This content originally appeared on DEV Community and was authored by Abel Ayalew
How many of us use archiver extractor tools to extract zip files. On top of that some tools are not user-friendly, so we have hard time utilizing them how we want to. However, with python extracting files has always been easier. Let's look at some common examples.
For the task we will use python's built-in module zipfile
.
From the various functionalities that this module provides, we are going to see how to:-
- Extract all files in a zip file.
- Extract a single file.
- Extract specific files using certain conditions.
- Extracting password protected files.
Let's talk about them in a bit more detail. We are going to use a common snippet for all of them and change only the required lines.
from zipfile import ZipFile
path_of_zip_file = input("Enter path of the zip file > ")
path_to_extract_to = input("Enter the path you want to extract to > ")
with ZipFile(path_of_zip_file , 'r') as files:
# here is where the codes are gonna go
1. Extract all files in a zip file.
For this we will just use the extractall()
method.
files.extractall(directory_to_extract_to)
2. Extract a single file.
To extract a single file we are going to use read()
method of ZipFile
objects. The read()
method returns the bytes of the file name in the archive, so we are gonna take that and create the file with it.
ZipFile.read(name, pwd=None)
method takes two parameters Name and Password. If you are working with encrypted file, don't forget to add your password.
Now, we are going create a variable for our file name below the paths we declared on the common code snippet, but you can pass the file name directly too.
We are going to import os
module, to write our file in the path we chose.
file_name= os.path.join(directory_to_extract_to, file_to_extract)
Now we can create our file.
with open(file_name, 'wb') as file:
file.write(files.read(file_name))
3. Extract specific files using certain conditions.
Sometimes we may not want to extract everything in a zip file. For example we may want to extract .py files only. We can so this very easily using conditions. Our condition doesn't have to be file extension, but to easily demonstrate we will be using that here.
for file in files.namelist():
if file.endswith(".py"):
files.extract(file)
4. Extracting password protected files.
This is pretty straightforward like we mentioned earlier. Just create a variable for your password and pass it in or you can just pass it in as it is too.
files.extractall(directory_to_extract_to, pwd=b'password')
❗NOTE:
Decryption is extremely slow as it is implemented in native Python rather than C.
I wanted to show how we can give our console app a user-friendly GUI using the Gooey module
, but I didn't want the post to be longer than it is. However, you can check the implementation in my Github. It is a very basic implementation, so read the documentation of gooey and you can add many features to it.
Don't forget to leave a star if you like it😉.
If you like what you read, follow for more.
This content originally appeared on DEV Community and was authored by Abel Ayalew
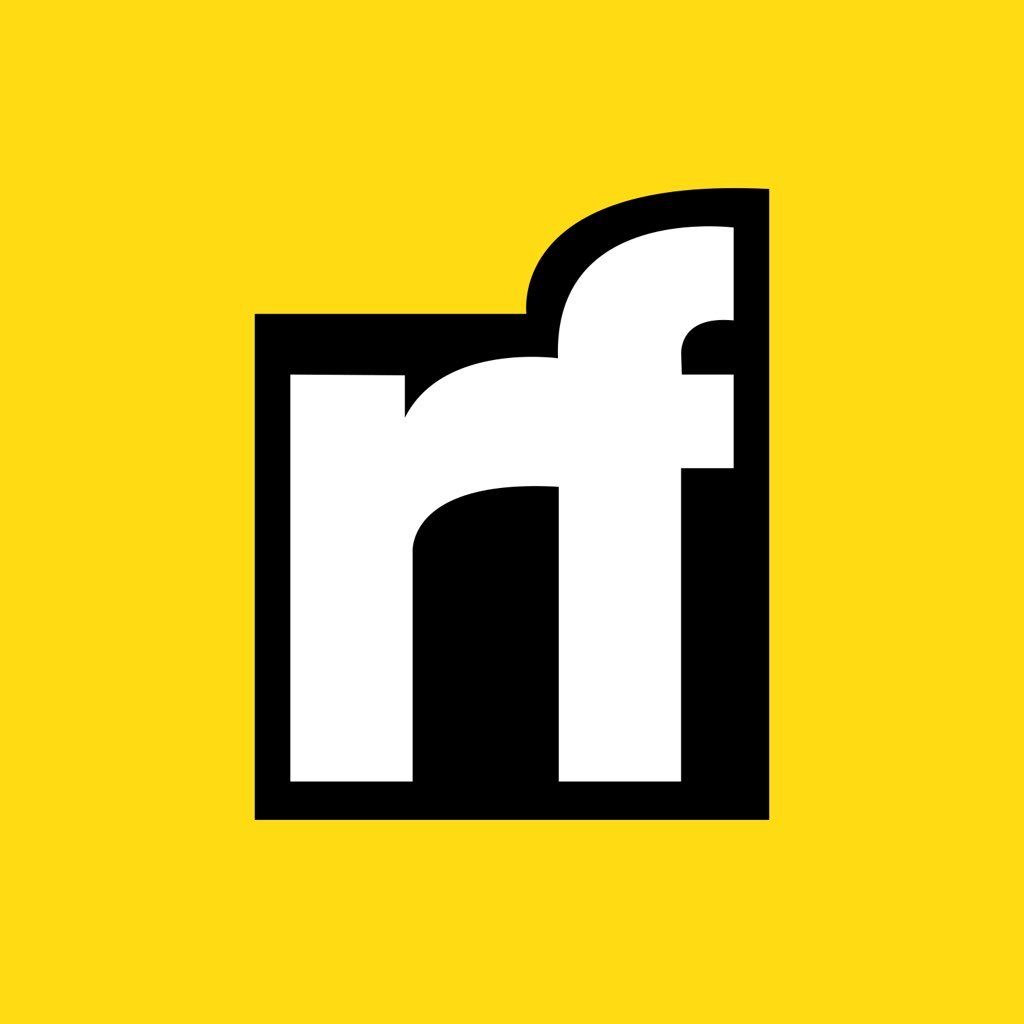
Abel Ayalew | Sciencx (2021-10-03T11:12:40+00:00) Archive Manager in Python with simple GUI. Retrieved from https://www.scien.cx/2021/10/03/archive-manager-in-python-with-simple-gui/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.