This content originally appeared on Level Up Coding - Medium and was authored by Artiom Khalilyaev
Get to Know Your iOS Device: A Swift Guide to Retrieving Device Information
Device info is very useful data in any mobile app. It can be used for observing battery state, volume level, brightness etc. and notifying users when some of those values changed. For example, in food delivery app you can show an alert if volume level is too low, so the courier won’t miss notifications about new orders. Almost every app uses device info for analytics. It helps to be aware of what iOS version users have and what devices they use. Such data can be helpful in case you want to upgrade your app minimum iOS version.
To get device info, you can use UIDevice class, that provides information about current device and its battery state. Another data like volume level or brightness are stored into other classes. In this article, I will show what data you can get using native API provided by Apple. And we will take a look at one great third-party library, that helps to get device info with ease.
UIDevice
UIDevice is a class from native UIKit library. It can provide you with information about your phone like its name, OS version, model, battery level and state, proximity level, and orientation. It also gives you an opportunity to manage battery, proximity, and orientation notifications.
To get access to all this information, you need to access your current device first. It can be achieved with help of current class property of the UIDevice.
UIDevice.current
To get information about current device, its model, OS and OS version, you can use next properties:
UIDevice.current.name
UIDevice.current.model
UIDevice.current.systemName
UIDevice.current.systemVersion
UIDevice.current.name represents the name of the device, for iOS 15 and earlier it returns a user-assigned device name, like "Artem’s iPhone 13 Pro" Max. For iOS 16.0 and later versions, it returns a generic name like “iPhone”or “iPad”.
UIDevice.current.model represents the type of the current device. For any iPhone it will be “iPhone”, for iPads it will be “iPad” and so on. If you need more specific information, like “iPhone 13 Pro Max” you need to use another approach. We will talk about it later in this article.
UIDevice.current.systemName represents the OS running on the current device, for example for any iPhone it will be “iOS”. UIDevice.current.systemVersion represents version of the OS, like 16.2.
Battery Info
UIDevice also provides us with information about battery. First, isBatteryMonitoringEnabled needs to be enabled, if you want to get some battery related info. You can enable it, by just setting its value to true.
UIDevice.current.isBatteryMonitoringEnabled = true
To get current battery level, the batteryLevel property can be used. It returns a value in a range from 0 to 1.0, so you need to multiply it by 100 if you want to get a percentage value. If isBatteryMonitoringEnabled is false, you will get -1.0.
batteryState property represents current battery state of the device. It’s an enumeration with 4 possible cases:
- unknown The battery state for the device can’t be determined;
- unplugged The device isn’t plugged into power; the battery is discharging;
- charging The device is plugged into power and the battery is less than 100% charged;
- full The device is plugged into power and the battery is 100% charged.
Also, UIDevice has two notifications allowing us to observe battery level and state changes. They are UIDevice.batteryLevelDidChangeNotification and UIDevice.batteryStateDidChangeNotification respectively. You can set up observers using NotificationCenter like this:
NotificationCenter.default.addObserver(self,
selector: #selector(didChangeBatteryLevel(_:)),
name: UIDevice.batteryLevelDidChangeNotification,
object: nil)
NotificationCenter.default.addObserver(self,
selector: #selector(didСhangeBatteryState(_:)),
name: UIDevice.batteryStateDidChangeNotification,
object: nil)
@objc private func didChangeBatteryLevel(_ notification: Notification) {
// Check battery level and perform some actions
}
@objc private func didСhangeBatteryState(_ notification: Notification) {
// Check battery state and perform some actions
}
Orientation
The last property from UIDevice we will talk about today is the device orientation. There is an enumeration UIDeviceOrientation with 6 possible cases:
- unknown The orientation of the device can’t be determined;
- portrait The device is in portrait mode, with the device held upright and the front-facing camera at the top;
- portraitUpsideDown The device is in portrait mode but upside down, with the device held upright and the front-facing camera at the bottom;
- landscapeLeft The device is in landscape mode, with the device held upright and the front-facing camera on the left side;
- landscapeRight The device is in landscape mode, with the device held upright and the front-facing camera on the right side;
- faceUp The device is held parallel to the ground with the screen facing upwards;
- faceDown The device is held parallel to the ground with the screen facing downwards.
To get current device orientation, you can use the orientation property of the UIDevice. UIDeviceOrientation also has a set of boolean properties that help to determine current orientation. They are isFlat, isPortrait, and isLandscape. Here is an example:
UIDevice.current.orientation
UIDevice.current.orientation.isFlat
UIDevice.current.orientation.isPortrait
UIDevice.current.orientation.isLandscape
Same as with a battery, we can observe orientation changes with orientationDidChangeNotification notification. But to be able to receive notifications, we need to enable this feature first. To do so, you need to use beginGeneratingDeviceOrientationNotifications() method. To stop generating orientation notifications, use endGeneratingDeviceOrientationNotifications() method.
UIDevice.current.beginGeneratingDeviceOrientationNotifications()
NotificationCenter.default.addObserver(self,
selector: #selector(didСhangeOrientation(_:)),
name: UIDevice.orientationDidChangeNotification,
object: nil)
// Call when you don't need orientation notifications
UIDevice.current.beginGeneratingDeviceOrientationNotifications()
@objc private func didСhangeOrientation(_ notification: Notification) {
// Check orientation and perform some actions
}
Brightness
To get current device brightness and ability to observe its changes, you need to use UIScreen class and its properties and notifications. To get current device brightness, you need to use brightness property. There is also a notification for brightness changes UIScreen.brightnessDidChangeNotification.
UIScreen.main.brightness
NotificationCenter.default.addObserver(self,
selector: #selector(didСhangeBrightness(_:)),
name: UIScreen.brightnessDidChangeNotification,
object: nil)
@objc private func didСhangeBrightness(_ notification: Notification) {
// Check brightness and perform some actions
}
Device Marketing Model
By device marketing model, I mean something like iPhone 12 Pro Max or iPhone 8 Plus. UIDevice does not provide us with such data, but there is still a way to get it. Using native iOS SDK, we can use current device code. Device code isn’t equal to marketing model, but when we have a code, we can map it into marketing model. Using the following function, you can get your current device code:
func getDeviceCode() -> String? {
var systemInfo = utsname()
uname(&systemInfo)
let modelCode = withUnsafePointer(to: &systemInfo.machine) {
$0.withMemoryRebound(to: CChar.self, capacity: 1) {
ptr in String.init(validatingUTF8: ptr)
}
}
return modelCode
}
If you run this function on simulator, you will get one of the following values: “i386”, “x86_64”, or “arm64”. And on real device, you will get a device code. I have 2 working devices and one personal. For my working iPhone 12 device code is “iPhone13,2”, for iPhone 7 Plus it’s “iPhone9,4” and for iPhone XS Max it’s “iPhone11,6”. As you can see, device code is kinda confusing. The only way to map device code into a marketing model is to have a hardcoded dictionary with all device codes and marketing models for them. If you don’t want to hardcode it yourself, you can use a third-party library DeviceKit. Let’s take a look at it.
DeviceKit
DeviceKit is a lightweight third-party library, that easily allows you to get information about device and its properties. Here is the link for its GitHub repository.
It allows you to get device model, battery state, brightness, available disk space, and other additional info about your device. If you check its source code, you will find out most of the values are hardcoded, but this is a popular and trusted library. It has more than 4000 stars on GitHub, and it always stays up to date, so fell free to use it in your project if you want to get all info about your device from one place.
DeviceKit uses an enum for device types, and this enum is called Device. You can access your current device through Device.current. Since Device is an enum, you can easily use it with == operator in if-else blocks or within switch statements.
let currentDevice = Device.current
if currentDevice == .iPhone12Pro {
// Do something
}
switch currentDevice {
case .iPhone12:
//Do something
case .iPhone4, .iPhone5:
//Do something
default:
//Do something
}
It provides an opportunity to determine device family and is it a simulator or not. Also, if the current device is a simulator, you can determine what simulator is it.
if currentDevice.isPod {
// iPods (real or simulator)
} else if currentDevice.isPhone {
// iPhone (real or simulator)
} else if currentDevice.isPad {
// iPad (real or simulator)
}
if currentDevice.isSimulator {
// Do something for simulator
}
if case .simulator(.iPhone12) = currentDevice {
// Do something for iPhone 12 simulator
}
Battery info is also provided by the DeviceKit. To get a battery level, you can use batteryLevel property. DeviceKit uses Int instead of Float for battery level, so no need to multiply its value by 100 to get value in percentages.
currentDevice.batteryLevel
You can get current battery state, DeviceKit has a BatteryState enum, that provides you with more data, than default UIDevice batteryState enum.
switch currentDevice.batteryState {
case .full:
print("Your dive is fully charged")
case .charging(let batteryLevel):
print("Device is charging, currently at \(batteryLevel)%")
case .unplugged(let batteryLevel):
print("The device is not plugged into power; currently at \(batteryLevel)%")
default:
break
}
BatteryState enum has a lowPowerMode boolean property, that determines whenever lower power mode is on.
if currentDevice.batteryState.lowPowerMode {
print("Low Power mode is enabled")
} else {
print("Low Power mode is disabled")
}
DeviceKit has an info about current brightness inside too. The beauty is that you don’t need to use UIScreen for brightness. With DeviceKit all device related data is stored in one place. To get brightness, you need to access screenBrightness property. Same as a batteryLevel, this property is an Int.
if currentDevice.screenBrightness > 50 {
print("Take care of your eyes!")
}
In this last paragraph, we will take a look at properties which represent disk space. They are:
- volumeTotalCapacity, the volume’s total capacity in bytes;
- volumeAvailableCapacity, the volume’s available capacity in bytes;
- volumeAvailableCapacityForImportantUsage, the volume’s available capacity in bytes for storing important resources;
- volumeAvailableCapacityForOpportunisticUsage, the volume’s available capacity in bytes for storing non-essential resources.
This data can be used when you need to check available disk space before downloading huge amount of data.
DeviceKit provides a lot of more data, than I mentioned in this article. It provides info about Apple Pencil support, camera, thermal state, CPU, ppi, etc. But for me, the main benefit is that all data is stored in one place and can be accessed through Device.current.
Device info is very useful data in every iOS app and everybody should know how to get it. You can find links for all resources below.
Resources
Apple Documentation: UIDevice class
Apple Documentation: UIScreen class
DeviceKit GitHub repository
Thanks for your time. I hope you found this article useful. Feel free to share your opinion in the comments.
Check out my other articles about iOS Development
https://medium.com/@artem.khalilyaev
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Device Information In Swift was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Artiom Khalilyaev
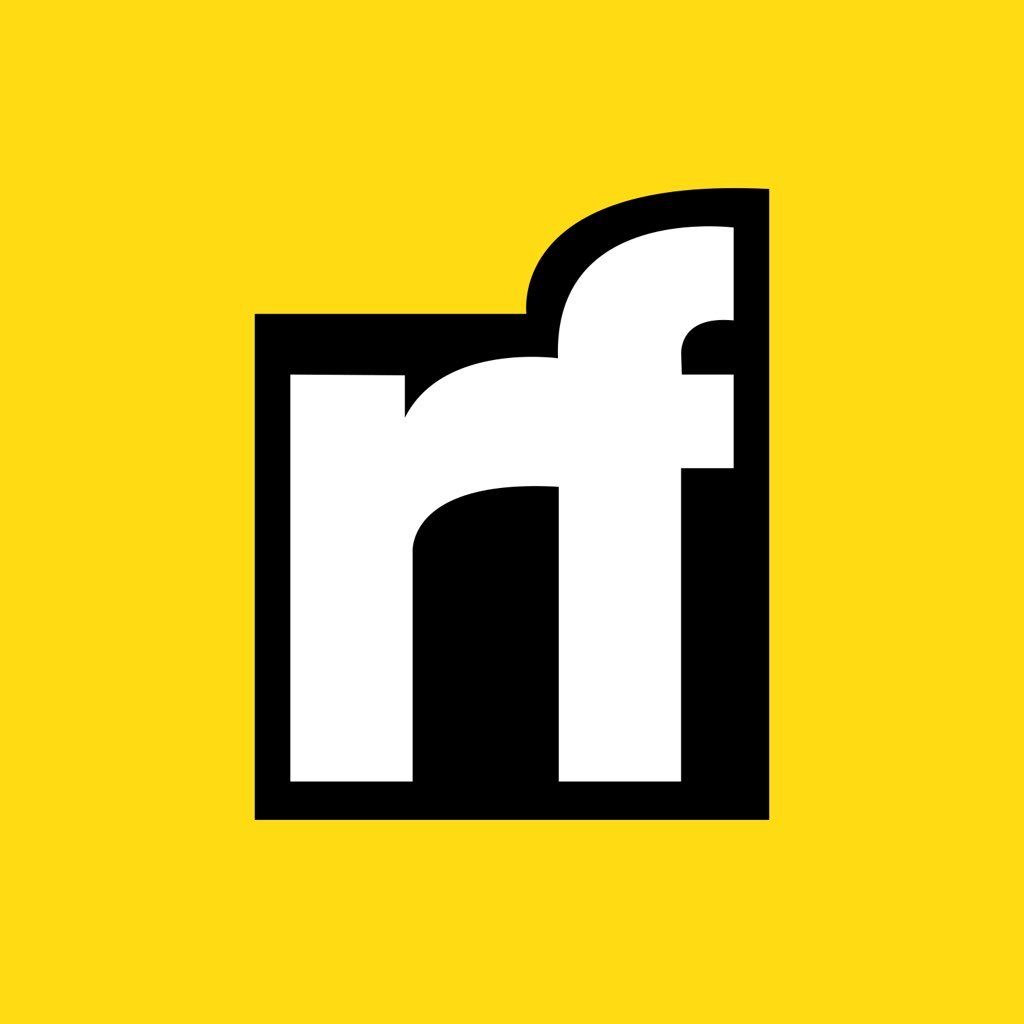
Artiom Khalilyaev | Sciencx (2023-03-14T15:25:35+00:00) Device Information In Swift. Retrieved from https://www.scien.cx/2023/03/14/device-information-in-swift/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.