This content originally appeared on Level Up Coding - Medium and was authored by Yevhenii Datsenko
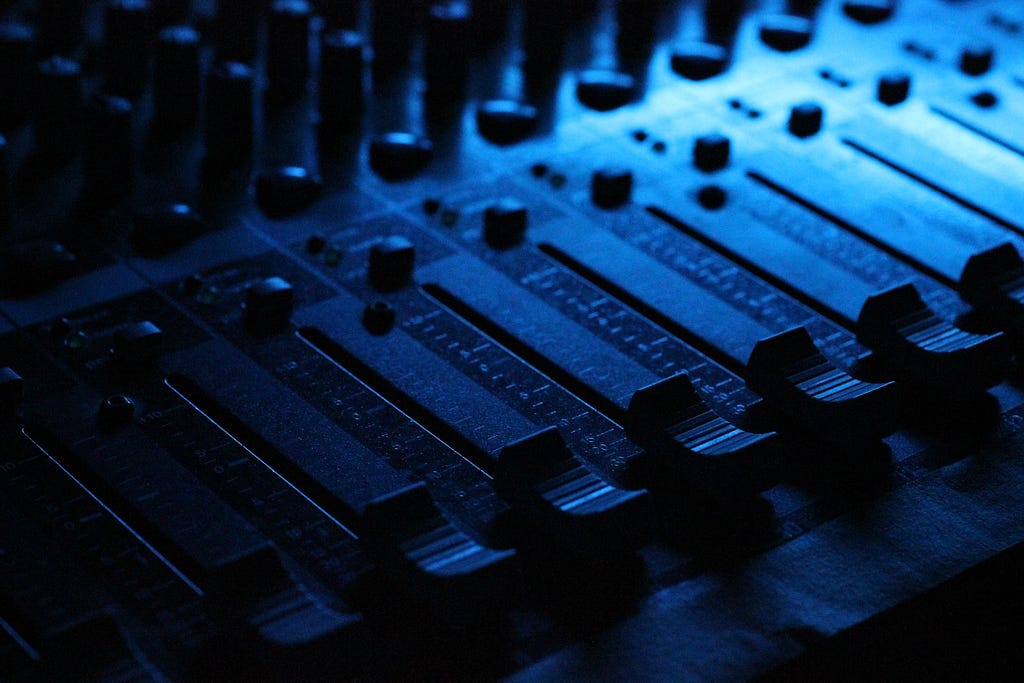
When we use custom sound for a notification channel, we might observe that system shows notifications without sound. Playing custom sound might be crucial for your application, let’s find out why it might happen.
Beginning
I faced an issue that users don’t hear the notification sounds for some notifications. So I started investigating the reason. I analyzed a changelog of the release with the issue and didn’t find anything explicit. After that, I realized that only users with Android 8.0+(API 26+) face this issue. It brought me to the idea that the issue may be related to notification channels.
Android Notification Channel
According to the docs:
Starting in Android 8.0 (API level 26), all notifications must be assigned to a channel. For each channel, you can set the visual and auditory behavior that is applied to all notifications in that channel. Then, users can change these settings and decide which notification channels from your app should be intrusive or visible at all.
Let’s imagine that we use the following code block to create and configure the channel:
private fun initNotificationChannel() {
if (Build.VERSION.SDK_INT < Build.VERSION_CODES.O) return
val channelName = ...
val channelDescription = ...
val importance = NotificationManagerCompat.IMPORTANCE_HIGH
val channel = NotificationChannelCompat.Builder(CHANNEL_ID, importance).apply {
setName(channelName)
setDescription(channelDescription)
setSound(
Uri.parse("${ContentResolver.SCHEME_ANDROID_RESOURCE}://${requireContext().packageName}/${R.raw.iphone_ringtone}"),
Notification.AUDIO_ATTRIBUTES_DEFAULT
)
}
NotificationManagerCompat.from(requireContext()).createNotificationChannel(channel.build())
}
It is a common way to create and configure the channel that we can find in the docs. However, we are setting a custom sound that is stored in the raw folder.
From the first glance, everything should be fine and this code works great. But in long run, it will cause a problem. We will see it in a few minutes.
Channel specifics
According to documentation, once a developer creates the notification channel, the dev can modify only a few things.
After you create a notification channel, you cannot change the notification behaviors — the user has complete control at that point. Though you can still change a channel’s name and description.
Fan fact, if we create a channel with ID “A”, delete it, and create a new channel with ID “A” — the framework will restore the previously deleted channel with this ID. You can find more in the docs here.
The second chance to spot the issue
Take a look at the code below and try to understand what could cause missed notification sound after update to the next app version.

If you don’t see, it is not a problem. In any case, I will show you in a second.
The problem
I was creating the notification channel with a custom sound URI that points to a specific ID in the R file. However, IDs in the R file might change after rebuild. It doesn’t happen often. I reproduced in the following steps:
- Add\remove a folder in the app resources, or add a file in the same folder before the target one
- Clear the Gradle cache
- Build and run the app
It caused IDs regeneration and the notification channel was pointing to the old ID. That is it :)
How to fix
First of all, we should remove the notification channel and create a new one with a new notification channel id.
Secondly, we should modify a sound URI and use the raw file by its name.
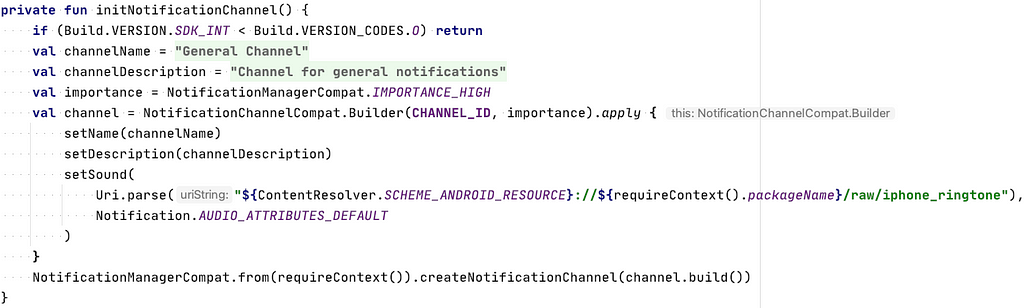
Conclusion
I spend a few days identifying this issue, hope it will be useful for someone. However, missed notification sound may be caused by: setting low priority/importance level to notification/channel; muting the notification channel by a user; interrupting by another notification sound, etc.
This solution works fine with obfuscated code. By the way, make sure that your file will survive resource shrinking. In this example, I am using the ID of the sound file for creating notification sound for users on Android pre 8.0. In this case, the file is used from the code so won’t be removed.
If you like the article follow me on Twitter)
The project sample could be found on GitHub. Take a look at the commits, they will provide additional information on when the sound was broken and when it was fixed.
A reason why custom notification sound might not work on Android was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Yevhenii Datsenko
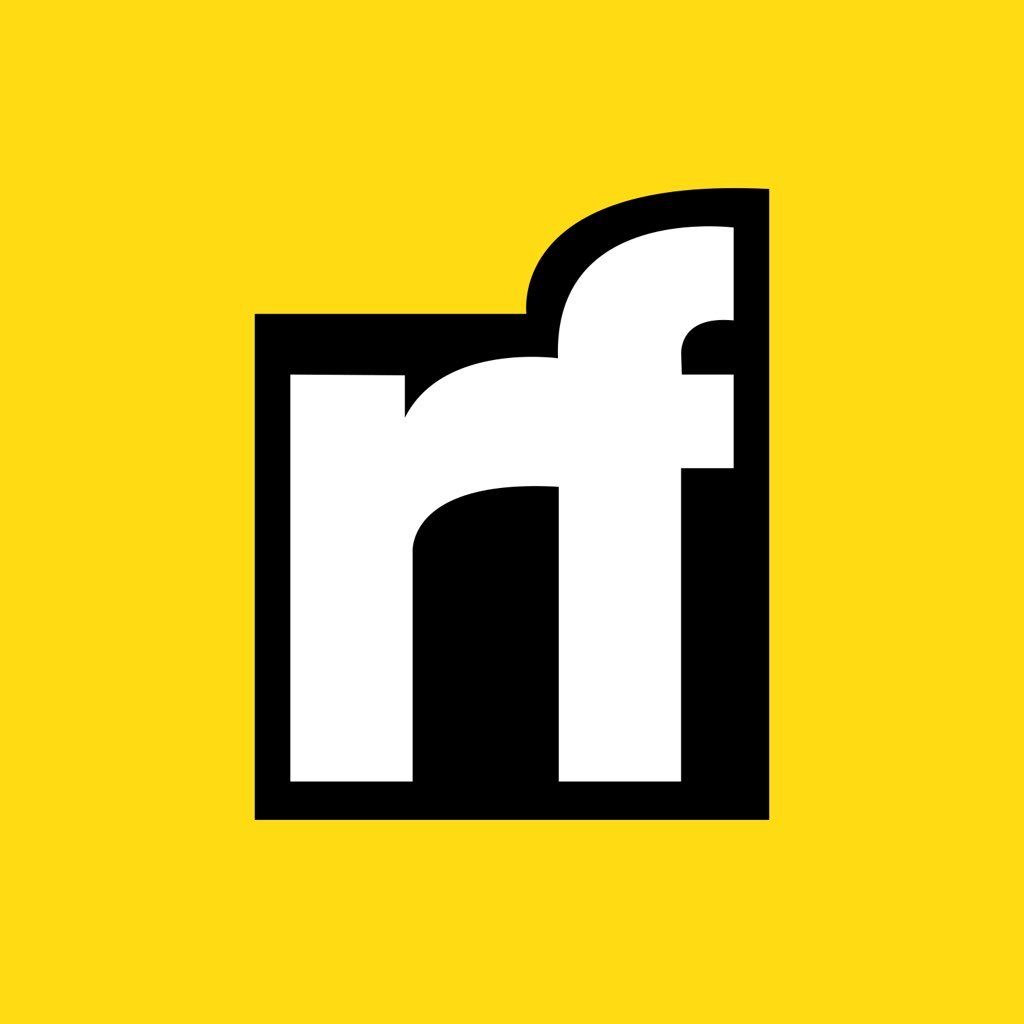
Yevhenii Datsenko | Sciencx (2021-06-29T00:25:47+00:00) A reason why custom notification sound might not work on Android. Retrieved from https://www.scien.cx/2021/06/29/a-reason-why-custom-notification-sound-might-not-work-on-android/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.