This content originally appeared on DEV Community and was authored by DEV Community
When we watch a website that has cool animations, we tend to stay on the website for a long time.
It could be said that these animation features could help attract visitors to your website.
Today, I'll share with you guys how to implement fade-in animation on scroll with Vanilla Javascript.
Using IntersectionObserver to monitor if the element that you plan to animate is in the browser or not, you can make a fade-in animation that fires when the element enters the browser.
Sample & Goals
Here are two samples.
The first one is added fade-in animation on scroll for one container includes five items.
The second sample is applied fade-in animation for every five-item.
You can try to scroll and check animation again and again.
Blueprint
First, prepare an element that you want to apply fade-in animation with HTML. And add '.appear' class.
Elements with the '.appear' class will be the target of the fade-in animation.
Second, write a fade-in animation with CSS. When the '.inview' class is added to an element with a '.appear', set the opacity to 1 and transform: translateY(40px) to none.
Third, control the '.inview' class using IntersectionObserver of Javascript.
Monitor a single element and control classes with IntersectionObserver
Let's try to make a fade-in animation on scroll!
1.Prepare the element that has the 'appear' class according to the above blueprint.
<div class="container appear">
<div class="item">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
</div>
This time, I applied the 'appear' class at container div.
2.Prepare CSS animation.
.appear {
transition: all 0.8s;
opacity: 0;
transform: translateY(40px);
}
.appear.inview {
opacity: 1;
transform: none;
transition-delay: 0.3s;
}
If an element has 'appear' class, it's applied 'transition','opacity' and 'transform'.
In this case, I wrote the CSS so that once the 'inview' class is added, the translateY value will disappear. By doing so, the element that has 'appear' class will move upward from 40px down from its normal position.
And then the element can appear to the browser changing 'opacity'.
3.Monitor an element and control classes with IntersectionObserver
You can use the IntersectionObserver to determine if the monitored element is in the browser or not and add or remove the 'inview' class.
const appear = document.querySelector('.appear');
const cb = function(entries){
entries.forEach(entry => {
if(entry.isIntersecting){
entry.target.classList.add('inview');
}else{
entry.target.classList.remove('inview');
}
});
}
const io = new IntersectionObserver(cb);
io.observe(appear);
I will explain how I am using 'IntersectionObserver'.
1.Get the element to be monitored.
const appear = document.querySelector('.appear');
In this case, it's the 'container' class div.
2.Write a callback function.
const cb = function(entries){
entries.forEach(entry => {
if(entry.isIntersecting){
entry.target.classList.add('inview');
}else{
entry.target.classList.remove('inview');
}
});
}
IntersectionObserver pass a callback function as an parameter.
In this code, a callback function is named 'cb'.
To find out which elements have been intersected using 'forEach'.
The 'entry.isIntersecting' can be used for the condition that the element is inside the screen.
And the if-else statement can be used to write code to add or remove classes.
If the element is intersected, add 'inview'class to 'appear' class.
3.Call the constructor to create an Intersection observer and pass a callback function.
const io = new IntersectionObserver(cb);
4.Specifies the target element to be monitored.
io.observe(appear);
It's monitoring the element with 'appaer' class.
This content originally appeared on DEV Community and was authored by DEV Community
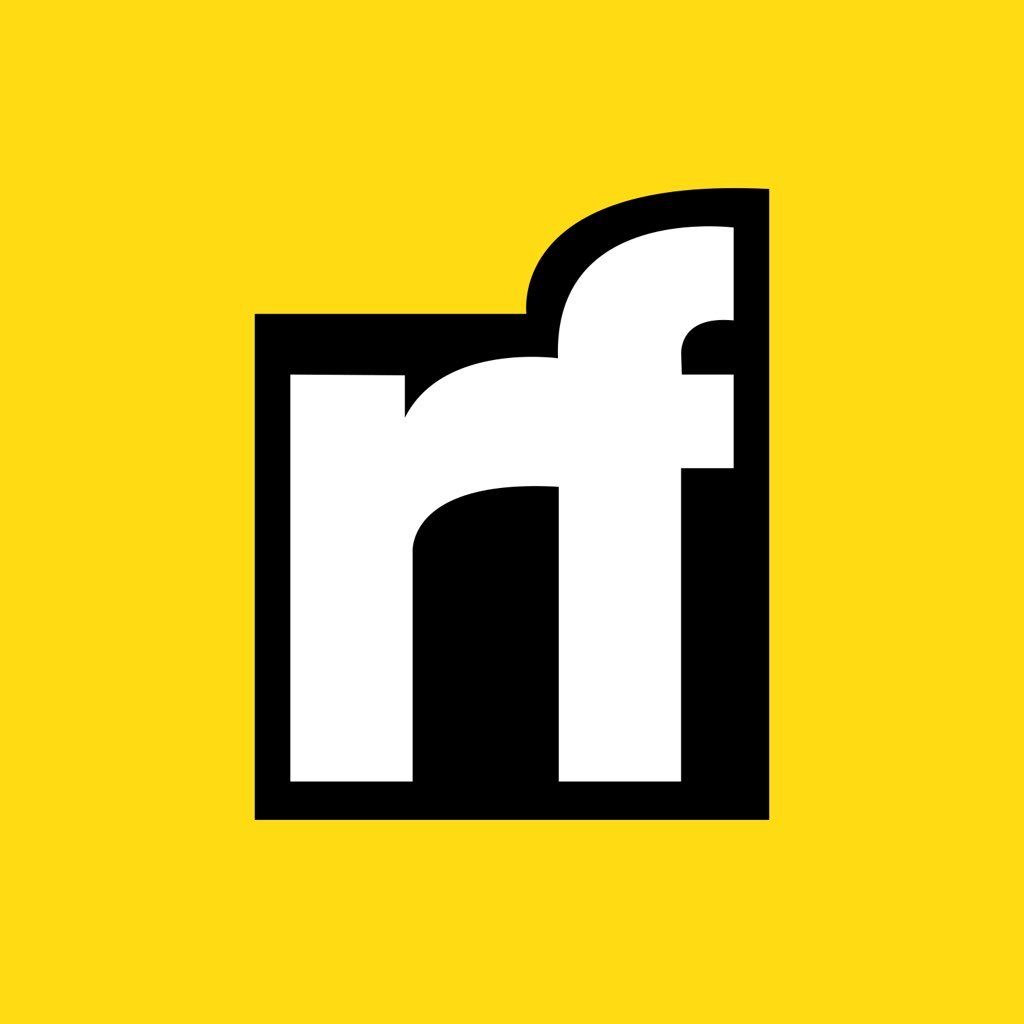
DEV Community | Sciencx (2021-10-01T02:36:52+00:00) Fade-in animation on scroll with IntersectionObserver(Vanilla JS). Retrieved from https://www.scien.cx/2021/10/01/fade-in-animation-on-scroll-with-intersectionobservervanilla-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.