This content originally appeared on Level Up Coding - Medium and was authored by Umut Arpat
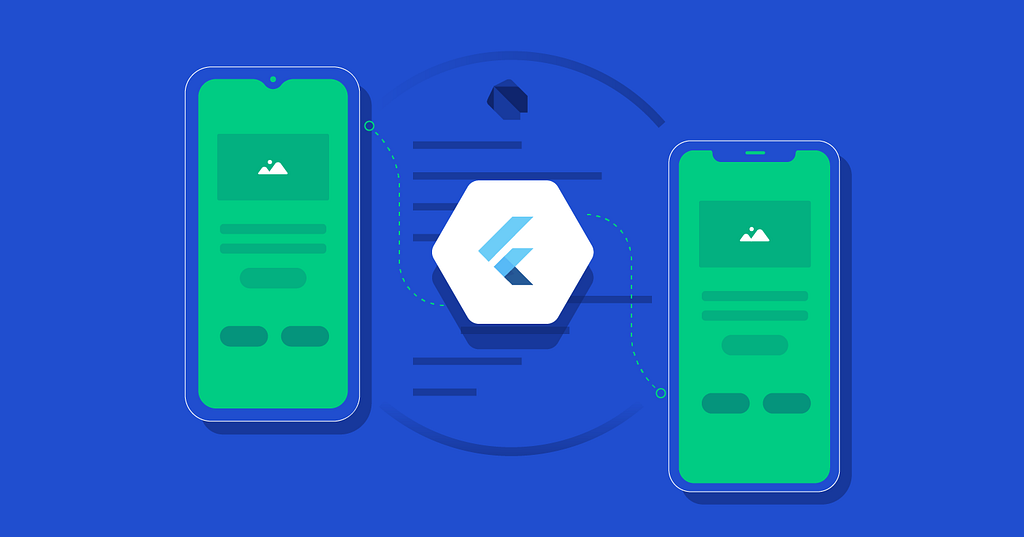
Welcome to my Flutter tutorial for writing Kotlin and Swift code for native integration.
The below content is for a native example written both in Kotlin and Swift for retrieving the device’s name and battery level.
I used GetX as state management here (which I don’t recommend anymore. Another topic for another day) but you can use any state management you want for your own implementation.
Why do we need to write native code?
As you might know, we have https://pub.dev/ for an enormous amount of Flutter packages which support us in our daily Flutter Developer life such as camera, permission handling, device info, etc.
But what if we didn’t have any native programming language experience and we didn’t have any packages like getting device info, handling permissions or being able to call the camera?
Well of course you would be fired instantly and replaced by someone who worked on Kotlin or Swift in the past :)
Thank god we have pub.dev for not making Flutter Devs unemployed.
Career
When I was interviewing for Flutter jobs, 6 out of 10 companies asked me for Kotlin knowledge. Of course, I am not saying if you don’t know Kotlin you can’t get a job in Flutter. Which would be a lie for now.
But if you know Kotlin or Swift you can really get far in the race.
Writing custom integrations or packages
If your company uses custom third party packages you may need native code knowledge for integration.
If your company wants you to implement new features for device’s platform and if there isn’t any package for that in pub.dev. You need native code knowledge for able to do that.
Lastly, it doesn’t hurt to learn something new and if you are thinking Flutter long term like me you should learn Kotlin or Swift.
Code
First we are going to create a View and a Controller. I am only going to retrieve device name and battery level as an example (note that if you are using simulator you can’t retrieve battery level as you need a real device for that)
View
It’s just a simple view where I use GetX controller to put values into UI as Text widgets.
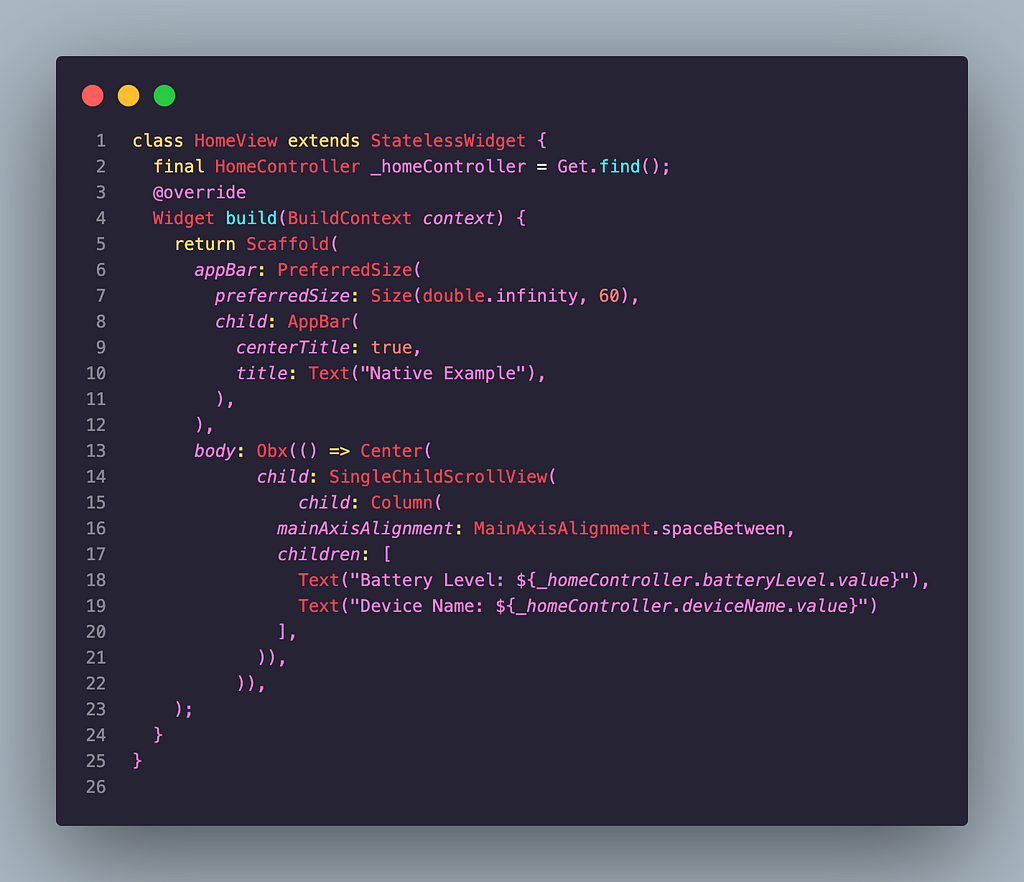
Controller
Here we have our controller for handling cross platform channel invocation.
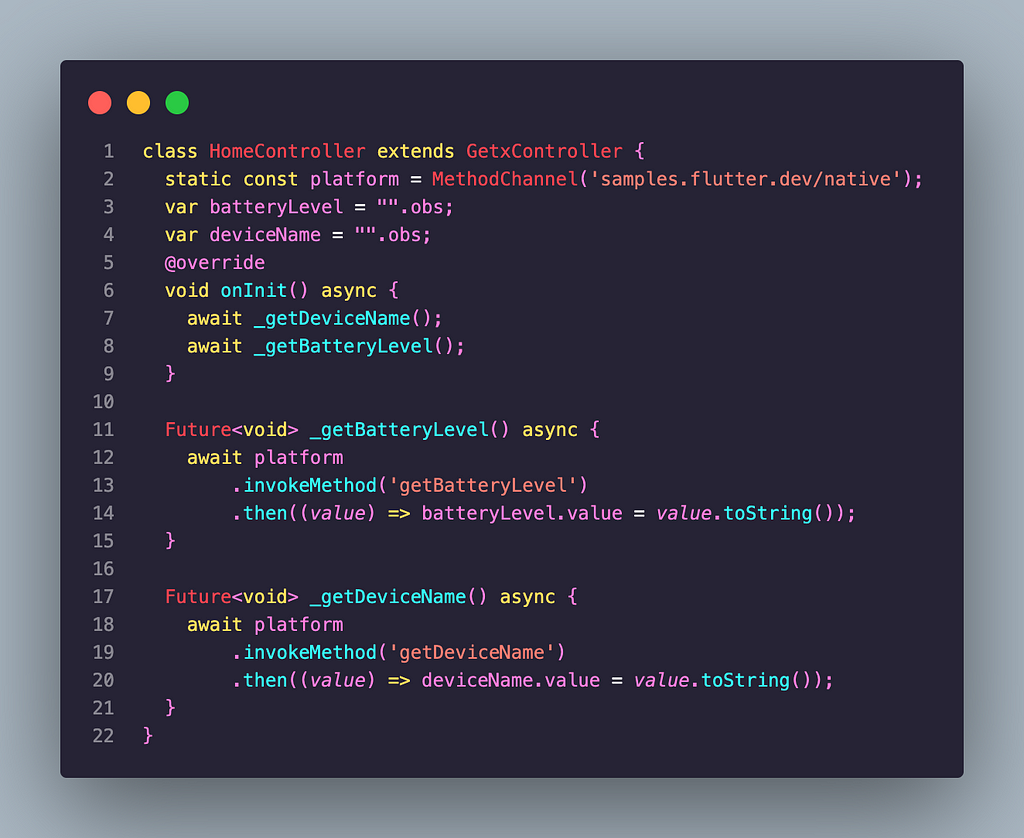
First we need to define a MethodChannel variable with a given name like:
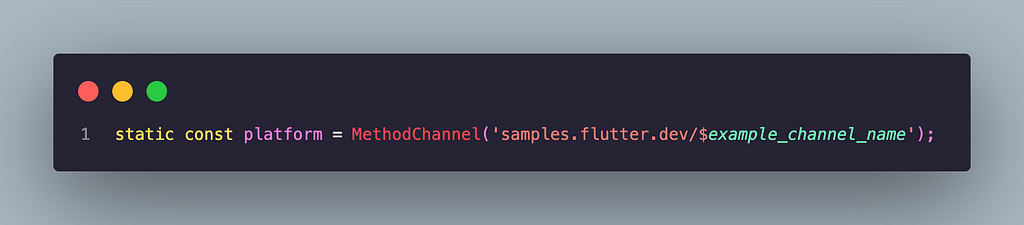
Then I am going to create two functions called getBatteryLevel and getDeviceName which they will be get called at the initial start with onInit lifecycle method.
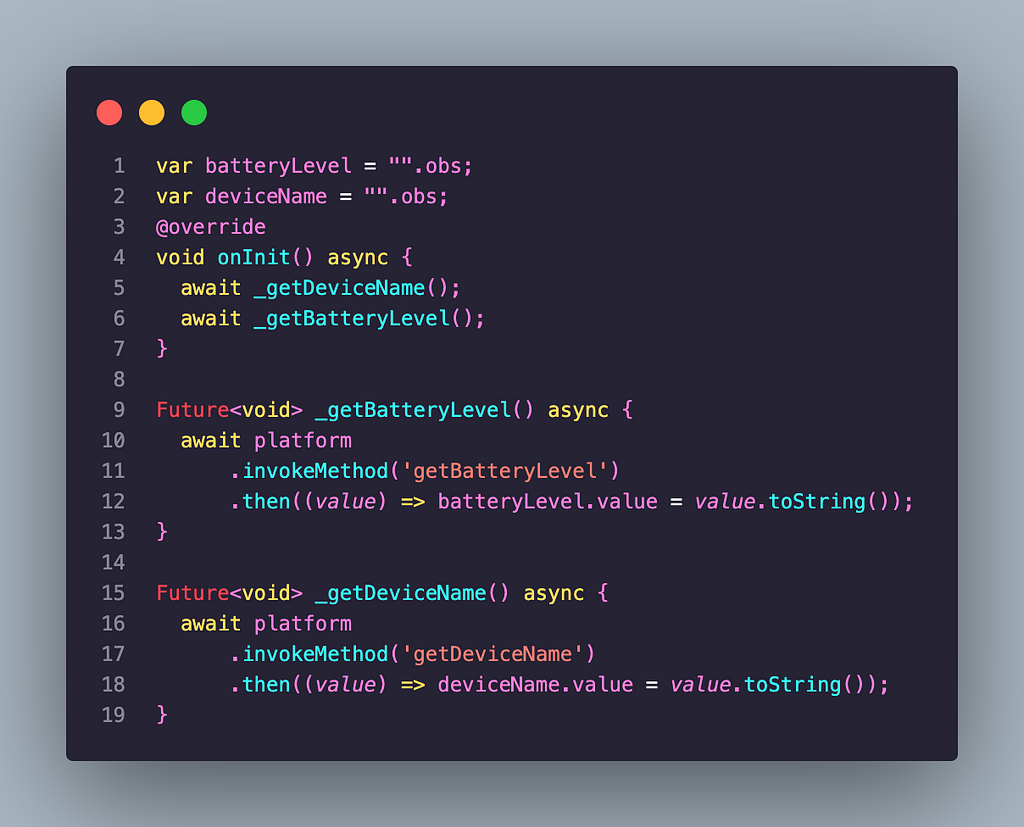
When two methods are going to get called onInit. It will invoke platform based functions with example_channel_name (ours is ‘native’)
Kotlin
In ‘android/app/src/main/kotlin/com/example/flutter_native/’ path we are going to find ‘MainActivity.kt’ file to write our custom Kotlin code.

First things first; CHANNEL variable at the top should be same as our channel name in our dart code for integration.
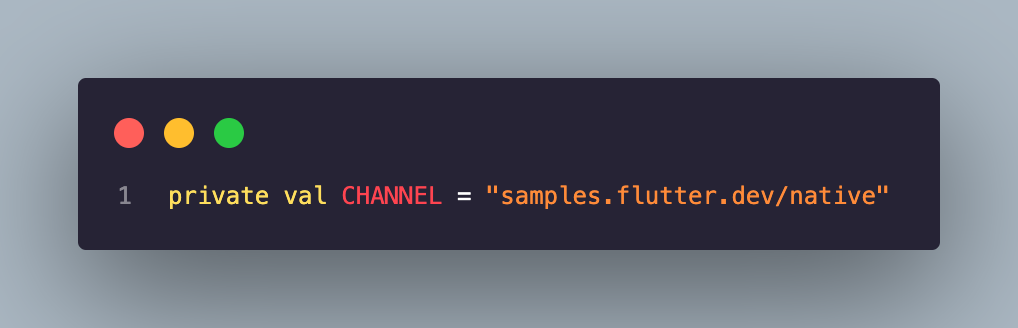
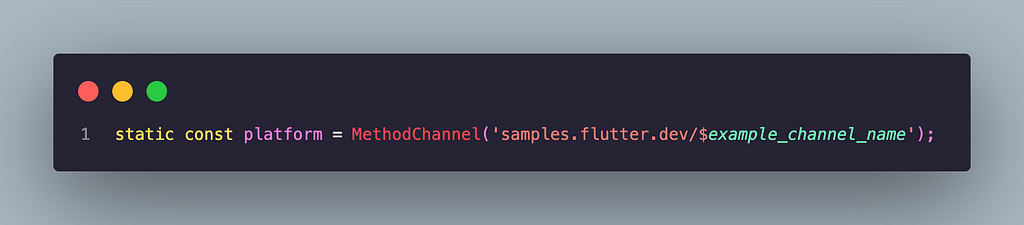
Then we are going to create our configureFlutterEngine function.
This will allow us to handle method invocations from our dart code and do business logic.
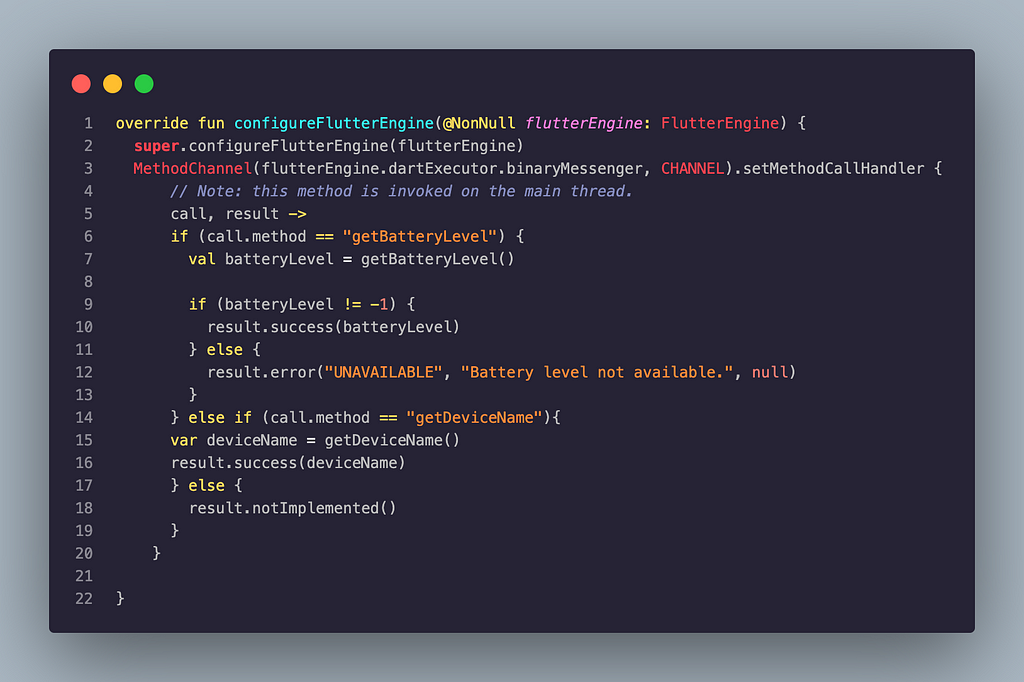
As you can see we are checking method names such as ‘getBatteryLevel’ for our business logic implementation. We did give a name for invoke method earlier in our controller.
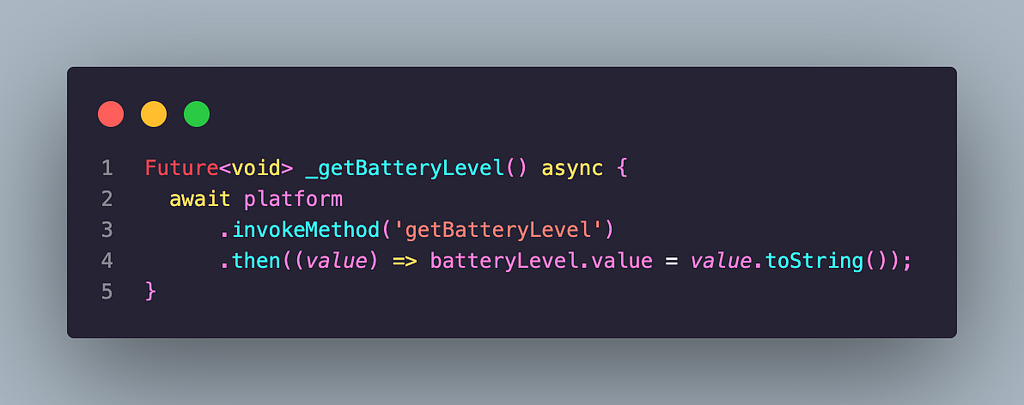
Functions for getting battery level and Device name
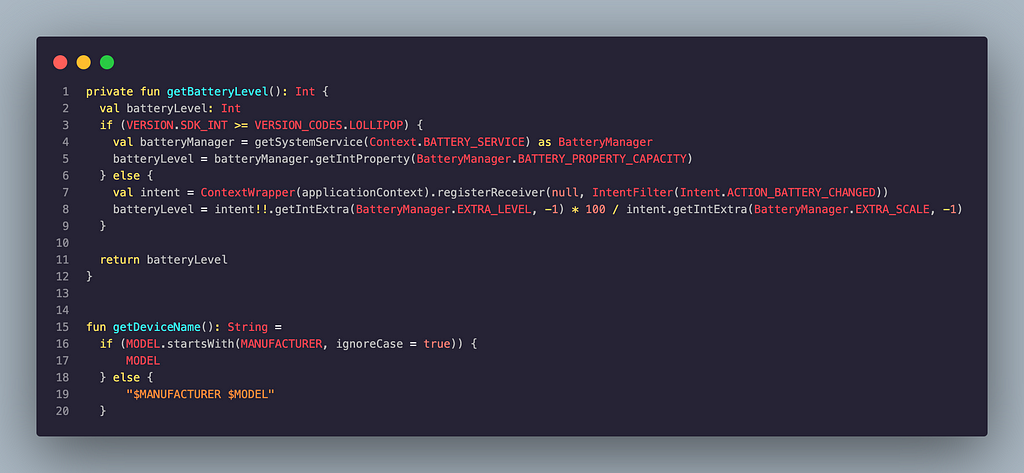
Swift
It has a very similar implementation for native code handling just like Kotlin.
In ‘ios/Runner/’ path we are going to find ‘AppDelegate.swift’ file to write our custom Swift code.
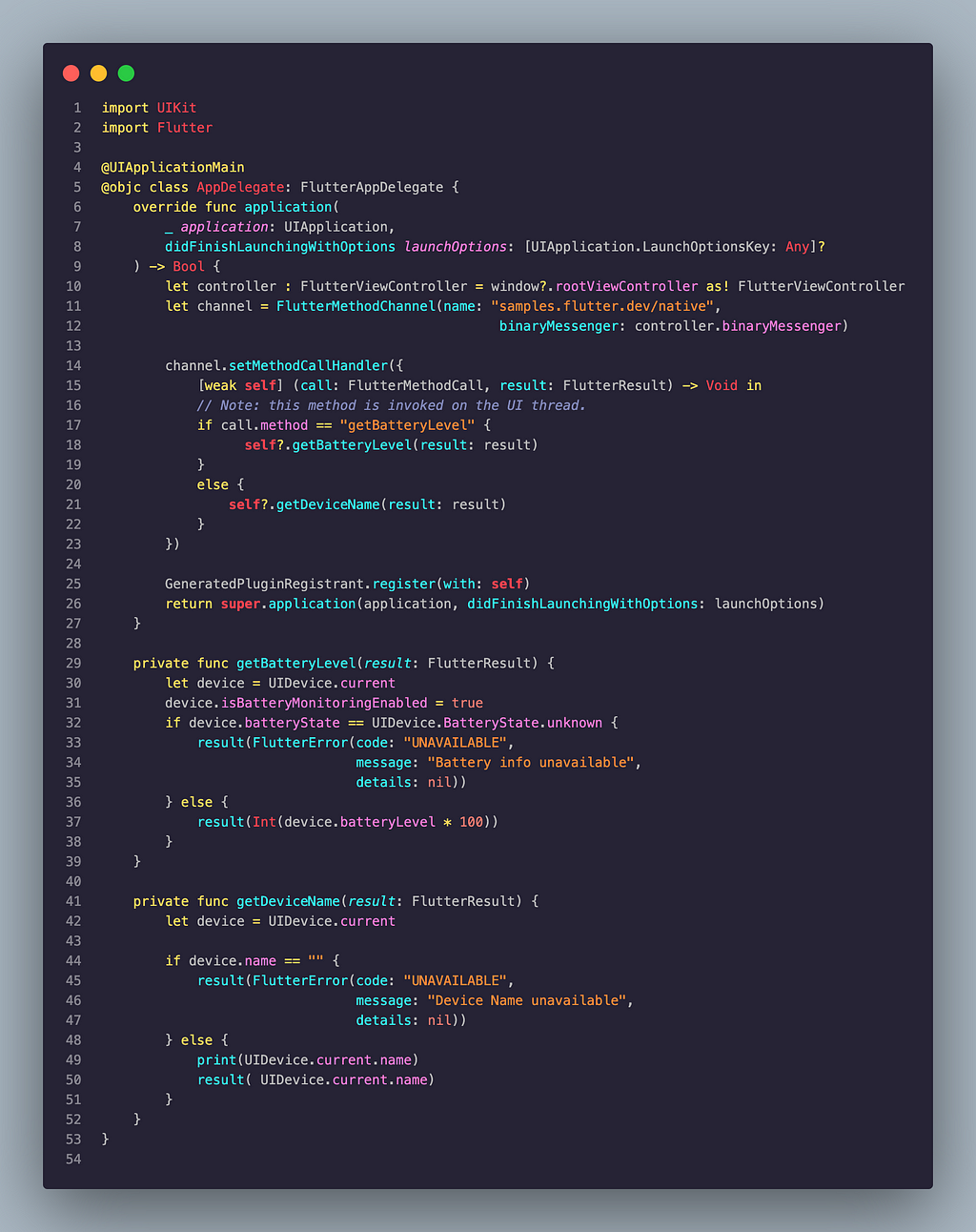
Inside application function we are going to write our method handling
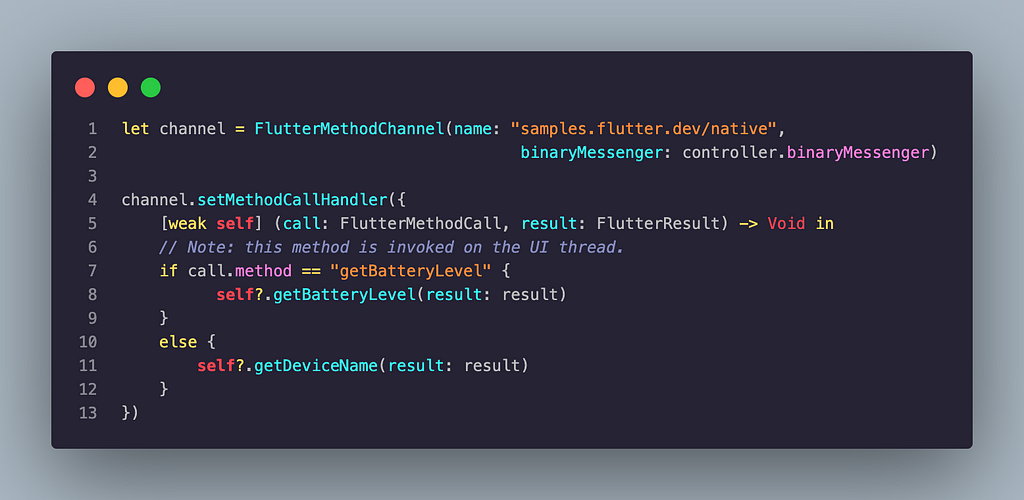
Functions for getting battery level and Device name:
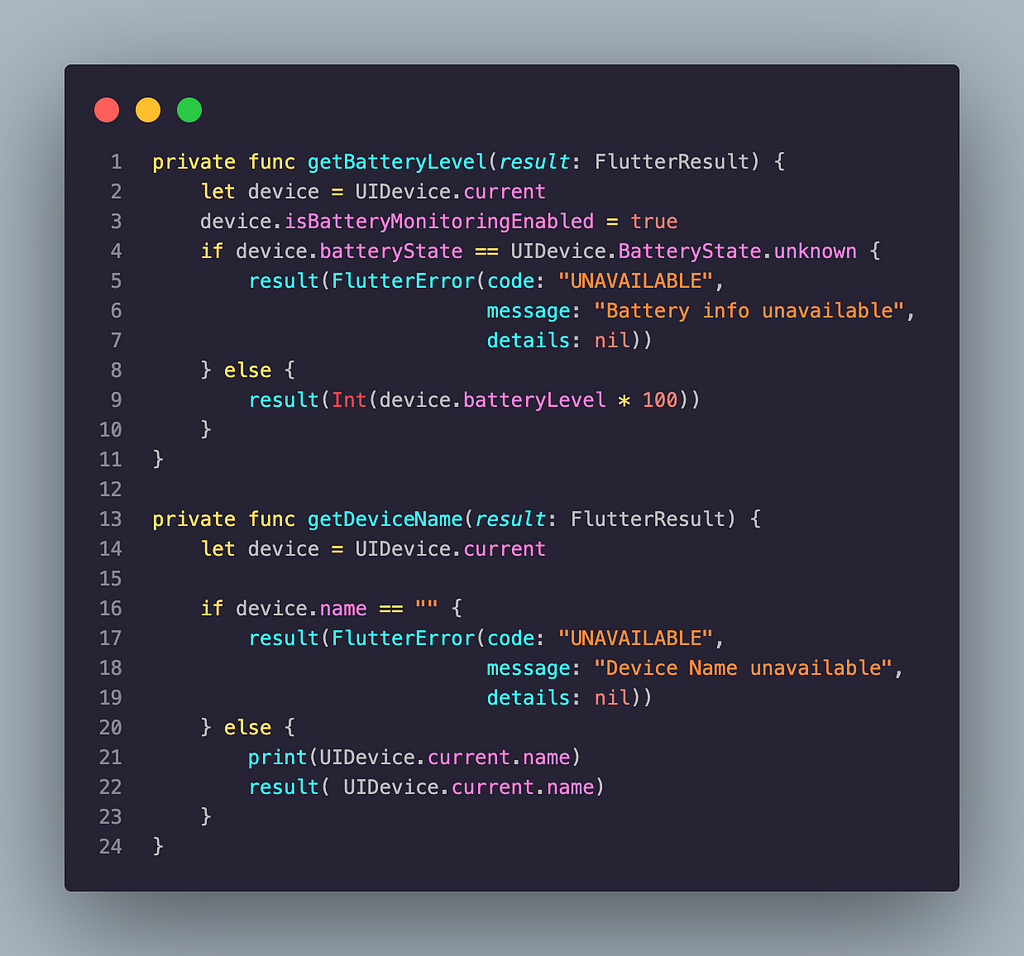
Thank you for reading.
You can find related repository here:
https://github.com/umutarpat/flutter_native
Here is Why and How You Can Write Native Code in Flutter for Both Kotlin and Swift was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Umut Arpat
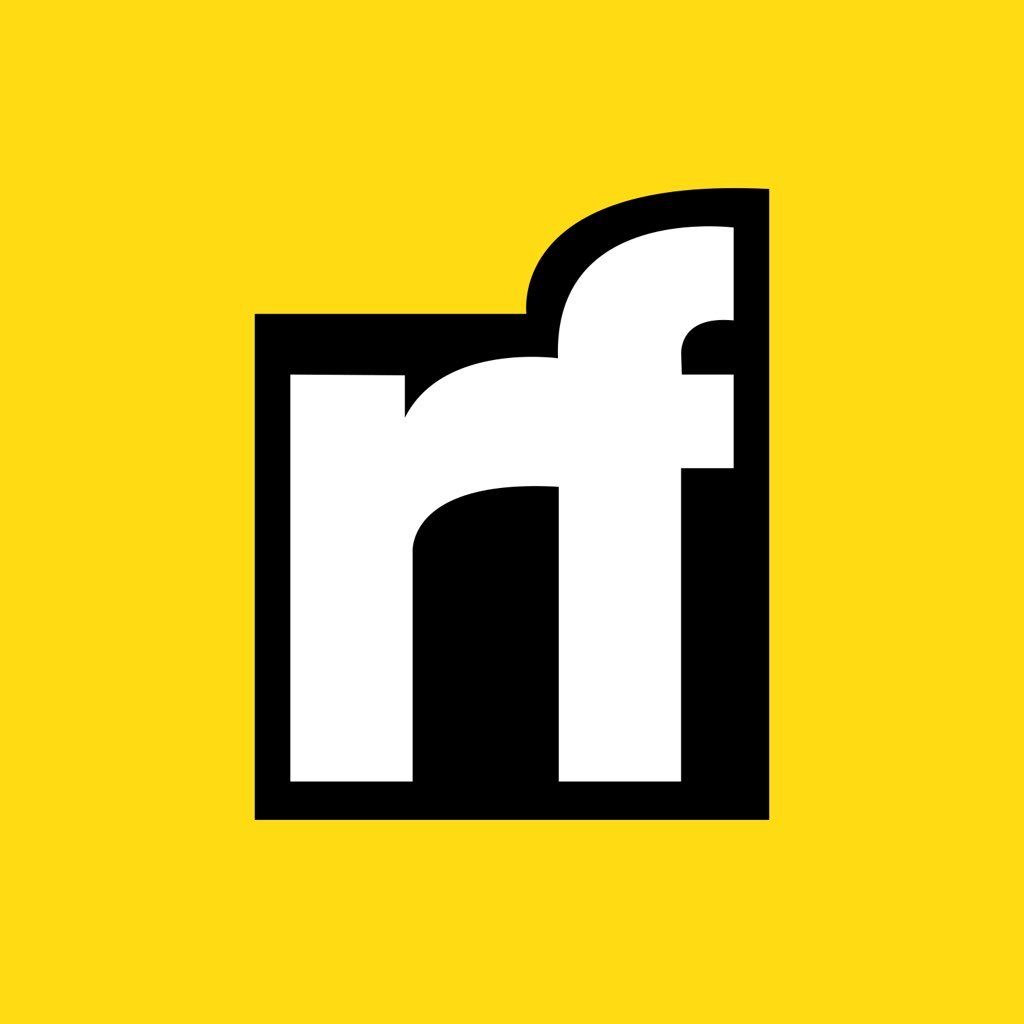
Umut Arpat | Sciencx (2022-11-16T03:08:00+00:00) Here is Why and How You Can Write Native Code in Flutter for Both Kotlin and Swift. Retrieved from https://www.scien.cx/2022/11/16/here-is-why-and-how-you-can-write-native-code-in-flutter-for-both-kotlin-and-swift/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.