This content originally appeared on DEV Community and was authored by Michel Canta
Svelte-Kit has been an amazing tool to create great responsive and reactive websites. But with its roots as a compiler, I wondered how I could use it to create a Chrome Extension, and as it turns out… it's really simple.
Today, we're going to see how we can build a simple extension with Svelte-kit and use Chrome's Manifest V3 which will allow us to use promisified versions of many of the Chrome API's methods.
Note: this method can work with Manifest V2 as well
1. Setting Up Our Repo
We'll start with an empty repo where we will create the template svelte kit project.
npm init svelte@next my-extension
cd my-app
npm install
npm run dev
We are going to replace the default index page that is created with the following code that will allow us to get the url of the current tab:
Note: We are using the Chrome api as other methods are blocked by the extension executer for safety reasons.
Update the body
tag in the the app.html
file to configure the max size of the extension popup as such:
Once updated we will create our manifest file in the /static
folder. We will be using manifest V3 here as that is the latest from the Chrome team.
{
"name": "My first Svelte Extension",
"description": "A browser extension made with Svelte Kit",
"version": "1.0.0",
"manifest_version": 3,
"permissions": [
"activeTab"
],
"action": {
"default_title": "Svelte Extension",
"default_icon": "favicon.png",
"default_popup": "index.html"
}
}
Now when we build our extension, the manifest file will be included in our build folder making it much easier and cleaner.
Finally we need to change our adapter to the sveltekit-adapter-chrome-extension
as the usual @sveltekit/adapter-static
creates an inline script that is blocked in Manifest V3 due to Content Security Policies (The same is true for Manifest V2 but you are able to define a Content Security Policy rule with a the sha256 of the script or a nonce, that bypasses it in Manifest V2).
npm install -D sveltekit-adapter-chrome-extension
We are then able to build our project running npm run build
2.Testing our Extension
Click on the extension icon in your chrome browser and then click on manage extensions
Make sure that developer mode is turned on (on the top right side of the page) then click on Load Unpacked
.
Select your build
folder from the file picker and open it. You should now see your extension on the page.
Make sure it is enabled and navigate to any page you want. Toggle the extension and press the button!
There you go! You've just made your first Chrome extension using Svelte Kit and Manifest v3!
This content originally appeared on DEV Community and was authored by Michel Canta
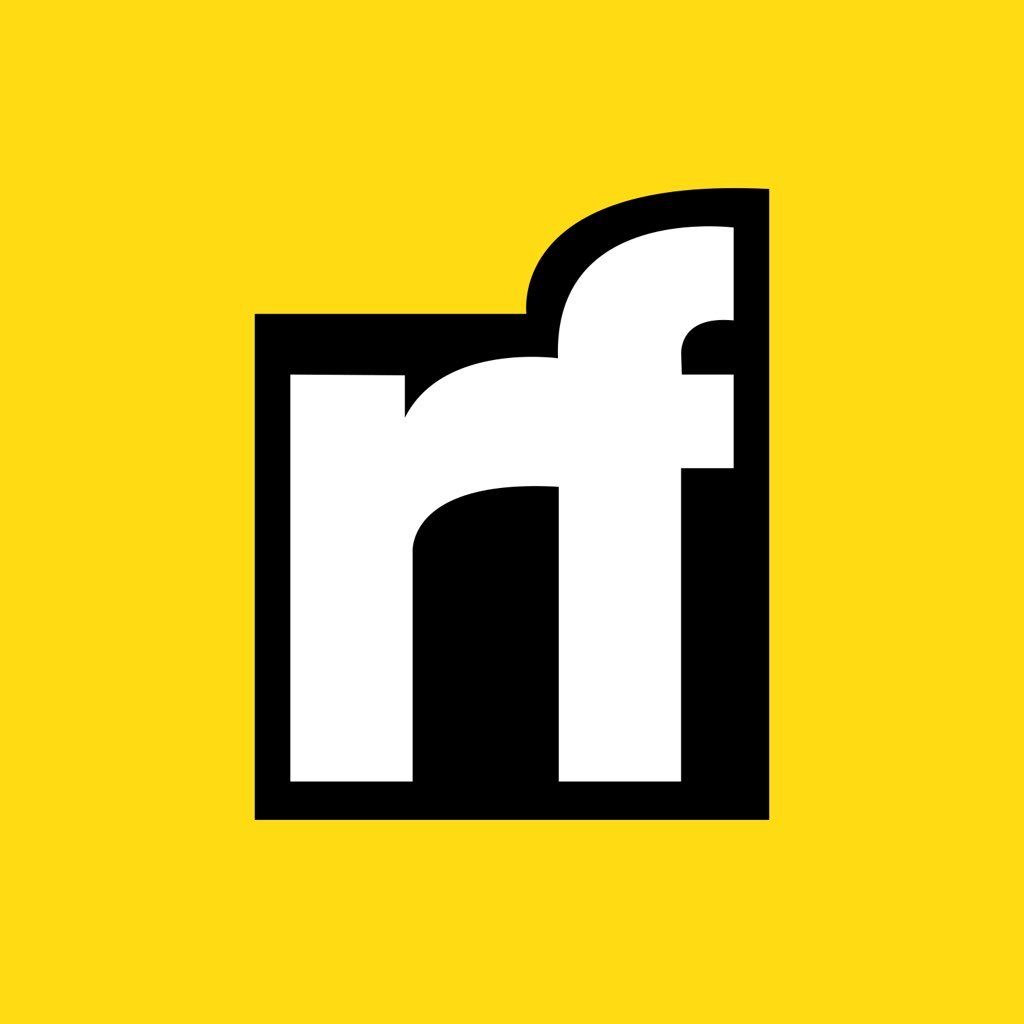
Michel Canta | Sciencx (2022-01-16T08:12:35+00:00) Writing Chrome Extensions Using Svelte-Kit and Manifest v3. Retrieved from https://www.scien.cx/2022/01/16/writing-chrome-extensions-using-svelte-kit-and-manifest-v3/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.