This content originally appeared on DEV Community and was authored by hwangs12
I have a story to tell but the story is different each time I am in a different universe. So, I want to show audiences those stories whenever they travel through different universes. For example, the story in finite vector space will be a little bit different from inifinite vector space. In another example, Disney world will have different themes and rides from Universal Studios.
My story is different a set of math universes that are rather focused on the different math textbooks and different proofs that each textbook brings in each book. So, I need to control the title of the book state and the axioms in each book state. I wanted to sync it and here it is how I did it.
Collection of Components
import React, { useState } from "react";
import Hookers from "./Hookers";
import Statements from "./Statements";
const data = [
{
title: "Calculus by Michael Spivak",
statements: [
{ id: 1, statement: "square root of two is irrational" },
{ id: 2, statement: "there are infinite number of prime numbers" },
{ id: 3, statement: "y=x^2 is a continuous at 0" },
{ id: 4, statement: "e is irrational" },
{ id: 5, statement: "pi is irrational" },
],
},
{ title: "Linear Algebra by Stephen Friedberg", statements: [] },
{
title: "Contemporary Abstract Algebra by Joseph Gallian",
statements: [],
},
];
function Components() {
const [repo, setRepo] = useState(data[0]);
const handleClick = () => {
if (repo.title === data[0].title) {
setRepo(data[1]);
} else if (repo.title === data[1].title) {
setRepo(data[2]);
} else {
setRepo(data[0]);
}
};
console.log(repo);
return (
<>
<div className="header">
<Hookers title={repo.title} handleClick={handleClick} />
</div>
<div className="mainbody">
<Statements statements={repo.statements} />
</div>
</>
);
}
export default Components;
Hookers Components
import React from "react";
const Hookers = (props) => {
return (
<>
<div className="title">
<h1>{props.title}</h1>
</div>
<button className="btn" onClick={props.handleClick}>
CLICK
</button>
</>
);
};
export default Hookers;
Statements Component
import React, { useState, useEffect } from "react";
const Statements = (props) => {
const [axioms, setAxioms] = useState(props.statements);
useEffect(() => {
setAxioms(props.statements);
}, [props.statements]);
const removeItem = (id) => {
let unremovedItem = axioms.filter((sentence) => {
return sentence.id !== id;
});
setAxioms(unremovedItem);
};
return (
<>
<ol>
{axioms.map((sentence) => {
const { id, statement } = sentence;
return (
<li key={id} className="sentences">
<p>
<strong>{statement}</strong>
</p>
<button>EDIT</button>
<button onClick={() => removeItem(id)}>
REMOVE
</button>
</li>
);
})}
</ol>
<div className="form">
<input type="text" name="axiom" id="" />
<button>ADD</button>
</div>
</>
);
};
export default Statements;
This content originally appeared on DEV Community and was authored by hwangs12
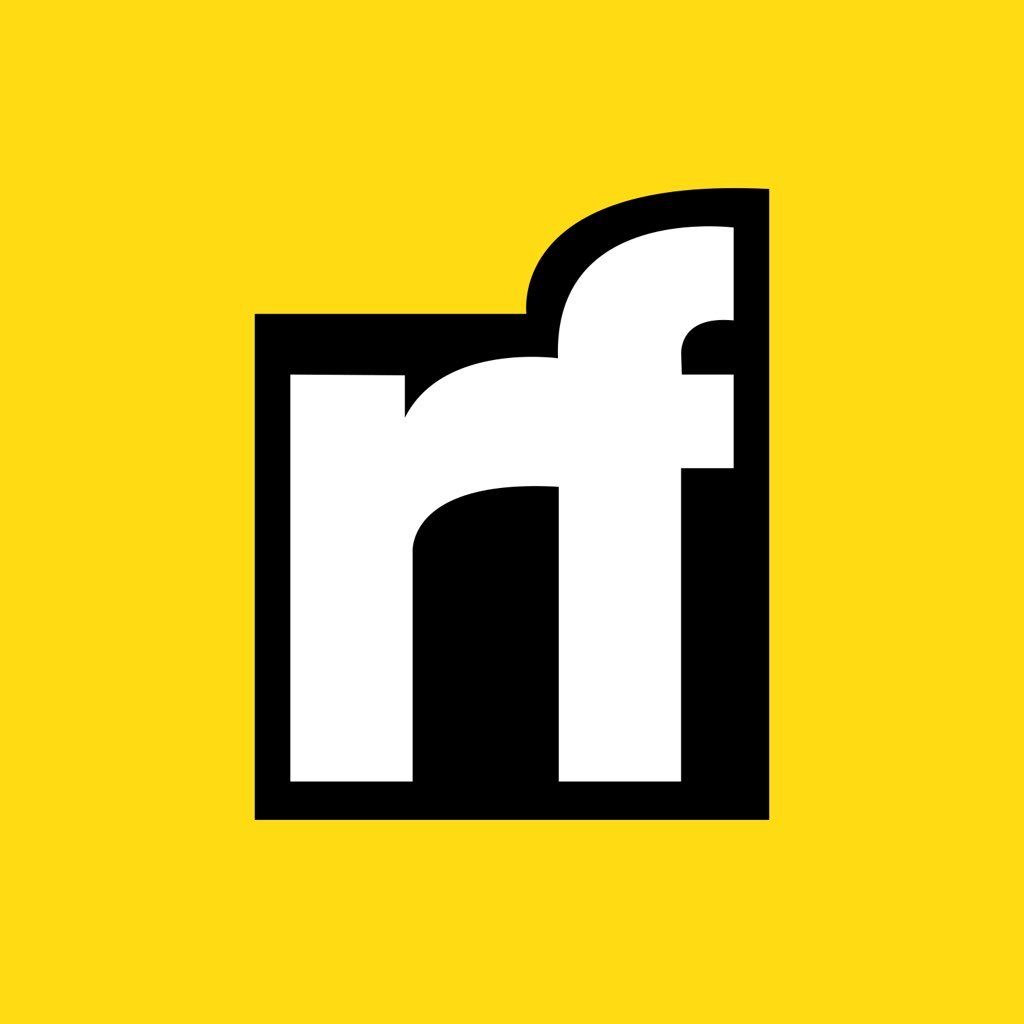
hwangs12 | Sciencx (2021-07-22T03:12:53+00:00) Daily React – 2: State Sharing. Retrieved from https://www.scien.cx/2021/07/22/daily-react-2-state-sharing/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.