This content originally appeared on David Walsh Blog and was authored by David Walsh
JavaScript Arrays are probably my favorite primitive in JavaScript. You can do all sorts of awesome things with arrays: get unique values, clone them, empty them, etc. What about getting a random value from an array?
To get a random item from an array, you can employ Math.random
:
const arr = [ "one", "two", "three", "four", "tell", "me", "that", "you", "love", "me", "more" ]; const random1 = arr[(Math.floor(Math.random() * (arr.length)))] const random2 = arr[(Math.floor(Math.random() * (arr.length)))] const random3 = arr[(Math.floor(Math.random() * (arr.length)))] const random4 = arr[(Math.floor(Math.random() * (arr.length)))] console.log(random1, random2, random3, random4) // tell one more two
As for when you would need random values from an array is up to your individual application. It’s nice to know, however, that you can easily get a random value. Should Array.prototype.random
exist?
The post Get a Random Array Item with JavaScript appeared first on David Walsh Blog.
This content originally appeared on David Walsh Blog and was authored by David Walsh
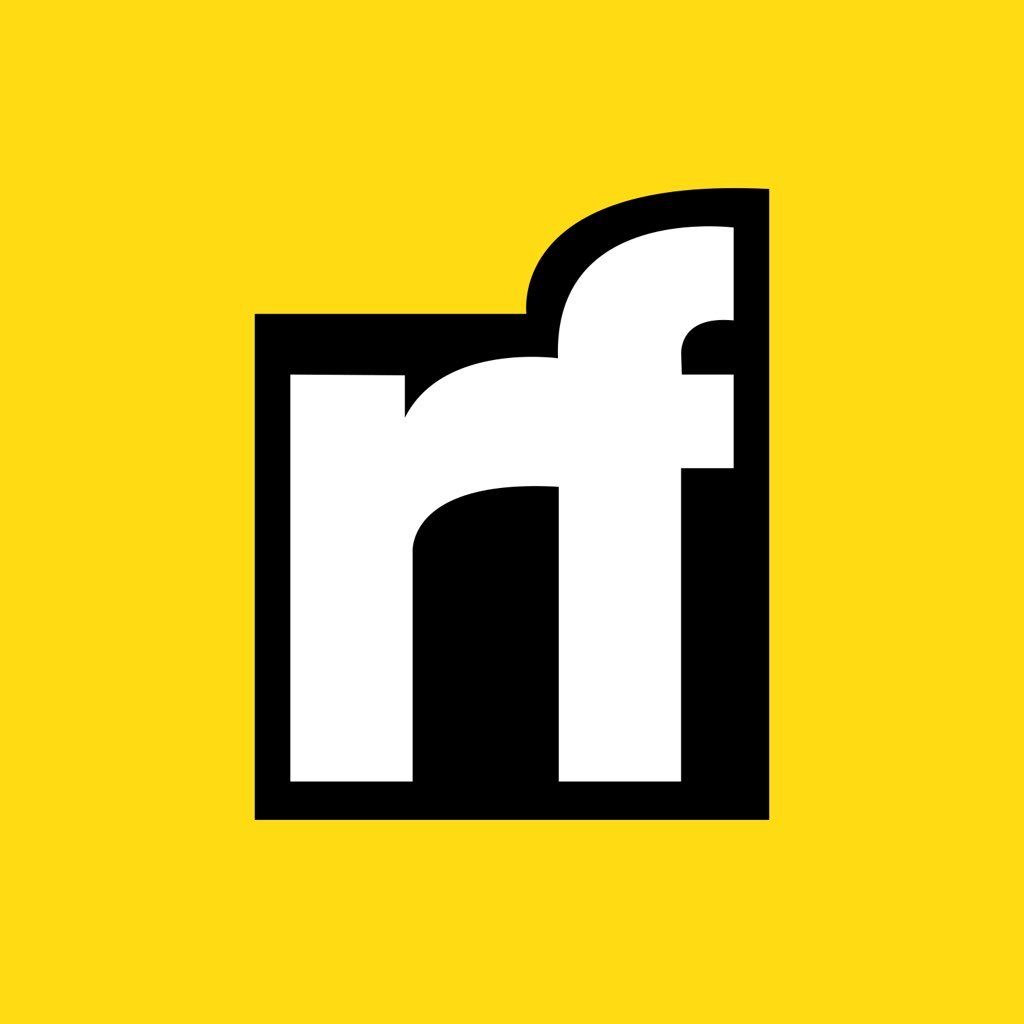
David Walsh | Sciencx (2022-05-09T10:59:17+00:00) Get a Random Array Item with JavaScript. Retrieved from https://www.scien.cx/2022/05/09/get-a-random-array-item-with-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.