This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This post is part of a new series where we build a clone of Airbnb with Next.js. See the first post here.
- Part 1: Let’s start by installing Next.js
- Part 2: Build the list of houses
- Part 3: Build the house detail view
- Part 4: CSS and navigation bar
- Part 5: Start with the date picker
- Part 6: Add the sidebar
- Part 7: Add react-day-picker
- Part 8: Add the calendar to the page
- Part 9: Configure the DayPickerInput component
- Part 10: Sync the start and end dates
- Part 11: Show the price for the chosen dates
- Part 12: Login and signup forms
- Part 13: Activate the modal
- Part 14: Send registration data to the server
- Part 15: Add postgres
- Part 16: Implement model and DB connection
- Part 17: Create a session token
- Part 18: Implement login
- Part 19: Determine if we are logged in
- Part 20: Change state after we login
- Part 21: Log in after registration
- Part 22: Create the models and move data to the db
- Part 23: Use the database instead of the file
- Part 24: Handle bookings
- Part 25: Handle booked dates
- Part 26: Prevent booking if already booked
- Part 27: Adding Stripe for payments
- Part 28: Airbnb clone, handling Stripe webhooks
After the booking and the payment, the person that purchases the holiday at a house is now redirected to the /bookings
URL, where we now have a placeholder page.
That’s managed by the pages/bookings.js
file.
Right now we added some placeholer code there:
pages/bookings.js
import Layout from '../components/Layout'
export default function Bookings() {
return <Layout content={<p>TODO</p>} />
}
We’re going to list all the bookings made by the user.
We replicate a bit what we did in pages/index.js
to list houses, except now we list bookings:
pages/bookings.js
import axios from 'axios'
import Head from 'next/head'
import { Booking, House } from '../model.js'
import Layout from '../components/Layout'
export default function Bookings(props) {
return (
<Layout
content={
<div>
<Head>
<title>Your bookings</title>
</Head>
<h2>Your bookings</h2>
<div className="bookings">
{props.bookings.map((booking, index) => {
const house = props.houses.filter(
(house) => house.id === booking.houseId
)[0]
return (
<div className="booking" key={index}>
<img src={house.picture} alt="House picture" />
<div>
<h2>
{house.title} in {house.town}
</h2>
<p>
Booked from {new Date(booking.startDate).toDateString()}{' '}
to {new Date(booking.endDate).toDateString()}
</p>
</div>
</div>
)
})}
</div>
<style jsx>{`
.bookings {
display: grid;
grid-template-columns: 100%;
grid-gap: 40px;
}
.booking {
display: grid;
grid-template-columns: 30% 70%;
grid-gap: 40px;
}
.booking img {
width: 180px;
}
`}</style>
</div>
}
/>
)
}
export async function getServerSideProps({ req, res, query }) {
const bookings = await Booking.findAndCountAll()
const houses = await House.findAndCountAll()
return {
props: {
bookings: bookings.rows.map((booking) => {
booking.dataValues.createdAt = '' + booking.dataValues.createdAt
booking.dataValues.updatedAt = booking.dataValues.updatedAt + ''
return booking.dataValues
}),
houses: houses.rows.map((house) => house.dataValues)
}
}
}
Awesome!
See how we get, in addition to the houses data, the bookings data.
Now all the upcoming bookings should show up:
We can also add a link to this page in the components/Header.js
component.
Add:
<li>
<Link href='/bookings'>
<a>Bookings</a>
</Link>
</li>
before the line:
<li>
<a>Logged in</a>
</li>
Here’s the end result:
Only seeing your own bookings
There’s a big problem in pages/bookings.js
, and I’m sure you already noticed.
This screen prints all the bookings. Our bookings, and all the bookings of other people using the site. Also, all bookings are shown even if you’re not logged in.
Let’s first make sure if we’re not logged in we can’t see this page.
First, import the cookies
npm library.
import Cookies from 'cookies'
In getServerSideProps
, import and get the cookie that we set when the user logs in:
const cookies = new Cookies(req, res)
const nextbnb_session = cookies.get('nextbnb_session')
If the cookie is not found, redirect to /
:
if (!nextbnb_session) {
res.writeHead(301, {
Location: '/'
})
res.end()
return { props: {} }
}
Now, also set the loggedIn
easy-peasy state when loading this page from the server, like we do for the other pages.
Add
nextbnb_session: nextbnb_session || null,
to the returned props of getServerSideProps
, then at the top import:
import { useEffect } from 'react'
import { useStoreActions } from 'easy-peasy'
And inside the Bookings component, first list the props that we accept:
export default function Bookings({ bookings, houses, nextbnb_session }) {
Then proceed to set the state based on the cookie value:
const setLoggedIn = useStoreActions((actions) => actions.login.setLoggedIn)
useEffect(() => {
if (nextbnb_session) {
setLoggedIn(true)
}
}, [])
Now in getServerSideProps
based on the session cookie value, we get the user and we filter its bookings:
const user = await User.findOne({
where: { session_token: nextbnb_session }
})
bookings = await Booking.findAndCountAll({ where: { userId: user.id } })
This is the final code for pages/bookings.js
:
import axios from 'axios'
import Head from 'next/head'
import Cookies from 'cookies'
import { Booking, House, User } from '../model.js'
import { useEffect } from 'react'
import { useStoreActions } from 'easy-peasy'
import Layout from '../components/Layout'
export default function Bookings({ bookings, houses, nextbnb_session }) {
const setLoggedIn = useStoreActions((actions) => actions.login.setLoggedIn)
useEffect(() => {
if (nextbnb_session) {
setLoggedIn(true)
}
}, [])
return (
<Layout
content={
<div>
<Head>
<title>Your bookings</title>
</Head>
<h2>Your bookings</h2>
<div className="bookings">
{bookings.map((booking, index) => {
const house = houses.filter(
(house) => house.id === booking.houseId
)[0]
return (
<div className="booking" key={index}>
<img src={house.picture} alt="House picture" />
<div>
<h2>
{house.title} in {house.town}
</h2>
<p>
Booked from {new Date(booking.startDate).toDateString()}{' '}
to {new Date(booking.endDate).toDateString()}
</p>
</div>
</div>
)
})}
</div>
<style jsx>{`
.bookings {
display: grid;
grid-template-columns: 100%;
grid-gap: 40px;
}
.booking {
display: grid;
grid-template-columns: 30% 70%;
grid-gap: 40px;
}
.booking img {
width: 180px;
}
`}</style>
</div>
}
/>
)
}
export async function getServerSideProps({ req, res, query }) {
const cookies = new Cookies(req, res)
const nextbnb_session = cookies.get('nextbnb_session')
let bookings
if (!nextbnb_session) {
res.writeHead(301, {
Location: '/'
})
res.end()
return { props: {} }
}
const user = await User.findOne({
where: { session_token: nextbnb_session }
})
bookings = await Booking.findAndCountAll({ where: { userId: user.id } })
const houses = await House.findAndCountAll()
return {
props: {
bookings: bookings
? bookings.rows.map((booking) => {
booking.dataValues.createdAt = '' + booking.dataValues.createdAt
booking.dataValues.updatedAt = booking.dataValues.updatedAt + ''
return booking.dataValues
})
: null,
houses: houses.rows.map((house) => house.dataValues),
nextbnb_session: nextbnb_session || null
}
}
}
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
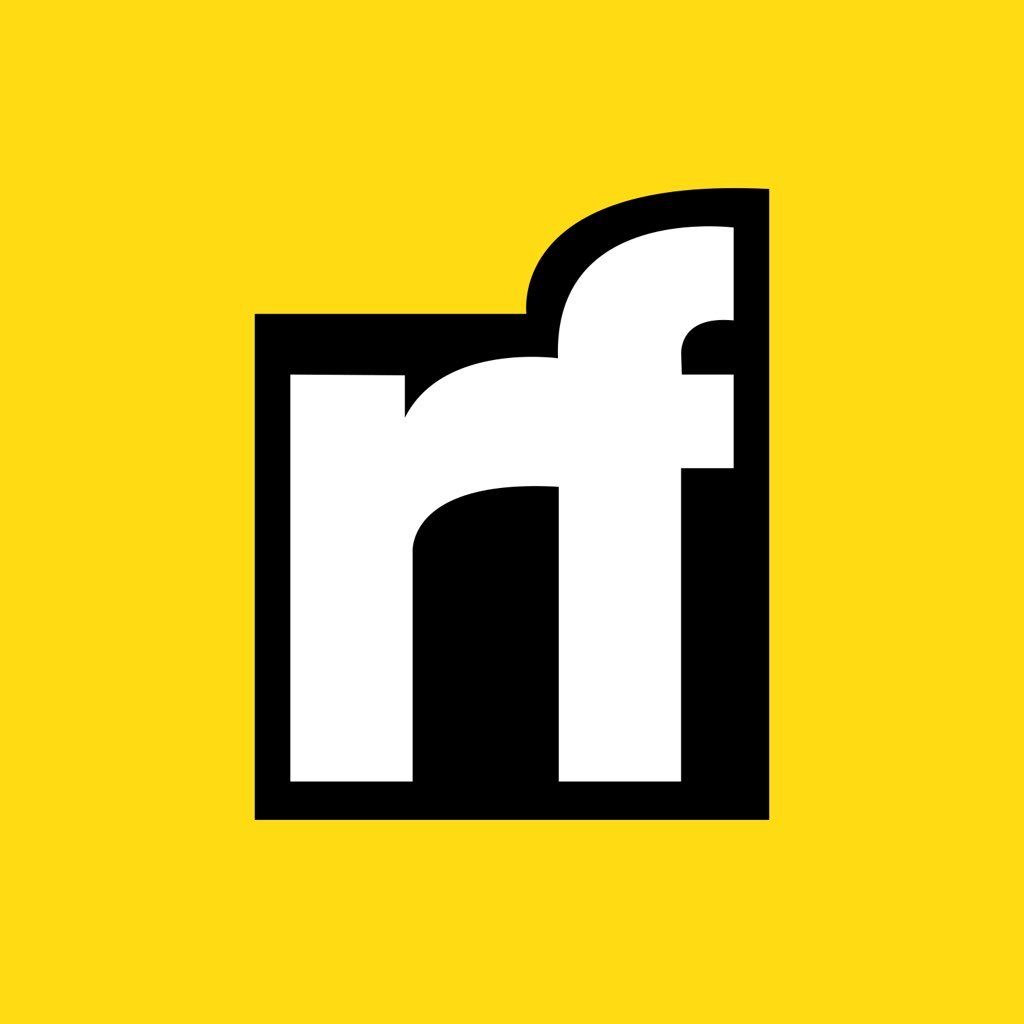
flaviocopes.com | Sciencx (2021-12-29T05:00:00+00:00) Airbnb clone, view bookings. Retrieved from https://www.scien.cx/2021/12/29/airbnb-clone-view-bookings/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.