This content originally appeared on Level Up Coding - Medium and was authored by Omkar Nath Mandal
Too much concrete is troublesome.
This is the fourth blog in the Software Design Pattern series. Let’s continue to dive deep into another complex pattern. This will be a build-up and we will cover the actual pattern in the next blog 😎 . You might be wondering why a build-up requires another blog in itself. Well, it's because I will try to explain the pre-requisite in depth so that we will understand the actual pattern correctly.
Let’s talk about the ‘new’ keyword
The ‘new’ keyword is fundamental to any object-oriented language and when I say object-oriented, I am particular to languages like Java and C++. JavaScript also has a concept of ‘this’ but it’s not object-oriented. By the way, You can kill me in the comments for saying JavaScript is not object-oriented.👻
In our previous blogs, we have stressed one of the design principles which is to program to an interface and not to an implementation.
Okay okay enough, but what’s the issue with the ‘new’ keyword. ‘new’ is the only way to create an object right? What’s the problem with that?
Well, there is no problem. But if you think closely, whenever we do, Object obj=new myObj(), we are indeed talking concrete here. Whenever there is a change from the Product or Management, the developer has to revisit this code and will probably need to re-write or modify some of the existing parts, thus violating “Code should be open for extension but closed for modification”.
Let’s take an example to fully understand what’s the issue with this. I think we have overused the pizza example which is the de-facto in the Headfirst pattern book. Also, I am on a diet so we will not talk about Pizza. I hope you can understand. 🥺
I was browsing my food delivery app and found an outlet that sells rolls from where I like to order on my cheat days. Let’s call them “RollingTaste”. We will try to de-mystify their code logic. Let’s implement a very basic class for ‘RollingTaste’.
public class RollingTaste {
Roll orderRole(string type){
Roll roll;
if(type.equals('chicken')){
roll = new ChickenRoll();
}
else if(type.equals('egg')){
roll = new EggRoll();
}
else if(type.equals('veg')){
roll = new VegRoll();
}
roll.prepare();
roll.pack();
return roll;
}
}
At first glance it seems there is nothing wrong with this code and that is true there is nothing wrong with the code but it is not perfect either. There is always scope for improvement.
We can see that there is a particular part of code that is implementation heavy and depends on conditions at runtime for creating roll objects of different types. Now think of other similar classes where this roll instantiation might be needed.
There can be a class in our apps such as RollsMenu where we need to get rolls for their description and price. What we will have to do in that case? Obviously,
Code Duplication
Code duplication is not good for the developer’s health 💀
We will try to apply the learnings from our very first Strategy Pattern. We talked something about encapsulating the things that can change frequently. What if we can have a class that is just responsible for creating pizza objects (God! I miss pizza. Sorry for the typo. I am talking about rolls.) 😢 based on the type? It seems like a good solution. Let us write the code to understand this as well
public class RollingTaste {
RollFactory factory;
public RollingTaste(RollFactory factory){
this.factory = factory;
}
Roll orderRoll(String type){
Roll roll;
roll = factory.createRoll(type);
roll.prepare();
roll.pack();
return roll;
}
}
Now, we will see what’s RollFactory is,
public class RollFactory {
public Roll createRoll(String type){
Roll roll=null;
if(type.equals('chicken')){
roll = new ChickenRoll();
}
else if(type.equals('egg')){
roll = new EggRoll();
}
else if(type.equals('veg')){
roll = new VegRoll();
}
return roll;
}
}
So we delegated the responsibility of creating roll objects to the RollFactory class instead of having it in multiple places. If we look closely we still need to change the code if we want to introduce a new Roll type but now the change will be only in one place.
Congratulations 🤟! We have implemented the Simple Factory which is not a design pattern. It’s more of a programming idiom.
Wait what? why did we do all this if its not even a design pattern? Everything was fine already 😩
Well, this was necessary for understanding what’s coming up next 😊. Again please go through the references for a better understanding and more examples, and please don’t hate me if you find anything wrong or incomplete🥺 . I am trying to do better ❤️
References:
- https://www.codeproject.com/Articles/1131770/Factory-Patterns-Simple-Factory-Pattern
- https://stackoverflow.com/questions/27007107/how-to-apply-simple-factory-pattern-java
Delegating work is an underrated skill was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Omkar Nath Mandal
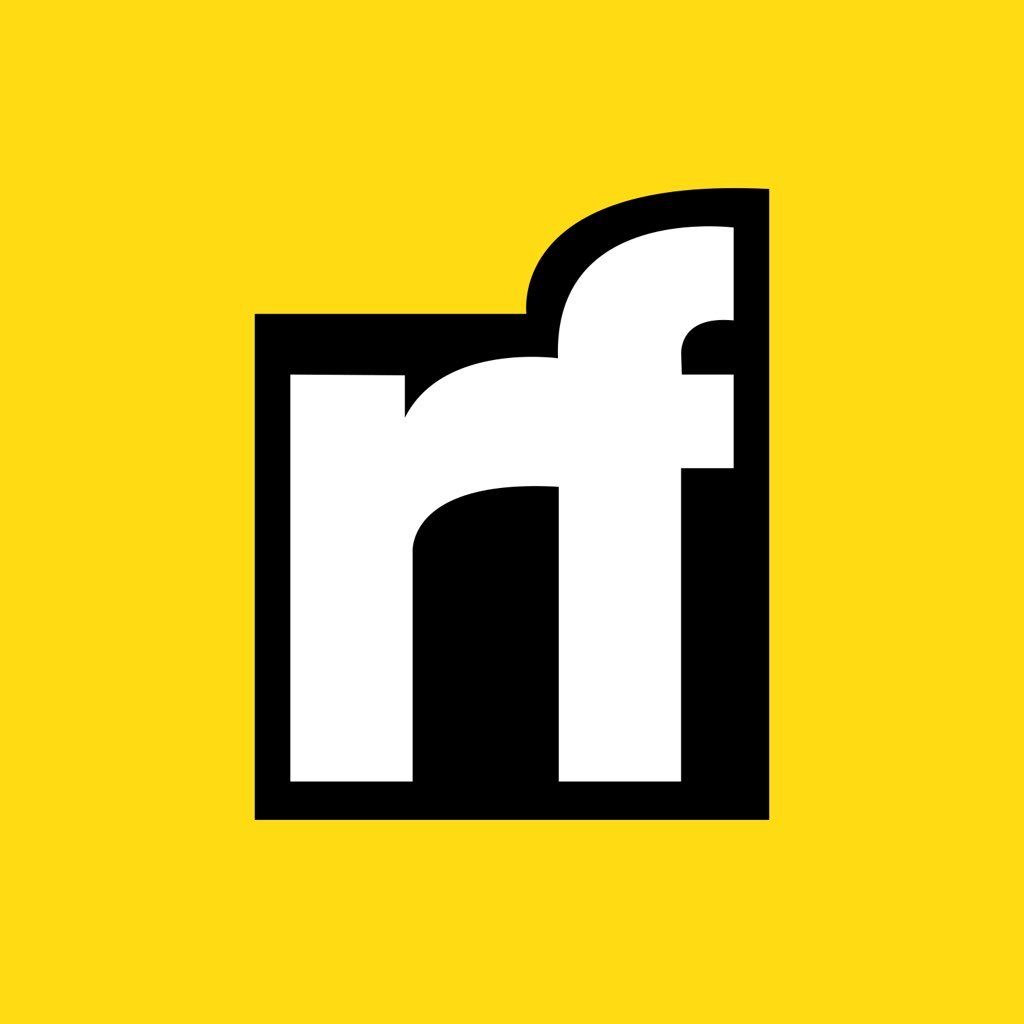
Omkar Nath Mandal | Sciencx (2023-01-01T23:58:39+00:00) Delegating work is an underrated skill. Retrieved from https://www.scien.cx/2023/01/01/delegating-work-is-an-underrated-skill/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.