This content originally appeared on DEV Community and was authored by RedEyeMedia⭕
In this tutorial you will learn how to do efficient GET-requests in React.
This will include:
- create-react-app for creating our boilerplate code
- Axios for the actual HTTP operation
- Async / Await to handle asynchronous promises
- React Hooks
- Ternary operator in the JSX
Tutorial on YouTube:
The code:
import { useEffect, useState } from "react";
import axios from "axios";
function App() {
const [fetchedData, setFetchedData] = useState([]);
useEffect(() => {
const getData = async () => {
const data = await axios.get(
"https://jsonplaceholder.typicode.com/todos/1"
);
setFetchedData(data);
};
getData();
}, []);
console.log("data: ", fetchedData);
return (
<div className="App">
<h1>test</h1>
{fetchedData.data ? <h2>title: {fetchedData.data.title}</h2> : null}
</div>
);
}
export default App;
Axios
Let's begin with the dependency, namely Axios. Axios is a HTTP client for nodejs which makes doing requests very intuitive. Here we are only doing GET-requests so we don't need to pass any additional parameters like a body in our request. That means it will simply be axios.get(URL). The result is stored in a local variable called data. We are fetching our data from JSON placeholder which is a useful fake API for when you're just doing testing.
Aync / Await
The function that wraps the GET-request is called getData and is marked as async, meaning it is asynchronous and expects a promise. Because of this marking, we need to specify the data or the promise we expect with a property of await. This makes sure that the function literally waits for the response to get here.
useEffect Hook
Furthermore, the getData function is defined inside a useEffect Hook. This is strictly not necessary, and it can be moved outside of it. The important part is that the function call happens inside it. We also have a empty dependency array (the empty brackets at the end of the useEffect Hook) that makes sure that whatever is inside only runs once on render.
useState Hook
Another thing related to Hooks that are worth mentioning is the useState Hook. Here we have initialized it to hold our fetchedData. We also have the set function for setting a new value to that variable, and we utilize that in the getData function. So when the function is called on render it fetches the data from JSON placeholder and sets the new state to be whatever came back. In this case data.
The JSX
Lastly we render our the title property of our single data object to the page in our JSX. Here we must use a ternary operator to check for potential null values. Meaning that the data might not be there when we expect it to and React will throw an error. So by using this expression we guard for that and React is happy.
Conclusion
GET-requests in React have a few key points, that can be handy to remember.
Hooks in the form of useState and useEffect, for covering the local state and rendering correctly. Axios is optional of course, but makes the job pretty easy to understand in my opinion. And finally the null check in the JSX prevents you from getting errors.
Now that you know how to do GET-requests the right way, it isn't difficult to change this into a POST request or whatever you might need.
If you feel like the code can be even more efficient, please post it in the comments!
Hope this was helpful to you.
Have a nice day.
Follow and support me:
I am especially grateful for subscriptions to my YouTube channel. And if you want to follow me on Twitter, or just give some feedback that's awesome too!
? YouTube
Want to read more?
Here are some other articles that I've written:
This content originally appeared on DEV Community and was authored by RedEyeMedia⭕
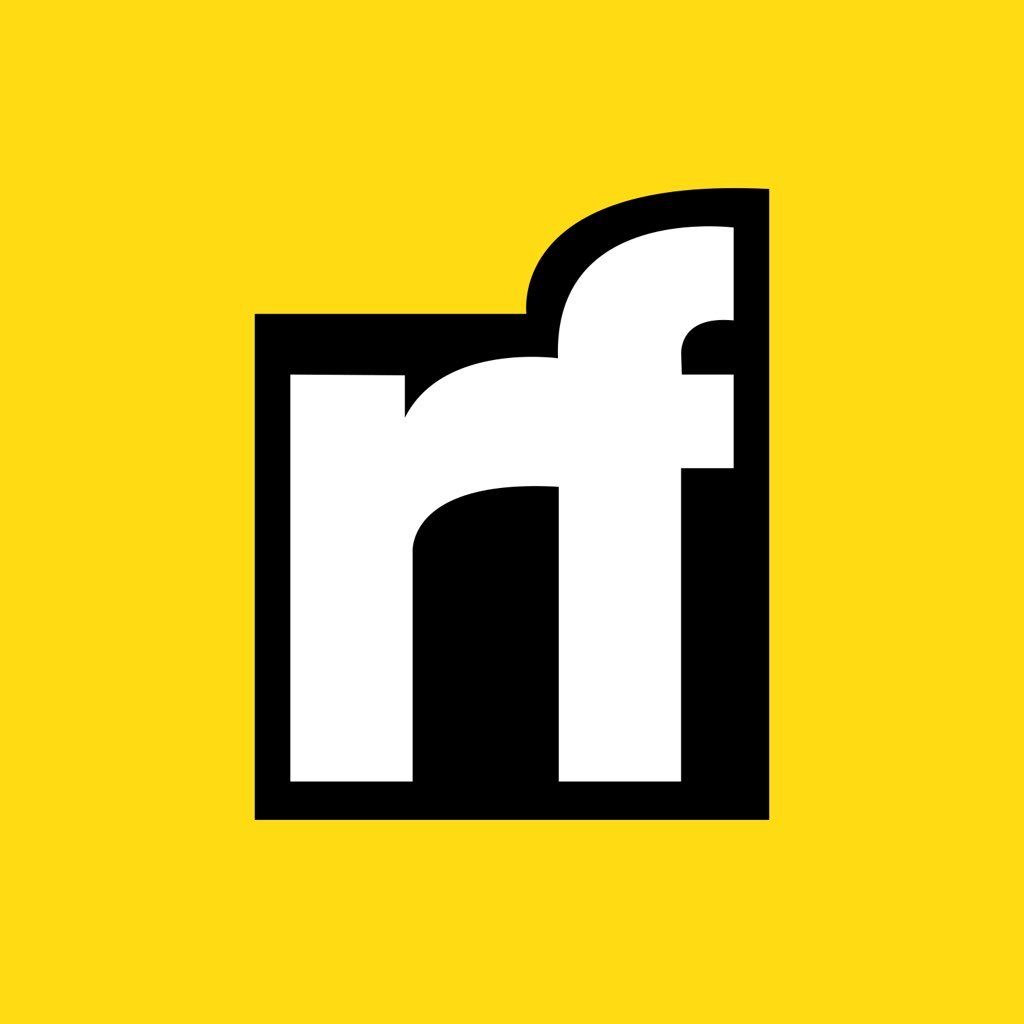
RedEyeMedia⭕ | Sciencx (2021-08-15T08:50:39+00:00) GET Requests in React Using Async/Await. Retrieved from https://www.scien.cx/2021/08/15/get-requests-in-react-using-async-await/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.