This content originally appeared on Bits and Pieces - Medium and was authored by Matthias Böhm

Debounce: Understand and learn how to use this essential JavaScript skill. Relevant for job interviews too!
What is debounce?
Debounce delays the processing of a function bound to a certain user input event until a certain amount of time has passed.
In other words the function is only executed once per specific user input event, even it the event is triggered multiple times. For example, this can prevent a user from constantly pressing a button more times than is required.
One well known use case for debounce is a search bar that gives suggestions while you type.
You may not want to fetch suggestions from your API every time a user presses a key, as this could create a huge load in the backend and hinder performance.
Instead, you can debounce this function, so only after the user has stopped typing for more than a certain amount of time (either pause between words or finished typing), only then a call to the API is made to fetch the suggestions.
To boil it down: Debounce delays calling a function until a certain amount of time has passed, in which the function has not been called.
Or with an example: Call this function only if 250 milliseconds have passed in which the function has not been called.
Why is this useful?
Besides the benefits listed, it is also a common interview question and interviewers love to ask you to implement your own version of debounce, because it involves some intermediate and advanced concepts of JavaScript.
Most of the time it is used when dealing with user input or DOM events. Those events tend to fire very often and you definitely do not want to call a function that reacts to this thousand times per second.
Here are some common use cases:
- Window resizing: Wait until the user is finished resizing the window, instead of triggering a function 100–1000x times;
- Search bar: Wait until the user has finished typing (or stopped for long enough), before fetching results from an API;
- Double Clicking: Prevent double clicking on buttons;
- Scrolling: React to scrolling events;
- Drag and drop: Improving performance (drastically) when implementing drag and drop functionality.
How is debounce different from throttle?
Debounce and throttle are very similar.
Both limit the execution of a function, so it is not called too often.
However, throttle limits the number of times a function can be called over time.
So if you throttle a function, that would normally run 3000 times in a second to execute only once every 100 milliseconds, it will only run 10 times in a second.
Debounce however, makes sure, that a certain amount of time has passed until the function is executed.
So if a user types very fast, say every 50 milliseconds and your debounce threshold is set to 500 milliseconds, 500 milliseconds after the user has stopped typing the function will be executed.
Or if they type very fast, but pauses in between for more than 500 milliseconds (for example, after finishing one word), then the function is executed as well.
How to implement debounce in JavaScript
Here is a possible implementation of the debounce function is JavaScript.
function debounce(func, delay = 250) {
let timerId;
return (...args) => {
clearTimeout(timerId);
timerId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
The debounce function is a higher-order function, meaning you can pass your own function to it and it returns a debounced version of it.
This is done by forming a closure around the function you pass into it, while storing the delay and the timer id.
Every time the debounced function is called, it sets a timeout after which the actual function is executed. Let’s say the timeout is 300 ms.
It also stores the timer id, so if the debounced function is called earlier than those 300ms, the previously scheduled function will be cleared and a new execution of the function is scheduled after 300 ms.
If the debounced function is not called within the timeout, the actual function is executed. If not, the timeout is cleared and as described above the execution is scheduled again.
How to use it?
Let’s say we have a function that reacts to any kind of user input by printing into the console.
We just need to debounce this function and use the debounced version where ever we like.
function userInput(){
console.log('User input');
}
const debouncedUserInput = debounce(userInput);
For example on a button:
<button onclick="debouncedUserInput()">User input</button>
Or on an input field:
<input type="text" id="searchBar" onkeyup="debouncedUserInput()" />
Or on a resize window event:
window.addEventListener('resize', debouncedUserInput);
And course everywhere in your JavaScript code.
Implementations of debounce in JavaScript libraries
Now you know how to implement and use your own debounce function.
While this knowledge is very useful, you might also want to use the debounce function implemented in famous JavaScript libraries.
Here you find the implementations of debounce in different JavaScript libraries:
Build component-driven. It’s better, faster, and more scalable.
Forget about monolithic apps, start building component-driven software. Build better software from independent components and compose them into infinite features and apps.
OSS Tools like Bit offer a great developer experience for building component-driven. Start small and scale with many apps, design systems or even Micro Frontends. Give it a try →
How to Delay a Function in JavaScript was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Matthias Böhm
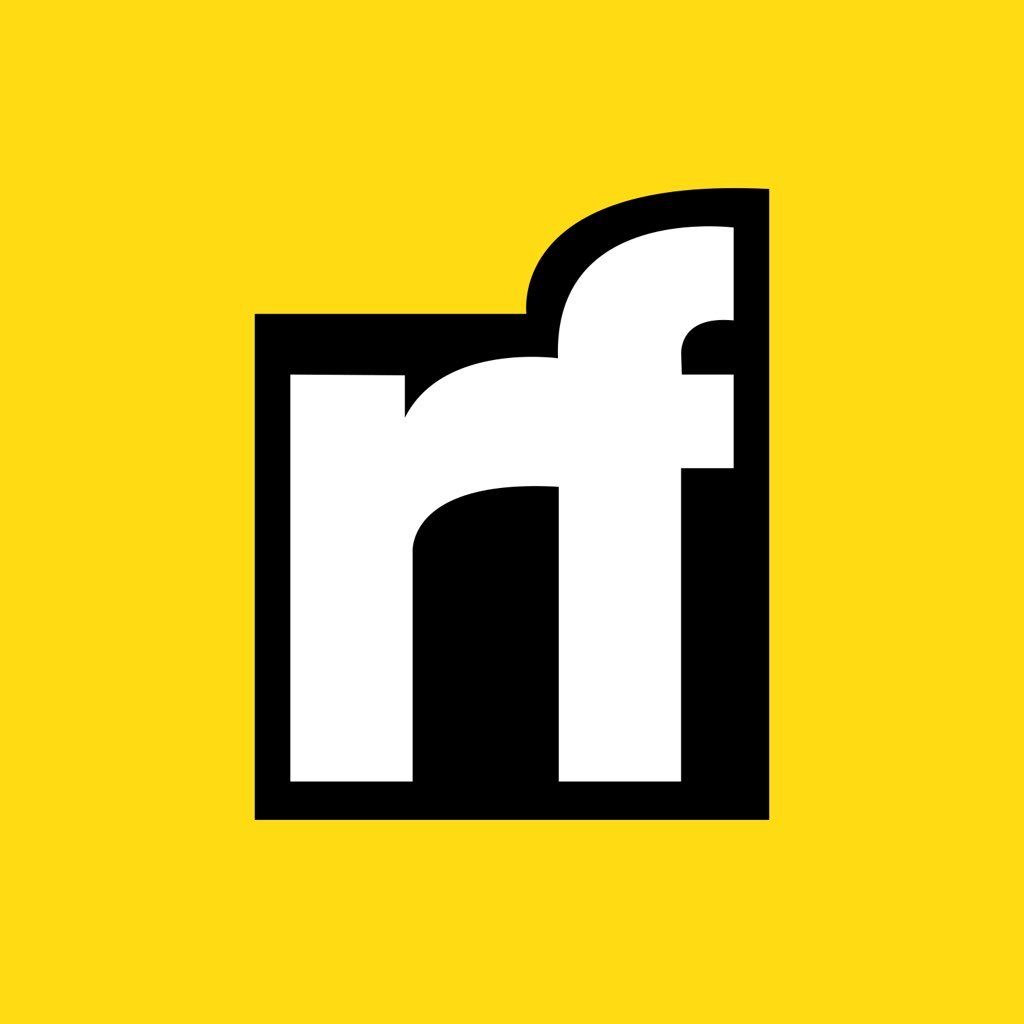
Matthias Böhm | Sciencx (2022-01-25T10:51:10+00:00) How to Delay a Function in JavaScript. Retrieved from https://www.scien.cx/2022/01/25/how-to-delay-a-function-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.