This content originally appeared on Level Up Coding - Medium and was authored by Anh T. Dang
For profiling your application
Let's assume that our function is:
def my_func(a, b):
retval = a+b
return retval
Now, we want to measure the time taken by this function. Simply, we add some lines to achieve this.
import time
def my_func(a, b):
start_time = time.time();
retval = a+b
print("the function ends in ", time.time()-start_time, "secs")
return retval
Storing the current time in start_time variable and then I let the method execute and then subtract the start_time from the current time to get the actual method execution time.
Output:
my_func(1, 2)
the function ends in 7.152557373046875e-07 secs
But we can do better using decorations to wrap any function.
from functools import wraps
import time
def timer(func):
@wraps(func)
def wrapper(a, b):
start_time = time.time();
retval = func(a, b)
print("the function ends in ", time.time()-start_time, "secs")
return retval
return wrapper
Now we can use this timer decorator to decorate the my_func function.
@timer
def my_func(a, b):
retval = a+b
return retval
When calling this function, we get the following result.
my_func(1, 2)
the function ends in 1.1920928955078125e-06 secs
What if the function takes more than two variables since we don’t know how many arguments we are going to need?
Simply, we use *args allows us to pass a variable number of non-keyword arguments to a Python function.
def timer(func):
@wraps(func)
def wrapper(*args):
start_time = time.time();
retval = func(*args)
print("the function ends in ", time.time()-start_time, "secs")
return retval
return wrapper
We can add @timer to each of our functions for which we want to measure.
@timer
def my_func(a, b, c):
retval = a+b+c
return retval
Output:
my_func(1,2, 3)
the function ends in 1.6689300537109375e-06 secs
Easy, right?
Using a Decorator to Measure Execution Time of a Function in Python was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anh T. Dang
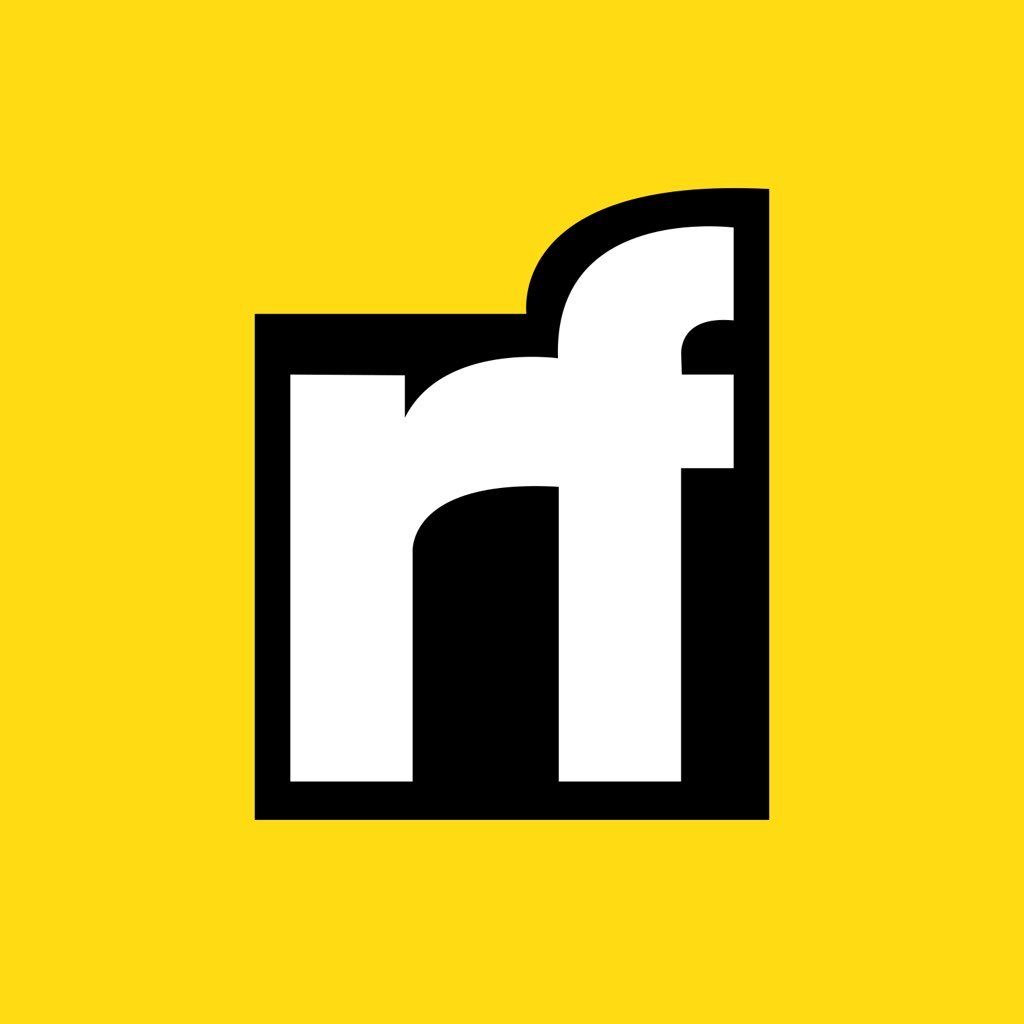
Anh T. Dang | Sciencx (2022-11-14T03:22:48+00:00) Using a Decorator to Measure Execution Time of a Function in Python. Retrieved from https://www.scien.cx/2022/11/14/using-a-decorator-to-measure-execution-time-of-a-function-in-python/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.