This content originally appeared on Level Up Coding - Medium and was authored by Jacob Bennett
Git is a powerful tool that feels great to use when you know how to use it.
I’ve used these features of Git for years across teams and projects. I’m still developing opinions around some workflows (like to squash or not) but the core tooling is powerful and flexible (and scriptable!).
Going through Git logs
Git logs are gross to go through out of the box.
git log is basic
Using git log gives you some information. But it’s extremely high-resolution and not usually what you’re looking for.
git log
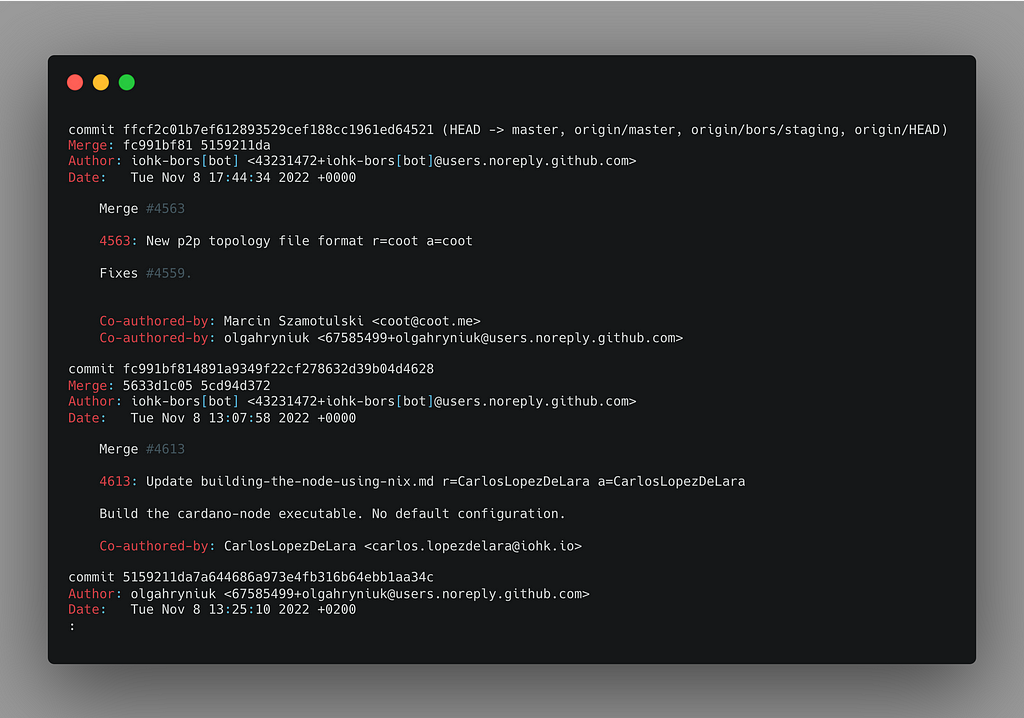
Let’s be real. These logs aren’t impressing anyone. They are boring. And they’re full of information that you don’t really need right now. You’re trying to get a high-level understanding of what has been going on in your project.
There’s a better way.
git log with more visibility
Using --graph and --format we can quickly get a summary view of git commits in our project.
git log --graph --format=format:'%C(bold blue)%h%C(reset) - %C(bold green)(%ar)%C(reset) %C(white)%an%C(reset)%C(bold yellow)%d%C(reset) %C(dim white)- %s%C(reset)' --all
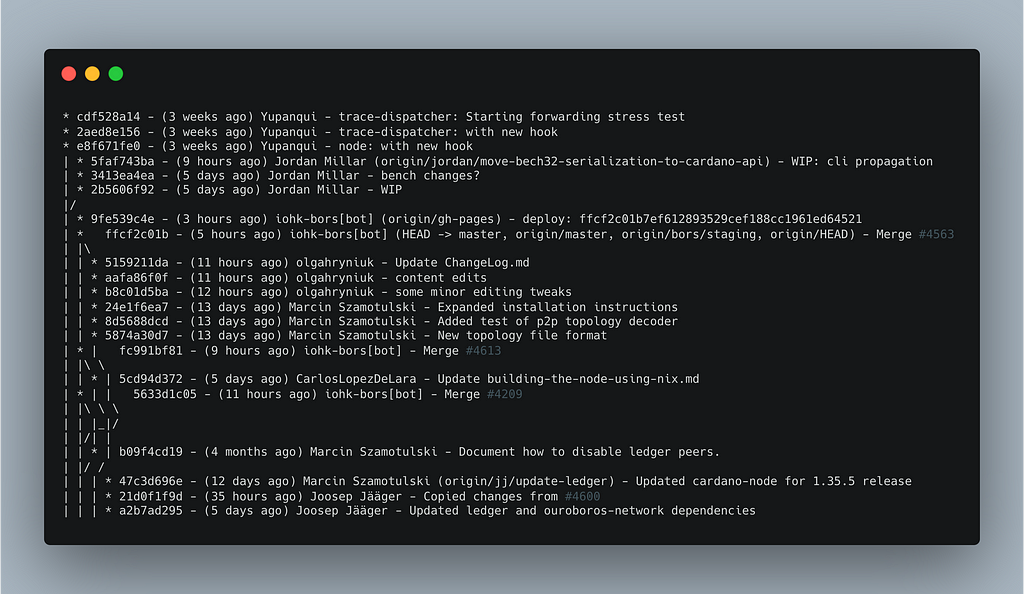
Wow! These are some good-looking logs! There’s even a semblance of a branched tree beside it.
These logs show you who has been working on what, when changes were made, and where your changes fit into the bigger picture.
--graph adds the tree graph to the left. It’s not the most stylish graph, but it helps to visualize changes in the project’s branches. (Read the docs here.)
--format lets you customize the format of your logs. There are preset formats to choose from, or you can write your own format like this example. (Read the docs here.)
--all includes all of the refs, tags, and branches in the logs (including remote branches). You might not want everything so adjust this as you see fit. (Read the docs here.)
See the git-log docs for more information on how you can level up your git logs. →
Understanding a particular commit
You’ll often want to understand what’s happening with a specific commit. git show can show you a high-level view of changes in a commit, but it also lets you see changes to specific files.
View the summary of a commit
git show <commit> --stat
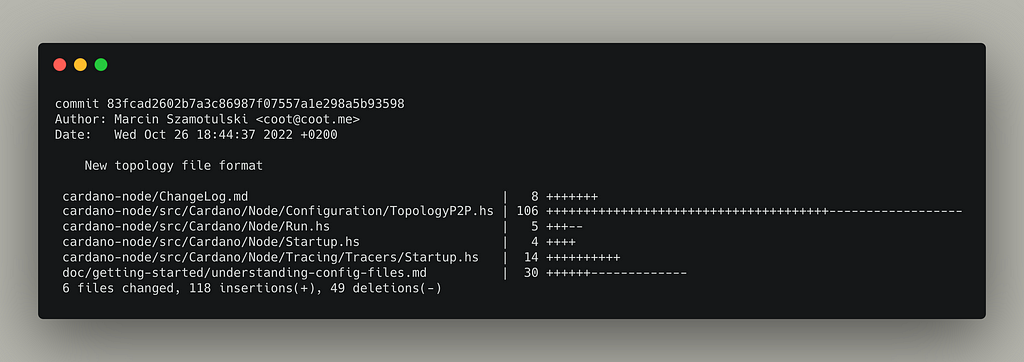
Using the --stat flag you’ll see the commit summary along with the files that changed and details on how they changed.
View specific file changes for a commit
When you want to dive into the specific line changes in a particular file, use git show with the file path.
git show <commit> -- <filepath>
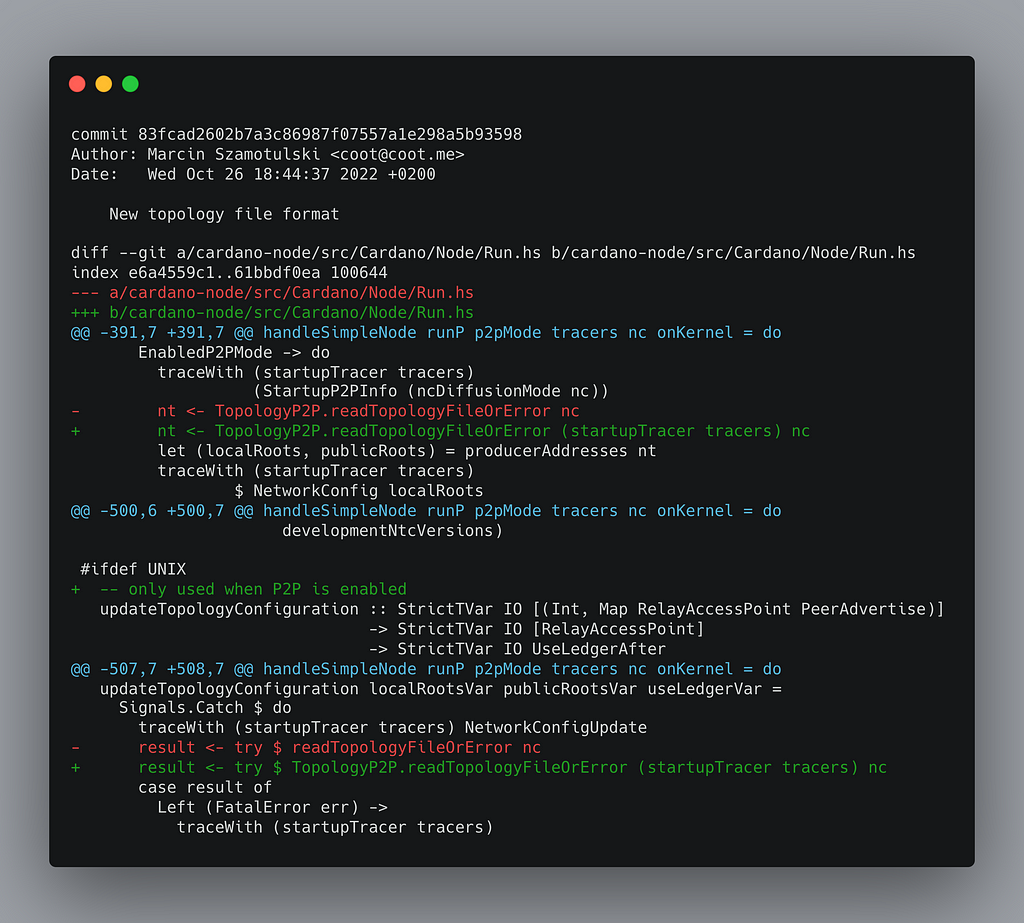
This gives you specific line changes for your file. By default, it will show you line changes along with 3 additional lines on either end to give you the context of where the changed lines are in the file.
See the git-show docs for more information on how you can level up your git commit understanding. →
Making changes
You’ve made a branch on the project, committed some changes to your branch, and you’re ready to merge those changes back into main. Since you branched, another engineer has made changes to the same files. 😱
If you’re using a service like GitHub, your PR will tell you if you have merge conflicts.

Git will prompt you to resolve those merge conflicts before you shove your changes back into main. This is good since you don’t want to stomp out all the hard work others are doing.
To get started resolving this locally you will usually take one of two paths: merge or rebase.
git merge vs git rebase
When there are changes on the main branch that you want to incorporate into your branch, you can either merge the changes in or rebase your branch from a different point.
merge takes the changes from one branch and merges them into another branch in one merge commit.
git merge origin/main your-branch
rebase adjusts the point at which a branch actually branched off (i.e. moves the branch to a new starting point from the base branch).
git rebase origin/main your-branch
Generally, you’ll use rebase when there are changes in an upstream branch (like main) that you want to include in your branch. You’ll use merge when there are changes in a branch that you want to put back into main.
To squash or not to squash
I used to be pro-squash. But an article by Dr. Derek Austin 🥳 changed my opinion on this. I recommend the article and don’t think I have anything useful to add beyond what he said.
Why I Prefer Regular Merge Commits Over Squash Commits
Resources
- git-log documentation
- git-show documentation
- When do you use Git rebase instead of Git merge? (Stack Overflow)
Use Git like a senior engineer was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Jacob Bennett
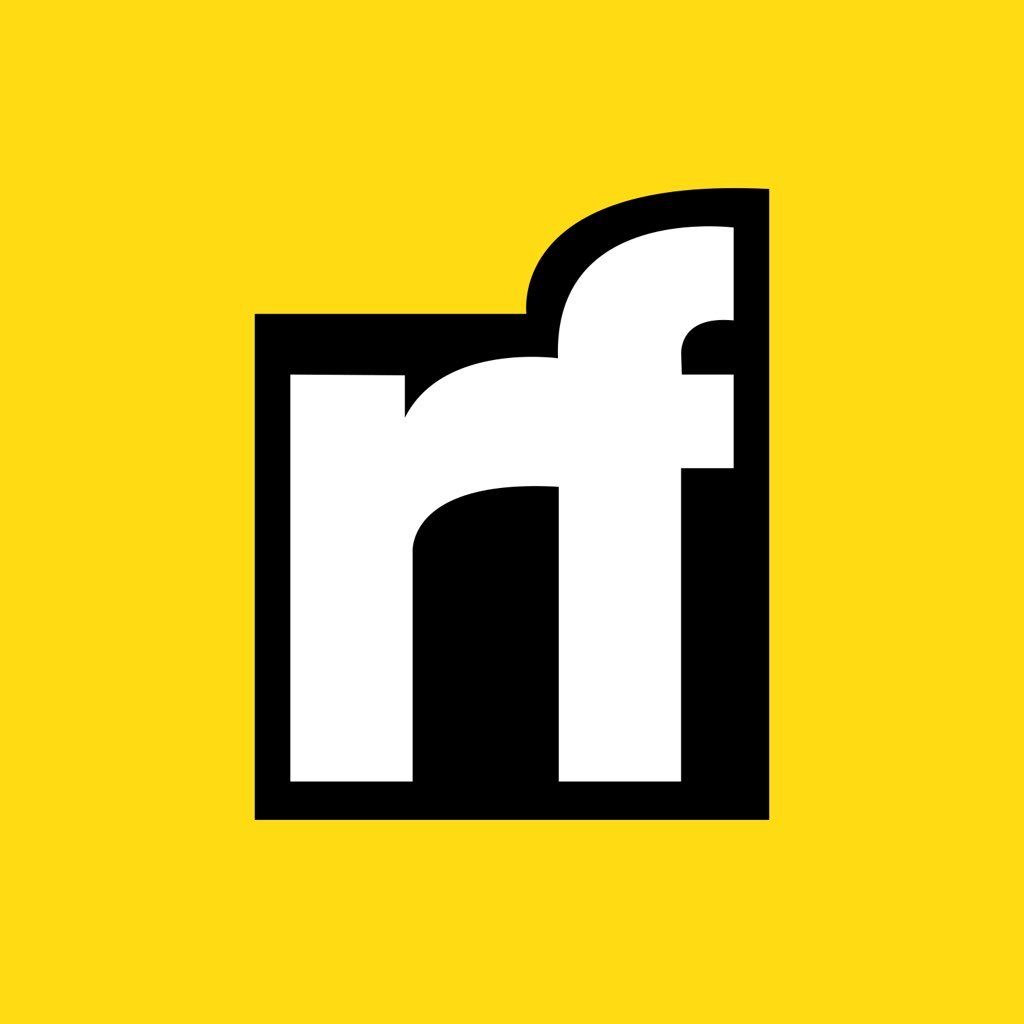
Jacob Bennett | Sciencx (2022-11-15T03:28:58+00:00) Use Git like a senior engineer. Retrieved from https://www.scien.cx/2022/11/15/use-git-like-a-senior-engineer/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.