This content originally appeared on DEV Community 👩💻👨💻 and was authored by JS
The Issue I need to solve
Simply put, I need to check if a node package is installed in runtime. The reason is that I’m building a new toolkit ZenStack, for building secure CRUD apps with Next.js + Typescript. Since it is built above Prisma, when running the CLI provided by ZenStack, it must check whether Prisma has already been installed. Otherwise, it would prompt the user to install Prisma first.
Get the answer
Let’s try to ask ChatGPT directly:
Cool! The answer looks compelling. Problem solved!
The side effect
It does work, but it brings one side effect. In my CLI project, I use async-exit-hook to handle uncaught errors like the below:
// You can hook uncaught errors with uncaughtExceptionHandler(), consequently adding
// async support to uncaught errors (normally uncaught errors result in a synchronous exit).
exitHook.uncaughtExceptionHandler(err => {
console.error(err);
});
After the require(’prisma’)
is executed successfully, the uncaughtExceptionHandler
would never be triggered.
It looks like some code is running when Prisma is imported. I’m not sure whether Prisma provides any flag to control it; even if it does, it still doesn’t sound like a clean solution to me because it is like Pandora's box. You won’t know what gets executed.
Clean solution
The most direct way to avoid a side effect is to use a separate process. So how would you do that if the job is assigned to you? I will use the npm command. So let’s ask the omniscient again:
This looks like a clean solution to me.
Push harder
As good developers, we should always try to think further. What if the npm command is not installed or is broken? If we know how npm finds the module, we could do that ourselves.
Let’s ask again:
As the npm command obviously won’t execute any code for the package, it does find the name and version as the answer specified:
It will display the names and versions of all the installed package
So, where does it get the version information? Of course, from package.json file. Actually, you can easily verify it by creating dummy-package
folder under node_modules
, and then creating the package.json
file with the below content:
{
"name": "dummy-package",
"version": "9.9.9"
}
Then after running npm list --depth=0 dummy-package
, you could see the package info:
helloworld@1.0.0 /Users/jiasheng/branch/helloworld
└── dummy-package@9.9.9 extraneous
Therefore, instead of requiring the module like the original solution, we could change it to require the package.json file like:
const prisma:any = require('prisma/package.json')
Not only does it get rid of the side effect, but also you could get more information for that package, like version, etc.
Final Words
Anyway, you can see ChatGPT really could help us a lot, even writing the code for us, but it is we who actually think and resolve the issue thoroughly. Coming to the thought that AI would replace developers, I think Google should worry about that rather than us. 😉
This content originally appeared on DEV Community 👩💻👨💻 and was authored by JS
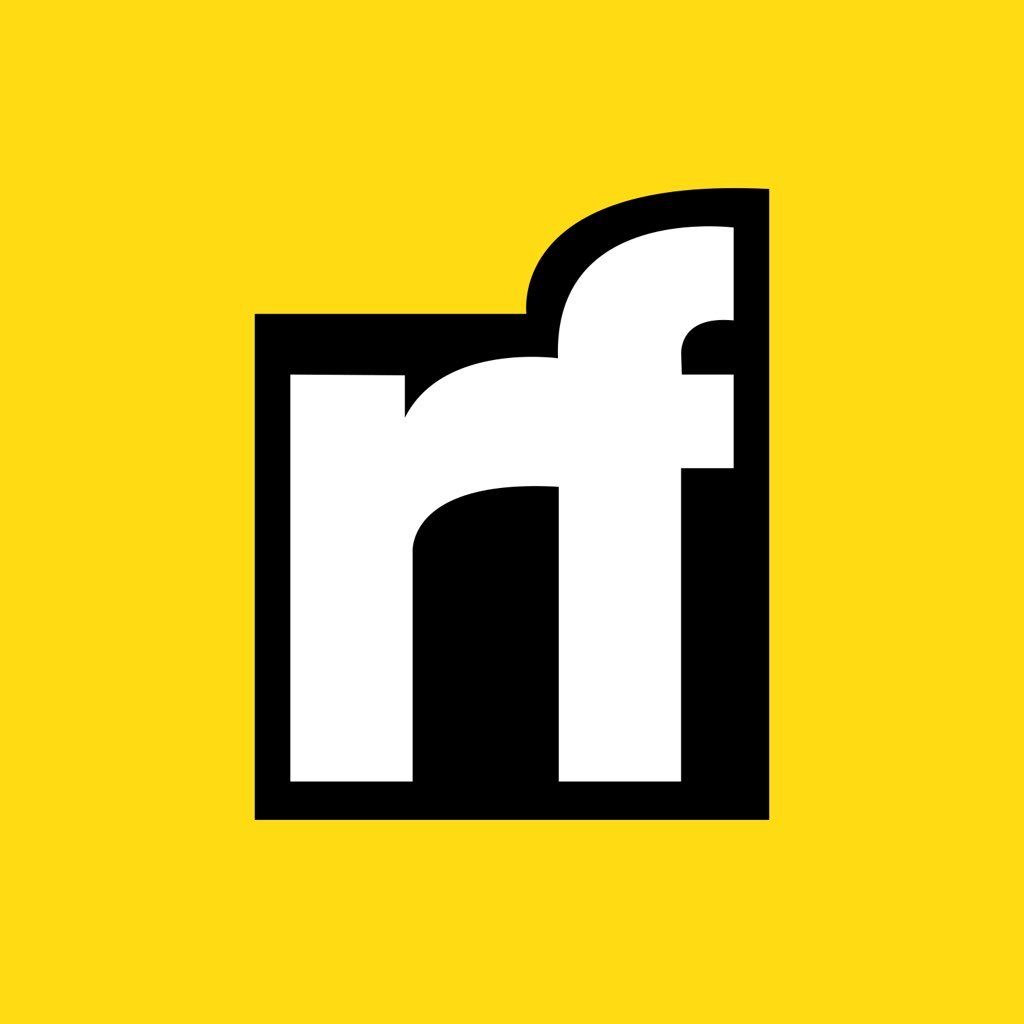
JS | Sciencx (2022-12-27T11:43:48+00:00) How to solve coding issues using ChatGPT. Retrieved from https://www.scien.cx/2022/12/27/how-to-solve-coding-issues-using-chatgpt/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.