This content originally appeared on Level Up Coding - Medium and was authored by Jared Amlin
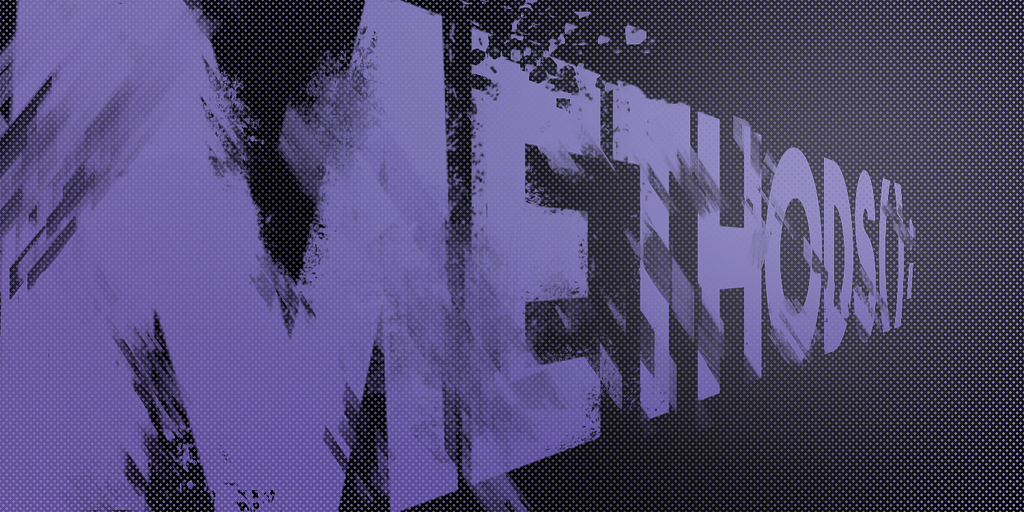
What are methods? Methods and Functions can be used interchangeably, only Methods are specific to C#. Methods are like a subset of instructions that can be injected into a script for added cleanliness and functionality. Methods, like scripts, run top to bottom. When you call a method from a script, that method will run in it’s entirety before continuing on from where it was called.
Methods can also take Parameters. When calling a method with required parameters, the call must include a value with the matching data type to be passed through. A method can ask for as many parameters as needed to complete the task at hand.
As a challenge, I have been tasked with passing an object and a color through some method parameters to be triggered by the space key.
A generic 3D cube is created and assigned via the inspector to the cubePrimitive3D variable in the ChangeColor class component attached to the Main Camera. A new color variable is declared and assigned to blue.
In void update, the space key is checked. When pressed, the ChangeObjectColor method is called, and the two required parameters are met using the cubePrimitive3D and newColor variables.
The ChangeObjectColor method takes two parameters and then gets to work. The object in question is null checked for life. If the object is active, the MeshRenderer is grabbed using GetComponent, and then the color is assigned the newColor value (blue).
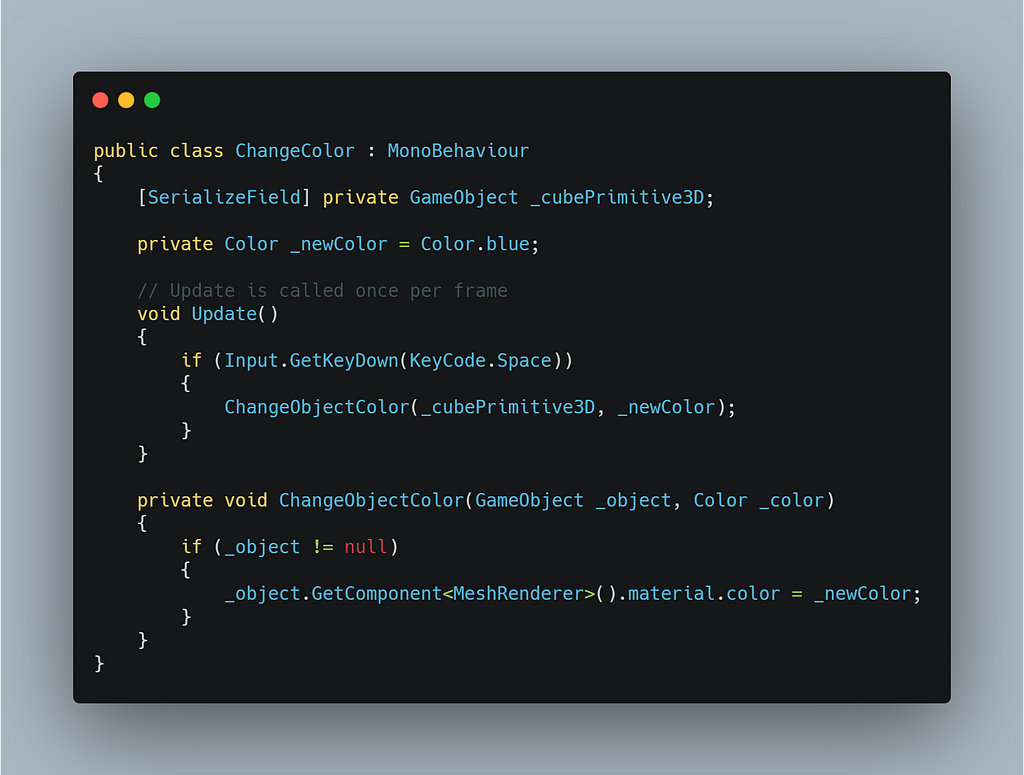
Here is a clip of the cube turning blue when the space key is pressed.
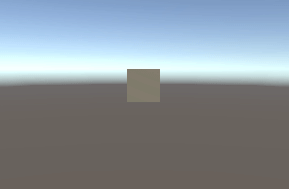
For another challenge, the player must be damaged by a random amount, the player health must not go below zero, and the method can’t be called again after the player health reaches zero. I start with two int values. The first holds a maxHealth value of 100 and the second an unassigned value for currentHealth. A variable of data type bool is introduced to check if the player isDead or not.
In void Start, I assign the currentHealth to be the maxHealth value.
In void Update, the space key is checked and the isDead bool being false is added as a condition to pass through. A local int variable is introduced called damageAmount, and assigned a random range between 10 and 20. The PlayerDamage method is then called, which requires a mandatory int value to be passed through. The damageAmount which is random between 10 and 20 is what gets passed through every time the player presses the space key.
The DamagePlayer method takes the damageAmount and immediately subtracts that value from the currentHealth amount. A local int variable called healthClamp is declared and assigned the value of Mathf.Clamp, which is used to keep the currentHealth value between a specified minimum and maximum value.
After assigning the currentHealth value to be the new clamped value, I check to see if the currentHealth is less than 1. If the player’s health is less than 1, the console receives a message that the player has died, and the isDead bool flips to true to keep the space key from firing the methods again.
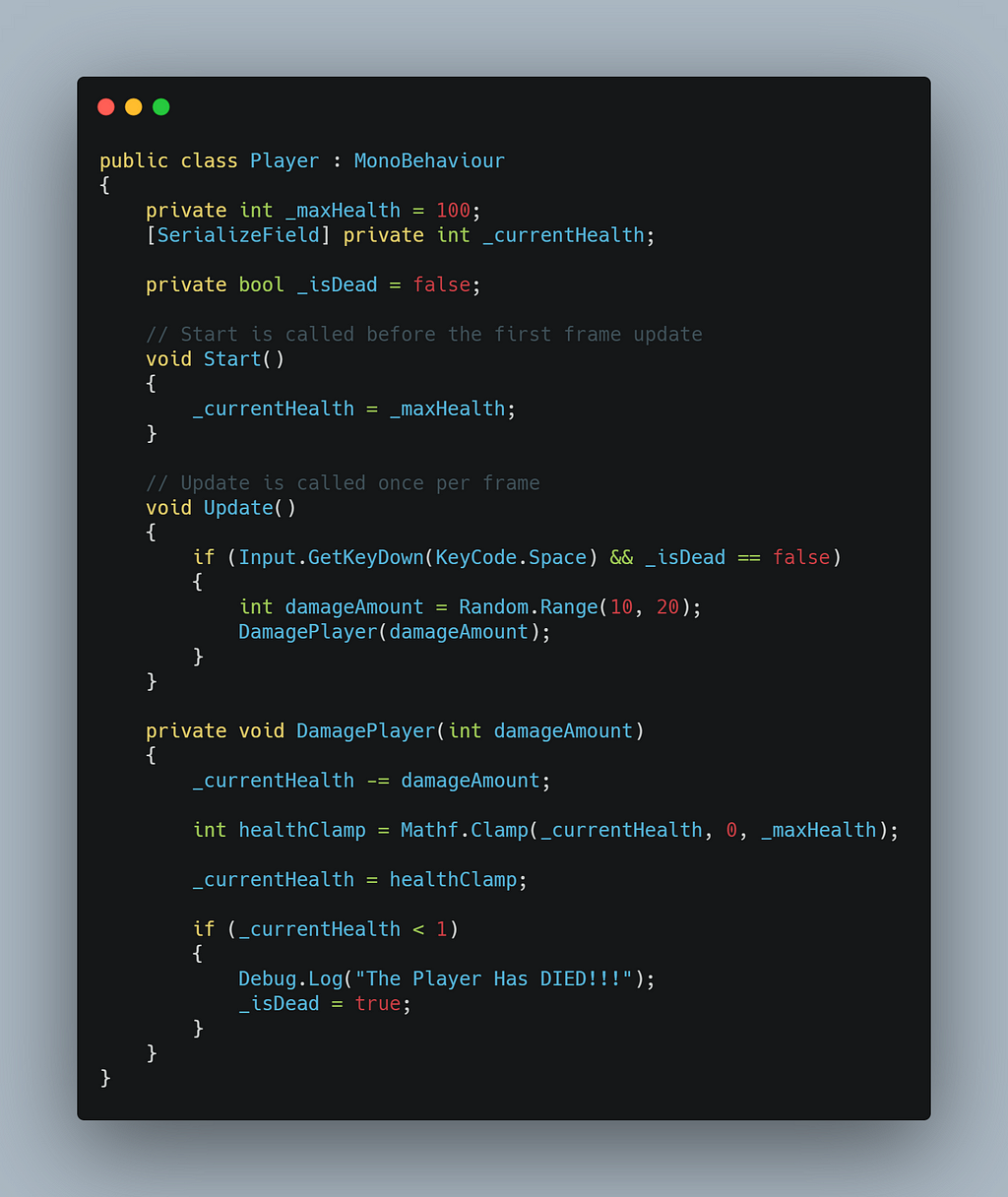
Here you can see the currentHealth value decreasing in the inspector.
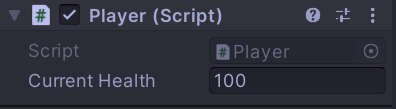
The console tells me that the player has died!
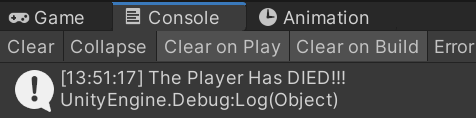
For an additional challenge, I have been tasked with storing an array of 5 positions. I must then use one method to randomize the return positions, and another to assign the position to the game object. Rather than do this one time in void Start, I decided to add to my space key check in void update.
I start with an int variable called randomPosition, which will simply store a random index value for the array to return. A Vector3 array called positions is declared, and 5 different positions are assigned.
The randomPosition variable is assigned the value of the RandomPositionIndex method, which returns a public int value between zero and however many positions are in the array. Now that the randomPosition variable has been run through the Index method to get a proper stored value, it can be used to assign the final position value via the NewRandomPosition method. This method which takes an int value from the position index, and that number will reference which position is assigned to the transform of the object!
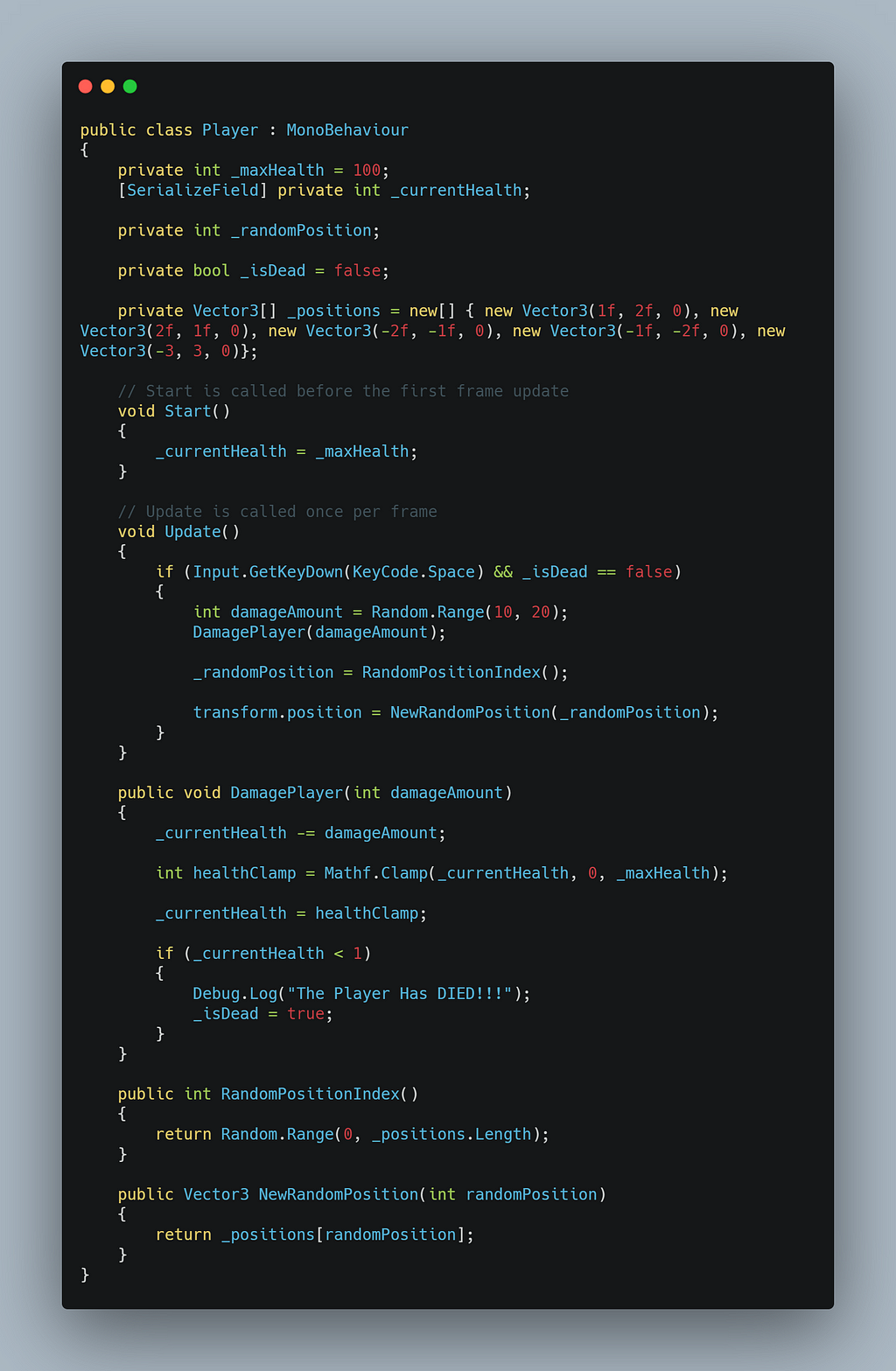
Here is a clip of the cube changing positions when the space key is pressed.
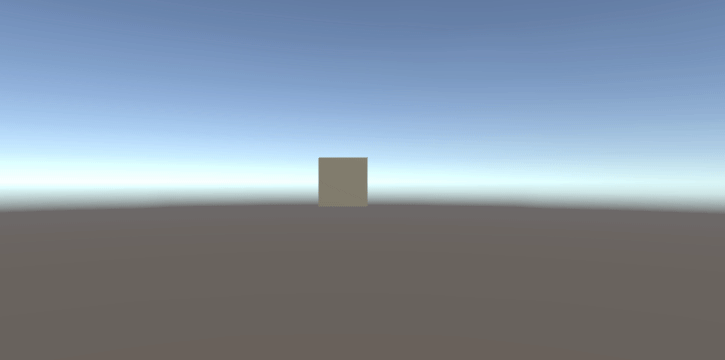
Methods and method parameters really open powerful doors when it comes to writing clear and functional code. Thanks for reading along!
Methods was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Jared Amlin
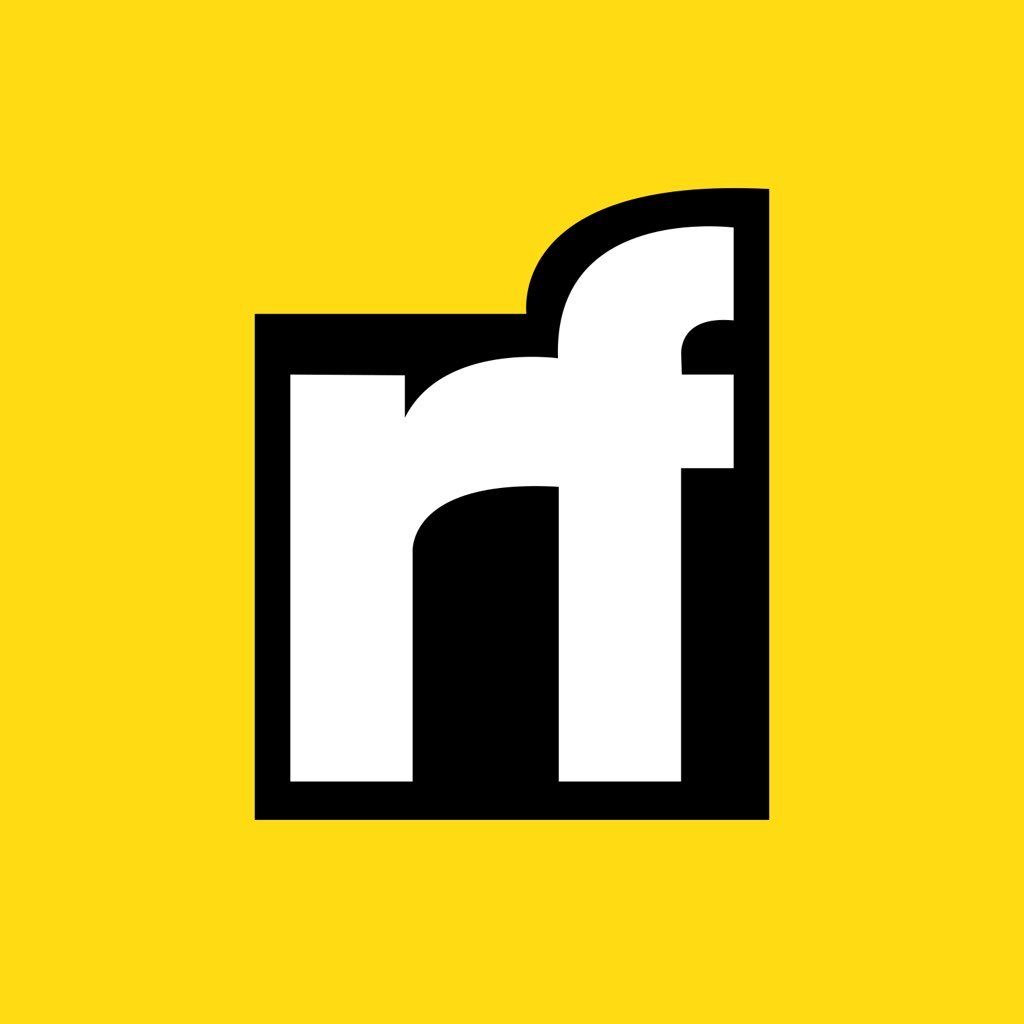
Jared Amlin | Sciencx (2021-11-07T20:02:08+00:00) Methods. Retrieved from https://www.scien.cx/2021/11/07/methods-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.