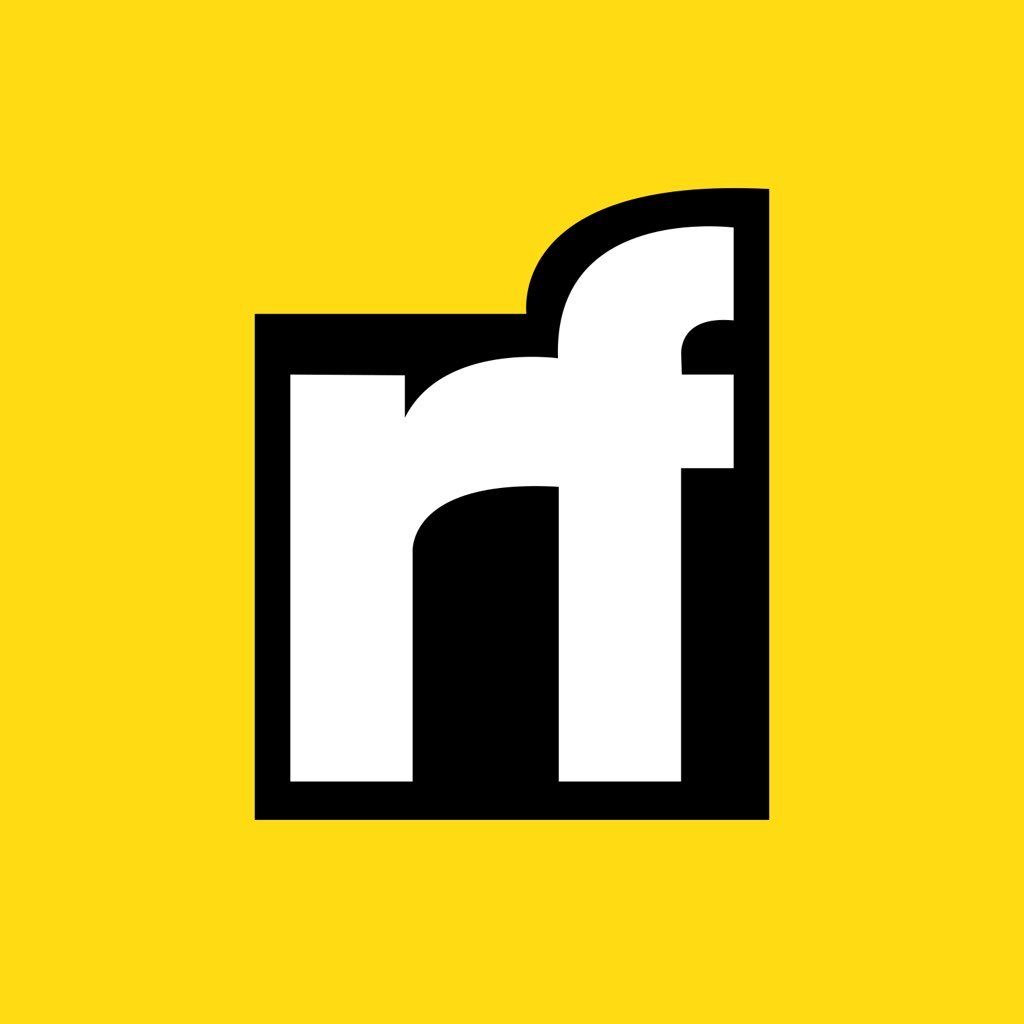
Youssef Allali | Sciencx (2021-05-07T05:21:36+00:00) Express JS Hello World. Retrieved from https://www.scien.cx/2021/05/07/express-js-hello-world/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.