This content originally appeared on DEV Community and was authored by Dias
The ternary operator is a nice way to write concise value assignments without having to write a more lengthy if/else
.
For example:
// This...
let value;
if (test) value = 1;
else valeu = 2;
// can be written as this:
const value = test ? 1 : 2;
However it's easy to misuse the ternary operator for things where simpler operators could often have been a better choice. So here are some alternatives for common mistakes.
Static true/false assignments:
const value = test ? true : false;
// can be replaced by boolean casting:
const value = !!test;
// or even
const value = Boolean(test); // I prefer the shorter alternative
Nullable assignment (falsy case)
const value = test ? test : null;
// can be written like this
const value = test || null;
Note: The code above will return null as long as
test
ortest
are falsy.
Nullable assignment (nullish case)
const value = test !== null || test !== undefined ? test : null;
// can be written like this:
const value = test ?? null;
By the way...
const test = a === null || a === undefined;
// can be simplified as:
const test = a == null;
Checking for undefined
I have seen this a few times. I promise.
const value = obj ? obj.a : undefined;
// which can simply be:
const value = obj && obj.a;
// or in more recent implementations
const value = obj?.a;
The ternary operator with disability
This is my favorite one, and also an honest mistake. Some people get overexcited with the simplicity of the ternary operator and might think it is just a "shorter" if/else
statement.
let value;
test ? value = 8 : null;
// when they meant
if (test) value = 8;
The single-line if
statement is simple and clean enough for that purpose, and we know test ? value = 8
will not work. The ternary operator needs to have an else
return value. If you don't need it, use a single-line if
.
Wrapping up...
In a nutshell, if your ternary operator does not have a structure like the one below, you should raise an eyebrow and check if there really aren't other simpler alternatives.
const value = test ? otherValue : anotherValue;
Can you think of other examples you have seen of poor use of the ternary operator? Please let me know in the comments below.
This content originally appeared on DEV Community and was authored by Dias
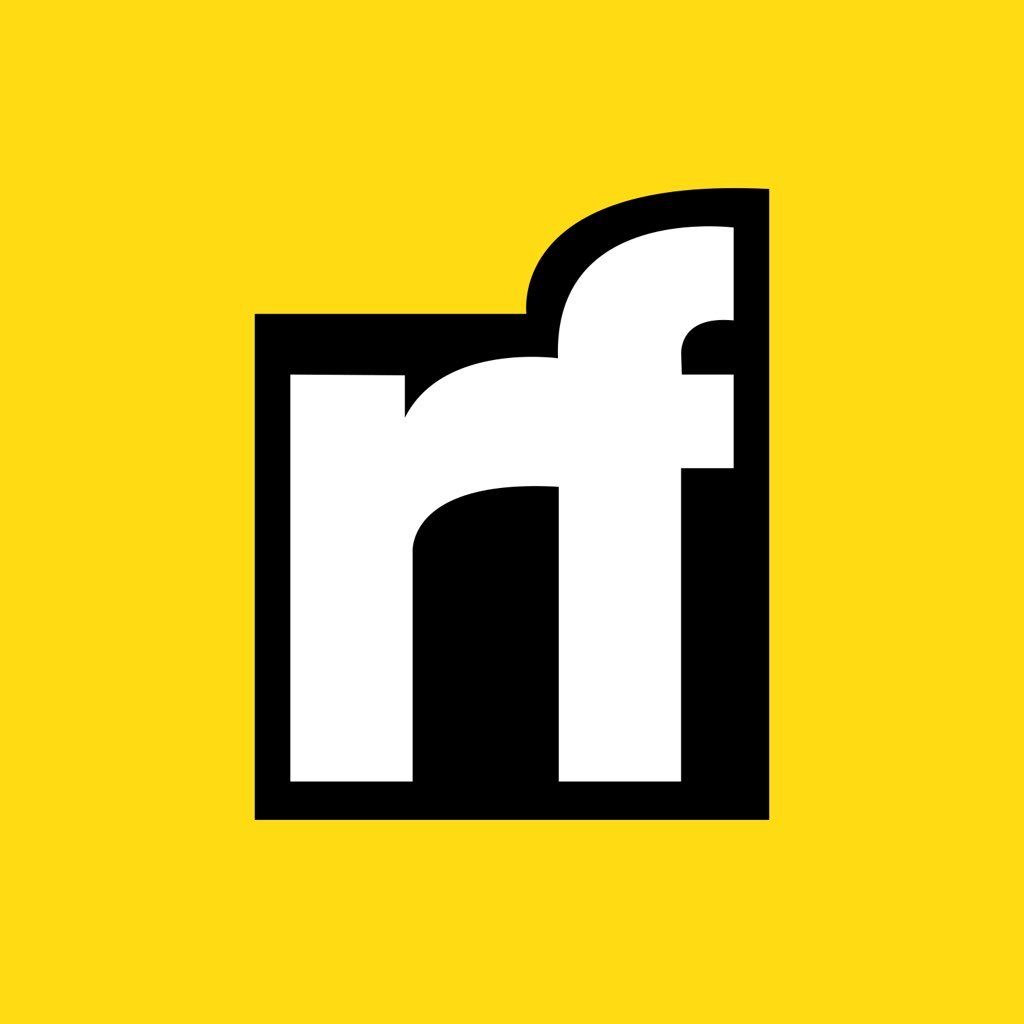
Dias | Sciencx (2021-07-01T03:35:15+00:00) Ternary Operator: Better Alternatives. Retrieved from https://www.scien.cx/2021/07/01/ternary-operator-better-alternatives/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.