This content originally appeared on DEV Community and was authored by Md Farid Hossain
What is Custom Hook?
Custom Hook is a JavaScript function which we create by ourselves, when we want to share logic between other JavaScript functions. It allows you to reuse some piece of code in several parts of your app.
When and How to Use
When we want to share the logic between other components, we can extract it to a separate function. According to official documents, the custom hook has to:
start with the key word use
call other hooks
Because custom hook is a JS function, the Rules of Hooks apply to it as well. Those are:
Never call Hooks from inside a loop, condition or nested function
Hooks should sit at the top-level of your component
Only call Hooks from React functional components
Never call a Hook from a regular function
Hooks can call other Hooks
How to Create Custom Hook?
You create the hook same way as you create any JS function. Look at it as a refactoring of code into another function to make it reusable. It will speed up your productivity and save your time.
Let's consider the following example, where we have useEffect()hook which updates the document title:
import {useState, useEffect } from 'react';
export const Counter=()=> {
const [count, setCount] = useState(0);
const incrementCount = () => setCount(count + 1);
useEffect(() => {
document.title = `You clicked ${count} times`
});
return (
<div>
<p>You clicked {count} times</p>
<button onClick={incrementCount}>ClickMe</button>
</div>
)
}
What we want to do is to create a custom hook, which accepts a piece of text and updates the document title for us. Here is how we do that:
const useDocumentTitle = (title) => {
useEffect(() => {
document.title = title;
}, [title])
}
Our useDocumentHook() now accepts the string and calls useEffect() hook inside, which updates the document title with a given title, when the title was changed (we pass title as dependency here).
So our final code would look like this:
import { useState, useEffect } from "react";
const useDocumentTitle = (title) => {
useEffect(() => {
document.title = title;
}, [title]);
};
export const Counter = () => {
const [count, setCount] = useState(0);
const incrementCount = () => setCount(count + 1);
useDocumentTitle(`You clicked ${count} times`);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={incrementCount}>Click me</button>
</div>
);
};
And that is all, as simple as that :) In my next blog post I'll share 5 the most commonly used custom hooks, which make your development faster and easier.
This content originally appeared on DEV Community and was authored by Md Farid Hossain
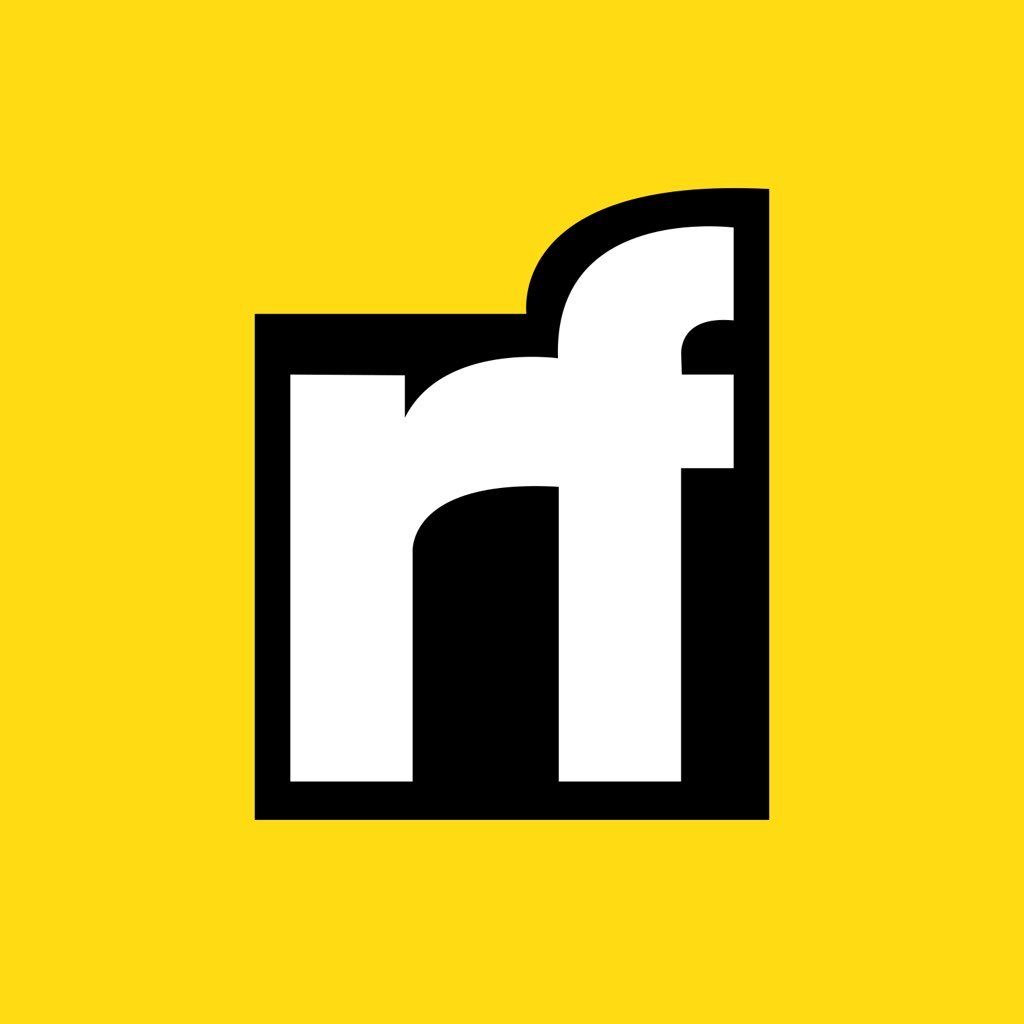
Md Farid Hossain | Sciencx (2021-12-23T17:02:34+00:00) What is Custom Hook?. Retrieved from https://www.scien.cx/2021/12/23/what-is-custom-hook/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.