This content originally appeared on DEV Community and was authored by Taslim Arif
Any fool can write code that a computer can understand. Good programmers write code that humans can understand.
– Martin Fowler
Writing clean, understandable, and maintainable code is a skill that is crucial for every developer to master.
When I say clean code I mean:
- Proper variable name, function name, class name, Proper indentation, Proper spacing, Proper comment and Proper/Unique code style throughout the team.
- Minimal number of lines of code, classes, functions etc. Less code means less headache, less bugs and less maintenance cost.
- No duplication of code. Duplication in your software shows bad software, lack of knowledge and how bad developer you are.
- No magic numbers. Magic numbers are bad, they are not readable, they are hard to maintain and they are not reusable.
- Minimum/No hard coding. It is bad, not reusable, not testable and not maintainable.
- Code should pass 100% test cases(even 99% shows that you are screwed).
- Code is easy and cheap to maintain.
Tips to write clean code
- Varibale, function or class names should be descriptive. If it requires a comment to describe what the name does, it's bad naming convention.
let s; // number of seconds ---> Bad naming convention
Good naming convention:
let numberOfSeconds, customerName, employeeName, mailMessage, mailFolder
- Do not include any redundent information/ noise words in naming. Some of noise words:
- Data
- Object
- Info
- Information
- Varibale
- String
Bad naming convention:
userInfo, userData, userVaribale, userObject, accountInformation, employeeString etc.
Good naming convention:
user, employee, account etc.
- Be consistant while naming varibales of similar tasks. For example while naming any varibale which stores fetched data from say API call or Database use only one of these: get, retrieve, fetch. Don't use more than one as they do same task.
Say you decide to follow get convention:
getUser, getName, getFile, getAccount etc.
- Don't use magic words:
Bad convention:
if(age> 18) "eligible for voting"
Good Convention:
MINIMUM_AGE_FOR_VOTING=18;
if(age > MINIMUM_AGE_FOR_VOTING) "eligible for voting"
Never leave code inside comments because it makes other developers scary to remove them because they don't know whether it is for comment purpose or left to use for later.
-
Always for coding style defined by the programming language which you are using. Few conventions in Typescript:
- Varibale name should be in camel case.
- Function name should be in camel case.
- Class name should be in pascal case.
Always follow DRY (Don't Repeat Yourself) principle.
Function name should be a verb, class and varibale name should be a noun.
Conclusion
Skill of writing clean code comes from using it again and again in software development. It is least you should follow while writing a software code.
This content originally appeared on DEV Community and was authored by Taslim Arif
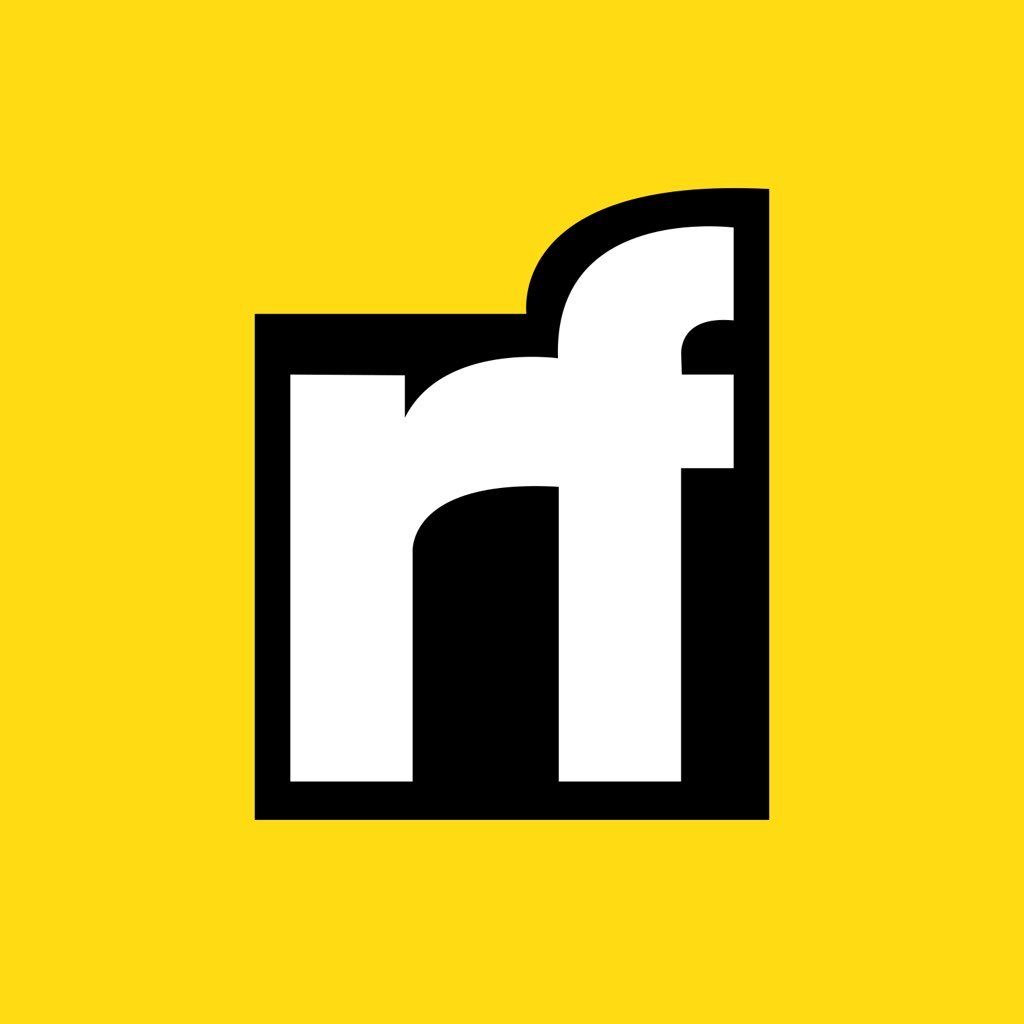
Taslim Arif | Sciencx (2021-09-26T08:24:15+00:00) Clean Code In few lines. Retrieved from https://www.scien.cx/2021/09/26/clean-code-in-few-lines/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.