This content originally appeared on Level Up Coding - Medium and was authored by Arpan Patel
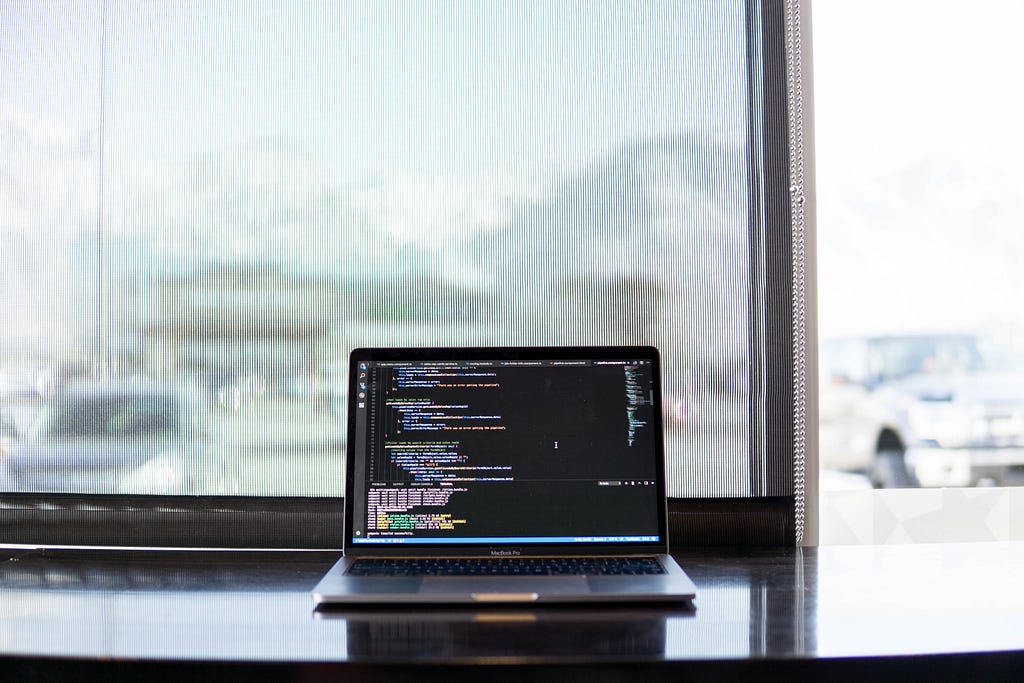
Today we are going to learn about custom form validation in angular.
Angular has some built-in validators for us, but it’s not sufficient for all our use cases. Sometimes we need to create our own custom validator.
Basic Of Custom Validators
Here is the basic structure of the custom validation function.
export function yourCustomFunctionName(): ValidatorFn {
return (control: AbstractControl | form: FormGroup): ValidationErrors | null => {
return condition ? { key: value } : null
}
}
Here you can see, yourCustomFunctionName is a function that returns the validator function.
The validator function takes an Angular AbstractControl object if the validation is used at the field level or FormGroup object when used at the form level.
We will see both types of validation later in this article.
It will return null If the control value is valid, Or a validation error object If there is an error.
Implementing Custom Validators
Now you get the basic idea. So without further ado let implement our custom form validation.
We are going to use the reactive form in this example.
<form [formGroup]="validationForm">
<div>
<label>Name</label>
<input formControlName="name">
</div>
<div>
<label>From</label>
<input type="number" formControlName="from">
</div>
<div>
<label>To</label>
<input type="number" formControlName="to">
</div>
<button type="submit" class="btn btn-primary">Submit</button></form>
Here we can see the simple form with three fields, one is for name input and the other two are for range input.
Here is the component class for this template.
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
validationForm = this.fb.group({
name: ['', [Validators.required, nameValidation()]],
from: ['', [Validators.required]],
to: ['', [Validators.required]]
}, {
validators: [rangeValidation()]
});
constructor(private fb: FormBuilder) {}
get name() { return this.validationForm.get('name'); }
}
You can see here, That we apply two custom validation. One at the field level (nameValidation) and another at the form level (rangeValidation).
You can also notice that we have one get method for our name control. That we use later in the component template to check our validation.
If you are confused about when to use field-level validation and when to use form level. Then it’s easy, if you want to validate input based on its own value then you can use field-level. But if your validation is based on another field of form then you can use form level validation.
Let implement these two validations one by one respectively.
Field level Custom Validators
For sake of this article, we are going to implement very simple validation.
The name field value always includes the word “angular” in it.
export function nameValidation(): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const isValid = /angular/gm.test(control.value);
return isValid ? null : { name: true };
};
}
Let’s understand what is going on here. We can see here that our nameValidator() function return ValidatorFn . And It’s not a validator function itself.
Validator function takes a control object and returns null If the control value is valid, or else returns an ValidationErrors object.
Form level Custom Validators
Now let’s implement our form-level custom validation function. For that also we are going to implement simple validation.
Check to value is always greater thanfrom value.
export function rangeValidation(): ValidatorFn {
return (form: FormGroup): ValidationErrors | null => {
const from = Number(form.get('from')?.value);
const to = Number(form.get('to')?.value);
return from >= to ? {range: true} : null;
};
}
You can see that the function structure is almost the same as the above function. But the major difference is here validation function take FormGroup object as a parameter.
Here we check if from value is greater than or equal to to value. It’s a return error object or else returns null.
How to display errors in templates?
Now we learned how to create our own custom validation. Let’s use this validation in our template.
For brevity, I’m going to check only our custom validation, Not going to check input touched state.
Check Field level Custom Validation
<div>
<label>Name</label>
<input class="form-control" formControlName="name">
<div *ngIf="name.errors?.name">Please enter valid name</div>
</div>
Check Form level Custom Validation
<div class="form-group">
<label>To</label>
<input type="number" class="form-control" formControlName="to">
<div *ngIf="validationForm.errors?.range">Please enter valid range</div>
</div>
Play with running app in stackblitz
Custom Form Validation in Angular - StackBlitz
Source Code
GitHub - arpanpatel/Custom-Form-Validation-In-Angular: Created with StackBlitz ⚡️
We did it.
Custom form validation in angular was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Arpan Patel
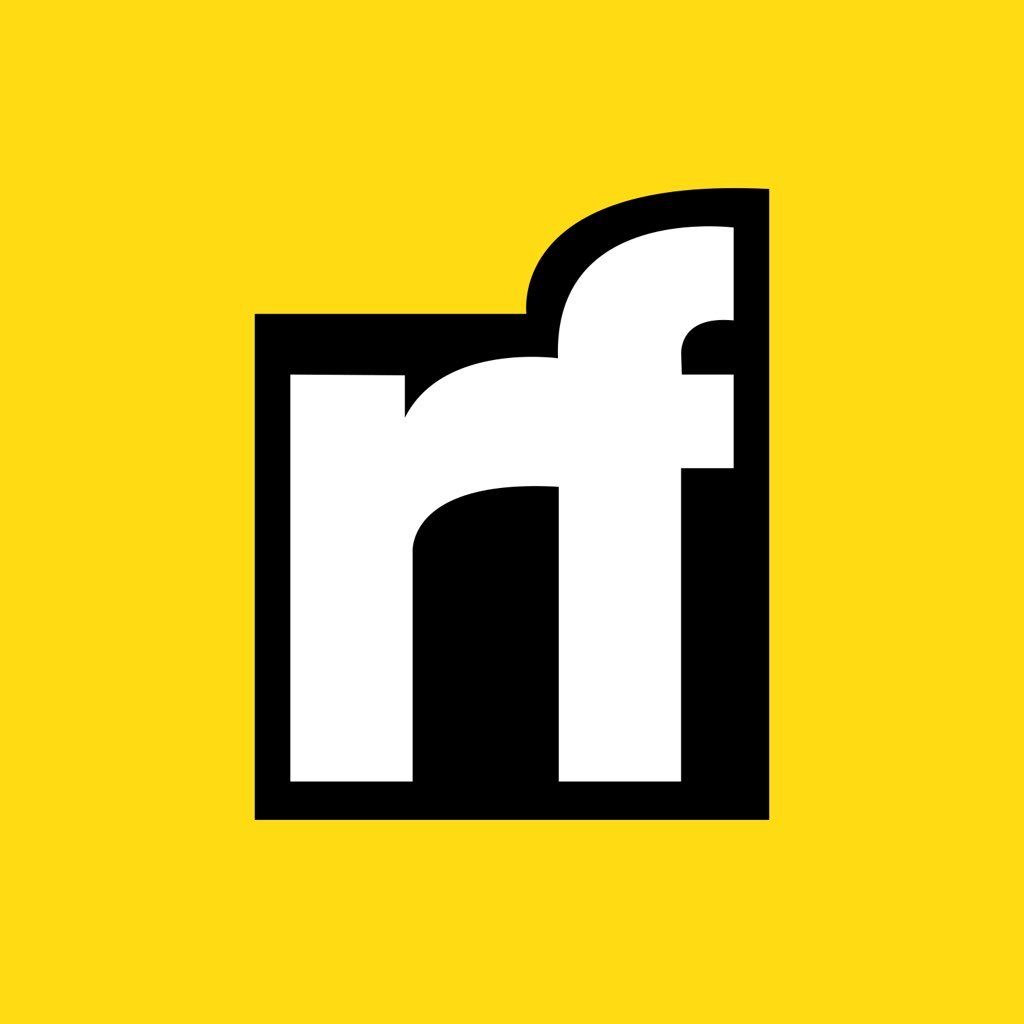
Arpan Patel | Sciencx (2021-08-31T17:27:34+00:00) Custom form validation in angular. Retrieved from https://www.scien.cx/2021/08/31/custom-form-validation-in-angular/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.