This content originally appeared on DEV Community and was authored by Kamran Ahmad
Numeric Separator
Large Numeric Literals Are Difficult For The Human Eye To Parse Quickly.
The Numeric Separators Allow You To Add Underscores Between Digital In Literal Numeric, Which Makes Them More Readable.
let value1 = 250000000;
let value2 = 250_000_000; //same as value1 i.e 250000000
The Underscores will be stripped out automatically when the files get parsed.
Nullish Coalescing
The Nullish Coalescing Operator(??) allow us to check nullish values instead of falsy values.
let count1 = 0;
let result1 = count1 ?? 1;
console.log(result1); //output - 0
let count2 = null;
let result2 = count2 ?? 1;
console.log(result2); // output - 1
Nullish Coalescing Operator (??) is better choice than the logical OR operator (||) if you want to assign a default value to a variable.
BigInt
The maximum number you can store as an integer in JavaScript is 2^53-1
And BigInt allow you to go even beyond that, such that it provides a way to represent whole number larger than 2^53-1
A BigInt is created by appending n to end of an Integer literal of by calling the function BigInt that creates BigInt form string, number et.
const Bigint = 123456789012345678901234567890123456789n;
const sameBigint = Bigint("123456789012345678901234567890n");
const BigintfronNumber = Bigint(10); //same as 10n
Optional Chaining
The Optional Chaining Operator allow you to access properties of potentially undefined/null values without throwing an exception.
it allow you to access nested object property without worrying if the property exist or not.
const user = {
dog: {
name: "chee"
}
};
console.log(user.monkey?.name); //undefined
console.log(user.dog? .name); // chee
Promise.any()
Promise.any() takes an iterable of promise objects.
it returns a single promise that resolves as soon as any of the promises in the iterable fulfill. with the value of the fulfilled promise.
const promise1 = /*some*/;
const promise2 = /*some*/;
const promise3 = /*some*/;
const promises = [promise1, promise2, promise3];
promise.any(promises).then((value)=>console.log(value));
This content originally appeared on DEV Community and was authored by Kamran Ahmad
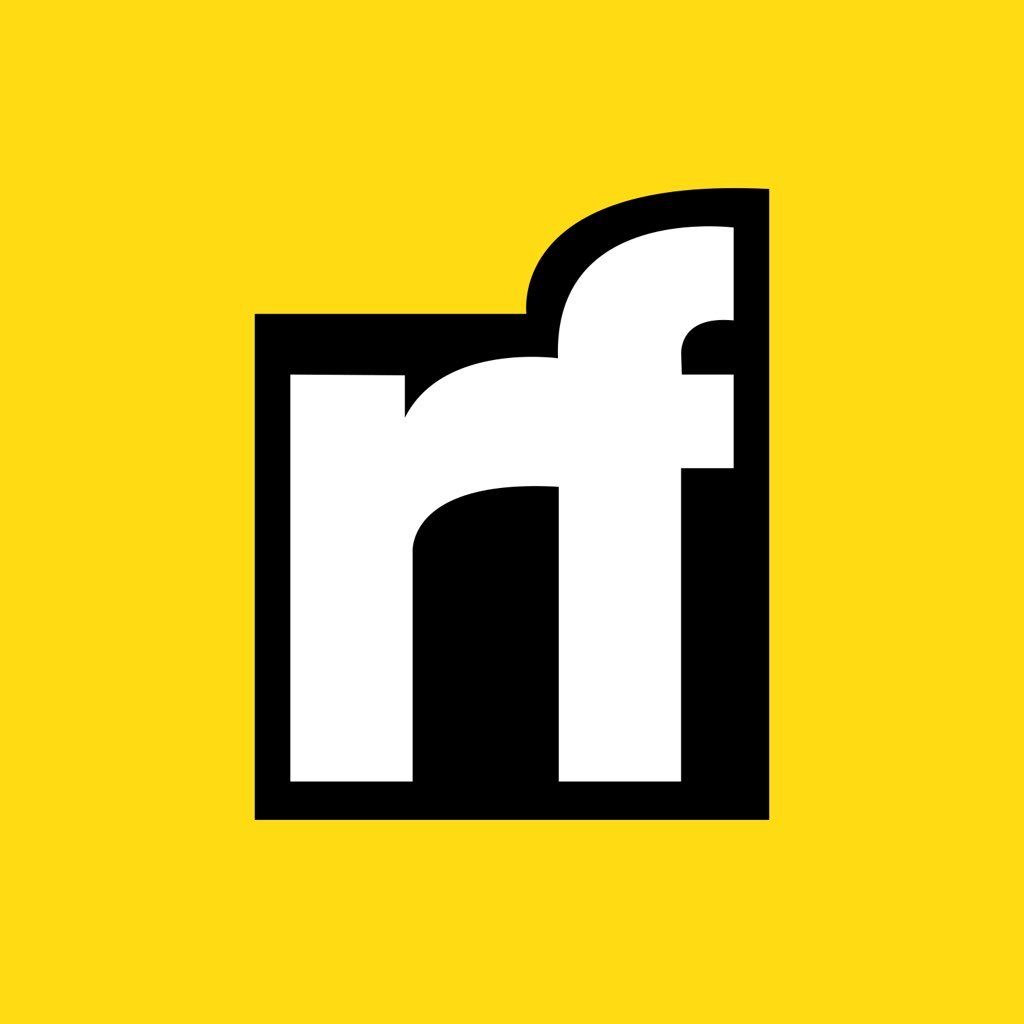
Kamran Ahmad | Sciencx (2021-12-24T18:15:59+00:00) Latest Javascript Feature you should know. Retrieved from https://www.scien.cx/2021/12/24/latest-javascript-feature-you-should-know/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.