This content originally appeared on Bits and Pieces - Medium and was authored by Ashutosh Kumar
A beginner’s guide to how React renders the UI on the browser
One of the most important features of React is components. Components are said to be the building blocks of a React app, they let you break up the user interface into separate pieces that can then be reused and handled independently.
A React component can be either “stateful” or “stateless.” It takes an optional input and returns a React element which is rendered on the screen.
We will dive deep into this topic a little later in this article.
React and React DOM
To work with React in the browser, we need to include 2 libraries: React and ReactDOM.
React library is responsible for creating views and ReactDOM library is responsible to actually render UI in the browser.
React has a Declarative Programming Approach where we don’t directly update the DOM instead we just tell react how the UI should be and then React and React DOM handles the DOM Updates for us.
But where does ReactDOM come in?
ReactDOM is the JavaScript library that allows React to interact with the DOM.
React has nothing to do with the browser or DOM Updates. React DOM plays an intermediator between React and the DOM. After Creating the Components using React Library we want to display them on the browser, React DOM handles this rendering process of displaying the component on Screen by updating the DOM using the ReactDOM.render() function
JSX and React Elements
We know in React UI is divided into components— a React component can be class-based or functional-based. Let’s take an example of a functional component in React:
const App = ()=>
{
return <h1>Hello World!</h1>;
}
From the code, we can say that the component returns the UI, but it’s not HTML that gets returned although the code looks like HTML it’s called JSX.
JSX is a JavaScript extension that gives your JS code an HTML look.
Still, Confused? Let’s understand by an example what happens behind the scenes.
const App = () => {
return <h1>Hello World!</h1>;
}
If you go to the babel website and enter this code into a babel transpiler for React you get the below output:

This shows that JSX is simply syntactic sugar for creating very specific JavaScript objects.
What is React.createElement?
The React element is just a JavaScript object that describes the DOM element in memory. We can create a React element using React’s createElement method.
So until now when we were creating Components we were creating JS Objects that had information for the browser on how the UI should look like.
But if the browser doesn’t understand the React element, then how does the Component get rendered onto the Screen?
As discussed above that’s where ReactDOM comes in, It is the middleman that renders the React element in the browser. ReactDOM recursively creates nodes depending on their ‘type’ property and updates the DOM.
Virtual DOM and Reconciliation
React maintains a copy of the Real DOM called Virtual DOM which is only a virtual representation of the DOM, this is one of the reasons why React is fast as Real DOM Manipulations are costly.
When we make changes or add data, React creates a new virtual DOM and compares it with the previous one. This comparison algorithm is called diffing, the changes then get batched and the real DOM is updated with minimum changes without repainting the whole DOM.
This process of Creating a Virtual DOM and comparing changes with previous virtual DOM (Diffing) and updating the Browser DOM is called Reconciliation
Rendering Process
There are two phases to the React rendering cycle: The render phase and the commit phase.
1. Rendering Phase
There are also two types of renders. An initial render and a re-render. Initial rendering is when your app first starts up. A re-render is when your state or props have been updated.
Here is how the Flow Looks like for Initial Render
- Components are parsed and then the JSX is converted to React elements using React.createElement and stored in memory
- Virtual DOM is created using the React elements and then sent to the Commit Phase
2. Commit Phase
The commit phase is where React actually manipulates the DOM and makes changes.
Once React has done the comparison between its new and previous Virtual DOM (in case of initial render there is just one Virtual DOM) using the diffing algorithm It will then apply the changes to real DOM using the React DOM library.
How Re-Render works
Re-render is triggered when the state is updated or a prop is changed in the component. The component which triggered the state change gets flagged and it's re-rendered and all child components too are re-rendered.
Here is how the flow looks like for re-render
- Flag the component which triggered the state change.
- That component and its child components are parsed and then the JSX is converted to React elements using React.createElement and stored in memory
- A new virtual DOM is created using the React elements and then compared with the previous virtual DOM through the diffing algorithm
- Changes are sent to the commit phase
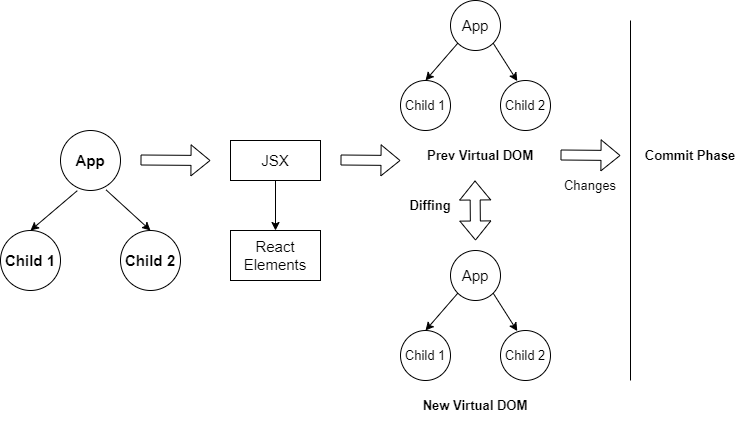
Note: If you update a State Hook to the same value as the current state, React will bail out without rendering the children or firing effects.
React uses the Object.is comparison algorithm, this is the reason why state changes should be immutable.
There are a lot of good resources and articles which explain each concept in depth which I referred to and will share the links below. The reason for writing this article is because I didn’t find many resources which explain the rendering process in detail and this is very important to understand when we wish to optimize the React app performance
I will be discussing a few techniques on How to avoid unnecessary re-renders in my next article.
Build composable web applications
Don’t build web monoliths. Use Bit to create and compose decoupled software components — in your favourite frameworks like React or Node. Build scalable and modular applications with a powerful and enjoyable dev experience.
Bring your team to Bit Cloud to host and collaborate on components together, and speed up, scale, and standardize development as a team. Try composable frontends with a Design System or Micro Frontends, or explore the composable backend with serverside components.
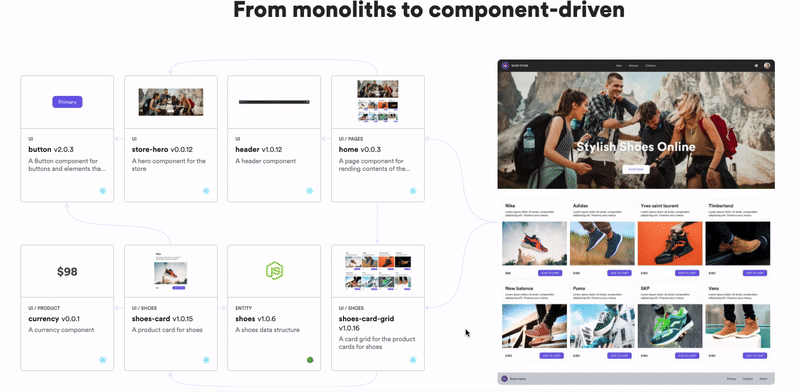
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- The Composable Enterprise: A Guide
- How to build a composable blog
- Meet Component-Driven Content: Applicable, Composable
How React Renders a Component on Screen was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Ashutosh Kumar
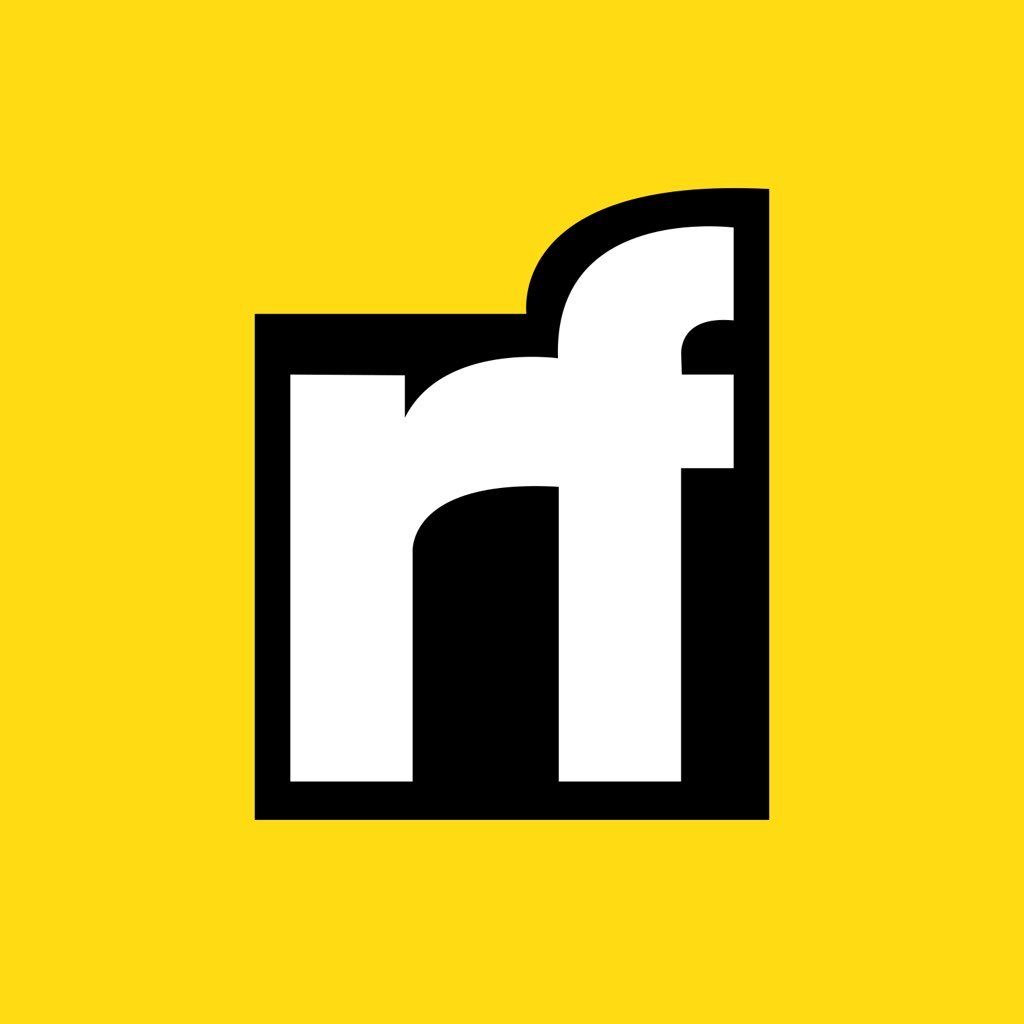
Ashutosh Kumar | Sciencx (2022-05-09T09:59:25+00:00) How React Renders a Component on Screen. Retrieved from https://www.scien.cx/2022/05/09/how-react-renders-a-component-on-screen/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.