This content originally appeared on Bits and Pieces - Medium and was authored by Charuka Herath
Make Your Business Logic Independent From UI Components
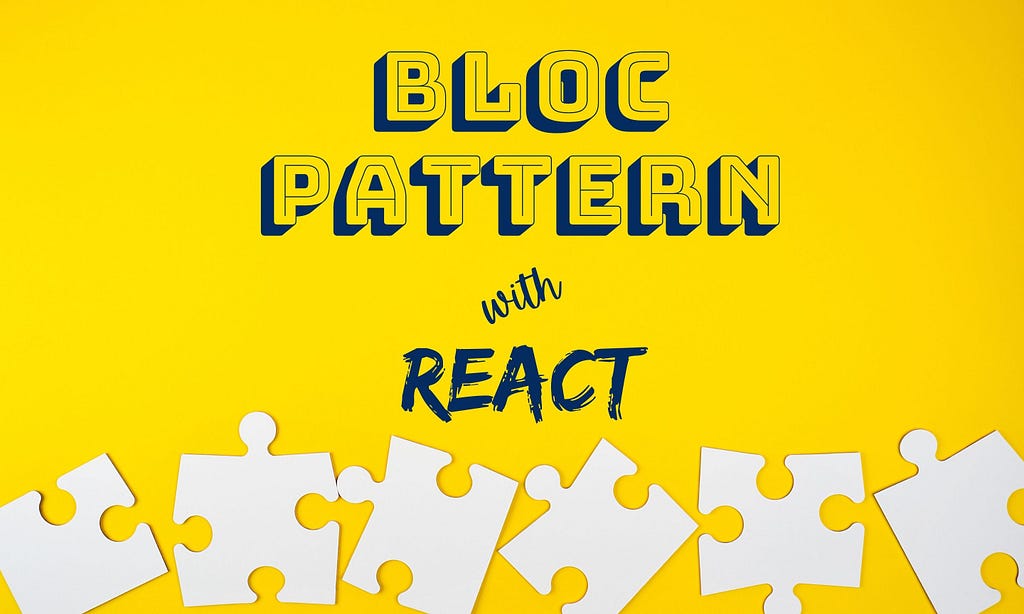
Initially, the Business Logic Component (BLoC) pattern was introduced by Google as a solution to handle states in Flutter applications. It allows you to reduce the workload on UI components by separating the business logic from them.
Over time, other frameworks also started to support the BLoC pattern. And, in this article, I will discuss how we can use this BLoC pattern with React.
Benefits of Using BLoC Pattern with React
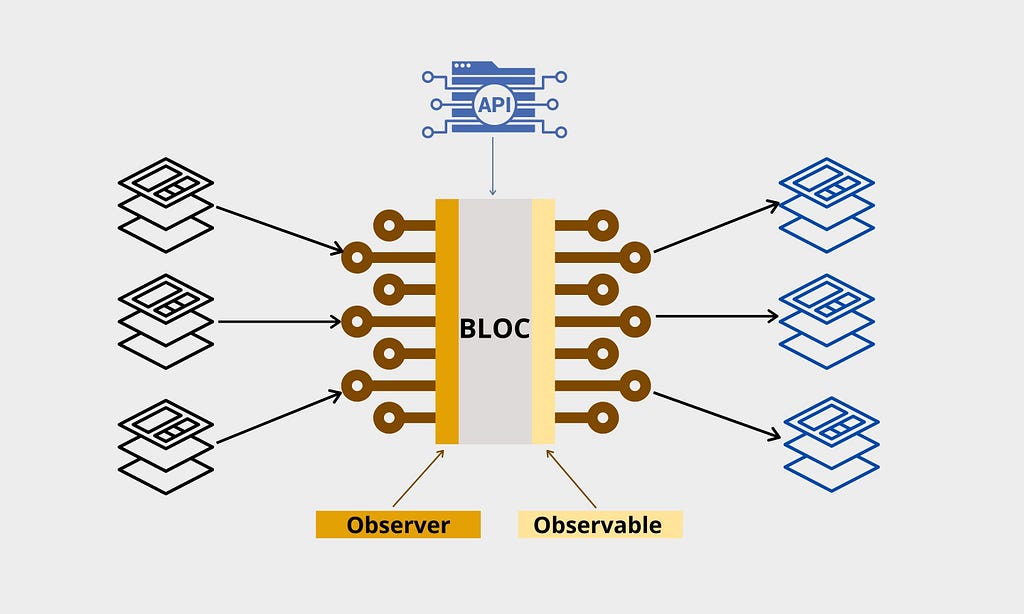
The concept behind the BLoC pattern is straightforward. As you can see in the above figure, business logic will be separated from UI components. First, they will send events to the BLoC using an observer. And then, after processing the request, UI components will be notified by the BLoC using observables.
So, let’s look at the advantages of this approach in detail.
1. Flexibility to update application logic
When the business logic is standalone from UI components, the impact on the application will be minimum. You will be able to change the business logic any time you want without affecting the UI components.
2. Reuse logic
Since the business logic is in one place, UI components can reuse logic without duplicating the code so that the simplicity of the app will increase.
3. Ease of testing
When writing test cases, developers can focus on the BLoC itself. So the code base is not going to be messed up.
4. Scalability
Over time, application requirements may change, and business logic can keep growing. In such situations, developers can even create multiple BLoCs to maintain the clarity of the codebase.
Moreover, BLoC patterns are platform and environment independent so that developers can use the same BLoC pattern in many projects.
Concept into Practice
Let’s build a small counter app to demonstrate the usage of the BLoC pattern.
Step 01: Create a React application and structure it.
First, we need to start by setting up a React app. I will name it bloc-counter-app. Also, I will be using rxjs as well.
// Create React app
npx create-react-app bloc-counter-app
// Install rxjs
yarn add rxjs
Then you need to remove all unnecessary code and structure the application as follows.
- Blocs — Keep all the bloc classes we need.
- Components — Keep the UI components.
- Utils — Keep utility files of the project.
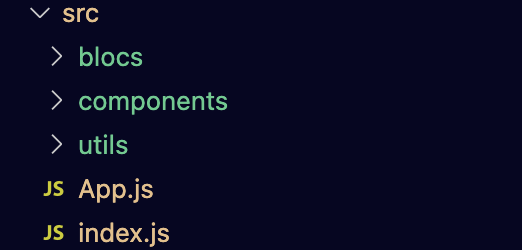
Step 02: Implementation of the BLoC.
Now, let’s implement the BLoC class. The BLoC class will be responsible for implementing all subjects related to business logic. In this example, it is responsible for the counter logic.
So, I have created a file named CounterBloc.js inside the bloc directory and used a pipe to pass the counter to the UI components.
As you can see, there is simple logic in this class. However, when an app grows in size, imagine the complexity if we do not separate the business logic.
Step 03: Adding more beauty to the BLoC by an intermediate class.
In this step, I will create the StreamBuilder.js inside the utils directory to handle the counter request from the UI. Moreover, developers can handle errors and implement customer handlers within this.
In the AsyncSnapshot class, we can initialize a constructor, handle our data (check availability, etc. ), and handle errors. But in this example, I have only returned the data for ease of demonstration.
The initial data is passed into AysncSnapshot class and stored in the snapshot state for each subscription. Then it will get rendered in the UI components.
Note: Ensure to unsubscribe from all the observables and dispose of the BLoCs upon unmounting UI components.
Step 04: Implementing UI components.
As you can see now, increase() and decrease() methods are called directly within the UI component. However, output data is handle by a stream builder.
It is better to have an intermediate layer to implement custom handlers to handle errors.
In the app.js file, the BLoC is initialized using the CounterBloc class. Thus, the Counter component is used by passing the BLoC as a prop.
That’s it. Now you can treat your business logic as a separate entity outside your UI components and change it as you need.
To use and improve this example app please refer to the project repository and do not forget to make a PR. ?
Final Thoughts
Based on my experience, the BLoC pattern could become an overhead for small-scale projects.
But, as the project grows, using BLoC patterns helps to build modular applications.
Also, you must have a basic understanding of rxjs and how observables work to implement the BLoC pattern for your React projects.
So, I invite you to try out BLoC pattern and share your thoughts in the comment section.
Thank you for Reading !!!
Build & share independent JS components with Bit
Bit is an extensible tool that lets you create truly modular applications with independently authored, versioned, and maintained components.
Use it to build modular apps & design systems, author and deliver micro frontends, or simply share components between applications.
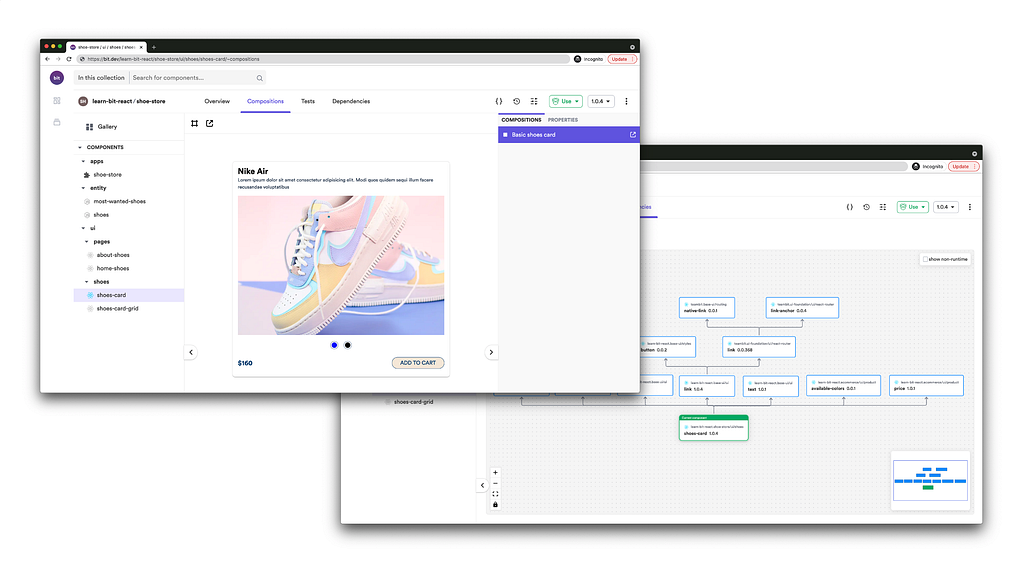
Bit: The platform for the modular web
Read More
- Independent Components: The Web’s New Building Blocks
- Redux-free state management with Jotai
- Middleware for the Async Flow in Redux
Using BLoC Pattern with React was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Charuka Herath
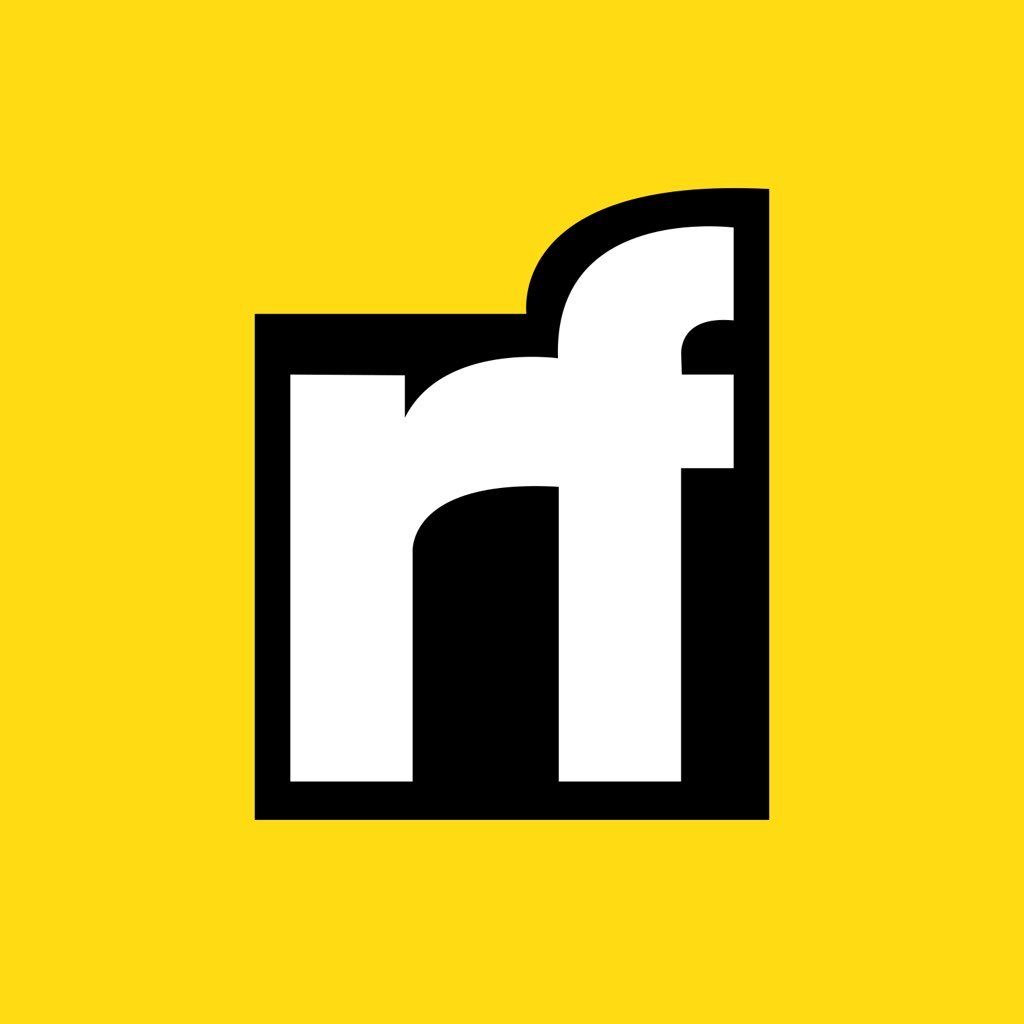
Charuka Herath | Sciencx (2021-07-27T16:41:09+00:00) Using BLoC Pattern with React. Retrieved from https://www.scien.cx/2021/07/27/using-bloc-pattern-with-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.