This content originally appeared on Level Up Coding - Medium and was authored by Daniil Slobodeniuk
Yo, fellow engineer!✌️ In this article I wanted to tell you how I managed to leverage design patterns in the production and how it wasn’t as smooth as I had thought it would be😮💨
As a side hustle, I work at a start-up Picklang where we were implementing 2 new features — smart feed for our beloved users and word bank. I decided to dump all my knowledge of the patterns and OO design to make it as cleverly designed as possible. Let’s look at what has happened…
Learn languages with the help of AI🤖: https://picklang.ml
❗️Disclaimer: I cannot disclose production code or any sensitive info regarding inner workings, but I will show you the overall concept and guide you how you should tackle similar problems
Structure:
- Intro
- Problem with design
- Issues with my solution
- MVC digression
- Outro: suggestions and references
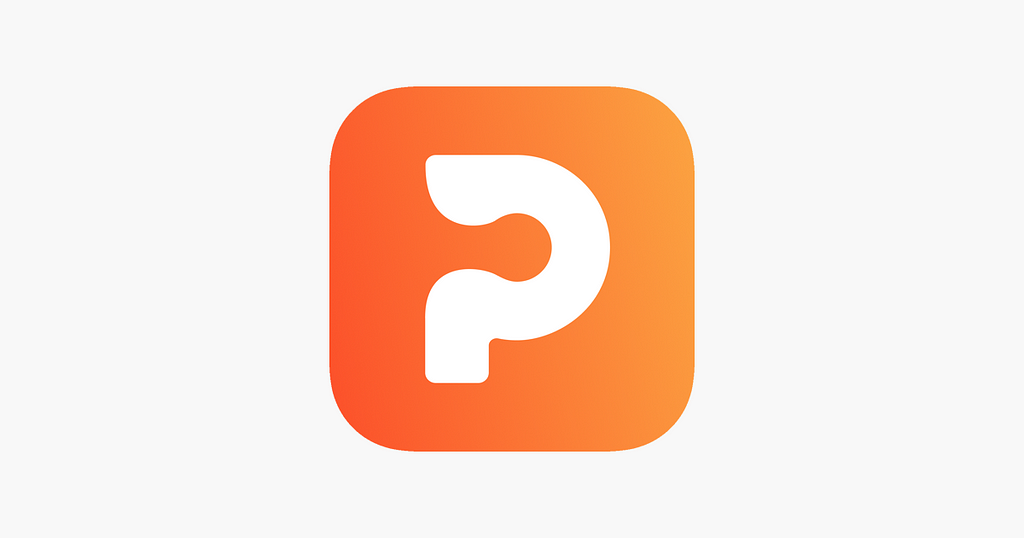
Intro
All we know that OOP is a cool stuff which we want to use in our work/projects to make code with high cohesion, less dependency (and other lofty words). Especially, if you come from Java background.
But what if I tell you that OOP is not always an option, especially if you write in other languages, for example Python🐍.
Stop, what?😱 Yeah, Python, Kotlin allows you to use function-based approach and use it to write less complex code if not such required.
As a wise swe🥷🏼 from Picklang told me: “OOP is good, but it is not always an option which needs to be leveraged. OOP stems from high relation between data and functions. For example, List and HashMap are both classes which have data under them, hence they also posses methods to deal with them. It is better to start with functions and then convert to classes with methods if complexity asks for it”
At first I hadn't accepted such a comment. How could I? I was freshly graduated from O'Reilly Media Design Patterns book. We need OOP and nothing more💪
Alas, I was wrong and some chunks of the code were then rewritten by me after 3 pages of Google document comments and long discussions in Discord.
BTW, read my article about knowledge I have got from Design Patterns book: https://blog.devgenius.io/what-ive-learned-from-design-patterns-book-daniil-slobodeniuk-ea61bd2cd609
Problem with design
As I mentioned in the beginning, there were 2 tasks for me. Those features used some similar data under the hood, that’s why I decided to use patterns to squash duplicate code and look cool🙋🏼♂️
Clever guys designed smart-feed for the users and gave me a bunch of functions which I needed to incorporate in the production⚙️
So, as a cool engineer I wrote design on the whiteboard and got down to my laptop👨💻
Design in the studio🎨:
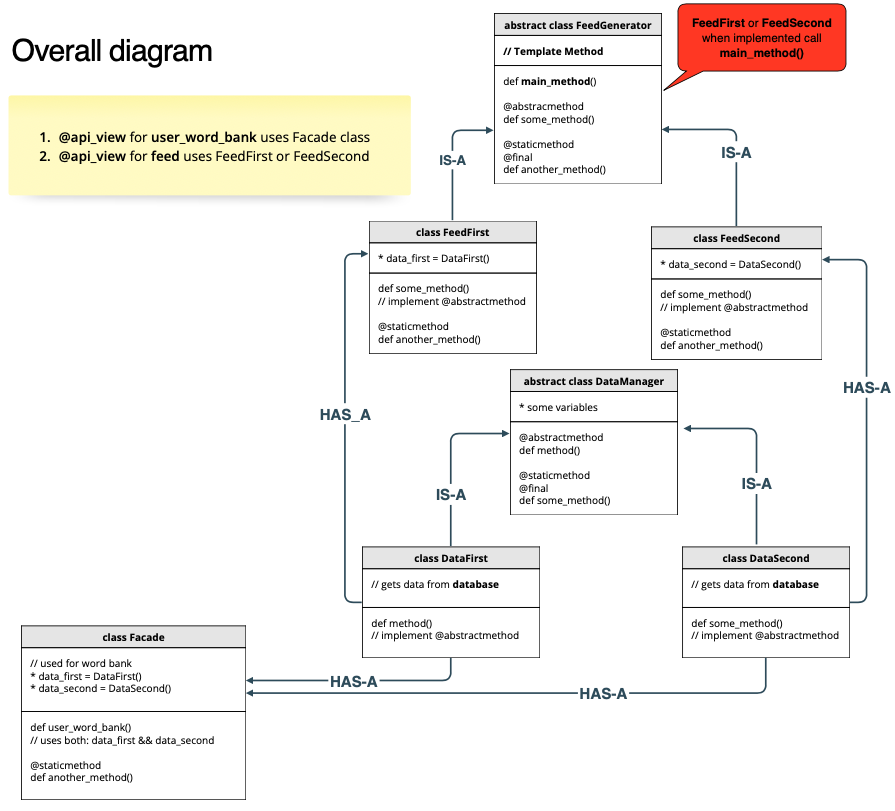
I hope you got the gist from the scheme, but a couple of clarifying points:
There are 2 APIs:
- /feed uses FeedFirst or FeedSecond which in turn calls template method from FeedGenerator . This template method has all the pipeline for the feed. DataFirst and DataSecond are two strategy objects which are placed in FeedFirst and FeedSecond by composition.
- /user_word_bank uses Facade class which encapsulates all the nitty-gritty callings. This class has by composition same classes for extracting data as /feed does
At a first glance everything is stellar✨. And actually, not the whole design was bad. But certain issues arose and hence I re-engineered some code.
Read my articles about Facade pattern and Template Pattern to get the more from current article and enrich your knowledge
Issues with my solution
Let’s name a couple of issues right away👋:
- I messed up totally with MVC (more about it later)
- Some of classes didn’t have any purpose: just a couple of methods and no real data underneath
Solution🙌:
- Decided to keep Template Pattern with those FeedFirst and FeedSecond
- Split those DataFirst and DataSecond into methods which are packed in Python packages where certain modules are responsible for DataFirst and another — DataSecond
- Fixed naming a lot — not direct relation to design as one may consider, but definitely a major issue if you have a large project with lots of packages and complex logic. Believe me, especially, if you write a project that will be a part of something bigger
- Split Facade class into multiple functions as well and put inside module. Yes, Facade can be a plain function or two, not only class.
What is a package in Python — folder. What is a module — separate file inside package
MVC digression
As promised, I will give you a snapshot of MVC.
MVC stands for Model View Controller🤯
View — result on the UI part of the app or, in some cases, final representation of data. For example, if you get data from database and then process it in Pandas DataFrame — can be considered a view.
Model — in plain terms it is database or some other storage that keeps data.
Controller — kind of worker that glues View and Model.
And, ta-dam — MVC is actually a collection of design patterns which are used together. Which patterns and how?
- Look at open-source code of O'Reilly Media Design Patterns book, Download the code link: https://wickedlysmart.com/head-first-design-patterns/ . There you’ll find MVC example in DjView folder
- View is a classic Composite pattern which also has Controller class by leveraging Strategy Pattern
- Model uses Observer pattern to notify View when it has changed
Read my articles about Strategy, Observer, Composite patterns to learn more about them and how MVC actually operates by leveraging them
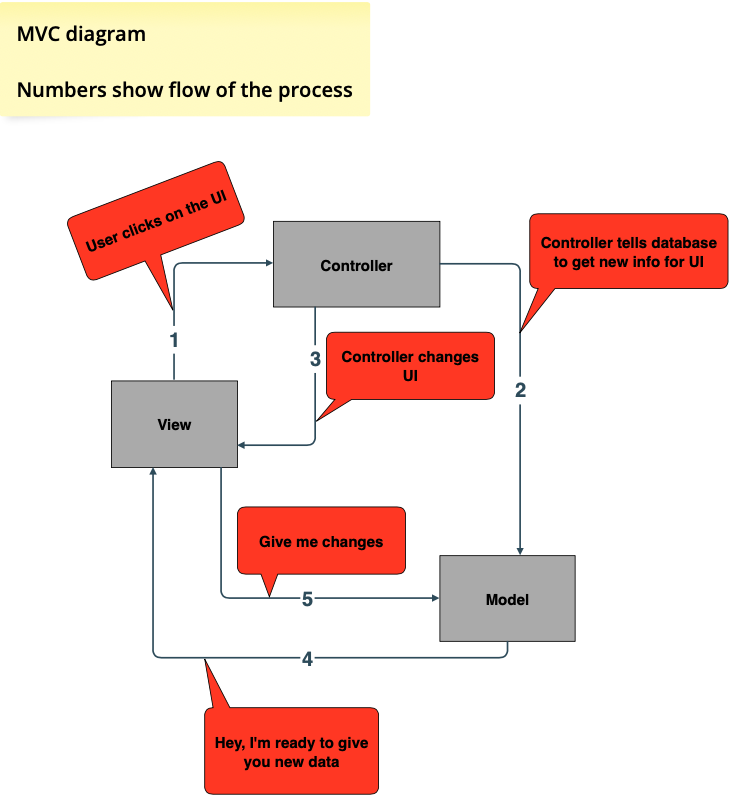
So, my classes connected with data, named DataFirst and DataSecond on the diagram, not only get data from database, but also transforms them into Pandas DataFrame — bad.
Our backend part is written in Django🐲 — it is not a direct MVC framework like Spring, hence some sideways are accepted. But still, if possible — responsibilities should be divided.
❗️Attention:❗️ our solution is not a direct MVC case, but traces of it can be found
Solution for this issue💥:
- Use FeedFirst or FeedSecond as a Controller to transmit call from API call to the database (in our case from @api_view)
- Retrieve data from Model
- Process it in the Controller
- Return raw data to the @api_view and transform it there to Dataframe
Outro😶🌫️
In closing I wanted to make a couple of comments:
- Always think through and draw the desired design as it may help you to spot problems🖋
- Be patient and open to good criticism. How do you plan to grow without proper feedback and mistakes?🎭
- Never stop learning…➿
Links to the guys in the start-up🤙:
- Software engineer/CTO who gave me wise comments and did initial code for the smart feed: https://www.linkedin.com/in/alexey-serdyukov-52624b213/
- Data Scientist/CEO who developed the theoretical part behind the smart feed: https://www.linkedin.com/in/svyatoslav-oreshin/
You can find me✌️:
- LinkedIn: www.linkedin.com/in/sleeplesschallenger
- GitHub: https://github.com/SleeplessChallenger
- Leetcode: https://leetcode.com/SleeplessChallenger/
- Telegram: @SleeplessChallenger
How I implemented design patterns in production was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Daniil Slobodeniuk
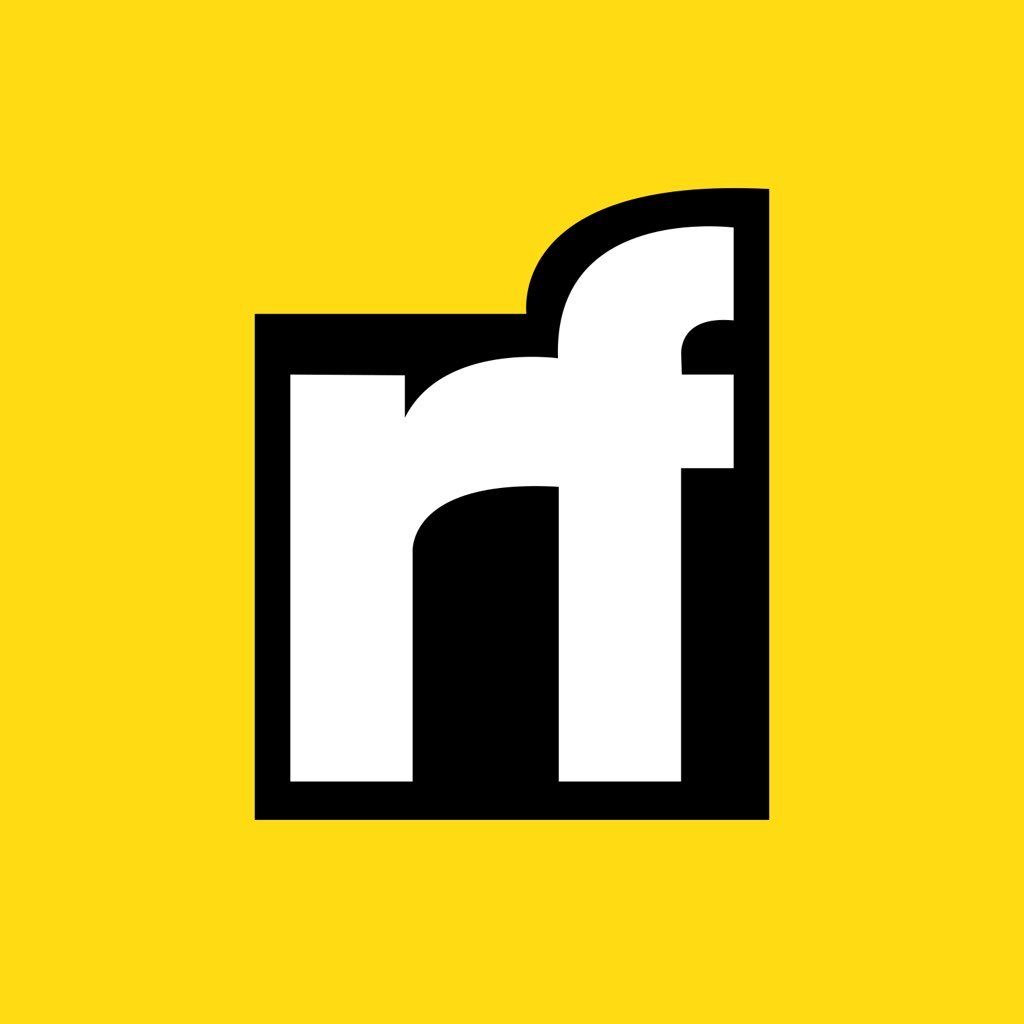
Daniil Slobodeniuk | Sciencx (2022-06-06T13:44:36+00:00) How I implemented design patterns in production. Retrieved from https://www.scien.cx/2022/06/06/how-i-implemented-design-patterns-in-production/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.