This content originally appeared on Blog and was authored by Blog
GSAP is a framework-agnostic animation library, that means that you can write the same GSAP code in React, Vue, Angular or whichever framework you chose, the core principles won't change.
That being said - there are some React-specific tips and techniques that will make your life easier!
Need some extra help? Hit up the Reactiflux community for expert advice.
Starter templates
- Simple CodePen starter
- ScrollTrigger and React
- ScrollSmoother and React
- Page Transition example
- Complete StackBlitz Collection
The game-changer - gsap.context()
Proper animation cleanup is crucial to avoid unexpected behaviour with React 18's strict mode. This pattern follows React's best practices.
gsap.context
makes cleanup nice and simple, all GSAP animations and ScrollTriggers created within the function get collected up so that you can easily revert()
ALL of them at once.
Here's the structure:
const comp = useRef(); // create a ref for the root level element (for scoping) const circle = useRef(); useLayoutEffect(() => { // create our context. This function is invoked immediately and all GSAP animations and ScrollTriggers created during the execution of this function get recorded so we can revert() them later (cleanup) let ctx = gsap.context(() => { // Our animations can use selector text like ".box" // this will only select '.box' elements that are children of the component gsap.to(".box", {...}); // or we can use refs gsap.to(circle.current, { rotation: 360 }); }, comp); // <- IMPORTANT! Scopes selector text return () => ctx.revert(); // cleanup }, []); // <- empty dependency Array so it doesn't re-run on every render // ...
In this example, React will first render the box and circle elements to the DOM, then GSAP will rotate them 360deg. When this component un-mounts, the animations are cleaned up using ctx.revert()
.
Blog Posts
These articles take a deep dive into some best practises and techniques for using GSAP in a React codebase.
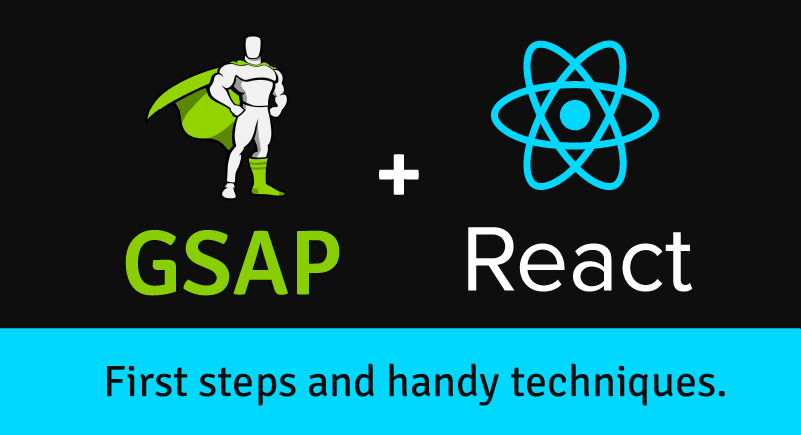
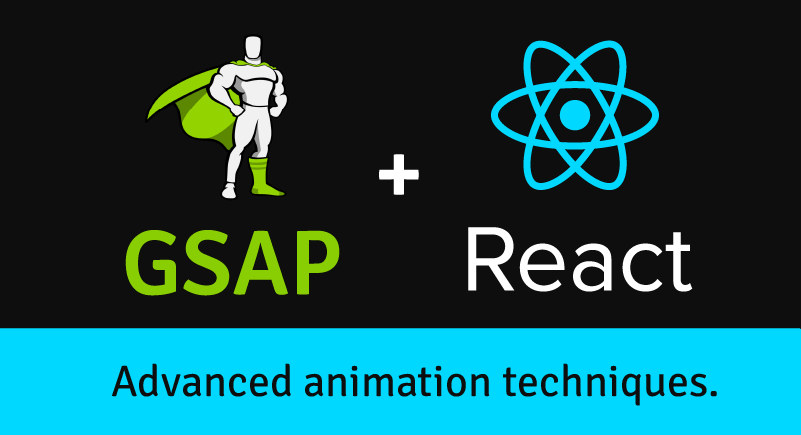
This content originally appeared on Blog and was authored by Blog
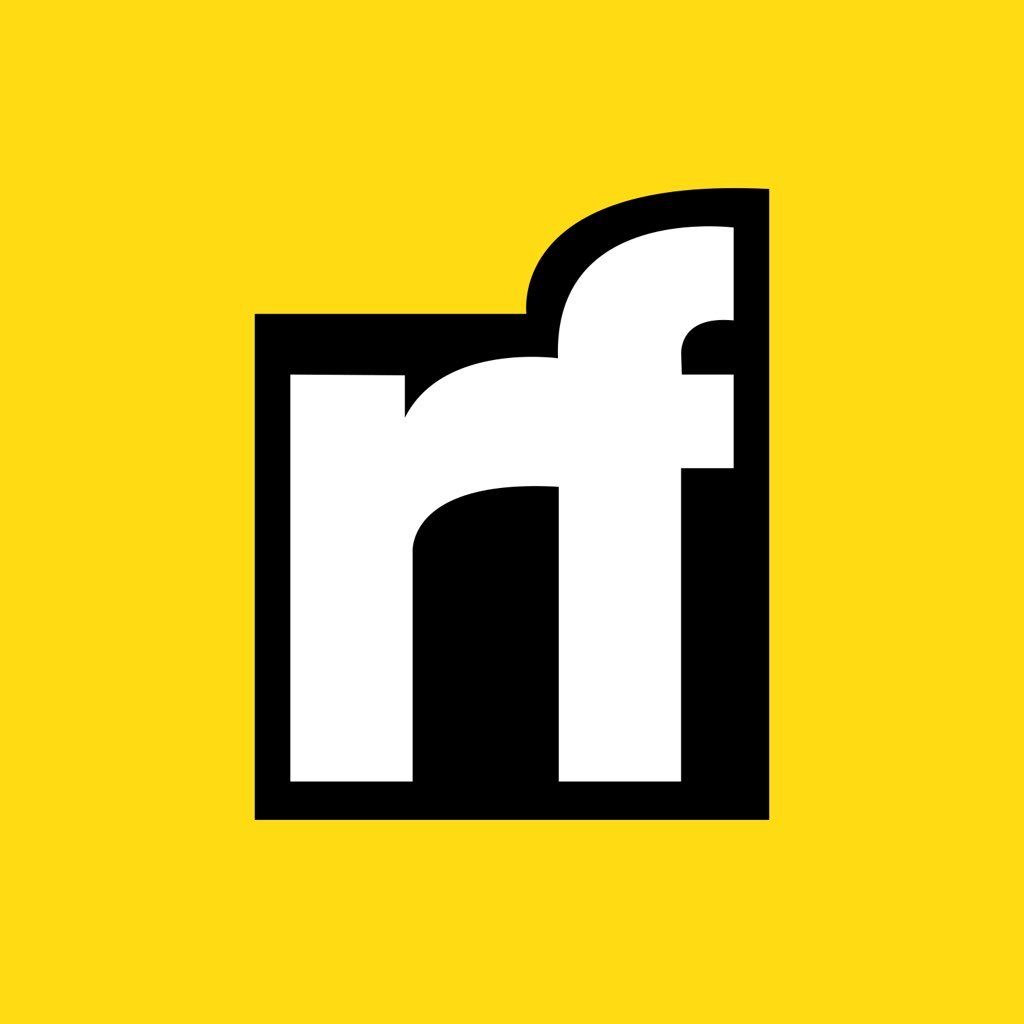
Blog | Sciencx (2023-02-23T15:23:00+00:00) GSAP X React. Retrieved from https://www.scien.cx/2023/02/23/gsap-x-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.