This content originally appeared on Bits and Pieces - Medium and was authored by Piyush Dubey
What is an ORM?
In simple terms, an ORM system is a technique where you use an object-oriented paradigm to create a mapping between application and database to perform data manipulation and query directly.
When it comes to retrieving and insertion over joins and relationships, it will follow the same paradigm to manipulate or query data related to operations.
For example, a simple inner-join query will query data like this:
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
This inner join query will return a list of data in rows but you might want to aggregate this into some more readable format.
Let’s begin
I have created a small boilerplate to get things kick-started earlier. I am also assuming you have an understanding of Node.js and know how to connect to databases before beginning. But whatever your skill level, you can find the source code here.
With our source code, run the following commands in the terminal:
1. docker-compose build
2. docker-compose up
Now if you go to route localhost:5000/ you will see an empty object prompt up. There is a folder named migrations where I have added 3 tables i.e users, projects, and pages related to linear fashion respectively in their migrations using db-migrate the package.
The app is built with Express so you can read about that as well if you haven’t used it before.
Schema
The current schema for our database is:
- User table
- Project table ( 1— m with User)
- Page table ( 1 — m with Project)
And our approach will be to create a class that will be inherited into all models files with the same methods that will be shared among all like, get or save.
Let’s create a folder and file named /models/index.js and create a class in it named DatabaseOperations:
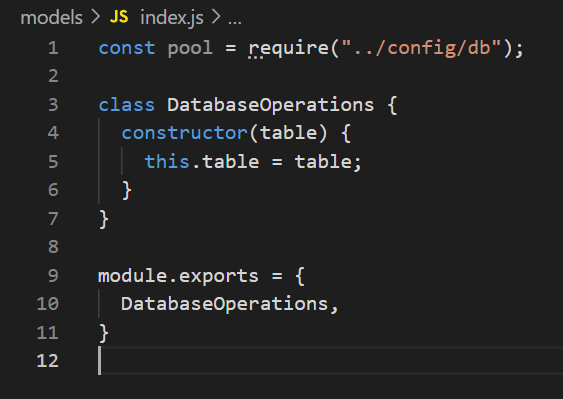
Here, we are exporting the class with a simple constructor method to get the common name.
Let’s create mode files and put some content there like the below pictures:
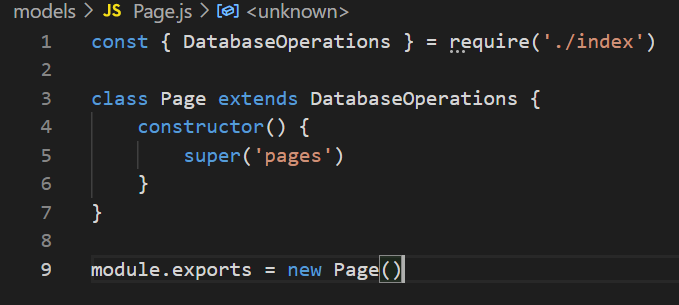
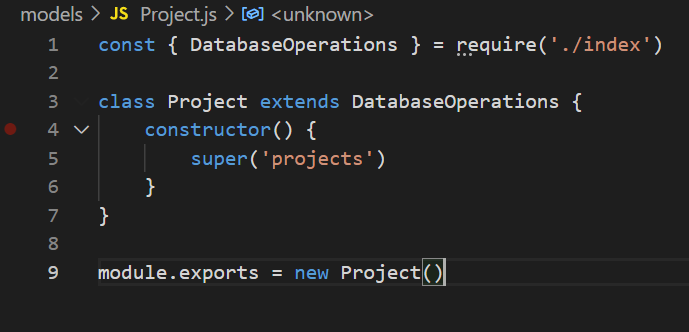
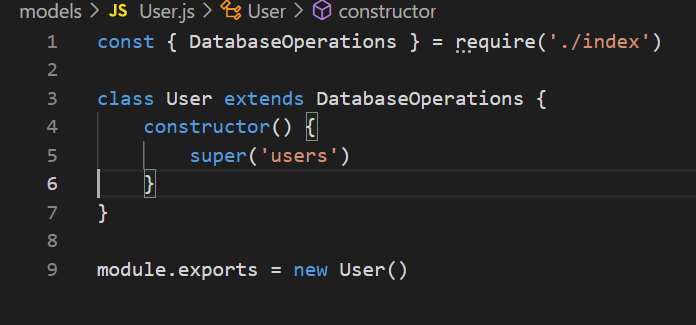
These are simple classes that are just using the database operations class as a parent.
Now, let’s create a method inside DatabaseOperation to fetch the records
But wait, even if we are using classes we need something to build our queries right so first create a query builder to generate queries:
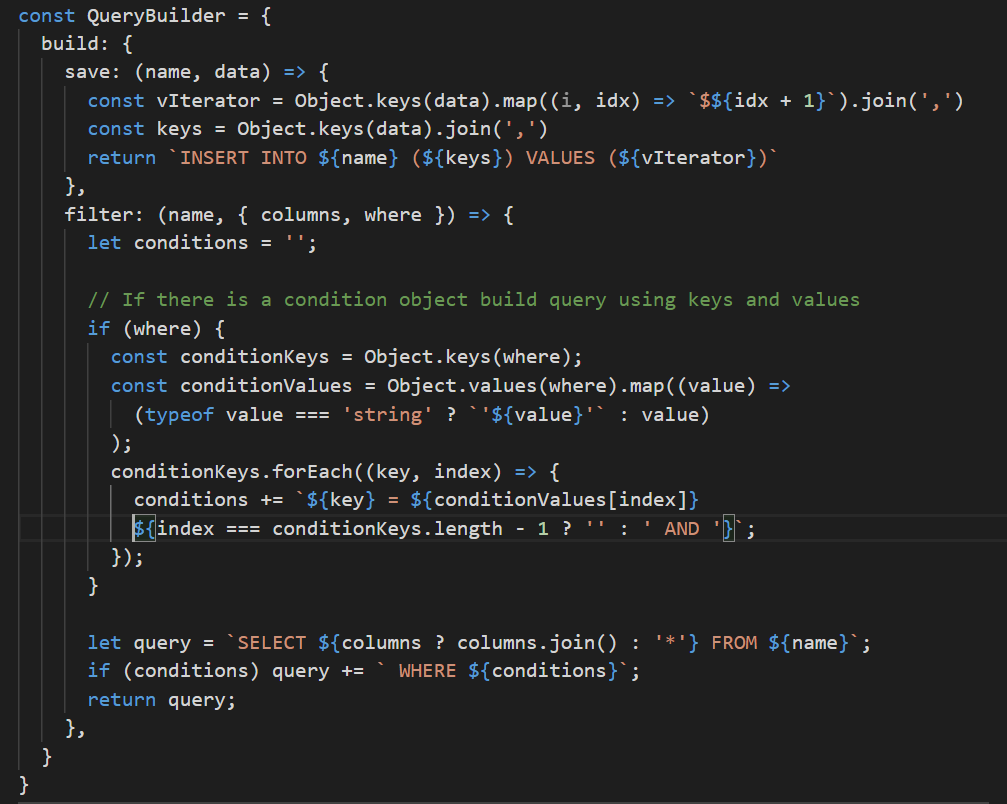
Here, we are using two methods to fetch with filter and save the record. It is just building the queries. This will not build the native queries instead build the package query to formulate the advantage of the package.
Let’s now look at creating the GET and POST methods for DatabaseOperation.
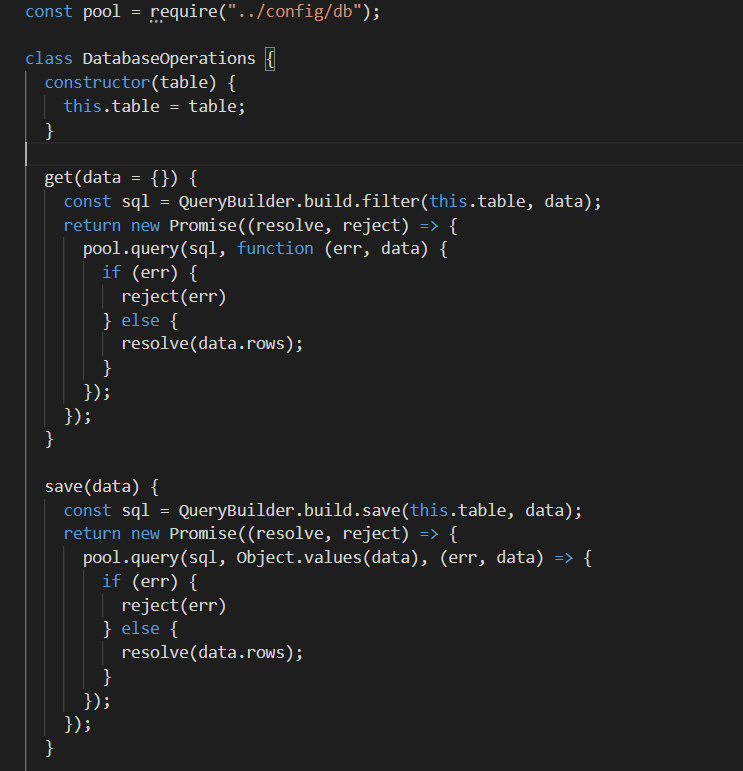
Let’s use them, update the routes to make use of these methods to store and get the records
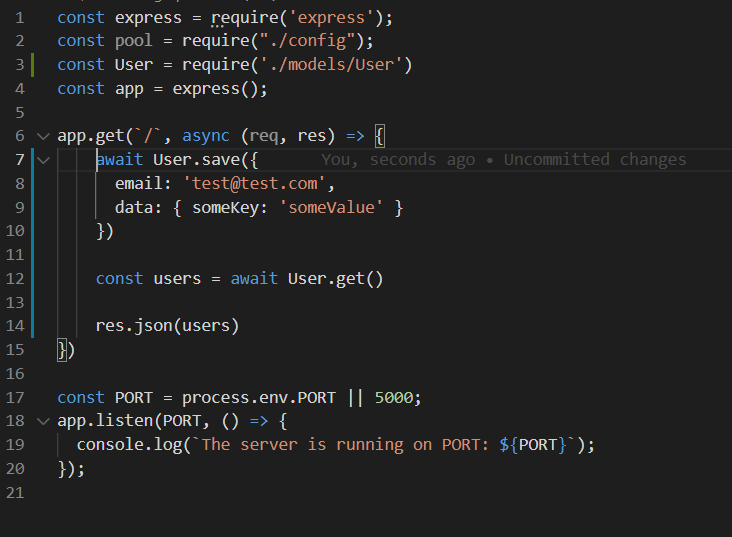
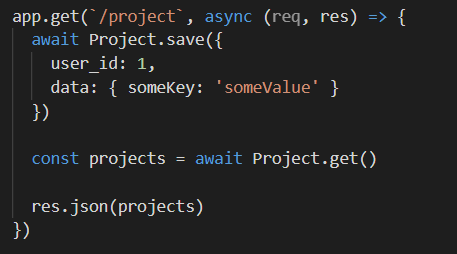
Manage Relationship
We can also manage relationships to maintain the order of data fetching. To manage we need to do some other items too, such as:
- Add a new method in the query builder
- Add a new method in DatabaseOperation class to include record
- Add an individual entry to get the relation
See how this is done in the links for the relationship item, I will update the article on some other day with the following and more robust approach to manage it.
- https://github.com/Piyush-Use-Personal/custom-orm/blob/orm/models/index.js#L20
- https://github.com/Piyush-Use-Personal/custom-orm/blob/orm/models/index.js#L122
- https://github.com/Piyush-Use-Personal/custom-orm/blob/orm/models/User.js#L9 ( for many to many )
- https://github.com/Piyush-Use-Personal/custom-orm/blob/orm/models/Project.js#L20 (for one to many relationships)
- Below is an example of a relationship that includes
const data = await User.include([User.PROJECT], {
where: {
user_id: userId
}
})
Conclusion
Now we have created our custom ORM to get, save, and other operations on multiple tables at the same time.
I have updated the repo with other methods too which include:
- update
- delete
- include (relationship)
You can find the source code in branch ORM on the repo.
Build with independent components, for speed and scale
Instead of building monolithic apps, build independent components first and compose them into features and applications. It makes development faster and helps teams build more consistent and scalable applications.
OSS Tools like Bit offer a great developer experience for building independent components and composing applications. Many teams start by building their Design Systems or Micro Frontends, through independent components.
How to Create an ORM in Node.js was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Piyush Dubey
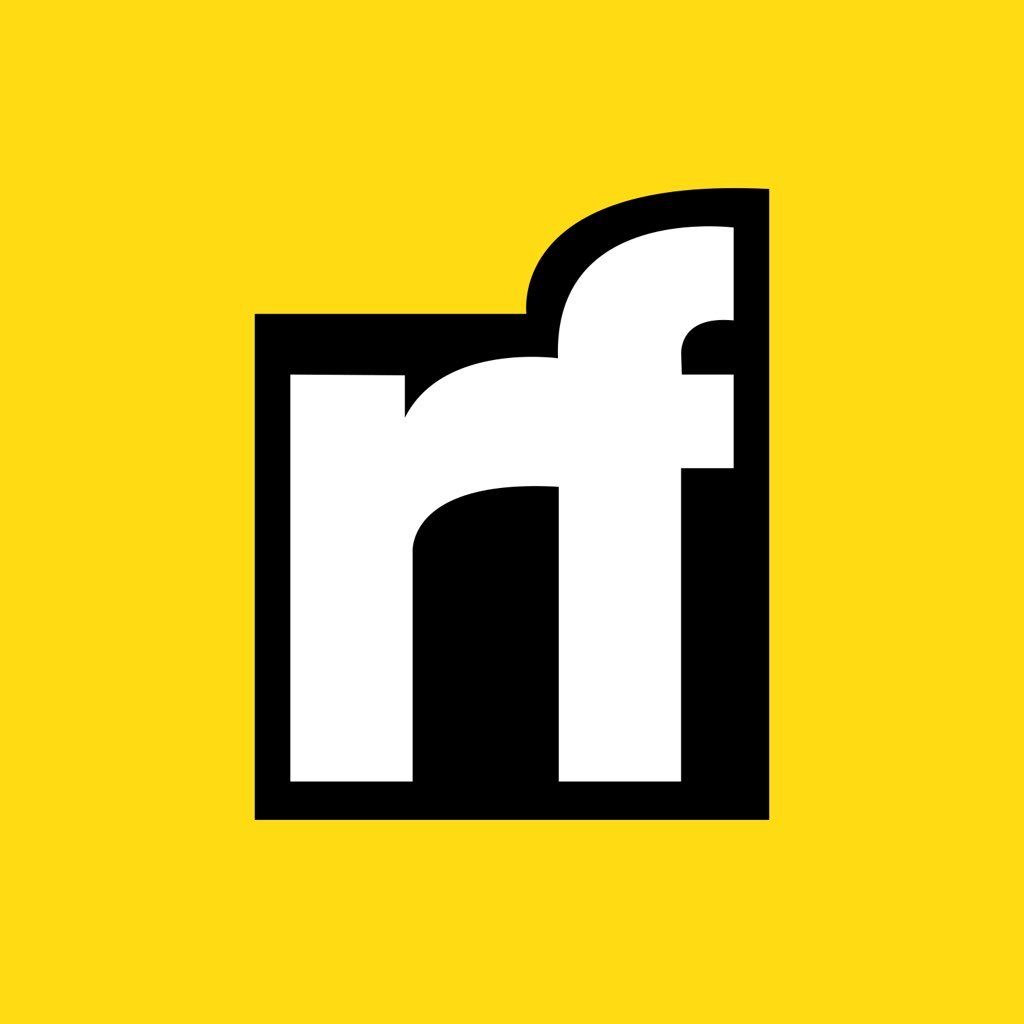
Piyush Dubey | Sciencx (2022-01-31T17:30:48+00:00) How to Create an ORM in Node.js. Retrieved from https://www.scien.cx/2022/01/31/how-to-create-an-orm-in-node-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.