This content originally appeared on Level Up Coding - Medium and was authored by Mike Ogodo
In this two part series, we are going to go through;
- The process of creating a simple lightweight RESTful API using Laravel Lumen framework
- Consuming this API in a react-native mobile app using Axios, Javascript’s library for querying the API and Redux library for state management.
So prepare a cup of coffee or get your favorite drink, put on some smooth background music,then lets get to it:
First Part: Building a simple API using the Lumen Framework
Lumen
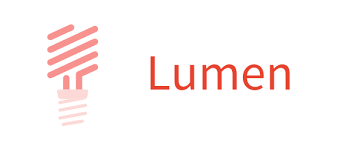
Lumen is a wonderful PHP micro-framework, “micro” in this case alludes to its minimalist approach or lightweight nature. Micro-frameworks such as lumen in our case and another example is flask the python micro-framework, allow you to get up and running(in terms of spinning up/ building an API) without getting bogged down by functionalities such as Accounts , authentication, authorization and roles that you most probably wont need to use for your simple application. That is why i chose Lumen, to concentrate on the integration of the back-end-API to the react-native (this can also be used for react web applications) mobile application.
Lumen’s Features include;
1. Routing
One has to define his or her routes for the application in the routes/web.php file. The most basic Lumen routes simply accept a URI and a closure, i.e:
$router -> get(‘foo’, function() {
return ‘Hello World’;
})
The router allows one to register routes that respond to any HTTP verb,i.e GET, POST,PUT, PATCH, DELETE,OPTIONS
2. Bootstrapping
Lumen’s Bootstrapping processes are located in the file lumen/bootstrap/app.php, this file does the following;
- creates the application
- Registers container Bindings
- Registers configuration files
- Registers application middle-ware
- Loads the Application Routes.
3. Events
Provides a simple observer implementation that allows one to subscribe and listen for events in your application. Event classes are held in the app/Events directory, and their respective listeners held in the application file app/Listeners.
4. Caching
Caching generally helps speed up the application and increase its efficiency. use of robust drivers like Memcached, Redis and Database is supported out of the box. One could install the illuminate/Redis.
5. Queuing
Queuing services are similar to the ones offered by laravel. Lumen queue service provides a unified API across a variety of different queue back-ends. Queues allow one to defer the processing of a time consuming task until a later time which drastically speeds up web requests to your application.
Building in Lumen Requires the Following dependencies
Composer
This is a dependency manager for PHP, if you do not have it installed in your computer, navigate to Composer official website and set it up in your machine.Composer is absolutely critical and should be installed first before continuing with the rest of the article.
PHP
Since its the language we will be using to cook up the API it should be installed in your system. Make sure the version is ≥ 7.2 ,get it here. Make sure the PHP extensions OpenSSL, PDO and Mbstring are installed in your system.
MySQL or PostgreSQL
I personally prefer and will be using PostgreSQL in this tutorial , get it here, however this is a personal preference and you could choose to use MySQL or any other database Management system you are comfortable with.
Building a Books And Characters API Rapidly with Lumen
The API will majorly serve two resource; Books and Authors , and we are going to pull this data from an external API: https://anapioficeandfire.com/api/
This is what we need the API to do:
- populate Books resources from an external API
- populate Characters resources from an external API
- Get all Books
- Get all characters
- Get a single Book
- Get a single Character
The endpoints for this API, given books and characters resource will have the following endpoint:
- Get all books — GET /api/books
- Get one book — GET /api/book/1
- Get all characters — GET /api/characters
- Get one character — GET /api/characters/1
The books and character attributes will include the following
- Book : name ,ISBN, authors , release_date, characters
- Character : name, gender, culture, aliases,books
Installing Lumen Micro-Framework
if you are on Linux environment navigate to /var/www create a folder with a name of your choice , i.e practice_folder , navigate into this folder and then run the following command below to create a new project with Lumen.

cd into the newly created project.

If you now run “php -S localhost:3000 -t public” from the projects root, your application will be served in your browser as shown below:
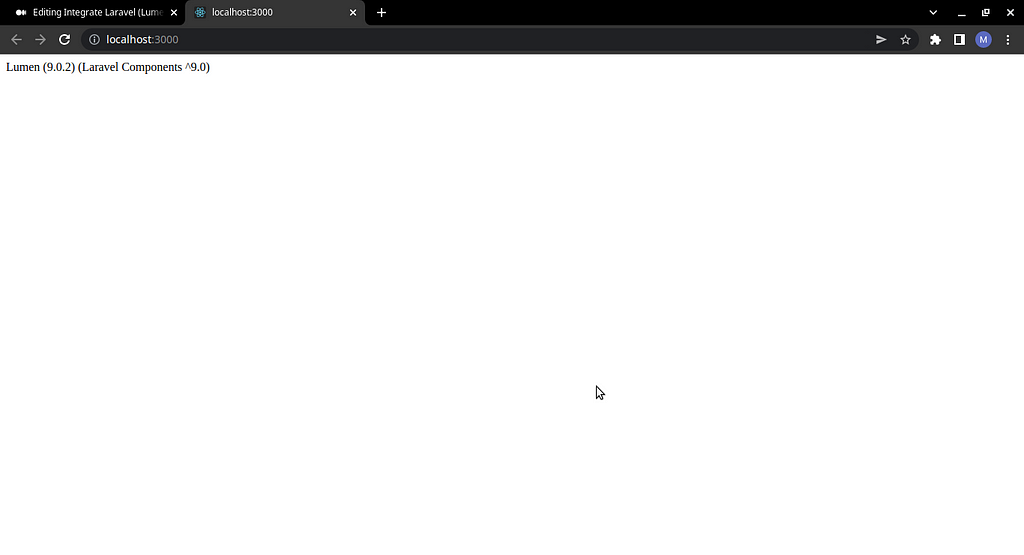
Eloquent and Facades
Eloquent is an object-relational mapper (ORM) that makes it a “breeze” to interact with your databases. When using Eloquent , each database table has a corresponding “model” that is used to interact with that table. Facades provide a “static” interface to classes that are available in the applications service container. All facades are defined in the Illuminate\Support\Facades namespace
Lumen’s entire bootstrap process is located in a single file. Open up the bootstrap/app.php and un-comment the following line;
// app ->withEloquent
Once un-commented, Lumen will attach the Eloquent ORM to your database using the values configured in the .env file.
Create a Database
move to your terminal and type in the following to log into the postgress terminal:
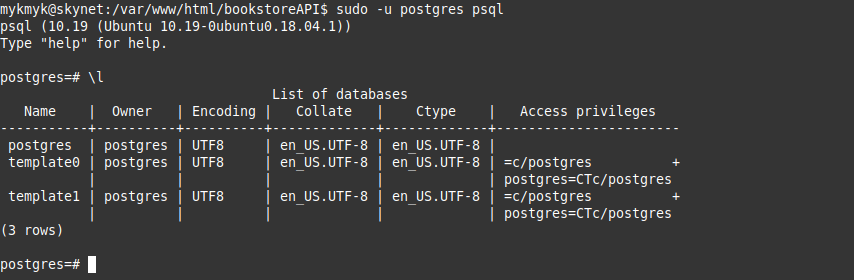
Then you can list the databases by using the command “\l”, after that we create a database for our API called “BooksCharactersAPI” using the command below:
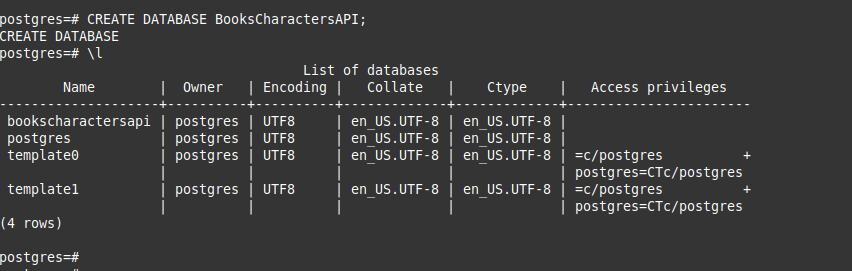
Open up .env file in the projects root and set the right configuration details, my .env file looks as shown below;
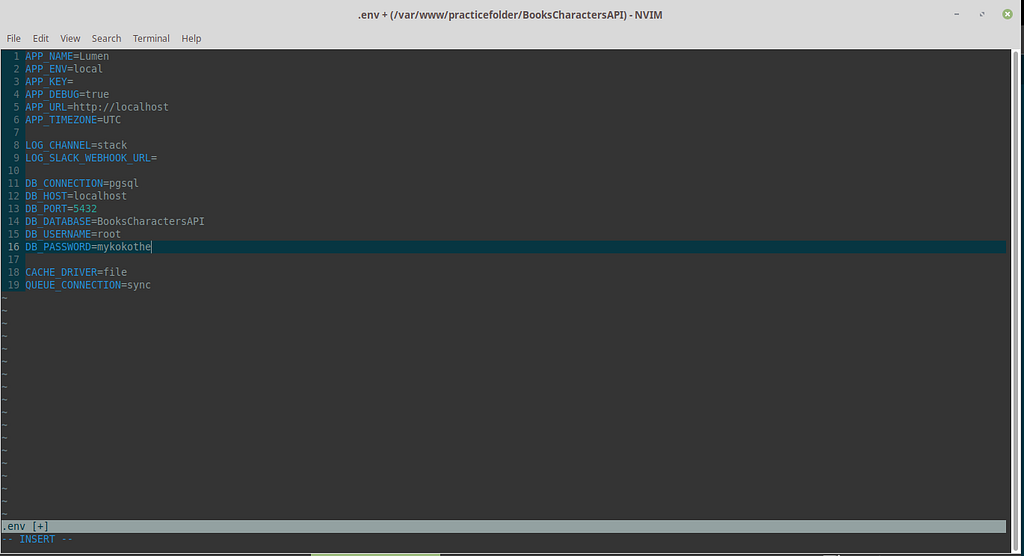
be sure to replace the values to the variables, DB_DATABASE, DB_USERNAME, DB_PASSWORD with your databases’ own, also note that for MySQL most of the values remain the same except for two;
- DB_CONNECTION =MySQL
- DB_PORT = 3306
before we get started we have to add some libraries, because of the lean nature of lumen it excludes some libraries that are present in laravel, for example we wont be able to make models using the command:
- php artisan make:model Book
To enable this, install those libraries using composer:

Then to enable it in our bootstrap/app.php file:
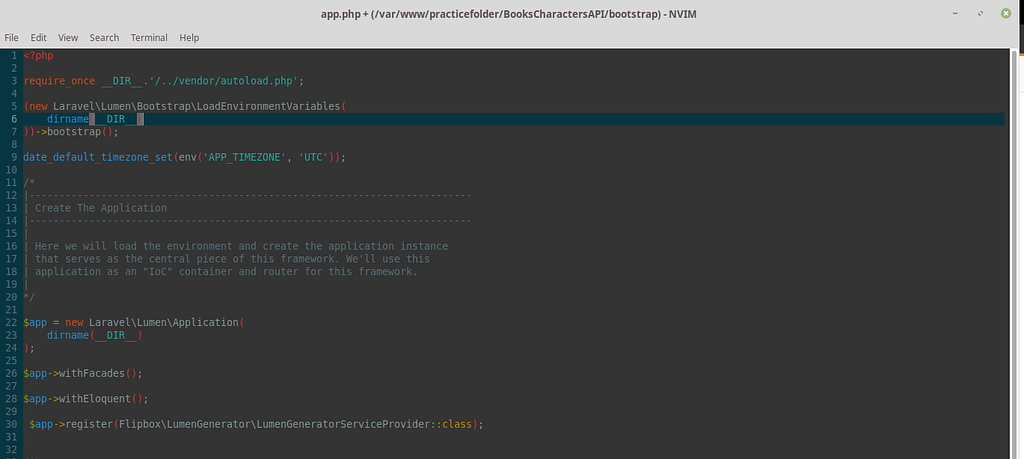
notice in the image above we have also un-commented two lines
- $app->withFacades
- $app->withEloquent
Setting Up Database Models and Migrations
Using our already set up PostgreSQL database that we called “bookscharactersapi”, we will now make use of this database:
First we will create a migration for the books table and characters table. Migrations are a type of version control for your database. They allow a team to modify the database schema and stay up to data on the current schema state.Migrations are usually used in tandem with Schema Builder to easily manage ones applications schema.
books make migrations;

results in

characters make migrations;

results in

These new migrations will be placed in your database/migrations directory. Each migration file contains a timestamp, which allows Lumen to determine the order of the migrations. We will now modify these migrations to include our attributes for characters and books tables:
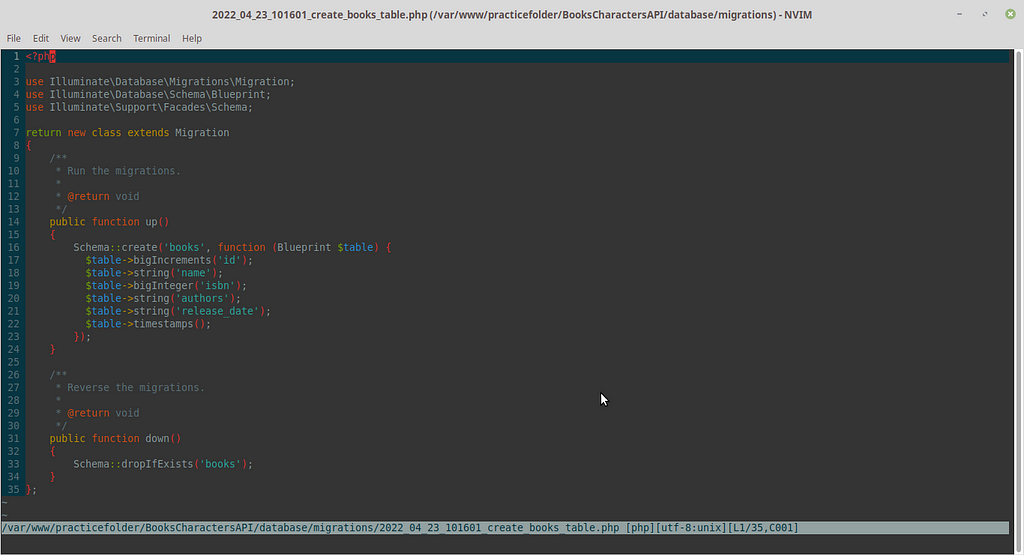
for characters results in;
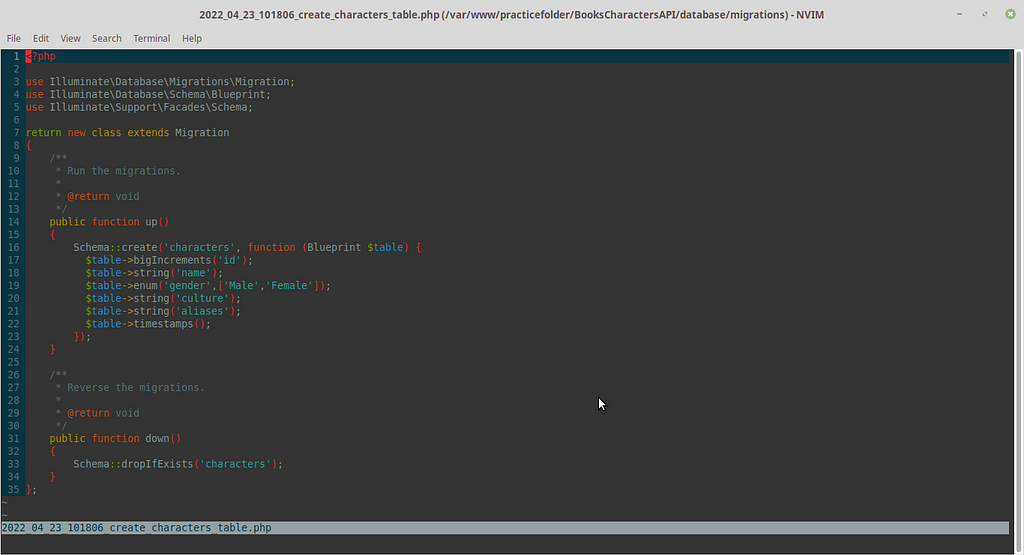
in the files above we are adding a few extra columns to the books and the characters tables i.e name, gender, aliases for characters and ISBN,name for books.
Now we run the migration using the command below

If you run into the following error, relax. We will solve it right below:

The above error shows that our system is missing the PostgreSQL Database driver for PHP, we solve it by installing the driver as below:

if it fails find out your PHP version number, like mine is 8.0.18 and plug this in the above command, it will now look like the one below,
- sudo apt-get install php8.0-pgsql
a successful migration command will result in the below screen:

now if you check your database, you should have books and migrations as below;
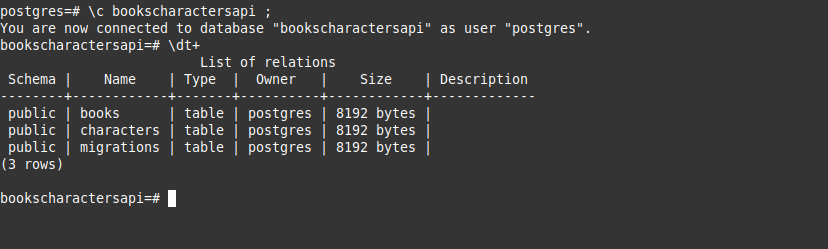
Next, on the root folder run the command below
- php artisan migrate:model Book
- php artisan migrate: model Character
This will generate Model files that live in the directory app\Models and that extends the Illuminate\Database\Eloquent\Model , open both files and fill them as below:
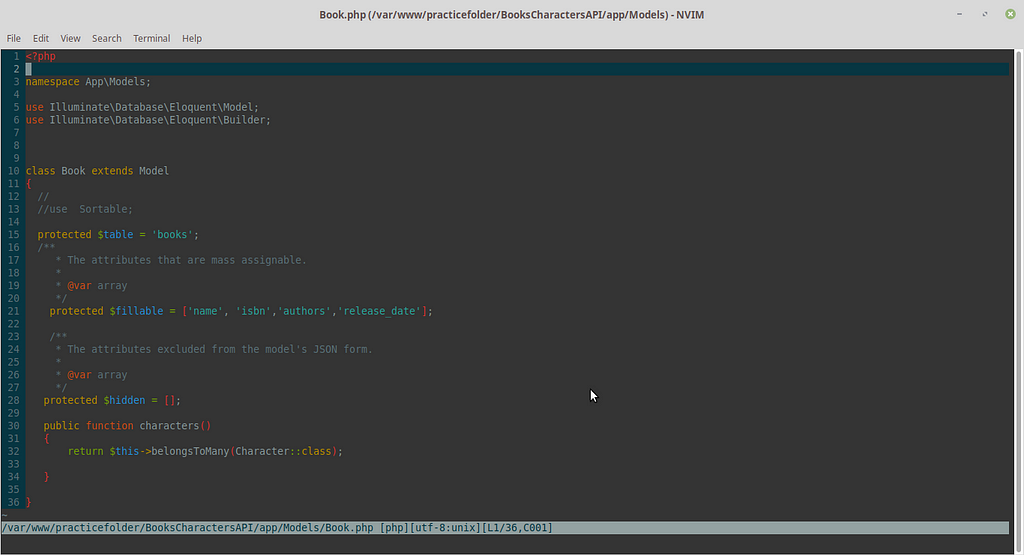
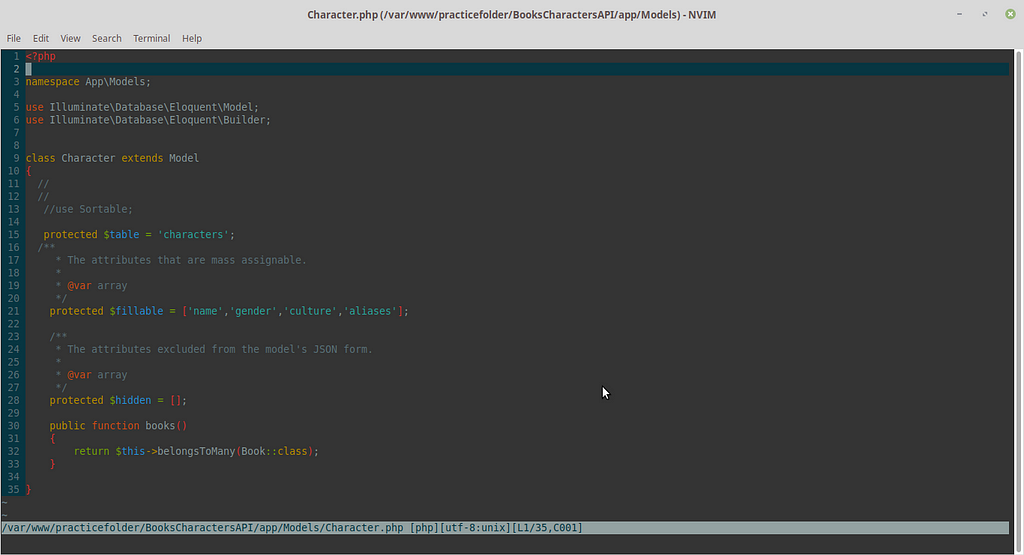
Set up Routes
Lumen routing is fairly simple, we define all the routes for our applications in the routes/web.php file. The most basic Lumen routes simply accept a URI and a closure.
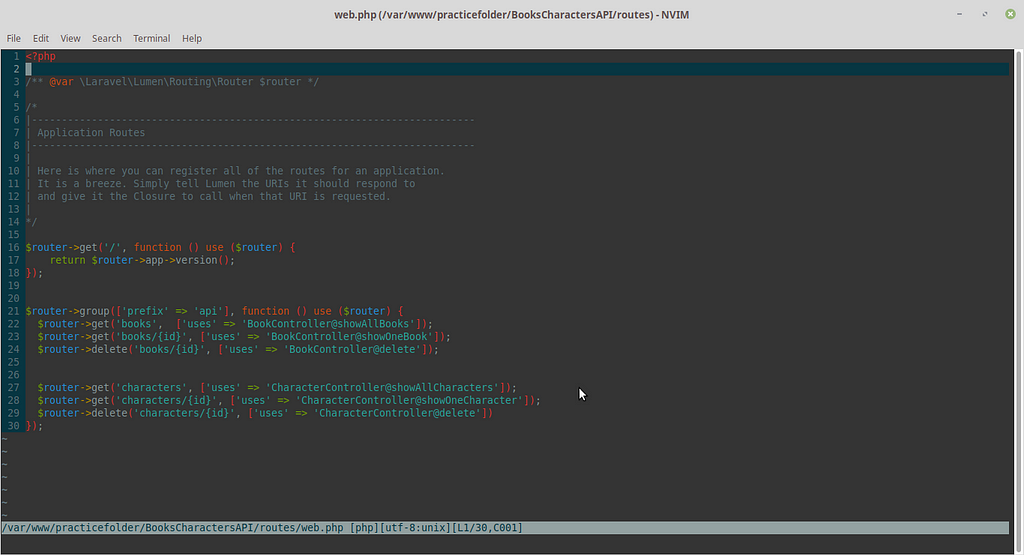
In the code above , we have abstracted the functionality of each route into two controller namely, BookController and CharacterController. Route groups allows one to share route attributes , such as middle-ware or namespaces across a large number of routes without needing to define those attributes on each route. Therefore, as constructed above each route will have a prefix of /api. Next lets create our BookController and CharacterController.
Build Our Book Controller
create a new file, BookController.php in the folder app/Http/Controllers , the code of that file should look like below:
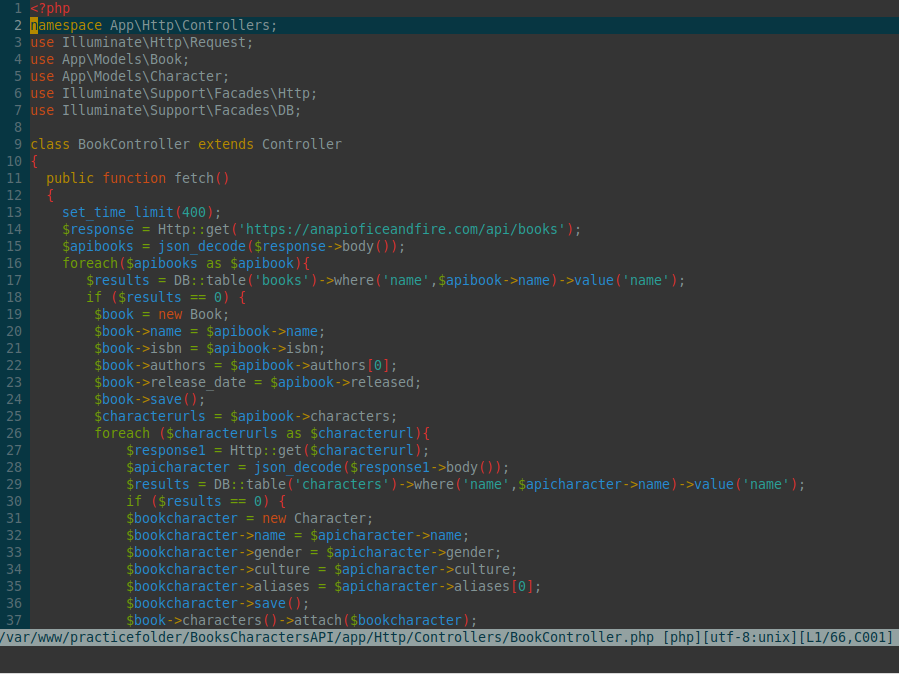
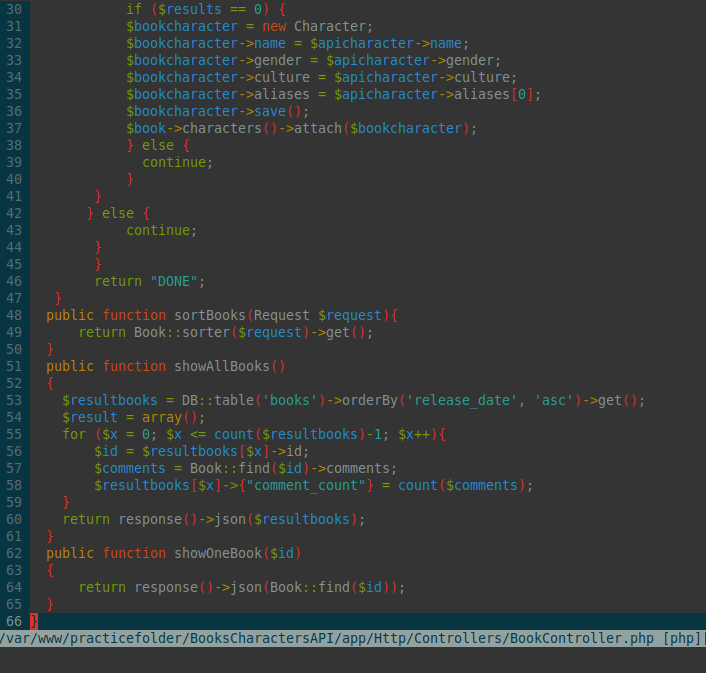
In the code above we have;
required our two models using the lines, “use App\Models\Book” for our Book model and “use App\Models\Character” for our Character model that we had created earlier.
Proceeding, we have created the following methods:
- fetch — /GET -used to fetch all the books and characters from the external API
- sortBooks — /GET -Used to get books in sorted form, one could sort by name etc.
- showAllBooks — /GET - Used to get all books without any modification to the search query
- showOneBook — /GET -Use to get a specific book from the API
for our CharacterController , it looks like below:
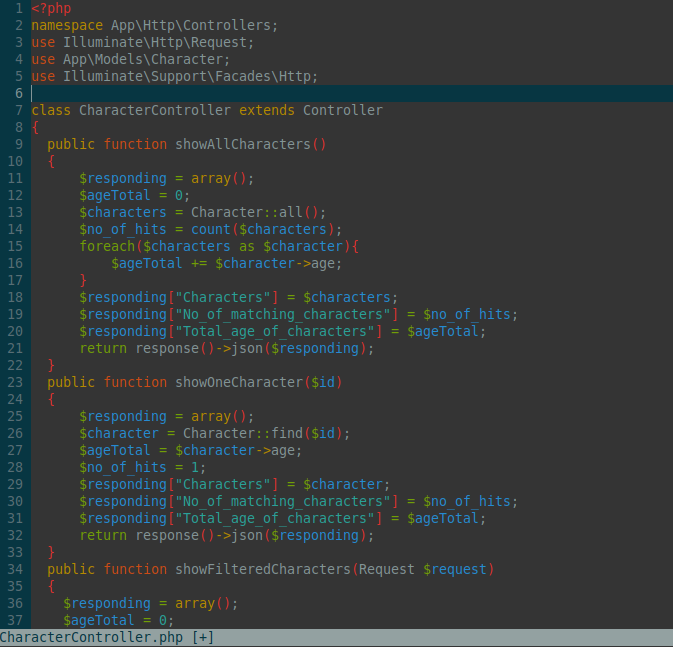
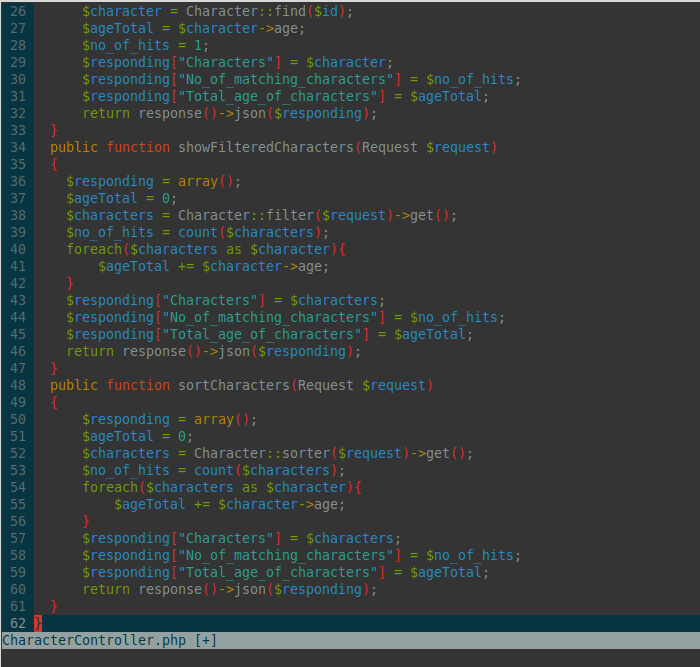
After building out the Controllers, we have to populate our database, to do this we have to invoke the fetch endpoint i.e visit /api/fetch/, which will take sometime to populate the books table and characters table simultaneously.
If you run into the error “”Class “GuzzleHttp\HandlerStack” not found”, check out my answer on stack overflow here (remember to up-vote it).
You can refer to the final mutated code here : https://github.com/myk4040okothogodo/bookstoreAPI/
In the next sequel i talk about filtering and sorting of our books and resource using our own modules, then we will test our APIs(Using POSTMAN) and finally build out our front-end react native application to consume this API.Goodbye, see u then.
Integrate Laravel (Lumen) API with React-Native mobile app Using Redux and Axios. was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Mike Ogodo
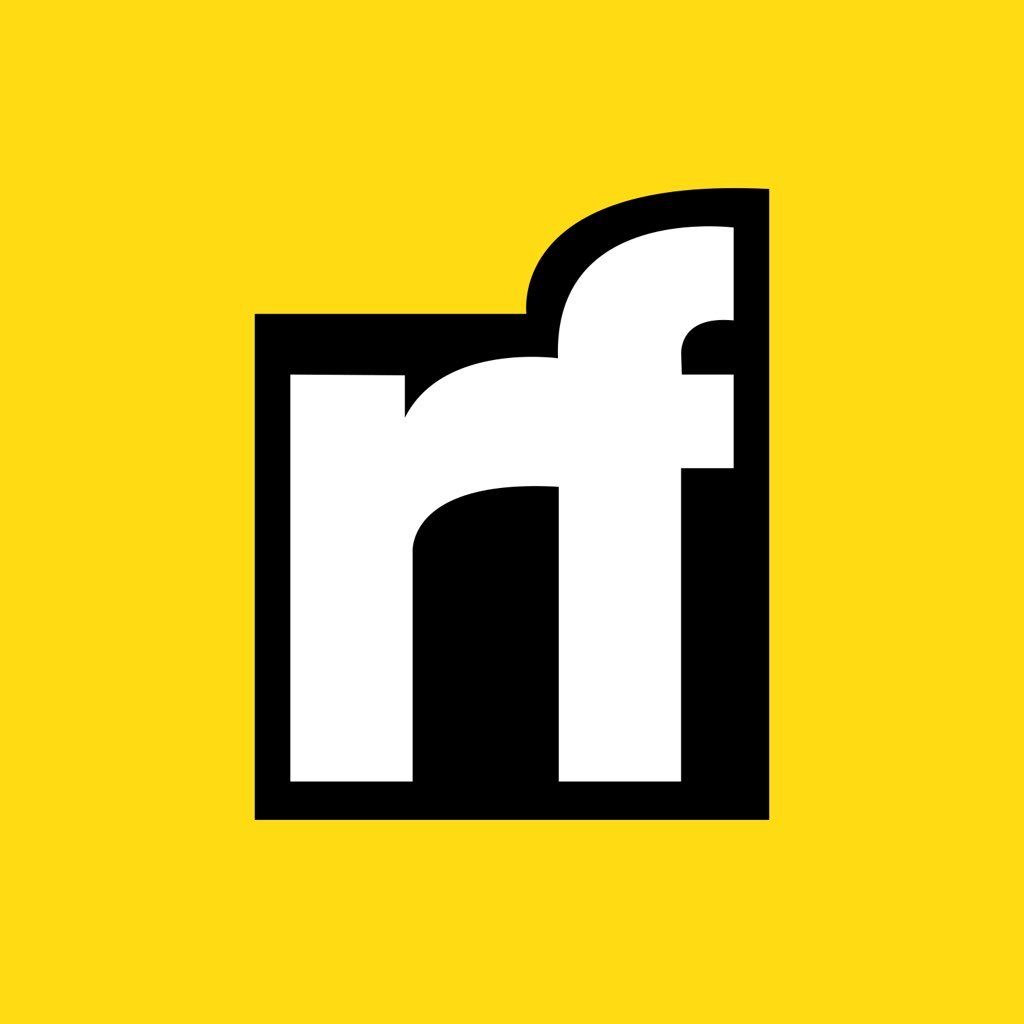
Mike Ogodo | Sciencx (2022-05-05T01:39:58+00:00) Integrate Laravel (Lumen) API with React-Native mobile app Using Redux and Axios.. Retrieved from https://www.scien.cx/2022/05/05/integrate-laravel-lumen-api-with-react-native-mobile-app-using-redux-and-axios/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.