This content originally appeared on Level Up Coding - Medium and was authored by Shamaz Saeed
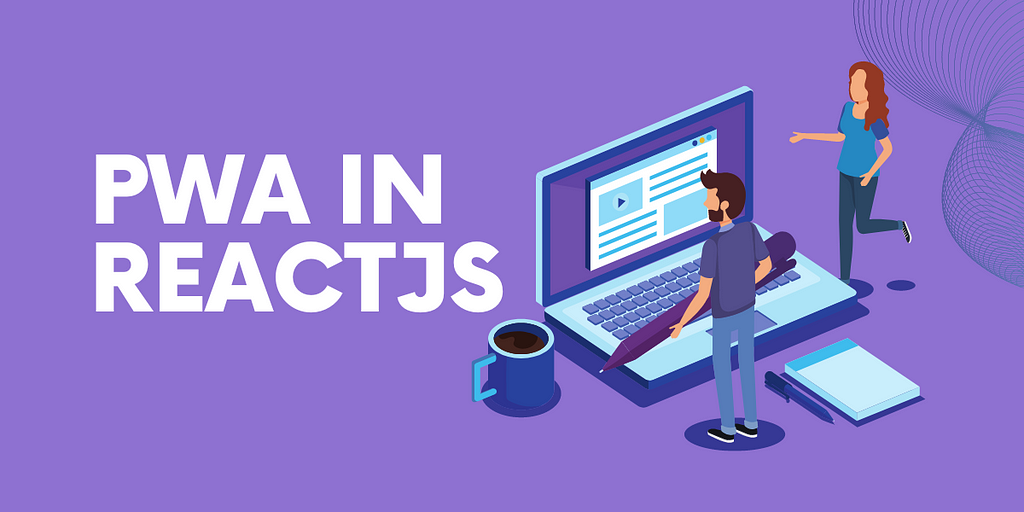
Progressive Web Applications (PWAs) have become increasingly popular as they provide users with a native app-like experience while still being delivered via the web. With the use of modern web technologies, PWAs can be fast, reliable, and engaging, and can work offline or on low-quality networks. In this article, we’ll explore how to build a PWA with ReactJS and how to take advantage of the PWA features provided by modern web browsers.
What is a Progressive Web Application?
A Progressive Web Application is a web application that is designed to work seamlessly on any device or platform, regardless of the network condition. PWAs can be installed on a device’s home screen, providing users with quick access to the app and a seamless experience similar to native mobile applications. PWAs can also work offline or on a low-quality network, thanks to modern web technologies such as Service Workers, Web App Manifests, and IndexedDB.
Advantages of PWAs over traditional web applications:
- Native app-like experience
- Faster loading times and better performance
- Can work offline or on low-quality networks
- Easier to develop and deploy than native apps
- No need to download from an app store
- Can be discovered by search engines
Building a Progressive Web Application with ReactJS:
To build a ReactJS PWA, we need to add the PWA features to the ReactJS project. This can be done by following these steps:
- Setting up a ReactJS project: We can set up a ReactJS project using popular tools like Create React App or Next.js. Once the project is set up, we can add the PWA features to it.
- Adding PWA features to a ReactJS project: To add PWA features to a ReactJS project, we need to create a Web App Manifest file and a Service Worker file. The Web App Manifest file provides metadata about the application, such as the name, icons, and theme color, and is used by the browser to install the application to the device’s home screen. The Service Worker file is a JavaScript file that intercepts network requests and allows the application to work offline or on a low-quality network.
Here’s an example of a simple Web App Manifest file:
{
"name": "My PWA",
"short_name": "My PWA",
"start_url": "/",
"background_color": "#ffffff",
"theme_color": "#2196f3",
"display": "standalone",
"icons": [
{
"src": "icon-192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
And here’s an example of a simple Service Worker file :
const CACHE_NAME = 'my-pwa-cache';
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open(CACHE_NAME)
.then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/app.js'
]);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request)
.then((response) => {
if (response) {
return response;
}
return fetch(event.request);
})
);
});
This Service Worker file intercepts all network requests and checks if the requested resource is available in the cache. If the resource is available in the cache, the Service Worker returns the cached resource. If the resource is not available in the cache, the Service Worker fetches it from the network and caches it for future requests.
Optimizing a ReactJS PWA for performance and user experience: To optimize a ReactJS PWA for performance and user experience, we can use the following techniques:
- Caching and serving assets with service workers: Service Workers can cache assets like HTML, CSS, JavaScript, and images, which allows the application to load faster on subsequent visits. We can also use a technique called “cache-first” to serve cached assets before trying to fetch them from the network.
- Lazy-loading components and images: Lazy-loading is a technique that delays the loading of non-critical resources, such as images or components that are not visible above the fold. This technique can improve the initial loading time of the application.
- Optimizing the critical rendering path: The critical rendering path is the series of steps the browser takes to render a web page. We can optimize the critical rendering path by minimizing the number of requests, reducing the size of resources, and using techniques like server-side rendering.
- Using web APIs to enhance user experience: Modern web browsers provide many APIs that allow us to enhance the user experience of PWAs, such as the Geolocation API, the Push API, and the Web Notifications API.
Deploying a ReactJS PWA:
To deploy a ReactJS PWA, we can use popular hosting platforms like Netlify, Firebase Hosting, or GitHub Pages. We can also use tools like Google’s Lighthouse to check if our PWA meets the requirements for being installable and discoverable.
Conclusion:
Building a Progressive Web Application with ReactJS can provide users with a native app-like experience while still being delivered via the web. By following the steps outlined in this article, you can create a fast, reliable, and engaging PWA that works offline or on low-quality networks.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Building Progressive Web Applications with ReactJS: A Comprehensive Guide was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Shamaz Saeed
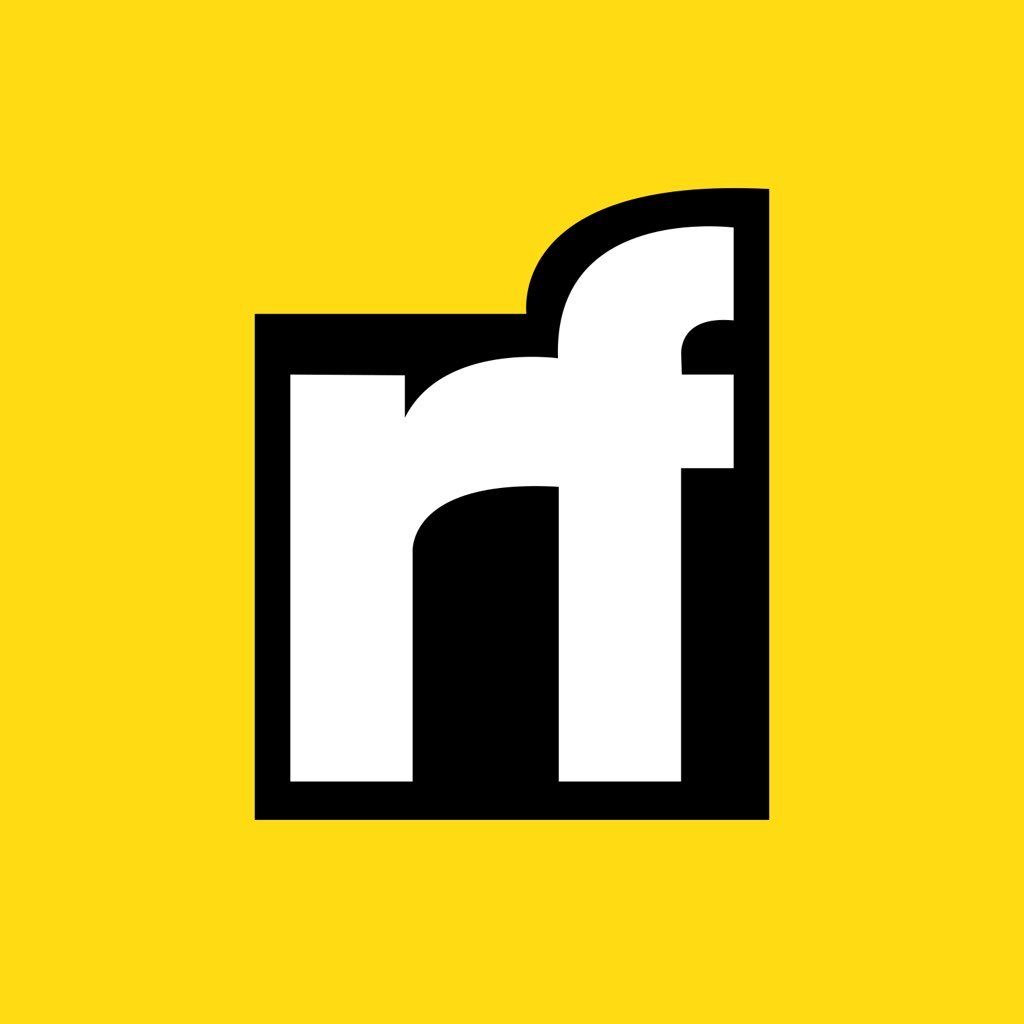
Shamaz Saeed | Sciencx (2023-03-01T03:04:17+00:00) Building Progressive Web Applications with ReactJS: A Comprehensive Guide. Retrieved from https://www.scien.cx/2023/03/01/building-progressive-web-applications-with-reactjs-a-comprehensive-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.