This content originally appeared on DEV Community and was authored by Alexis Guzman
JavaScript offers many ways to capitalize a string to make the first character uppercase. Learn the various ways, and also find out which one you should use, using plain JavaScript.
1. chartAt + slice
The first way to do this is through a combination of cartAt()
and slice()
. With chartAt()
uppercases the first letter, and with slice()
slices the string and returns it starting from the second character:
const name = 'codax';
const nameCapitalized = name.charAt(0).toUpperCase() + name.slice(1);
// expected output: "Codax"
You can create a function to do that:
const capitalize = (s) => {
return s.charAt(0).toUpperCase() + s.slice(1)
}
capitalize('codax'); // "Codax"
2. Replace function
My favorite is with replace()
because I love regex (even if I'm not an expert) and regex is more customizable in general.
const name = 'codax';
const nameCapitalized = name.replace(/^\w/, (c) => c.toUpperCase()));
// expected output: "Codax"
You can also make it a function:
const capitalize = (s) =>
return s.replace(/^\w/, (c) => c.toUpperCase()));
}
capitalize('codax'); // "Codax"
Conclusion
For readability, I recommend the first option (chartAt
+ slice
), and for speed, I recommend the second option (replace
).
If you know other/better ways please leave below in the comments, or how to improve on the ones mentioned here.
Thanks.
This content originally appeared on DEV Community and was authored by Alexis Guzman
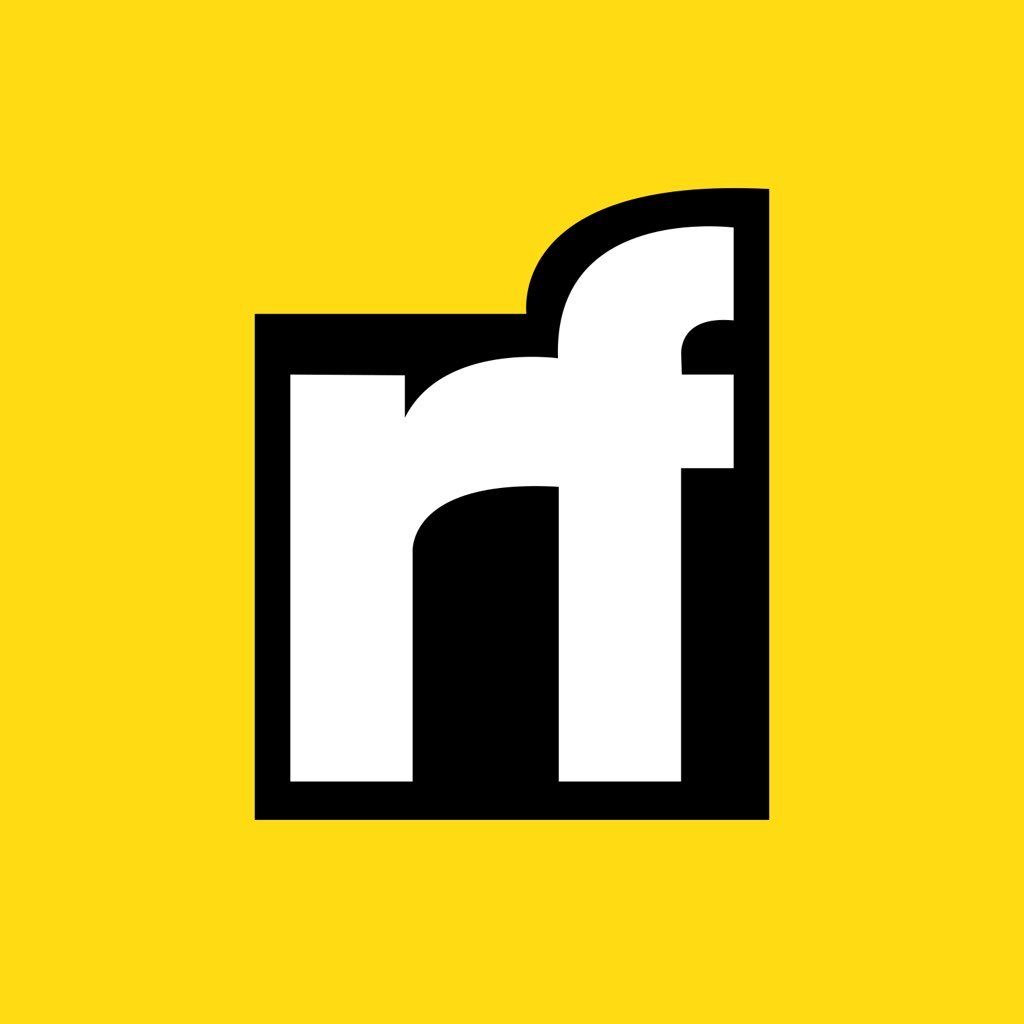
Alexis Guzman | Sciencx (2021-05-07T22:50:24+00:00) How to uppercase the first letter of a string in JavaScript. Retrieved from https://www.scien.cx/2021/05/07/how-to-uppercase-the-first-letter-of-a-string-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.