This content originally appeared on Bits and Pieces - Medium and was authored by Chameera Dulanga
Differences between the require and import statements in JavaScript
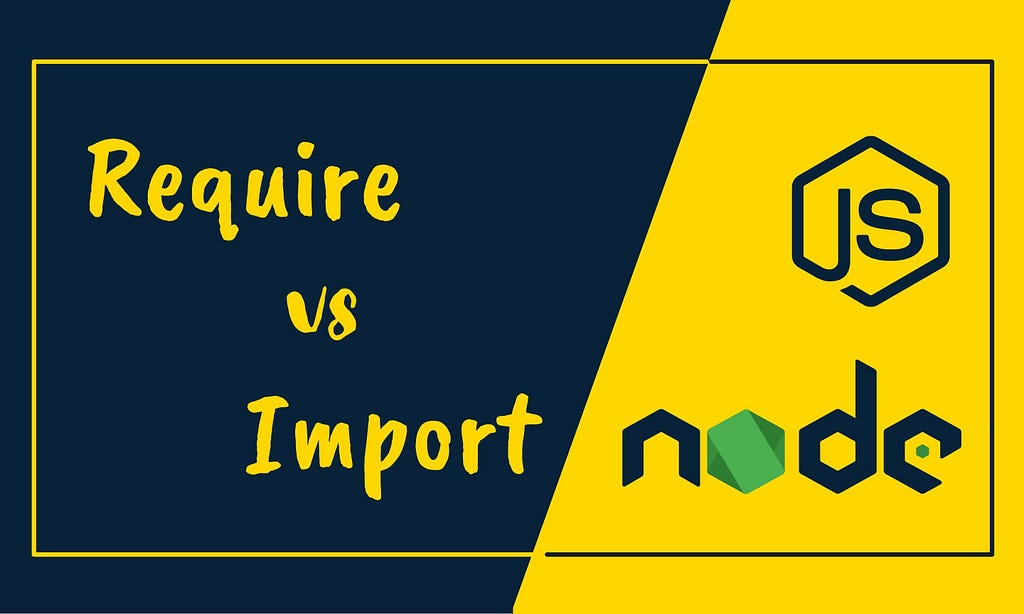
With modern web development, we use require or import to refer to JavaScript dependencies. And, sometimes, we use both in combination and use what works for the library.
But, do you know why both of these exists? What happens underneath? And what are the best practices when using one or the other?
In this article, I will discuss the usage of require and import and answer some of these common questions.
Background — JavaScript Module Types
Before discussing require and import, it is necessary to have an understanding of JavaScript modules. So, let’s see what the different types of JavaScript modules available are.
1. AMD — Asynchronous Module Definition
AMD modules were introduced to make modules more frontend friendly. They don’t require any bundlers, and all the dependencies are resolved dynamically.
AMD’s use require function is used to load external modules and is capable of acting as a wrapper for CommonJS modules.
define("exampleModule", [], () => {
return {
print: () => console.log("Hello World!");
}
}
define("main", ["require", "exampleModule"], (require) => {
const exampleModule= require("exampleModule");
exampleModule.print();
});
However, with the introduction of ES modules, usage of AMD has reduced drastically.
2. CommonJS Modules
CommonJS is the standard used by NodeJS to encapsulate JavaScript In modules. module.exports is used to export CommonJS modules, and import function is used to include modules into separate files.
Although CommonJS modules are widely used in NodeJS, they are not used in frontend development. The main reason behind that is the synchronous behavior of require function.
However, NodeJS only started to support ES modules from v13 onwards. Until then, most of the NodeJS modules, including NPM libraries, were developed using CommonJS modules.
Therefore, CommonJS modules are still widely used among developers.
And, CommonJS modules are equally important as ES modules, and I will discuss more in the upcoming sections of the article.
3. UMD — Universal Module Definition
UMD is a combination of AMD and CommonJS. It uses the syntax of CommonJS and asynchronous loading technique from AMD to make it suitable for both server-side and client-side.
UMD is used as a fallback module in bundlers like Webpack, and a simple UMD module example is shown below:
(function (root, factory) {
if (typeof define === "function" && define.amd) {
define(["jquery"], factory); // AMD
} else if (typeof exports === "object") {
module.exports = factory(require("jquery")); //CommonJS
} else {
root.returnExports = factory(root.jQuery);
}
})(this, function ($) {
function exampleFunction() {}
return exampleFunction;
});
4. ESM — ES Modules
ES Modules (ECMAScript Modules) is the official standard used in JavaScript. ES Modules use import and export statements to deal with modules. It resolves one of the biggest limitations of CommonJS, which is synchronous loading.
ES Modules import leads modules asynchronously while allowing static analysis on build time.
There were many debates among developers after introducing ES Modules, considering the compatibility with CommonJS. However, developers have adapted to use both, and we will discuss more details in the coming sections.
Since now you understand the background of JavaScript modules, let’s move onto discuss about require and import.
“Require” is built-in with NodeJS
require is typically used with NodeJS to read and execute CommonJS modules.
These modules can be either built-in modules like http or custom-written modules. With require, you can include them in your JavaScript files and use their functions and variables.
// built-in moduels
const http= require('http');
// local moduels
const getBlogName = require('./blogDetails.js')
However, if you are using require to get local modules, first you need to export them using module.exports.
For example, let’s assume that you have a file called blogDetails.js, and you need to use a function from that file in the index.js file.
You can also export multiple modules at once using modules.export as follows:
Note: Instead of using modules.export at the end, you can append export to start to each function as well. Eg: exports.getBlogContent = () => {};
I think now you understand when we should use require and how it works. But, before getting into the unique features of require, let’s see how import works. Then we will be able to compare and contrast them with a deeper level of understanding.
“Import” was introduced with ES6 modules
import is an ES module, and with export, they are known as ES6 import and export.
We can’t use import or export outside ES modules.
We can see that trying to import outside ES modules is a common developer mistake, and there are many StackOverflow threads about it.
If I take the same example, the only change I need to make is modifying modules.export to export default.
Then we can use import to include this file in our index.js file.
Note: Similar to require, you can also export each function individually by appending export to each function definition.
Eg: export const = getBlogContent = () => {};
So, I hope now you understand how and when you should use require and import. But, that’s not all; there are some significant differences in their functionality and usage. It’s time for a comparison.
Require Vs. Import
Both require and import are used to include modules. But they have several important features you should be aware of.
1. Require statements can be called anywhere in the code
Usually, we call the import or require statements at the beginning of a file.
But did you know that you have the freedom of using require statements anywhere in your code? Yes you can!
On the other hand, import statements can only be defined at the beginning of the file. Defining a import statement elsewhere will give you an error or automatically shift to the file’s beginning.
2. Require can be called conditionally
The statement require allows you to load modules conditionally or dynamically when the loaded module name isn’t predefined.
For example, you can call require inside a function or an if condition like below:
if(articleCount>0){
const getBlogTitle = require(‘./blogDetails.js’);
}
require statements have this flexibility because they are treated as functions. They are called at the run time, and there is no way to know anything before that. But, import statements are static, and we can’t use them conditionally or dynamically.
Note: You might see this as an advantage of require. But, since import statements are static, you can detect any error before your run the application.
3. Import statements are asynchronous
Being synchronous or asynchronous might not play a major role in small-scale applications. But, if we think of large-scale applications, there are hundreds of modules are used. So,
if you use require, modules will be loaded and processed one by one.
On the other hand, import statements solve this issue by working asynchronously, and they are known to perform well compared to require functions in large-scale applications.
4. ES modules are the future
As we discussed, the ES module system was introduced as a standard to maintain Client-side JavaScript modules. It’s also adopted by TypeScript with additions to define Type. Therefore, I don’t think that require can replace ES again since it has become a widely used standard among developers.
But since there are plenty of NodeJS modules and libraries written with CommonJS, we can’t keep require entirely aside. So we have to use both of them accordingly.
Also, we can configure compilers to convert output different modules types.
If you are using TypeScript, you can target different module systems as the output by making few changes to tsconfig.json file. For example, let’s assume that we need to output a code version that uses CommonJS.
All you need to do is, create a new tsconfig file for CommonJS output by extending the original tsconfig file and modifying the module parameter under CompilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"module": "CommonJS",
"outDir": "./lib/cjs"
},
}
Note: You can also use build tools like Webpack to convert ES Modules to CommonJS modules.
In addition to these significant differences, there are some minor changes in syntax between them. So, I have created an infographic comparison summarizing everything discussed in the article to clearly understand the differences between require and import.
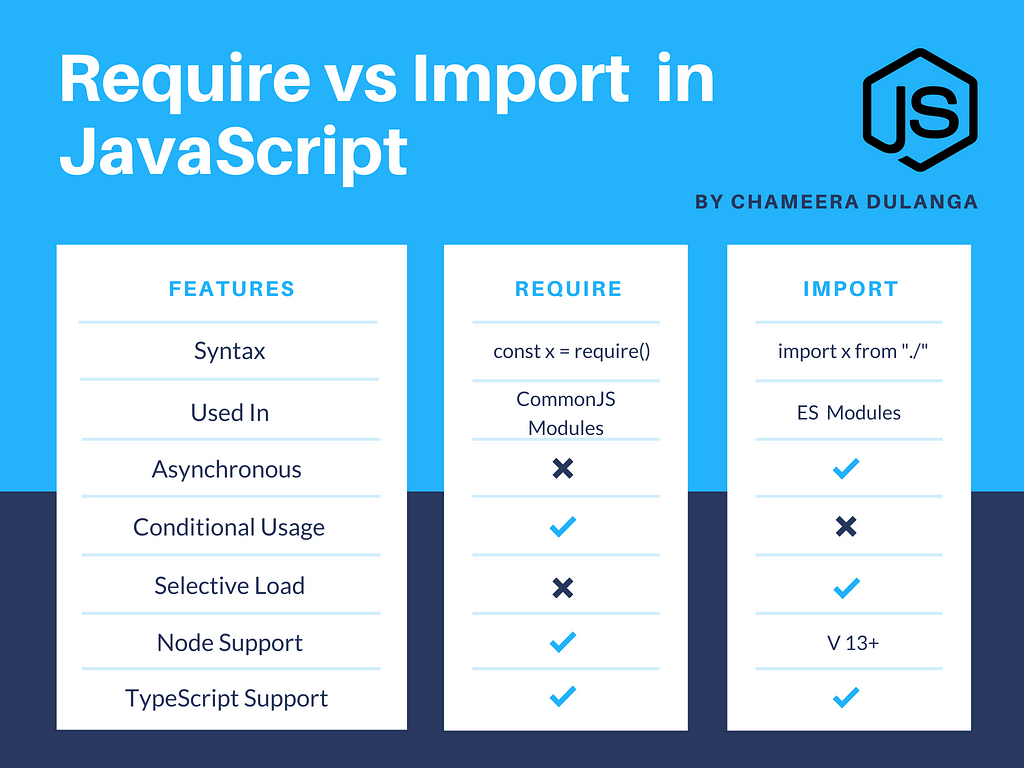
Build & share JS components with Bit
Bit is an extensible tool that lets you create truly modular applications with independently authored, versioned, and maintained components.
Use it to build modular apps & design systems, author and deliver micro frontends, or share components between applications.
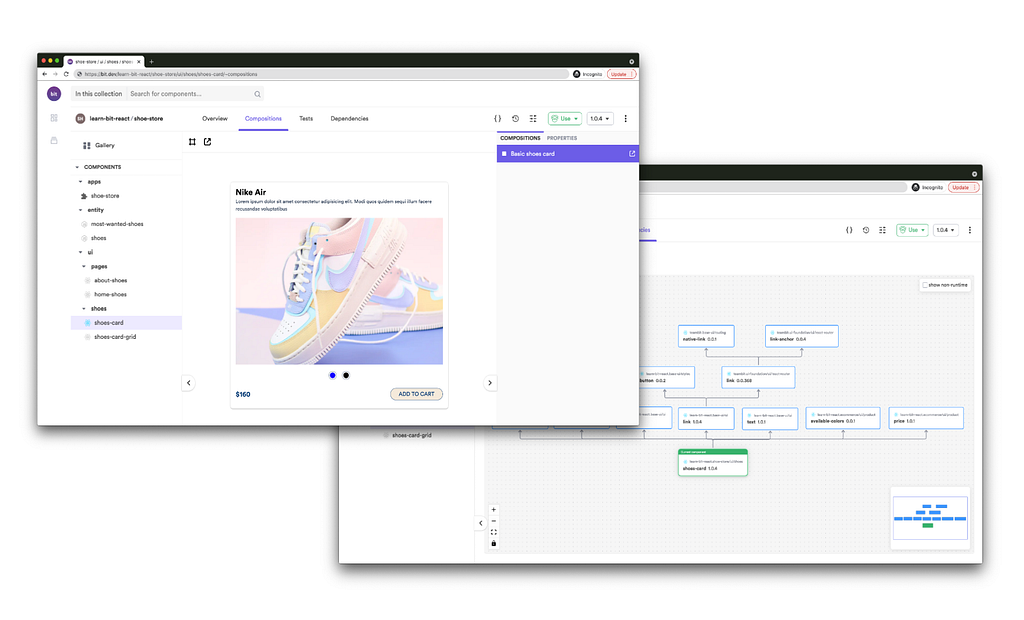
Bit: The platform for the modular web
Conclusion
In this article, I addressed a common question among many developers: the difference between require and import. Although ES import and export are the new standards used to include modules, require functions are widely used in NodeJS.
So, understanding the difference between them and their usage will help you minimize unwanted errors in your application.
Thank you for Reading !!!
Learn More
- Arrow Functions vs. Regular Functions in JavaScript
- “Double Quotes” vs ‘Single Quotes’ vs `Backticks` in JavaScript
JavaScript Require vs. Import was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Chameera Dulanga
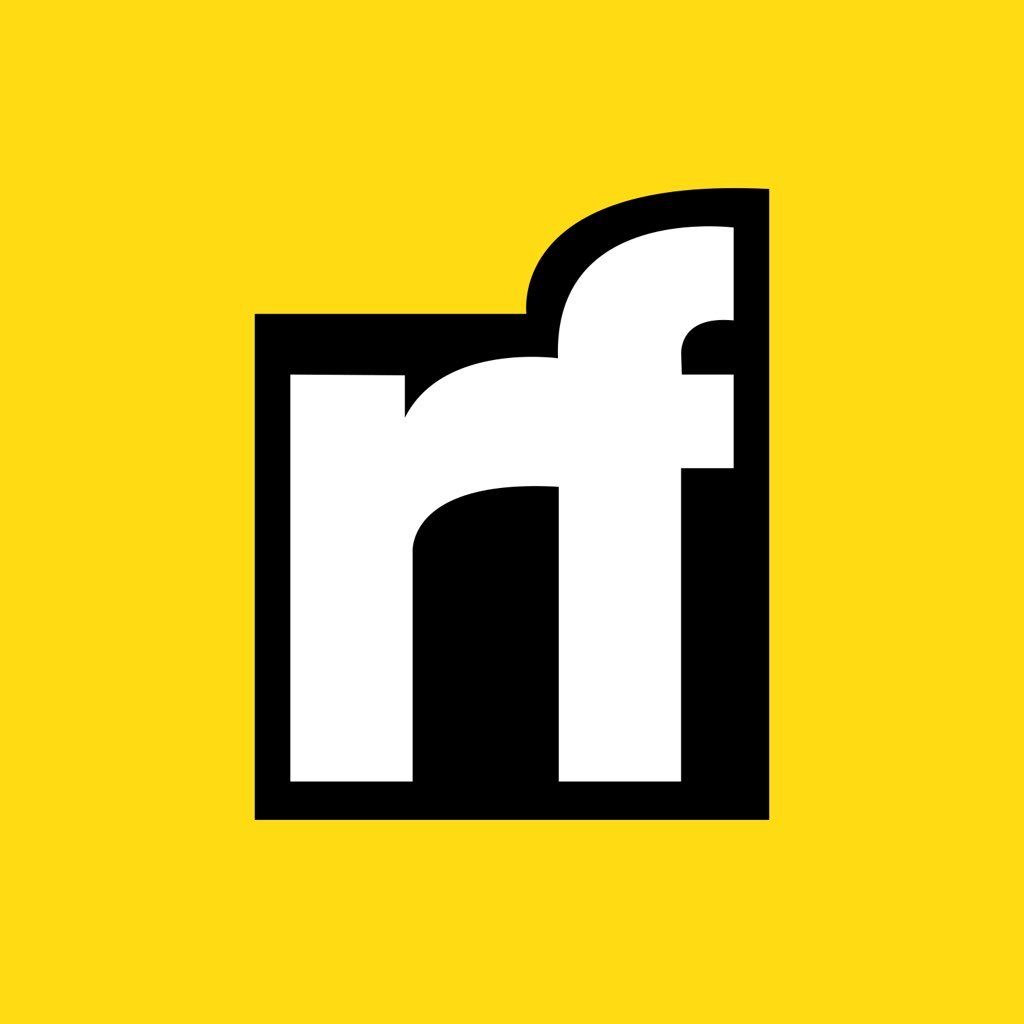
Chameera Dulanga | Sciencx (2021-08-12T17:51:45+00:00) JavaScript Require vs. Import. Retrieved from https://www.scien.cx/2021/08/12/javascript-require-vs-import/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.