This content originally appeared on DEV Community 👩💻👨💻 and was authored by Isabelle M.
Considering that Python list is one of the most used data structures, it's a given that at some point the need to check if a list is empty will arise.
There are several ways to do this, however, the most straightforward is to use a method called Truth Value Testing.
In Python, any object can be tested for truth value, including lists. In this context, lists are considered truthy if they are non-empty, meaning that at least one argument was passed to the list. So, if you want to check if a list is empty, you can simply use the not
operator.
empty_list = []
non_empty_list = [1, 2, 3]
not empty_list # True
not non_empty_list # False
The not
operator is a logical operator that returns the opposite of the value it is applied to. In the above code example we can see that it returns True
if the list is empty and False
if the list is not empty.
While the not
operator is the most straightforward and pythonic way to check if a list is empty, there are other ways to do this. For example, you can use the len()
function. This function returns the length of the list, which is 0 if the list is empty.
empty_list = []
non_empty_list = [1, 2, 3]
len(empty_list) == 0 # True
len(non_empty_list) == 0 # False
Another way is to compare the list to an empty list using the ==
operator.
empty_list = []
non_empty_list = [1, 2, 3]
empty_list == [] # True
non_empty_list == [] # False
This content originally appeared on DEV Community 👩💻👨💻 and was authored by Isabelle M.
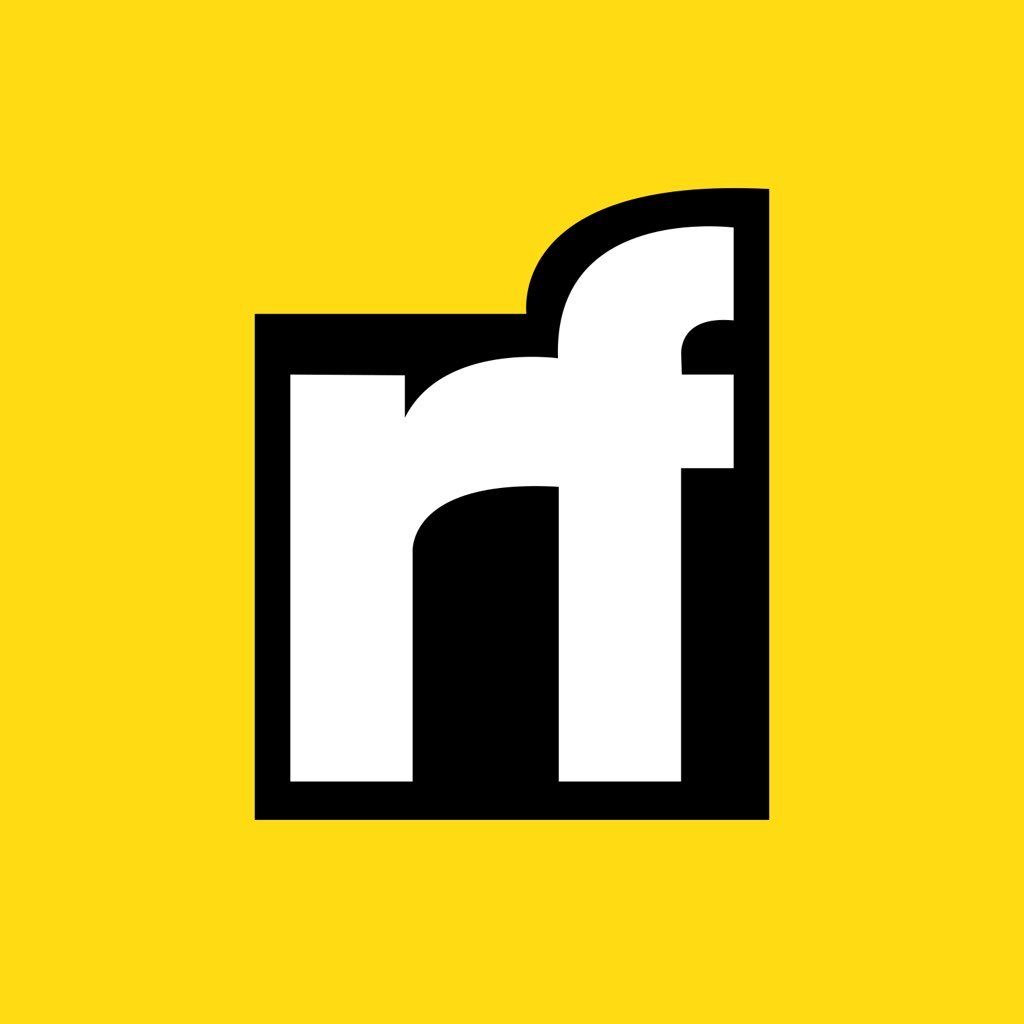
Isabelle M. | Sciencx (2022-12-11T10:00:00+00:00) How to check if a list is empty in Python?. Retrieved from https://www.scien.cx/2022/12/11/how-to-check-if-a-list-is-empty-in-python/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.