This content originally appeared on Bits and Pieces - Medium and was authored by Amit Kumar
State management is one of the most challenging parts of the application. There are tons of state management libraries out there. Understanding how to manage states so effectively that it doesn’t affect your app performance is crucial.
The “secret” behind excellent state management is understanding how your app maps state to the app’s tree structure.
Four mistakes commonly made with state management:
- Thinking Redux is a silver bullet to every state management problem.
- Storing local state in state stores like Redux.
- Storing all or multiple unrelated application states in a single object.
- Storing props passed to the component inside the state.
Give expensive state calculation a boost
Sometimes your initial state value needs to be generated using some expensive calculation or operation, such as from local storage. This little trick can boost the performance of your application by initializing those state values using a lazy initialization technique.
The technique is straightforward when using the `useState` hook; instead of directly initializing the state, pass a function to it that will return the initial value of the state.

It works because creating a function is quicker than directly performing expensive computations. When you pass a function to the `useState` hook, React will only execute the function when it needs the initial value of the state.
Manage asynchronous states like a pro
Managing states when your app is doing asynchronous operations may be a pain. To solve this problem, we can use a set of techniques to handle those complex scenarios very quickly. Check out this quick guide to help manage states in these difficult scenarios.
Manage complex states seamlessly
There are times when your app has complex state values. Handling those complex states becomes super easy with this small technique. It allows you to add your own custom logic to handle complex states in React seamlessly.
The technique is called the state reducer pattern. It is very simple to use. What you need to do is that you use: the `useReducer` hook. This hook allows you to pass a custom state reducer as the first parameter and an initial value as the second parameter. The reducer can use any custom logic to calculate the final state value. The value returned by the reducer will be used as the next state value.

Use these thumb rules for clear state management
- Avoid derived state. It means that you should never set values passed as props to the state. Every value should be either fully controlled (through props) or fully uncontrolled (in the local state).
- Avoid making truly local state global. Ask yourself: If this component was rendered twice, should this interaction reflect in the other parts of the app? If the answer is no, then keep state local only.
- To perform expensive calculations from props with performance, use the `useMemo` hook and pass those props in the list of dependencies.
- Do not store all or unrelated states together in single object. It will make them difficult to manage.
- Prefer using compound components when you need to share some common states in a tree of related components. Check out this guide to build components like a pro.
Go composable: Build apps faster like Lego
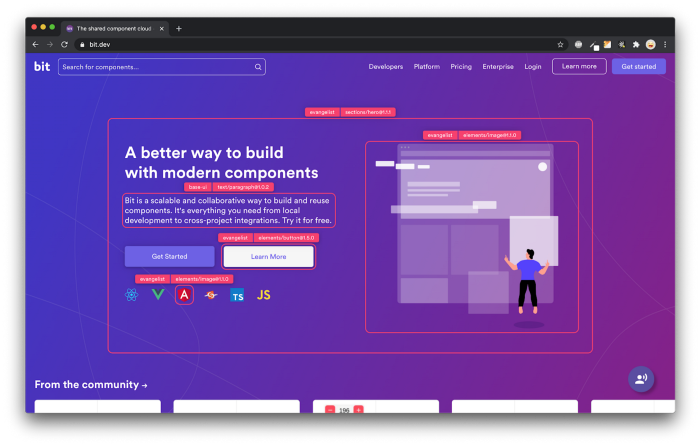
Bit is an open-source tool for building apps in a modular and collaborative way. Go composable to ship faster, more consistently, and easily scale.
Build apps, pages, user-experiences and UIs as standalone components. Use them to compose new apps and experiences faster. Bring any framework and tool into your workflow. Share, reuse, and collaborate to build together.
Help your team with:
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- The Bit Blog
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
How To Manage React States Like A Pro was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Amit Kumar
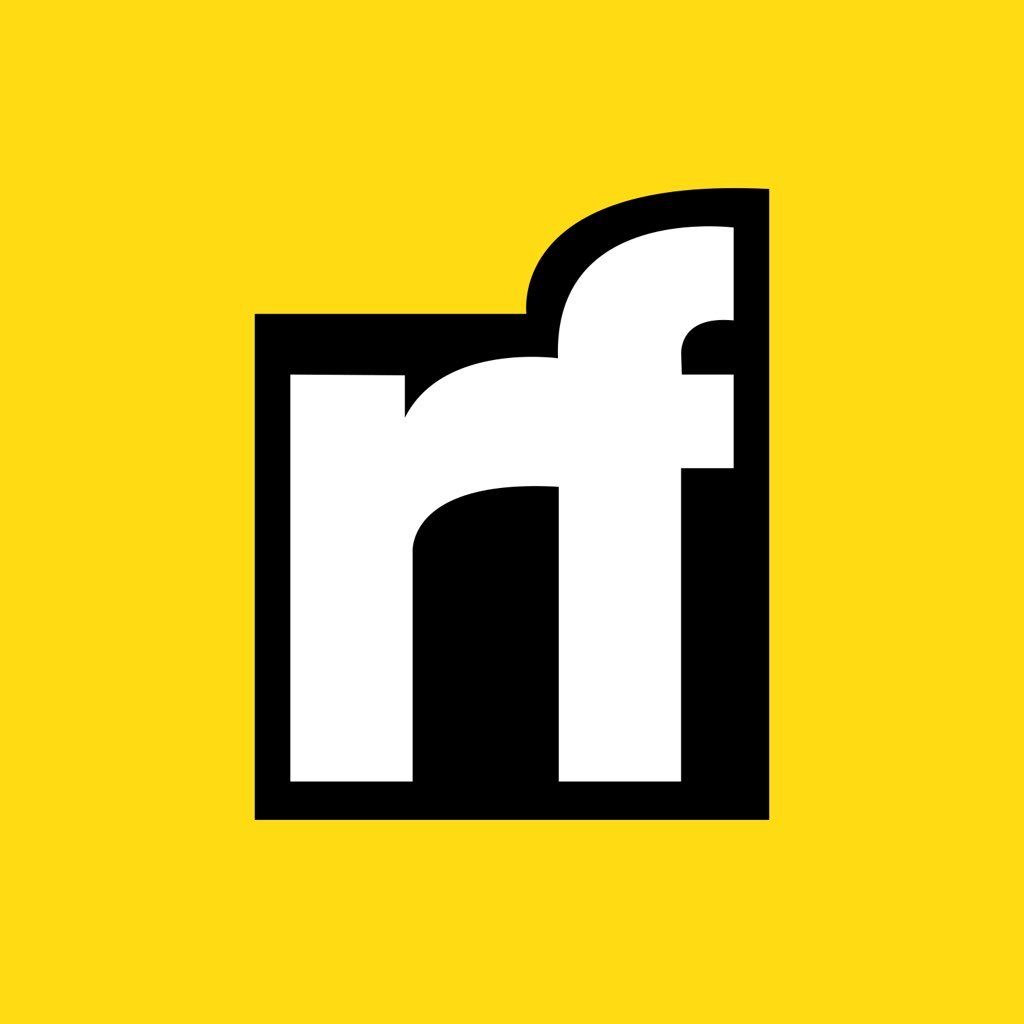
Amit Kumar | Sciencx (2022-09-20T07:42:59+00:00) How To Manage React States Like A Pro. Retrieved from https://www.scien.cx/2022/09/20/how-to-manage-react-states-like-a-pro/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.